
# COF Styling
You can also add your own styles for the card on file checkbox and for the card agreement.
# Checkbox
To style the checkbox, you can use regular payment form flow where you will need to pass CSS rules in customCss
object in the mount options when calling collect.mount
.
Code Sample:
const poyntCollect = document.createElement("script");
poyntCollect.src = "https://cdn.poynt.net/collect.js";
poyntCollect.async = true;
poyntCollect.onload = () => {
const collect = new TokenizeJs(
"business-uuid",
"urn:aid:application-uuid"
);
collect.mount("collect-container", document, {
...
customCss: {
cardOnFile: {
container: {
"width": "100%",
},
wrapper: {
"height": "100%",
},
checkbox: {
"color": "red",
},
label: {
"text-align": "left",
},
agreement: {
"background-color": "#000",
},
link: {
"text-decoration": "none",
},
},
},
enableCardOnFile: true,
cardAgreementOptions: {
businessName: "Example",
businessWebsite: "https://www.example.com/",
businessPhone: "(555) 555-5555",
},
});
};
document.head && document.head.appendChild(poyntCollect);
# Fields & HTML Elements
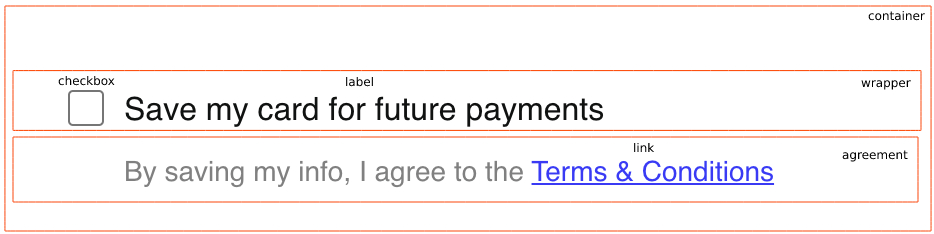
# Card Agreement
To style the card agreement modal you can pass additional fields to cardAgreementOptions
in the mount options when calling collect.mount
.
Code Sample:
const poyntCollect = document.createElement("script");
poyntCollect.src = "https://cdn.poynt.net/collect.js";
poyntCollect.async = true;
poyntCollect.onload = () => {
const collect = new TokenizeJs(
"business-uuid",
"urn:aid:application-uuid"
);
collect.mount("collect-container", document, {
...
enableCardOnFile: true,
cardAgreementOptions: {
businessName: "Example",
businessWebsite: "https://www.example.com/",
businessPhone: "(555) 555-5555",
style: {
globalContainer: {
"width": "100%",
},
agreementContainer: {
"height": "100%",
},
title: {
"font-weight": "700",
},
closeIconContainer: {
"margin-left": "10px",
},
closeIcon: {
"font-size": "20px",
},
mainText: {
"font-style": "italic",
},
businessNameText: {
"font-weight": "400",
},
businessWebsiteLink: {
"text-decoration": "none",
},
businessPhoneText: {
"font-weight": "400",
},
actionButtonsContainer: {
"display": "block",
},
acceptButton: {
"padding": "20px 40px",
},
declineButton: {
"padding": "20px 40px",
},
},
},
});
};
document.head && document.head.appendChild(poyntCollect);
# Fields & HTML Elements
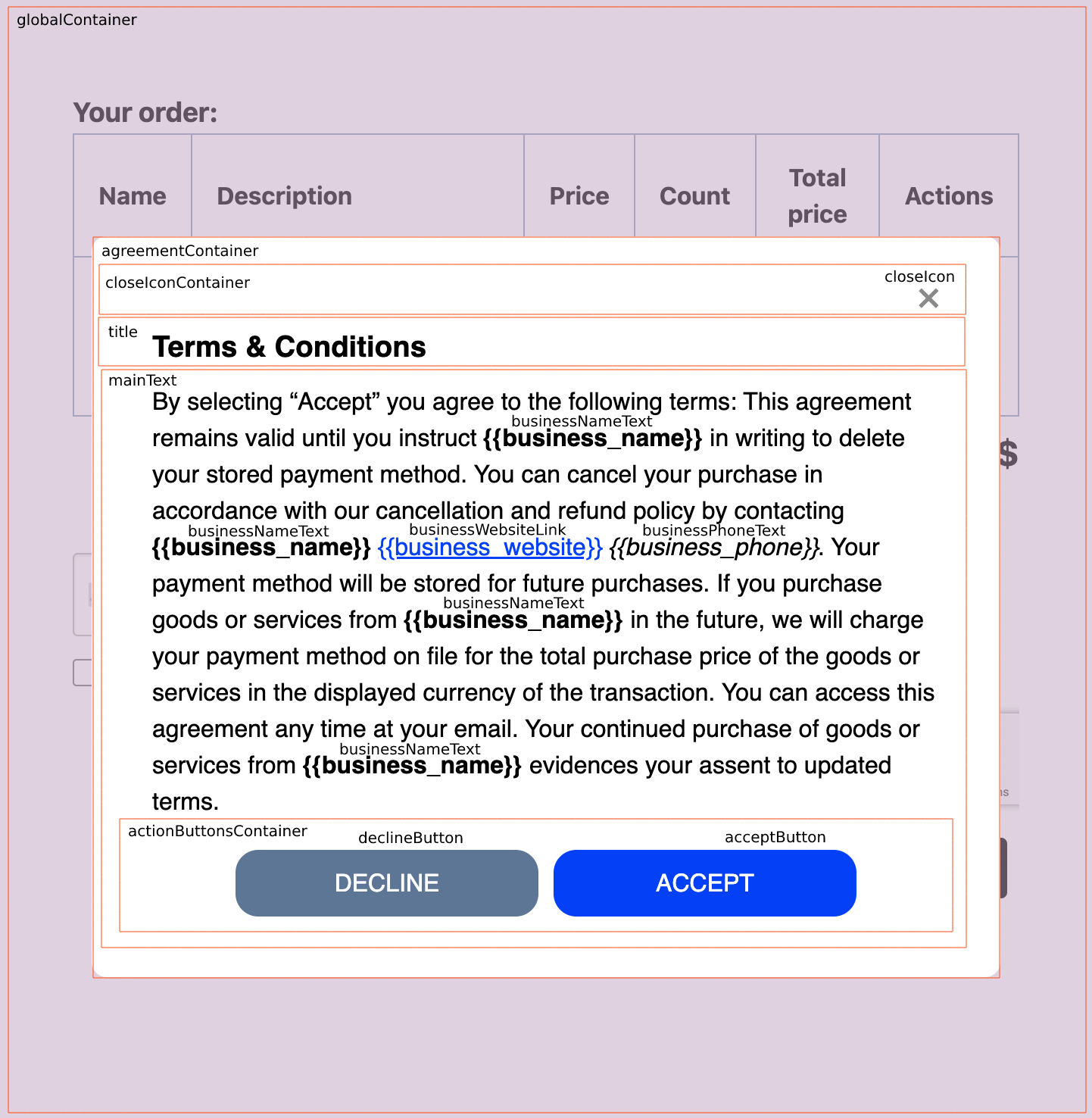