Poynt API
Just install the Poynt Node.js SDK (GitHub) and you are ready to go!
npm install poynt --save
Just install the Poynt Python SDK (GitHub) and you are ready to go!
pip install poynt
Introduction
Poynt REST APIs provide applications an ability to integrate with Poynt Services in the cloud.
Standards
All of GoDaddy Poynt’s APIs are built using RESTful design patterns that are very common in the industry. In this page, you will find all the Services and Entities exposed as Resources that you can access through standard HTTP semantics. HTTP status codes are used to communicate the success/failures states, OAuth2.0 is used for API Authentication and Authorization, and HTTP methods are used to operate on the resources.
Please take note of the common patterns listed below that would help in simplifying your integration process.
Endpoint
All of our APIs can be accessed at:
https://services.poynt.net
.
HTTP Headers
All APIs should be invoked with the following standard HTTP headers unless otherwise specified:
Api-Version
- Must be1.2
Authorization
-Bearer <poynt-issued-access-token>
Content-Type
- Usuallyapplication/json
POYNT-REQUEST-ID
- Unique request id to enforce idempotency where appropriate (usually for POST requests)
HTTP Status Code
GoDaddy Poynt’s APIs return one or more of the following codes.
200
- The operation was successful.201
- Created, when a new resource is created.204
- No Content, usually returned after deleting a resource.400
- Bad Request401
- Unauthorized, due to invalid access token or authorization needed.403
- Forbidden404
- Not Found500
- Internal Server Error
Dates/Times
All date time values are in GMT and is formatted in ISO 8601 format
YYYY-MM-DDThh:mm:ssZ
.
Resource Updates
The HTTP PATCH method is used to allow updates to existing Resources
using json-patch as
the request payload. Currently only the add
,
remove
, and replace
operations are
supported.
Pagination
Pagination is controlled by five parameters for most GET collection APIs. These parameters determine the result set and allow you to paginate through them.
If-Modified-Since
header- ISO8601 Time to filters result by item’s last updated time.
startAt
query- ISO8601 Time to filters result by a specific start time.
startOffset
query- Integer offset from first item.
endAt
query- ISO8601 Time to filters result by a specific end time.
limit
query- Integer value 1 to 100 to limit the number of items returned. Default is 10.
Clients are encouraged to begin paging through a collection by
specifying the If-Modified-Since
header at the start of
their paging request. A limit
should also be specified to
control the number of returned items. If there are more items than can
be returned, a HATEOAS link will also be returned.
If, however, the client wishes to specify a specific time window,
they should use the startAt
/endAt
time window.
This time window will be used to filter items out based on the item’s
updatedAt
time. When startAt
is specified, the
server will ignore the If-Modified-Since
header. If no
endAt
time is specified, it is assumed to be up to the
latest time (now).
Clients should generally not need to use the startOffset
parameter. This parameter is used by the server to control the paging
range in the HATEOAS links that it generates.
Oauth
Poynt APIs are secured with OAuth 2.0 to allow merchants to share data with developer applications as appropriate. The Poynt OAuth2.0 authorization API consist of a single token resource (end point) where client applications can obtain access tokens that can be used to invoke all other APIs.
Definition
POST /token
Sample Request
curl -H "api-version:1.2" -d "grantType=urn:ietf:params:oauth:grant-type:jwt-bearer&assertion=MY.SELFSIGNED.JWT" https://services.poynt.net/token
curl -H "api-version:1.2" -H "Authorization: MY.SELFSIGNED.JWT" -d "grantType=PASSWORD&businessId=d4b4ec82-0b3a-49c9-8424-1a6c16e3562a&username=Kelly&password=1234" https://services.poynt.net/token
Sample Response
{
"expiresIn":900,
"accessToken":"POYNT.ISSUED.JWT",
"refreshToken":"REFRESH_TOKEN",
"scope":"ALL",
"tokenType":"BEARER"
}
Definition
In the Node.js SDK, token generation and refresh is done
automatically behind the scenes. Simply initialize the
poynt
service with your application ID and either a
filename or a string containing your PEM-encoded private key you
downloaded from Poynt.net:
var poynt = require('poynt')({
applicationId: 'urn:aid:your-application-id',
filename: __dirname + '/key.pem'
; })
or
var poynt = require('poynt')({
applicationId: 'urn:aid:your-application-id',
key: '-----BEGIN RSA PRIVATE KEY-----\n.....\n-----END RSA PRIVATE KEY-----'
; })
Definition
In the Python SDK, token generation and refresh is done
automatically, behind the scenes, and so you don’t have to worry about
it yourself. Simply initialize the poynt
service with your
application ID and either a filename or a string containing your
PEM-encoded private key you downloaded from Poynt.net:
import poynt
= 'urn:aid:your-application-id'
poynt.application_id = '/path/to/your/key.pem' poynt.filename
or
import poynt
= 'urn:aid:your-application-id'
poynt.application_id = '-----BEGIN RSA PRIVATE KEY-----\n.....\n-----END RSA PRIVATE KEY-----' poynt.key
Token
Generate an access token with either Json Web Token (JWT) bearer, password, or refresh token grant type.
The JWT bearer grant requires that the input grantType
form parameter be
urn:ietf:params:oauth:grant-type:jwt-bearer
as defined by
the JWT
Profile for OAuth 2.0 specification. A self-signed JWT token must be
provided by the client that is signed using the client’s private
key.
The password grant requires that a self-signed JWT token be provided
via the Authorization
http header in addition to the
business user’s businessId
, username
, and
password
.
Refresh token is also granted along with Access tokens to allow applications to refresh tokens that have expired. These tokens must always be stored securely by the applications.
Response
Returns a TokenResponse.
Payments
Orders
Orders resource represents a customer’s request to purchase one or more items (goods or services) from a business. As such an order contains details about all the items that we purchased, discounts applied and transactions that paid for the order. An order can be in Opened, Cancelled or Completed states. The only thing these states really tell is whether the order is active from the merchant’s perspective or not. An active order will be in Opened state. An order that the merchant is done with will be in Completed or Cancelled state. Similarly items have their own states (Ordered, Fulfilled and Returned) and transactions their own (see documentation for Transactions). Typically a merchant would want to keep the Order in Opened state until all items in the order have either been Fulfilled or Returned and all payments have been completed.
Definition
GET /businesses/{businessId}/orders
Sample Request
curl --location --request GET 'https://services.poynt.net/businesses/a3f24e9e-6764-42f2-bbcd-5541e7f3be38/orders' \
--header 'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json'
Sample Response
{
"links": [
{
"href": "/businesses/a3f24e9e-6764-42f2-bbcd-5541e7f3be38/orders?startAt=2022-04-27T18%3A05%3A47Z&endAt=2023-02-10T08%3A59%3A39Z",
"rel": "next",
"method": "GET"
}
],
"orders": [
{
"taxExempted": false,
"valid": true,
"accepted": false,
"createdAt": "2022-04-14T04:28:09Z",
"updatedAt": "2022-04-14T04:28:09Z",
"context": {
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"transactionInstruction": "NONE",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"customer": {
"createdAt": "2022-04-14T04:28:02Z",
"updatedAt": "2022-04-14T04:28:02Z",
"id": 369327767,
"emails": {},
"phones": {},
"firstName": "",
"lastName": ""
},
"items": [
{
"taxExempted": false,
"createdAt": "2022-04-14T04:28:09Z",
"updatedAt": "2022-04-14T04:28:09Z",
"quantity": 1,
"id": 543,
"unitPrice": 2300,
"discount": 0,
"fee": 0,
"tax": 0,
"status": "ORDERED",
"name": "itemA",
"unitOfMeasure": "EACH"
}
],
"transactions": [
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-14T04:28:02Z",
"updatedAt": "2022-04-14T04:28:02Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-14T04:28:01Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2023,
"id": 369309072,
"numberFirst6": "405413",
"numberLast4": "6357",
"numberMasked": "4054136357",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/",
"serviceCode": "201",
"cardId": "6c08a9cc-b0f6-47a6-aa21-b1c85ab50a35"
},
"emvData": {
"emvTags": {
"0x1F8231": "18",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F37": "5384CD17",
"0x5F24": "231231",
"0x9F02": "000000002300",
"0x9F66": "82804000",
"0x5F20": "202F"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "908e5ea6-750c-4ee0-a067-4c749308ce33",
"type": "POYNT_ORDER"
}
],
"customerUserId": 369327767,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"approvedAmount": 2300,
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "265403b5-0180-1000-3394-854eaade8985",
"approvalCode": "188879",
"batchId": "1",
"retrievalRefNum": "265403b5-0180-1000-3394-854eaade8985"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 2300,
"orderAmount": 2300,
"tipAmount": 0,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "AUTHORIZED",
"id": "265403b5-0180-1000-3394-854eaade8985"
}
],
"customerUserId": 369327767,
"amounts": {
"subTotal": 2300,
"discountTotal": 0,
"feeTotal": 0,
"taxTotal": 0,
"netTotal": 2300,
"currency": "USD",
"authorizedTotals": {
"transactionFees": [],
"transactionAmount": 2300,
"orderAmount": 2300,
"tipAmount": 0,
"cashbackAmount": 0,
"currency": "USD"
}
},
"statuses": {
"fulfillmentStatus": "NONE",
"status": "OPENED",
"transactionStatusSummary": "PENDING"
},
"notes": "",
"id": "908e5ea6-750c-4ee0-a067-4c749308ce33"
},
{
"taxExempted": false,
"valid": true,
"accepted": false,
"createdAt": "2022-04-14T20:31:23Z",
"updatedAt": "2022-04-14T20:32:22Z",
"context": {
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"transactionInstruction": "NONE",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"customer": {
"createdAt": "2022-04-14T20:31:06Z",
"updatedAt": "2022-04-14T20:31:06Z",
"id": 369458304,
"emails": {},
"phones": {},
"firstName": "",
"lastName": ""
},
"items": [
{
"taxExempted": false,
"createdAt": "2022-04-14T20:31:23Z",
"updatedAt": "2022-04-14T20:31:23Z",
"quantity": 1,
"id": 543,
"unitPrice": 2500,
"discount": 0,
"fee": 0,
"tax": 0,
"status": "ORDERED",
"name": "itemA",
"unitOfMeasure": "EACH"
}
],
"transactions": [
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": true,
"amountsAdjusted": true,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-14T20:31:06Z",
"updatedAt": "2022-04-14T20:32:22Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-14T20:31:05Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2023,
"id": 369442365,
"numberFirst6": "405413",
"numberLast4": "6357",
"numberMasked": "4054136357",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/",
"serviceCode": "201",
"cardId": "885f6b75-5e8f-4933-a55d-cc53a8dda6e9"
},
"emvData": {
"emvTags": {
"0x1F8231": "18",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F37": "2F9BD81B",
"0x5F24": "231231",
"0x9F02": "000000002500",
"0x9F66": "82804000",
"0x5F20": "202F"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "71241c6f-ff98-46b2-879f-1805596465ca",
"rel": "CAPTURE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "38caea72-3965-48af-9dfe-ab81145abe4e",
"type": "POYNT_ORDER"
}
],
"customerUserId": 369458304,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"approvedAmount": 2500,
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "29c5b96d-0180-1000-d4b4-c6fb481f142d",
"approvalCode": "219475",
"batchId": "1",
"retrievalRefNum": "29c5b96d-0180-1000-d4b4-c6fb481f142d"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 7500,
"orderAmount": 2500,
"tipAmount": 5000,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "29c5b96d-0180-1000-d4b4-c6fb481f142d"
},
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": true,
"reversalVoid": false,
"createdAt": "2022-04-14T20:32:22Z",
"updatedAt": "2022-05-11T23:47:31Z",
"context": {
"businessType": "TEST_MERCHANT",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2023,
"id": 369442365,
"numberFirst6": "405413",
"numberLast4": "6357",
"numberMasked": "4054136357",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/",
"serviceCode": "201",
"cardId": "885f6b75-5e8f-4933-a55d-cc53a8dda6e9"
},
"emvData": {
"emvTags": {
"0x1F8231": "18",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F37": "2F9BD81B",
"0x5F24": "231231",
"0x9F02": "000000002500",
"0x9F66": "82804000",
"0x5F20": "202F"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "29c5b96d-0180-1000-d4b4-c6fb481f142d",
"rel": "AUTHORIZE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "38caea72-3965-48af-9dfe-ab81145abe4e",
"type": "POYNT_ORDER"
}
],
"customerUserId": 369458304,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "71241c6f-ff98-46b2-879f-1805596465ca",
"approvalCode": "440928",
"batchId": "1"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "SETTLED",
"action": "CAPTURE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 7500,
"orderAmount": 2500,
"tipAmount": 5000,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "71241c6f-ff98-46b2-879f-1805596465ca",
"parentId": "29c5b96d-0180-1000-d4b4-c6fb481f142d"
}
],
"customerUserId": 369458304,
"amounts": {
"subTotal": 2500,
"discountTotal": 0,
"feeTotal": 0,
"taxTotal": 0,
"netTotal": 2500,
"currency": "USD",
"capturedTotals": {
"transactionFees": [],
"transactionAmount": 7500,
"orderAmount": 2500,
"tipAmount": 5000,
"cashbackAmount": 0,
"currency": "USD"
}
},
"statuses": {
"fulfillmentStatus": "NONE",
"status": "OPENED",
"transactionStatusSummary": "COMPLETED"
},
"notes": "",
"id": "38caea72-3965-48af-9dfe-ab81145abe4e"
},
{
"taxExempted": false,
"valid": true,
"accepted": false,
"createdAt": "2022-04-14T20:36:38Z",
"updatedAt": "2022-04-14T20:37:13Z",
"context": {
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"transactionInstruction": "NONE",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"customer": {
"createdAt": "2022-04-14T20:36:25Z",
"updatedAt": "2022-04-14T20:36:25Z",
"id": 369460286,
"emails": {},
"phones": {},
"firstName": "",
"lastName": ""
},
"items": [
{
"taxExempted": false,
"createdAt": "2022-04-14T20:36:38Z",
"updatedAt": "2022-04-14T20:36:38Z",
"quantity": 1,
"id": 543,
"unitPrice": 4000,
"discount": 0,
"fee": 0,
"tax": 0,
"status": "ORDERED",
"name": "itemA",
"unitOfMeasure": "EACH"
}
],
"transactions": [
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": true,
"amountsAdjusted": true,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-14T20:36:25Z",
"updatedAt": "2022-04-14T20:37:13Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-14T20:36:25Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2023,
"id": 369444391,
"numberFirst6": "405413",
"numberLast4": "6357",
"numberMasked": "4054136357",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/",
"serviceCode": "201",
"cardId": "3bf41a39-a5f3-4d2d-b660-70373e607556"
},
"emvData": {
"emvTags": {
"0x1F8231": "18",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F37": "402061F8",
"0x5F24": "231231",
"0x9F02": "000000004000",
"0x9F66": "82804000",
"0x5F20": "202F"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "89e33222-1319-4619-84e4-492a08789452",
"rel": "CAPTURE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "e34dff21-1226-4af2-a42b-ca15275bd9fa",
"type": "POYNT_ORDER"
}
],
"customerUserId": 369460286,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"approvedAmount": 4000,
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "29ca99f3-0180-1000-d4b4-c6fb481f142d",
"approvalCode": "158881",
"batchId": "1",
"retrievalRefNum": "29ca99f3-0180-1000-d4b4-c6fb481f142d"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 5500,
"orderAmount": 4000,
"tipAmount": 1500,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "29ca99f3-0180-1000-d4b4-c6fb481f142d"
},
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": true,
"reversalVoid": false,
"createdAt": "2022-04-14T20:37:13Z",
"updatedAt": "2022-05-11T23:47:31Z",
"context": {
"businessType": "TEST_MERCHANT",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2023,
"id": 369444391,
"numberFirst6": "405413",
"numberLast4": "6357",
"numberMasked": "4054136357",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/",
"serviceCode": "201",
"cardId": "3bf41a39-a5f3-4d2d-b660-70373e607556"
},
"emvData": {
"emvTags": {
"0x1F8231": "18",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F37": "402061F8",
"0x5F24": "231231",
"0x9F02": "000000004000",
"0x9F66": "82804000",
"0x5F20": "202F"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "29ca99f3-0180-1000-d4b4-c6fb481f142d",
"rel": "AUTHORIZE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "e34dff21-1226-4af2-a42b-ca15275bd9fa",
"type": "POYNT_ORDER"
}
],
"customerUserId": 369460286,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "89e33222-1319-4619-84e4-492a08789452",
"approvalCode": "503831",
"batchId": "1"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "SETTLED",
"action": "CAPTURE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 5500,
"orderAmount": 4000,
"tipAmount": 1500,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "89e33222-1319-4619-84e4-492a08789452",
"parentId": "29ca99f3-0180-1000-d4b4-c6fb481f142d"
}
],
"customerUserId": 369460286,
"amounts": {
"subTotal": 4000,
"discountTotal": 0,
"feeTotal": 0,
"taxTotal": 0,
"netTotal": 4000,
"currency": "USD",
"capturedTotals": {
"transactionFees": [],
"transactionAmount": 5500,
"orderAmount": 4000,
"tipAmount": 1500,
"cashbackAmount": 0,
"currency": "USD"
}
},
"statuses": {
"fulfillmentStatus": "NONE",
"status": "OPENED",
"transactionStatusSummary": "COMPLETED"
},
"notes": "",
"id": "e34dff21-1226-4af2-a42b-ca15275bd9fa"
},
{
"taxExempted": false,
"valid": true,
"accepted": false,
"createdAt": "2022-04-14T21:10:57Z",
"updatedAt": "2022-04-14T21:11:29Z",
"context": {
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"transactionInstruction": "NONE",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"customer": {
"createdAt": "2022-04-14T21:10:55Z",
"updatedAt": "2022-04-14T21:10:55Z",
"id": 369473069,
"emails": {},
"phones": {},
"firstName": "",
"lastName": ""
},
"items": [
{
"taxExempted": false,
"createdAt": "2022-04-14T21:10:57Z",
"updatedAt": "2022-04-14T21:10:57Z",
"quantity": 1,
"id": 543,
"unitPrice": 5500,
"discount": 0,
"fee": 0,
"tax": 0,
"status": "ORDERED",
"name": "itemA",
"unitOfMeasure": "EACH"
}
],
"transactions": [
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-14T21:10:55Z",
"updatedAt": "2022-04-14T21:11:29Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-14T21:10:54Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2023,
"id": 369457464,
"numberFirst6": "405413",
"numberLast4": "6357",
"numberMasked": "4054136357",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/",
"serviceCode": "201",
"cardId": "be92c1e4-2fdd-4b28-a2ab-57addb085115"
},
"emvData": {
"emvTags": {
"0x1F8231": "18",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F37": "8CF4ACD8",
"0x5F24": "231231",
"0x9F02": "000000008000",
"0x9F66": "82804000",
"0x5F20": "202F"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "96d6ec95-aae0-45a9-bf29-2735f460ee59",
"rel": "CAPTURE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "ca62c3bb-90aa-4563-bd69-f95d91287627",
"type": "POYNT_ORDER"
}
],
"customerUserId": 369473069,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"approvedAmount": 8000,
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "29ea2e4d-0180-1000-d4b4-c6fb481f142d",
"approvalCode": "302550",
"batchId": "1",
"retrievalRefNum": "29ea2e4d-0180-1000-d4b4-c6fb481f142d"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 8000,
"orderAmount": 5500,
"tipAmount": 2500,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "29ea2e4d-0180-1000-d4b4-c6fb481f142d"
},
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": true,
"reversalVoid": false,
"createdAt": "2022-04-14T21:11:29Z",
"updatedAt": "2022-05-11T23:47:31Z",
"context": {
"businessType": "TEST_MERCHANT",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2023,
"id": 369457464,
"numberFirst6": "405413",
"numberLast4": "6357",
"numberMasked": "4054136357",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/",
"serviceCode": "201",
"cardId": "be92c1e4-2fdd-4b28-a2ab-57addb085115"
},
"emvData": {
"emvTags": {
"0x1F8231": "18",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F37": "8CF4ACD8",
"0x5F24": "231231",
"0x9F02": "000000008000",
"0x9F66": "82804000",
"0x5F20": "202F"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "29ea2e4d-0180-1000-d4b4-c6fb481f142d",
"rel": "AUTHORIZE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "ca62c3bb-90aa-4563-bd69-f95d91287627",
"type": "POYNT_ORDER"
}
],
"customerUserId": 369473069,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "96d6ec95-aae0-45a9-bf29-2735f460ee59",
"approvalCode": "657116",
"batchId": "1"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "SETTLED",
"action": "CAPTURE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 8000,
"orderAmount": 5500,
"tipAmount": 2500,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "96d6ec95-aae0-45a9-bf29-2735f460ee59",
"parentId": "29ea2e4d-0180-1000-d4b4-c6fb481f142d"
}
],
"customerUserId": 369473069,
"amounts": {
"subTotal": 5500,
"discountTotal": 0,
"feeTotal": 0,
"taxTotal": 0,
"netTotal": 5500,
"currency": "USD",
"capturedTotals": {
"transactionFees": [],
"transactionAmount": 8000,
"orderAmount": 5500,
"tipAmount": 2500,
"cashbackAmount": 0,
"currency": "USD"
}
},
"statuses": {
"fulfillmentStatus": "NONE",
"status": "OPENED",
"transactionStatusSummary": "COMPLETED"
},
"notes": "",
"id": "ca62c3bb-90aa-4563-bd69-f95d91287627"
},
{
"taxExempted": false,
"valid": true,
"accepted": false,
"createdAt": "2022-04-14T21:12:31Z",
"updatedAt": "2022-04-14T21:12:31Z",
"context": {
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"transactionInstruction": "NONE",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"customer": {
"createdAt": "2022-04-14T21:12:29Z",
"updatedAt": "2022-04-14T21:12:29Z",
"id": 369473629,
"emails": {},
"phones": {},
"firstName": "",
"lastName": ""
},
"items": [
{
"taxExempted": false,
"createdAt": "2022-04-14T21:12:31Z",
"updatedAt": "2022-04-14T21:12:31Z",
"quantity": 1,
"id": 543,
"unitPrice": 6000,
"discount": 0,
"fee": 0,
"tax": 0,
"status": "ORDERED",
"name": "itemA",
"unitOfMeasure": "EACH"
}
],
"transactions": [
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-14T21:12:29Z",
"updatedAt": "2022-04-14T21:12:29Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-14T21:12:28Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2023,
"id": 369458037,
"numberFirst6": "405413",
"numberLast4": "6357",
"numberMasked": "4054136357",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/",
"serviceCode": "201",
"cardId": "831f298b-0622-4e2a-9ad5-3a7cfabbb565"
},
"emvData": {
"emvTags": {
"0x1F8231": "18",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F37": "060F6C27",
"0x5F24": "231231",
"0x9F02": "000000009000",
"0x9F66": "82804000",
"0x5F20": "202F"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "4de10d8c-94b0-40c1-a4c6-d1420a543963",
"type": "POYNT_ORDER"
}
],
"customerUserId": 369473629,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"approvedAmount": 9000,
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "29eb9e2e-0180-1000-d4b4-c6fb481f142d",
"approvalCode": "528437",
"batchId": "1",
"retrievalRefNum": "29eb9e2e-0180-1000-d4b4-c6fb481f142d"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 9000,
"orderAmount": 6000,
"tipAmount": 3000,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "AUTHORIZED",
"id": "29eb9e2e-0180-1000-d4b4-c6fb481f142d"
}
],
"customerUserId": 369473629,
"amounts": {
"subTotal": 6000,
"discountTotal": 0,
"feeTotal": 0,
"taxTotal": 0,
"netTotal": 6000,
"currency": "USD",
"authorizedTotals": {
"transactionFees": [],
"transactionAmount": 9000,
"orderAmount": 6000,
"tipAmount": 3000,
"cashbackAmount": 0,
"currency": "USD"
}
},
"statuses": {
"fulfillmentStatus": "NONE",
"status": "OPENED",
"transactionStatusSummary": "PENDING"
},
"notes": "",
"id": "4de10d8c-94b0-40c1-a4c6-d1420a543963"
},
{
"taxExempted": false,
"valid": true,
"accepted": false,
"createdAt": "2022-04-14T21:13:47Z",
"updatedAt": "2022-04-22T18:03:53Z",
"context": {
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"transactionInstruction": "NONE",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"customer": {
"createdAt": "2022-04-14T21:13:45Z",
"updatedAt": "2022-04-14T21:13:45Z",
"id": 369474054,
"emails": {},
"phones": {},
"firstName": "",
"lastName": ""
},
"items": [
{
"taxExempted": false,
"createdAt": "2022-04-14T21:13:47Z",
"updatedAt": "2022-04-14T21:13:47Z",
"quantity": 1,
"id": 543,
"unitPrice": 2500,
"discount": 0,
"fee": 0,
"tax": 0,
"status": "ORDERED",
"name": "itemA",
"unitOfMeasure": "EACH"
}
],
"transactions": [
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-14T21:13:45Z",
"updatedAt": "2022-04-22T18:03:53Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-14T21:13:44Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2023,
"id": 369458474,
"numberFirst6": "405413",
"numberLast4": "6357",
"numberMasked": "4054136357",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/",
"serviceCode": "201",
"cardId": "e39a4f40-73f3-40b7-896e-4c4263d2ebb0"
},
"emvData": {
"emvTags": {
"0x1F8231": "18",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F37": "49249314",
"0x5F24": "231231",
"0x9F02": "000000006500",
"0x9F66": "82804000",
"0x5F20": "202F"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "89f9d20a-9b27-41f2-aea7-0c96939caaae",
"rel": "CAPTURE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "1800d850-34ea-4213-81d4-793dc640fe55",
"type": "POYNT_ORDER"
}
],
"customerUserId": 369474054,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"approvedAmount": 6500,
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "29ecc5cf-0180-1000-d4b4-c6fb481f142d",
"approvalCode": "687942",
"batchId": "1",
"retrievalRefNum": "29ecc5cf-0180-1000-d4b4-c6fb481f142d"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 6500,
"orderAmount": 2500,
"tipAmount": 4000,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "REFUNDED",
"id": "29ecc5cf-0180-1000-d4b4-c6fb481f142d"
},
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": true,
"reversalVoid": false,
"createdAt": "2022-04-14T21:13:57Z",
"updatedAt": "2022-05-11T23:47:31Z",
"context": {
"businessType": "TEST_MERCHANT",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2023,
"id": 369458474,
"numberFirst6": "405413",
"numberLast4": "6357",
"numberMasked": "4054136357",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/",
"serviceCode": "201",
"cardId": "e39a4f40-73f3-40b7-896e-4c4263d2ebb0"
},
"emvData": {
"emvTags": {
"0x1F8231": "18",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F37": "49249314",
"0x5F24": "231231",
"0x9F02": "000000006500",
"0x9F66": "82804000",
"0x5F20": "202F"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "5271d314-0180-1000-c2ea-a8a64c38d673",
"rel": "REFUND",
"method": "GET"
},
{
"href": "29ecc5cf-0180-1000-d4b4-c6fb481f142d",
"rel": "AUTHORIZE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "1800d850-34ea-4213-81d4-793dc640fe55",
"type": "POYNT_ORDER"
}
],
"customerUserId": 369474054,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "89f9d20a-9b27-41f2-aea7-0c96939caaae",
"approvalCode": "233168",
"batchId": "1"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "SETTLED",
"action": "CAPTURE",
"amounts": {
"customerOptedNoTip": false,
"transactionFees": [],
"transactionAmount": 6500,
"orderAmount": 2500,
"tipAmount": 4000,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "REFUNDED",
"id": "89f9d20a-9b27-41f2-aea7-0c96939caaae",
"parentId": "29ecc5cf-0180-1000-d4b4-c6fb481f142d"
},
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": false,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": true,
"reversalVoid": false,
"createdAt": "2022-04-22T18:03:53Z",
"updatedAt": "2022-05-11T23:47:31Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-22T18:03:52Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.services",
"mcc": "5812",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2023,
"id": 369458474,
"numberFirst6": "405413",
"numberLast4": "6357",
"numberMasked": "4054136357",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/",
"serviceCode": "201",
"cardId": "e39a4f40-73f3-40b7-896e-4c4263d2ebb0"
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "89f9d20a-9b27-41f2-aea7-0c96939caaae",
"rel": "CAPTURE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "1800d850-34ea-4213-81d4-793dc640fe55",
"type": "POYNT_ORDER"
}
],
"customerUserId": 369474054,
"processorOptions": {},
"processorResponse": {
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "5271d314-0180-1000-c2ea-a8a64c38d673",
"approvalCode": "813158",
"batchId": "1"
},
"customerLanguage": "en",
"settlementStatus": "SETTLED",
"action": "REFUND",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 6500,
"orderAmount": 2500,
"tipAmount": 4000,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "REFUNDED",
"id": "5271d314-0180-1000-c2ea-a8a64c38d673",
"parentId": "89f9d20a-9b27-41f2-aea7-0c96939caaae"
}
],
"customerUserId": 369474054,
"amounts": {
"subTotal": 2500,
"discountTotal": 0,
"feeTotal": 0,
"taxTotal": 0,
"netTotal": 2500,
"currency": "USD",
"refundedTotals": {
"transactionFees": [],
"transactionAmount": 6500,
"orderAmount": 2500,
"tipAmount": 4000,
"cashbackAmount": 0,
"currency": "USD"
}
},
"statuses": {
"fulfillmentStatus": "NONE",
"status": "OPENED",
"transactionStatusSummary": "REFUNDED"
},
"notes": "",
"id": "1800d850-34ea-4213-81d4-793dc640fe55"
},
{
"taxExempted": false,
"valid": true,
"accepted": false,
"createdAt": "2022-04-27T18:03:13Z",
"updatedAt": "2022-04-27T18:03:14Z",
"context": {
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"transactionInstruction": "NONE",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"customer": {
"createdAt": "2022-04-27T18:03:03Z",
"updatedAt": "2022-04-27T18:03:03Z",
"id": 372348487,
"emails": {},
"phones": {},
"firstName": "KASTUREEMA",
"lastName": "SARMA"
},
"items": [
{
"taxExempted": false,
"createdAt": "2022-04-27T18:03:13Z",
"updatedAt": "2022-04-27T18:03:13Z",
"quantity": 1,
"id": 12,
"unitPrice": 2500,
"discount": 0,
"fee": 0,
"tax": 0,
"status": "ORDERED",
"name": "item1",
"unitOfMeasure": "EACH"
}
],
"transactions": [
{
"signatureRequired": true,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": false,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-27T18:03:03Z",
"updatedAt": "2022-04-27T18:03:14Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-27T18:03:02Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 7,
"expirationYear": 2020,
"id": 372381668,
"numberFirst6": "473702",
"numberLast4": "2897",
"numberMasked": "4737022897",
"cardHolderFirstName": "KASTUREEMA",
"cardHolderLastName": "SARMA",
"cardHolderFullName": "SARMA/ KASTUREEMA",
"serviceCode": "201",
"cardId": "dde252ec-02f1-462d-9ea4-b61568006cea"
},
"emvData": {
"emvTags": {
"0x5F34": "00",
"0x9F36": "01BF",
"0x9F37": "384802E3",
"0x9F34": "5E0000",
"0x9F35": "21",
"0x9F10": "06010A03600000",
"0x9F33": "E0F0C8",
"0x84": "A0000000031010",
"0x5F2A": "0840",
"0x9F0E": "0000000000",
"0x82": "1800",
"0x9F0F": "FC70BC9800",
"0x9F0D": "FC50AC8800",
"0x9F09": "008C",
"0x9F07": "FF80",
"0x9F08": "0096",
"0x1F8231": "87",
"0x5F28": "0840",
"0x9F27": "40",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9B": "6800",
"0x9F03": "000000000000",
"0x9A": "220427",
"0x9F26": "0CD6F292E998049C",
"0x5F24": "200731",
"0x9F02": "000000002500",
"0x5F20": "5341524D412F204B415354555245454D41202020202020202020",
"0x95": "80C0008000",
"0x50": "56495341204445424954",
"0x9F1A": "0840"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "INTEGRATED_CIRCUIT_CARD"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "ecb82bdc-f355-4f9d-8656-929d238c424f",
"rel": "CAPTURE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "112ea7d1-b821-4579-8afe-3112778596c0",
"type": "POYNT_ORDER"
}
],
"customerUserId": 372348487,
"processorOptions": {},
"processorResponse": {
"approvedAmount": 2500,
"emvTags": {
"0x8A": "3030",
"0x89": "454139324645"
},
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "6c30db9b-0180-1000-7603-9ebe5180647c",
"approvalCode": "870321",
"batchId": "1",
"retrievalRefNum": "6c30db9b-0180-1000-7603-9ebe5180647c"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 2500,
"orderAmount": 2500,
"tipAmount": 0,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "6c30db9b-0180-1000-7603-9ebe5180647c"
},
{
"signatureRequired": true,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": false,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": true,
"reversalVoid": false,
"createdAt": "2022-04-27T18:03:14Z",
"updatedAt": "2022-05-11T23:47:31Z",
"context": {
"businessType": "TEST_MERCHANT",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 7,
"expirationYear": 2020,
"id": 372381668,
"numberFirst6": "473702",
"numberLast4": "2897",
"numberMasked": "4737022897",
"cardHolderFirstName": "KASTUREEMA",
"cardHolderLastName": "SARMA",
"cardHolderFullName": "SARMA/ KASTUREEMA",
"serviceCode": "201",
"cardId": "dde252ec-02f1-462d-9ea4-b61568006cea"
},
"emvData": {
"emvTags": {
"0x5F34": "00",
"0x9F36": "01BF",
"0x9F37": "384802E3",
"0x9F34": "5E0000",
"0x9F35": "21",
"0x9F10": "06010A03A00000",
"0x9F33": "E0F0C8",
"0x84": "A0000000031010",
"0x5F2A": "0840",
"0x9F0E": "0000000000",
"0x82": "1800",
"0x9F0F": "FC70BC9800",
"0x9F0D": "FC50AC8800",
"0x9F09": "008C",
"0x9F07": "FF80",
"0x9F08": "0096",
"0x1F8231": "87",
"0x5F28": "0840",
"0x9F27": "80",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9B": "6800",
"0x9F03": "000000000000",
"0x9A": "220427",
"0x9F26": "3D95355535AEF88E",
"0x5F24": "200731",
"0x9F02": "000000002500",
"0x5F20": "5341524D412F204B415354555245454D41202020202020202020",
"0x95": "80C0008000",
"0x50": "56495341204445424954",
"0x9F1A": "0840"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "INTEGRATED_CIRCUIT_CARD"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "6c30db9b-0180-1000-7603-9ebe5180647c",
"rel": "AUTHORIZE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "112ea7d1-b821-4579-8afe-3112778596c0",
"type": "POYNT_ORDER"
}
],
"customerUserId": 372348487,
"processorOptions": {},
"processorResponse": {
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "ecb82bdc-f355-4f9d-8656-929d238c424f",
"approvalCode": "133128",
"batchId": "1"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "SETTLED",
"action": "CAPTURE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 2500,
"orderAmount": 2500,
"tipAmount": 0,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "ecb82bdc-f355-4f9d-8656-929d238c424f",
"parentId": "6c30db9b-0180-1000-7603-9ebe5180647c"
}
],
"customerUserId": 372348487,
"amounts": {
"subTotal": 2500,
"discountTotal": 0,
"feeTotal": 0,
"taxTotal": 0,
"netTotal": 2500,
"currency": "USD",
"capturedTotals": {
"transactionFees": [],
"transactionAmount": 2500,
"orderAmount": 2500,
"tipAmount": 0,
"cashbackAmount": 0,
"currency": "USD"
}
},
"statuses": {
"fulfillmentStatus": "NONE",
"status": "OPENED",
"transactionStatusSummary": "COMPLETED"
},
"notes": "order notes",
"id": "112ea7d1-b821-4579-8afe-3112778596c0"
},
{
"taxExempted": false,
"valid": true,
"accepted": false,
"createdAt": "2022-04-27T18:03:44Z",
"updatedAt": "2022-04-27T18:03:50Z",
"context": {
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"transactionInstruction": "NONE",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"customer": {
"createdAt": "2022-04-27T18:03:38Z",
"updatedAt": "2022-04-27T18:03:38Z",
"id": 372348695,
"emails": {},
"phones": {},
"firstName": "KASTUREEMA",
"lastName": "SARMA"
},
"items": [
{
"taxExempted": false,
"createdAt": "2022-04-27T18:03:44Z",
"updatedAt": "2022-04-27T18:03:44Z",
"quantity": 1,
"id": 543,
"unitPrice": 4300,
"discount": 0,
"fee": 0,
"tax": 0,
"status": "ORDERED",
"name": "itemA",
"unitOfMeasure": "EACH"
}
],
"transactions": [
{
"signatureRequired": true,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-27T18:03:38Z",
"updatedAt": "2022-04-27T18:03:50Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-27T18:03:38Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 7,
"expirationYear": 2020,
"id": 372381880,
"numberFirst6": "473702",
"numberLast4": "2897",
"numberMasked": "4737022897",
"cardHolderFirstName": "KASTUREEMA",
"cardHolderLastName": "SARMA",
"cardHolderFullName": "SARMA/ KASTUREEMA",
"serviceCode": "201",
"cardId": "b19e3be7-138a-4dc5-a468-8b43b5ef8a49"
},
"emvData": {
"emvTags": {
"0x5F34": "00",
"0x9F36": "01C0",
"0x9F37": "19886D6B",
"0x9F34": "5E0000",
"0x9F35": "21",
"0x9F10": "06010A03600000",
"0x9F33": "E0F0C8",
"0x84": "A0000000031010",
"0x5F2A": "0840",
"0x9F0E": "0000000000",
"0x82": "1800",
"0x9F0F": "FC70BC9800",
"0x9F0D": "FC50AC8800",
"0x9F09": "008C",
"0x9F07": "FF80",
"0x9F08": "0096",
"0x1F8231": "87",
"0x5F28": "0840",
"0x9F27": "40",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9B": "6800",
"0x9F03": "000000000000",
"0x9A": "220427",
"0x9F26": "E6822924CDDAB441",
"0x5F24": "200731",
"0x9F02": "000000004300",
"0x5F20": "5341524D412F204B415354555245454D41202020202020202020",
"0x95": "80C0008000",
"0x50": "56495341204445424954",
"0x9F1A": "0840"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "INTEGRATED_CIRCUIT_CARD"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "7f1e5cc7-1dfa-4248-b7a2-3f9317b38a24",
"rel": "CAPTURE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "29198bfb-620b-41ca-940d-cae9fb415bcf",
"type": "POYNT_ORDER"
}
],
"customerUserId": 372348695,
"processorOptions": {},
"processorResponse": {
"approvedAmount": 4300,
"emvTags": {
"0x8A": "3030",
"0x89": "454139324645"
},
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "6c3166d4-0180-1000-7603-9ebe5180647c",
"approvalCode": "166143",
"batchId": "1",
"retrievalRefNum": "6c3166d4-0180-1000-7603-9ebe5180647c"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 4300,
"orderAmount": 4300,
"tipAmount": 0,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "6c3166d4-0180-1000-7603-9ebe5180647c"
},
{
"signatureRequired": true,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": true,
"reversalVoid": false,
"createdAt": "2022-04-27T18:03:50Z",
"updatedAt": "2022-05-11T23:47:31Z",
"context": {
"businessType": "TEST_MERCHANT",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 7,
"expirationYear": 2020,
"id": 372381880,
"numberFirst6": "473702",
"numberLast4": "2897",
"numberMasked": "4737022897",
"cardHolderFirstName": "KASTUREEMA",
"cardHolderLastName": "SARMA",
"cardHolderFullName": "SARMA/ KASTUREEMA",
"serviceCode": "201",
"cardId": "b19e3be7-138a-4dc5-a468-8b43b5ef8a49"
},
"emvData": {
"emvTags": {
"0x5F34": "00",
"0x9F36": "01C0",
"0x9F37": "19886D6B",
"0x9F34": "5E0000",
"0x9F35": "21",
"0x9F10": "06010A03A00000",
"0x9F33": "E0F0C8",
"0x84": "A0000000031010",
"0x5F2A": "0840",
"0x9F0E": "0000000000",
"0x82": "1800",
"0x9F0F": "FC70BC9800",
"0x9F0D": "FC50AC8800",
"0x9F09": "008C",
"0x9F07": "FF80",
"0x9F08": "0096",
"0x1F8231": "87",
"0x5F28": "0840",
"0x9F27": "80",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9B": "6800",
"0x9F03": "000000000000",
"0x9A": "220427",
"0x9F26": "124B9DAA8C1ED36F",
"0x5F24": "200731",
"0x9F02": "000000004300",
"0x5F20": "5341524D412F204B415354555245454D41202020202020202020",
"0x95": "80C0008000",
"0x50": "56495341204445424954",
"0x9F1A": "0840"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "INTEGRATED_CIRCUIT_CARD"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "6c3166d4-0180-1000-7603-9ebe5180647c",
"rel": "AUTHORIZE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "29198bfb-620b-41ca-940d-cae9fb415bcf",
"type": "POYNT_ORDER"
}
],
"customerUserId": 372348695,
"processorOptions": {},
"processorResponse": {
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "7f1e5cc7-1dfa-4248-b7a2-3f9317b38a24",
"approvalCode": "600024",
"batchId": "1"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "SETTLED",
"action": "CAPTURE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 4300,
"orderAmount": 4300,
"tipAmount": 0,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "7f1e5cc7-1dfa-4248-b7a2-3f9317b38a24",
"parentId": "6c3166d4-0180-1000-7603-9ebe5180647c"
}
],
"customerUserId": 372348695,
"amounts": {
"subTotal": 4300,
"discountTotal": 0,
"feeTotal": 0,
"taxTotal": 0,
"netTotal": 4300,
"currency": "USD",
"capturedTotals": {
"transactionFees": [],
"transactionAmount": 4300,
"orderAmount": 4300,
"tipAmount": 0,
"cashbackAmount": 0,
"currency": "USD"
}
},
"statuses": {
"fulfillmentStatus": "NONE",
"status": "OPENED",
"transactionStatusSummary": "COMPLETED"
},
"notes": "",
"id": "29198bfb-620b-41ca-940d-cae9fb415bcf"
},
{
"taxExempted": false,
"valid": true,
"accepted": false,
"createdAt": "2022-04-27T18:04:14Z",
"updatedAt": "2022-04-27T18:04:31Z",
"context": {
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"transactionInstruction": "NONE",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"customer": {
"createdAt": "2022-04-27T18:04:08Z",
"updatedAt": "2022-04-27T18:04:08Z",
"id": 372348900,
"emails": {},
"phones": {},
"firstName": "KASTUREEMA",
"lastName": "SARMA"
},
"items": [
{
"taxExempted": false,
"createdAt": "2022-04-27T18:04:14Z",
"updatedAt": "2022-04-27T18:04:14Z",
"quantity": 1,
"id": 543,
"unitPrice": 4500,
"discount": 0,
"fee": 0,
"tax": 0,
"status": "ORDERED",
"name": "itemA",
"unitOfMeasure": "EACH"
}
],
"transactions": [
{
"signatureRequired": true,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": true,
"amountsAdjusted": true,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-27T18:04:08Z",
"updatedAt": "2022-04-27T18:04:31Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-27T18:04:08Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 7,
"expirationYear": 2020,
"id": 372382095,
"numberFirst6": "473702",
"numberLast4": "2897",
"numberMasked": "4737022897",
"cardHolderFirstName": "KASTUREEMA",
"cardHolderLastName": "SARMA",
"cardHolderFullName": "SARMA/ KASTUREEMA",
"serviceCode": "201",
"cardId": "ef0b39f1-116c-4295-9761-a2951314b156"
},
"emvData": {
"emvTags": {
"0x5F34": "00",
"0x9F36": "01C1",
"0x9F37": "0EC12581",
"0x9F34": "5E0000",
"0x9F35": "21",
"0x9F10": "06010A03600000",
"0x9F33": "E0F0C8",
"0x84": "A0000000031010",
"0x5F2A": "0840",
"0x9F0E": "0000000000",
"0x82": "1800",
"0x9F0F": "FC70BC9800",
"0x9F0D": "FC50AC8800",
"0x9F09": "008C",
"0x9F07": "FF80",
"0x9F08": "0096",
"0x1F8231": "87",
"0x5F28": "0840",
"0x9F27": "40",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9B": "6800",
"0x9F03": "000000000000",
"0x9A": "220427",
"0x9F26": "870DF41226E24EAC",
"0x5F24": "200731",
"0x9F02": "000000004500",
"0x5F20": "5341524D412F204B415354555245454D41202020202020202020",
"0x95": "80C0008000",
"0x50": "56495341204445424954",
"0x9F1A": "0840"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "INTEGRATED_CIRCUIT_CARD"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "6b0d6b3d-e9da-419a-937e-f18652a6cac1",
"rel": "CAPTURE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "8471f591-252b-4112-bae1-02288161911a",
"type": "POYNT_ORDER"
}
],
"customerUserId": 372348900,
"processorOptions": {},
"processorResponse": {
"approvedAmount": 4500,
"emvTags": {
"0x8A": "3030",
"0x89": "454139324645"
},
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "6c31dbef-0180-1000-7603-9ebe5180647c",
"approvalCode": "831188",
"batchId": "1",
"retrievalRefNum": "6c31dbef-0180-1000-7603-9ebe5180647c"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 5000,
"orderAmount": 4500,
"tipAmount": 500,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "6c31dbef-0180-1000-7603-9ebe5180647c"
},
{
"signatureRequired": true,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": true,
"reversalVoid": false,
"createdAt": "2022-04-27T18:04:31Z",
"updatedAt": "2022-05-11T23:47:31Z",
"context": {
"businessType": "TEST_MERCHANT",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 7,
"expirationYear": 2020,
"id": 372382095,
"numberFirst6": "473702",
"numberLast4": "2897",
"numberMasked": "4737022897",
"cardHolderFirstName": "KASTUREEMA",
"cardHolderLastName": "SARMA",
"cardHolderFullName": "SARMA/ KASTUREEMA",
"serviceCode": "201",
"cardId": "ef0b39f1-116c-4295-9761-a2951314b156"
},
"emvData": {
"emvTags": {
"0x5F34": "00",
"0x9F36": "01C1",
"0x9F37": "0EC12581",
"0x9F34": "5E0000",
"0x9F35": "21",
"0x9F10": "06010A03A00000",
"0x9F33": "E0F0C8",
"0x84": "A0000000031010",
"0x5F2A": "0840",
"0x9F0E": "0000000000",
"0x82": "1800",
"0x9F0F": "FC70BC9800",
"0x9F0D": "FC50AC8800",
"0x9F09": "008C",
"0x9F07": "FF80",
"0x9F08": "0096",
"0x1F8231": "87",
"0x5F28": "0840",
"0x9F27": "80",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9B": "6800",
"0x9F03": "000000000000",
"0x9A": "220427",
"0x9F26": "0A384683B5A8DE88",
"0x5F24": "200731",
"0x9F02": "000000004500",
"0x5F20": "5341524D412F204B415354555245454D41202020202020202020",
"0x95": "80C0008000",
"0x50": "56495341204445424954",
"0x9F1A": "0840"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "INTEGRATED_CIRCUIT_CARD"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "6c31dbef-0180-1000-7603-9ebe5180647c",
"rel": "AUTHORIZE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "8471f591-252b-4112-bae1-02288161911a",
"type": "POYNT_ORDER"
}
],
"customerUserId": 372348900,
"processorOptions": {},
"processorResponse": {
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "6b0d6b3d-e9da-419a-937e-f18652a6cac1",
"approvalCode": "110271",
"batchId": "1"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "SETTLED",
"action": "CAPTURE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 5000,
"orderAmount": 4500,
"tipAmount": 500,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "6b0d6b3d-e9da-419a-937e-f18652a6cac1",
"parentId": "6c31dbef-0180-1000-7603-9ebe5180647c"
}
],
"customerUserId": 372348900,
"amounts": {
"subTotal": 4500,
"discountTotal": 0,
"feeTotal": 0,
"taxTotal": 0,
"netTotal": 4500,
"currency": "USD",
"capturedTotals": {
"transactionFees": [],
"transactionAmount": 5000,
"orderAmount": 4500,
"tipAmount": 500,
"cashbackAmount": 0,
"currency": "USD"
}
},
"statuses": {
"fulfillmentStatus": "NONE",
"status": "OPENED",
"transactionStatusSummary": "COMPLETED"
},
"notes": "",
"id": "8471f591-252b-4112-bae1-02288161911a"
},
{
"taxExempted": false,
"valid": true,
"accepted": false,
"createdAt": "2022-04-27T18:04:57Z",
"updatedAt": "2022-04-27T18:05:04Z",
"context": {
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"transactionInstruction": "NONE",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"customer": {
"createdAt": "2022-04-27T18:04:52Z",
"updatedAt": "2022-04-27T18:04:52Z",
"id": 372349189,
"emails": {},
"phones": {},
"firstName": "KASTUREEMA",
"lastName": "SARMA"
},
"items": [
{
"taxExempted": false,
"createdAt": "2022-04-27T18:04:57Z",
"updatedAt": "2022-04-27T18:04:57Z",
"quantity": 1,
"id": 543,
"unitPrice": 5500,
"discount": 0,
"fee": 0,
"tax": 0,
"status": "ORDERED",
"name": "itemA",
"unitOfMeasure": "EACH"
}
],
"transactions": [
{
"signatureRequired": true,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": true,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-27T18:04:52Z",
"updatedAt": "2022-04-27T18:05:04Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-27T18:04:52Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 7,
"expirationYear": 2020,
"id": 372382389,
"numberFirst6": "473702",
"numberLast4": "2897",
"numberMasked": "4737022897",
"cardHolderFirstName": "KASTUREEMA",
"cardHolderLastName": "SARMA",
"cardHolderFullName": "SARMA/ KASTUREEMA",
"serviceCode": "201",
"cardId": "d5d8f42c-84e5-49ef-8bb2-112604fb0cf1"
},
"emvData": {
"emvTags": {
"0x5F34": "00",
"0x9F36": "01C2",
"0x9F37": "65C9902E",
"0x9F34": "5E0000",
"0x9F35": "21",
"0x9F10": "06010A03600000",
"0x9F33": "E0F0C8",
"0x84": "A0000000031010",
"0x5F2A": "0840",
"0x9F0E": "0000000000",
"0x82": "1800",
"0x9F0F": "FC70BC9800",
"0x9F0D": "FC50AC8800",
"0x9F09": "008C",
"0x9F07": "FF80",
"0x9F08": "0096",
"0x1F8231": "87",
"0x5F28": "0840",
"0x9F27": "40",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9B": "6800",
"0x9F03": "000000000000",
"0x9A": "220427",
"0x9F26": "FC14EB2A11C5B560",
"0x5F24": "200731",
"0x9F02": "000000006000",
"0x5F20": "5341524D412F204B415354555245454D41202020202020202020",
"0x95": "80C0008000",
"0x50": "56495341204445424954",
"0x9F1A": "0840"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "INTEGRATED_CIRCUIT_CARD"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "ba5953f1-f637-4b2d-8639-4e2887d80d4c",
"rel": "REFUND",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "afc4a299-7f78-4c4c-964e-c481af333ee5",
"type": "POYNT_ORDER"
}
],
"customerUserId": 372349189,
"processorOptions": {},
"processorResponse": {
"approvedAmount": 6000,
"emvTags": {
"0x8A": "3030",
"0x89": "454139324645"
},
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "6c3287cb-0180-1000-7603-9ebe5180647c",
"approvalCode": "373470",
"batchId": "1",
"retrievalRefNum": "6c3287cb-0180-1000-7603-9ebe5180647c"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 6000,
"orderAmount": 5500,
"tipAmount": 500,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "VOIDED",
"id": "6c3287cb-0180-1000-7603-9ebe5180647c"
},
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": false,
"partiallyApproved": false,
"actionVoid": true,
"voided": true,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-27T18:05:04Z",
"updatedAt": "2022-04-27T18:05:04Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-27T18:05:03Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"mcc": "5812",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 7,
"expirationYear": 2020,
"id": 372382389,
"numberFirst6": "473702",
"numberLast4": "2897",
"numberMasked": "473702******2897",
"cardHolderFirstName": "KASTUREEMA",
"cardHolderLastName": "SARMA",
"cardHolderFullName": "SARMA/ KASTUREEMA",
"serviceCode": "201",
"cardId": "d5d8f42c-84e5-49ef-8bb2-112604fb0cf1"
},
"emvData": {
"emvTags": {
"0x5F34": "00",
"0x9F36": "01C2",
"0x9F37": "65C9902E",
"0x9F34": "5E0000",
"0x9F35": "21",
"0x9F10": "06010A03600000",
"0x9F33": "E0F0C8",
"0x84": "A0000000031010",
"0x5F2A": "0840",
"0x9F0E": "0000000000",
"0x82": "1800",
"0x9F0F": "FC70BC9800",
"0x9F0D": "FC50AC8800",
"0x9F09": "008C",
"0x9F07": "FF80",
"0x9F08": "0096",
"0x1F8231": "87",
"0x5F28": "0840",
"0x9F27": "40",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9B": "6800",
"0x9F03": "000000000000",
"0x9A": "220427",
"0x9F26": "FC14EB2A11C5B560",
"0x5F24": "200731",
"0x9F02": "000000006000",
"0x5F20": "5341524D412F204B415354555245454D41202020202020202020",
"0x95": "80C0008000",
"0x50": "56495341204445424954",
"0x9F1A": "0840"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "INTEGRATED_CIRCUIT_CARD"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "6c3287cb-0180-1000-7603-9ebe5180647c",
"rel": "AUTHORIZE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "afc4a299-7f78-4c4c-964e-c481af333ee5",
"type": "POYNT_ORDER"
}
],
"customerUserId": 372349189,
"processorResponse": {
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "ba5953f1-f637-4b2d-8639-4e2887d80d4c",
"approvalCode": "247998"
},
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "REFUND",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 6000,
"orderAmount": 5500,
"tipAmount": 500,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "VOIDED",
"id": "ba5953f1-f637-4b2d-8639-4e2887d80d4c",
"parentId": "6c3287cb-0180-1000-7603-9ebe5180647c"
}
],
"customerUserId": 372349189,
"amounts": {
"subTotal": 5500,
"discountTotal": 0,
"feeTotal": 0,
"taxTotal": 0,
"netTotal": 5500,
"currency": "USD",
"voidedTotals": {
"transactionFees": [],
"transactionAmount": 6000,
"orderAmount": 5500,
"tipAmount": 500,
"cashbackAmount": 0,
"currency": "USD"
}
},
"statuses": {
"fulfillmentStatus": "NONE",
"status": "OPENED",
"transactionStatusSummary": "CANCELED"
},
"notes": "",
"id": "afc4a299-7f78-4c4c-964e-c481af333ee5"
}
],
"count": 95
}
Definition
.exports.getOrders = function getOrders(options, next) { ... }
module/**
* Get all orders at a business.
* @param {String} options.businessId
* @param {String} options.startAt (optional) - the time from which to start fetching orders
* @param {Integer} options.startOffset (optional)
* @param {String} options.endAt (optional) - the time at which to stop fetching orders
* @param {Integer} options.limit (optional) - the number of orders to fetch
* @param {String} options.cardNumberFirst6 (optional) - limit results to orders with transactions done by cards starting with these 6 numbers
* @param {String} options.cardNumberLast4 (optional) - limit results to orders with transactions done by cards ending with these 4 numbers
* @param {Integer} options.cardExpirationMonth (optional) - limit results to orders with transactions done by cards expiring in this month
* @param {Integer} options.cardExpirationYear (optional) - limit results to orders with transactions done by cards expiring in this year
* @param {String} options.cardHolderFirstName (optional) - limit results to orders with transactions done by cards with this card holder first name
* @param {String} options.cardHolderLastName (optional) - limit results to orders with transactions done by cards with this card holder last name
* @param {String} options.storeId (optional) - only fetch orders for this store
* @param {String} options.includeStaysAll (optional)
* @return {OrderList} orders
*/
Sample Request
.getOrders({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
startOffset : 0,
limit : 1
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
})
Sample Response
{"count": 1,
"links": [
{"href": "/businesses/18f071cc-5ed4-4b33-80c1-305056d42bfb/orders?startAt=2017-02-03T02%3A34%3A14Z&endAt=2017-08-28T06%3A26%3A45Z&limit=1",
"method": "GET",
"rel": "next"
},
]"orders": [
{"amounts": {
"capturedTotals": {
"cashbackAmount": 0,
"currency": "USD",
"orderAmount": 625,
"tipAmount": 68,
"transactionAmount": 693
,
}"currency": "USD",
"discountTotal": 0,
"feeTotal": 0,
"netTotal": 625,
"subTotal": 625,
"taxTotal": 0
,
}"context": {
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"employeeUserId": 5247870,
"source": "INSTORE",
"storeDeviceId": "urn:tid:48c54303-6d51-39af-bdeb-4af53f621652",
"storeId": "c394627f-4f68-47fb-90a5-684ea801a352",
"transactionInstruction": "NONE"
,
}"createdAt": "2017-02-03T01:40:21Z",
"customerUserId": 5249839,
"id": "01a0c6f9-015a-1000-e3ce-bbbd758e32de",
"items": [
{"createdAt": "2017-02-03T01:40:21Z",
"discount": 0,
"fee": 0,
"id": 1,
"name": "water",
"productId": "f9faac3a-be56-4bff-b60b-84987cf021cb",
"quantity": 5,
"sku": "water",
"status": "FULFILLED",
"tax": 0,
"taxExempted": false,
"unitOfMeasure": "EACH",
"unitPrice": 125,
"updatedAt": "2017-02-03T01:40:21Z"
},
]"orderNumber": "4",
"statuses": {
"fulfillmentStatus": "FULFILLED",
"status": "OPENED",
"transactionStatusSummary": "COMPLETED"
,
}"taxExempted": false,
"transactions": [
{"action": "AUTHORIZE",
"actionVoid": false,
"adjusted": true,
"amounts": {
"cashbackAmount": 0,
"currency": "USD",
"customerOptedNoTip": false,
"orderAmount": 625,
"tipAmount": 68,
"transactionAmount": 693
,
}"amountsAdjusted": true,
"authOnly": false,
"context": {
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"businessType": "TEST_MERCHANT",
"employeeUserId": 5247870,
"mcc": "5812",
"source": "INSTORE",
"sourceApp": "co.poynt.services",
"storeDeviceId": "urn:tid:48c54303-6d51-39af-bdeb-4af53f621652",
"storeId": "c394627f-4f68-47fb-90a5-684ea801a352",
"transmissionAtLocal": "2017-02-03T01:40:08Z"
,
}"createdAt": "2017-02-03T01:40:09Z",
"customerUserId": 5249839,
"fundingSource": {
"card": {
"cardHolderFirstName": "LAWRENCE",
"cardHolderFullName": "LUK/LAWRENCE",
"cardHolderLastName": "LUK",
"expirationDate": 31,
"expirationMonth": 8,
"expirationYear": 2019,
"id": 244219,
"numberFirst6": "414720",
"numberLast4": "4308",
"status": "ACTIVE",
"type": "VISA"
,
}"debit": false,
"emvData": {
"emvTags": {
"0x50": "5649534120435245444954",
"0x5F20": "4C554B2F4C415752454E434520",
"0x5F24": "190831",
"0x5F2A": "0840",
"0x5F34": "02",
"0x82": "3C00",
"0x84": "A0000000031010",
"0x95": "0080008000",
"0x9A": "170202",
"0x9B": "E800",
"0x9C": "00",
"0x9F02": "000000000625",
"0x9F03": "000000000000",
"0x9F06": "A0000000031010",
"0x9F10": "06010A03A0A802",
"0x9F12": "43484153452056495341",
"0x9F1A": "0840",
"0x9F26": "376393F3F6B156AA",
"0x9F27": "80",
"0x9F33": "E0F0C8",
"0x9F34": "5E0000",
"0x9F35": "21",
"0x9F36": "0036",
"0x9F37": "94F3A4C5"
},
}"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "INTEGRATED_CIRCUIT_CARD"
,
}"type": "CREDIT_DEBIT"
,
}"id": "624fbc0f-794c-4866-a211-0f5be53b8345",
"links": [
{"href": "1b622bd1-c0db-4271-9fe1-4ed9fbf6610c",
"method": "GET",
"rel": "CAPTURE"
},
]"partiallyApproved": false,
"pinCaptured": false,
"processorResponse": {
"acquirer": "CHASE_PAYMENTECH",
"approvalCode": "534216",
"approvedAmount": 625,
"batchId": "1",
"emvTags": {
"0x89": "454139324645",
"0x8A": "3030"
,
}"processor": "MOCK",
"retrievalRefNum": "624fbc0f-794c-4866-a211-0f5be53b8345",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "624fbc0f-794c-4866-a211-0f5be53b8345"
,
}"references": [
{"id": "01a0c6f9-015a-1000-e3ce-bbbd758e32de",
"type": "POYNT_ORDER"
},
]"settled": false,
"signatureCaptured": true,
"signatureRequired": false,
"status": "CAPTURED",
"updatedAt": "2017-02-03T02:34:14Z",
"voided": false
,
}
{"action": "CAPTURE",
"actionVoid": false,
"adjusted": false,
"amounts": {
"cashbackAmount": 0,
"currency": "USD",
"customerOptedNoTip": false,
"orderAmount": 625,
"tipAmount": 68,
"transactionAmount": 693
,
}"amountsAdjusted": false,
"authOnly": false,
"context": {
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"businessType": "TEST_MERCHANT",
"employeeUserId": 5247870,
"mcc": "5812",
"source": "INSTORE",
"storeDeviceId": "urn:tid:48c54303-6d51-39af-bdeb-4af53f621652",
"storeId": "c394627f-4f68-47fb-90a5-684ea801a352"
,
}"createdAt": "2017-02-03T02:34:14Z",
"customerUserId": 5249839,
"fundingSource": {
"card": {
"cardHolderFirstName": "LAWRENCE",
"cardHolderFullName": "LUK/LAWRENCE",
"cardHolderLastName": "LUK",
"expirationDate": 31,
"expirationMonth": 8,
"expirationYear": 2019,
"id": 244219,
"numberFirst6": "414720",
"numberLast4": "4308",
"status": "ACTIVE",
"type": "VISA"
,
}"debit": false,
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "INTEGRATED_CIRCUIT_CARD"
,
}"type": "CREDIT_DEBIT"
,
}"id": "1b622bd1-c0db-4271-9fe1-4ed9fbf6610c",
"links": [
{"href": "624fbc0f-794c-4866-a211-0f5be53b8345",
"method": "GET",
"rel": "AUTHORIZE"
},
]"parentId": "624fbc0f-794c-4866-a211-0f5be53b8345",
"partiallyApproved": false,
"pinCaptured": false,
"processorResponse": {
"acquirer": "CHASE_PAYMENTECH",
"approvalCode": "283042",
"batchId": "1",
"processor": "MOCK",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "1b622bd1-c0db-4271-9fe1-4ed9fbf6610c"
,
}"references": [
{"id": "01a0c6f9-015a-1000-e3ce-bbbd758e32de",
"type": "POYNT_ORDER"
},
]"settled": false,
"signatureCaptured": true,
"signatureRequired": false,
"status": "CAPTURED",
"updatedAt": "2017-02-03T02:34:14Z",
"voided": false
},
]"updatedAt": "2017-02-03T01:40:21Z"
}
] }
Definition
@classmethod
def get_orders(cls, business_id, start_at=None, start_offset=None,
=None, limit=None, card_number_first_6=None,
end_at=None, card_expiration_month=None,
card_number_last_4=None, card_holder_first_name=None,
card_expiration_year=None, store_id=None):
card_holder_last_name"""
Get all orders at a business by various criteria.
Arguments:
business_id (str): the business ID
Keyword arguments:
start_at (int, optional): get orders created after this time in seconds
start_offset (int, optional): the numeric offset to start the list (for pagination)
end_at (int, optional): get orders created before this time in seconds
limit (int, optional): how many orders to return (for pagination)
card_number_first_6 (str, optional): return orders with card numbers starting with this
card_number_last_4 (str, optional): return orders with card numbers ending with this
card_expiration_month (str, optional): return orders with this card expiration month
card_expiration_year (str, optional): return orders with this card expiration year
card_holder_first_name (str, optional): return orders with first name matching this
card_holder_last_name (str, optional): return orders with last name matching this
store_id (str, optional): return orders from this store
"""
Sample Request
= poynt.Order.get_orders(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
=0,
start_offset=1
limit
)
if status_code < 300:
print(doc)
Sample Response
u'count': 20, u'orders': [{u'transactions': [{u'status': u'CAPTURED', u'adjusted': True, u'links': [{u'href': u'1b622bd1-c0db-4271-9fe1-4ed9fbf6610c', u'method': u'GET', u'rel': u'CAPTURE'}], u'voided': False, u'fundingSource': {u'entryDetails': {u'customerPresenceStatus': u'PRESENT', u'entryMode': u'INTEGRATED_CIRCUIT_CARD'}, u'emvData': {u'emvTags': {u'0x5F34': u'02', u'0x9C': u'00', u'0x9B': u'E800', u'0x9F27': u'80', u'0x9F26': u'376393F3F6B156AA', u'0x9F03': u'000000000000', u'0x9F02': u'000000000625', u'0x9F06': u'A0000000031010', u'0x9F37': u'94F3A4C5', u'0x9A': u'170202', u'0x9F33': u'E0F0C8', u'0x84': u'A0000000031010', u'0x5F2A': u'0840', u'0x82': u'3C00', u'0x9F1A': u'0840', u'0x5F24': u'190831', u'0x9F36': u'0036', u'0x5F20': u'4C554B2F4C415752454E434520', u'0x9F34': u'5E0000', u'0x9F35': u'21', u'0x9F10': u'06010A03A0A802', u'0x9F12': u'43484153452056495341', u'0x50': u'5649534120435245444954', u'0x95': u'0080008000'}}, u'type': u'CREDIT_DEBIT', u'card': {u'status': u'ACTIVE', u'expirationMonth': 8, u'cardHolderFullName': u'LUK/LAWRENCE', u'cardHolderLastName': u'LUK', u'cardHolderFirstName': u'LAWRENCE', u'expirationYear': 2019, u'expirationDate': 31, u'numberLast4': u'4308', u'type': u'VISA', u'id': 244219, u'numberFirst6': u'414720'}, u'debit': False}, u'references': [{u'type': u'POYNT_ORDER', u'id': u'01a0c6f9-015a-1000-e3ce-bbbd758e32de'}], u'updatedAt': u'2017-02-03T02:34:14Z', u'processorResponse': {u'status': u'Successful', u'approvalCode': u'534216', u'retrievalRefNum': u'624fbc0f-794c-4866-a211-0f5be53b8345', u'batchId': u'1', u'emvTags': {u'0x8A': u'3030', u'0x89': u'454139324645'}, u'statusMessage': u'Successful', u'approvedAmount': 625, u'transactionId': u'624fbc0f-794c-4866-a211-0f5be53b8345', u'processor': u'MOCK', u'acquirer': u'CHASE_PAYMENTECH', u'statusCode': u'1'}, u'id': u'624fbc0f-794c-4866-a211-0f5be53b8345', u'createdAt': u'2017-02-03T01:40:09Z', u'amounts': {u'tipAmount': 68, u'cashbackAmount': 0, u'currency': u'USD', u'customerOptedNoTip': False, u'orderAmount': 625, u'transactionAmount': 693}, u'customerUserId': 5249839, u'partiallyApproved': False, u'authOnly': False, u'amountsAdjusted': True, u'pinCaptured': False, u'signatureRequired': False, u'actionVoid': False, u'signatureCaptured': True, u'context': {u'businessType': u'TEST_MERCHANT', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'storeId': u'c394627f-4f68-47fb-90a5-684ea801a352', u'mcc': u'5812', u'sourceApp': u'co.poynt.services', u'source': u'INSTORE', u'employeeUserId': 5247870, u'transmissionAtLocal': u'2017-02-03T01:40:08Z', u'storeDeviceId': u'urn:tid:48c54303-6d51-39af-bdeb-4af53f621652'}, u'action': u'AUTHORIZE', u'settled': False}, {u'status': u'CAPTURED', u'adjusted': False, u'links': [{u'href': u'624fbc0f-794c-4866-a211-0f5be53b8345', u'method': u'GET', u'rel': u'AUTHORIZE'}], u'voided': False, u'fundingSource': {u'entryDetails': {u'customerPresenceStatus': u'PRESENT', u'entryMode': u'INTEGRATED_CIRCUIT_CARD'}, u'type': u'CREDIT_DEBIT', u'card': {u'status': u'ACTIVE', u'expirationMonth': 8, u'cardHolderFullName': u'LUK/LAWRENCE', u'cardHolderLastName': u'LUK', u'cardHolderFirstName': u'LAWRENCE', u'expirationYear': 2019, u'expirationDate': 31, u'numberLast4': u'4308', u'type': u'VISA', u'id': 244219, u'numberFirst6': u'414720'}, u'debit': False}, u'references': [{u'type': u'POYNT_ORDER', u'id': u'01a0c6f9-015a-1000-e3ce-bbbd758e32de'}], u'updatedAt': u'2017-02-03T02:34:14Z', u'processorResponse': {u'status': u'Successful', u'approvalCode': u'283042', u'batchId': u'1', u'acquirer': u'CHASE_PAYMENTECH', u'transactionId': u'1b622bd1-c0db-4271-9fe1-4ed9fbf6610c', u'processor': u'MOCK', u'statusMessage': u'Successful', u'statusCode': u'1'}, u'id': u'1b622bd1-c0db-4271-9fe1-4ed9fbf6610c', u'createdAt': u'2017-02-03T02:34:14Z', u'amounts': {u'tipAmount': 68, u'cashbackAmount': 0, u'currency': u'USD', u'customerOptedNoTip': False, u'orderAmount': 625, u'transactionAmount': 693}, u'customerUserId': 5249839, u'partiallyApproved': False, u'parentId': u'624fbc0f-794c-4866-a211-0f5be53b8345', u'authOnly': False, u'amountsAdjusted': False, u'pinCaptured': False, u'signatureRequired': False, u'actionVoid': False, u'signatureCaptured': True, u'context': {u'businessType': u'TEST_MERCHANT', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'storeId': u'c394627f-4f68-47fb-90a5-684ea801a352', u'mcc': u'5812', u'source': u'INSTORE', u'employeeUserId': 5247870, u'storeDeviceId': u'urn:tid:48c54303-6d51-39af-bdeb-4af53f621652'}, u'action': u'CAPTURE', u'settled': False}], u'amounts': {u'netTotal': 625, u'capturedTotals': {u'currency': u'USD', u'tipAmount': 68, u'transactionAmount': 693, u'cashbackAmount': 0, u'orderAmount': 625}, u'currency': u'USD', u'discountTotal': 0, u'feeTotal': 0, u'subTotal': 625, u'taxTotal': 0}, u'items': [{u'status': u'FULFILLED', u'sku': u'water', u'unitOfMeasure': u'EACH', u'fee': 0, u'name': u'water', u'tax': 0, u'discount': 0, u'updatedAt': u'2017-02-03T01:40:21Z', u'taxExempted': False, u'productId': u'f9faac3a-be56-4bff-b60b-84987cf021cb', u'unitPrice': 125, u'id': 1, u'createdAt': u'2017-02-03T01:40:21Z', u'quantity': 5.0}], u'customerUserId': 5249839, u'orderNumber': u'4', u'statuses': {u'status': u'OPENED', u'transactionStatusSummary': u'COMPLETED', u'fulfillmentStatus': u'FULFILLED'}, u'context': {u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'storeId': u'c394627f-4f68-47fb-90a5-684ea801a352', u'source': u'INSTORE', u'employeeUserId': 5247870, u'storeDeviceId': u'urn:tid:48c54303-6d51-39af-bdeb-4af53f621652', u'transactionInstruction': u'NONE'}, u'updatedAt': u'2017-02-03T01:40:21Z', u'taxExempted': False, u'id': u'01a0c6f9-015a-1000-e3ce-bbbd758e32de', u'createdAt': u'2017-02-03T01:40:21Z'}], u'links': [{u'href': u'/businesses/18f071cc-5ed4-4b33-80c1-305056d42bfb/orders?startAt=2017-02-03T02%3A34%3A14Z&endAt=2017-08-28T06%3A46%3A08Z&limit=1', u'method': u'GET', u'rel': u'next'}]} {
Get all orders
Get all orders that match the specified filters. If no filter is specified it will fetch all orders for the business since it started. We currently return 10 records at a time with a HATEOS link to the next 10. The following optional filters are supported:
- startTimeSec: the time from which to start fetching transactions,
- limit: the number of order to fetch,
- cardNumberFirst6: limit results to orders with transactions done by cards starting with these 6 numbers,
- cardNumberLast4: limit results to orders with transactions done by cards ending with these 4 numbers,
- cardExpirationMonth: limit results to orders with transactions done by cards expiring in this month,
- cardExpirationYear: limit results to orders with transactions done by cards expiring in this year,
- cardHolderFirstName: limit results to orders with transactions done by cards with this card holder first name,
- cardHolderLastName: limit results to orders with transactions done by cards with this card holder last name,
- storeId: only fetch transactions for this store.
- orderStatus: only fetch orders with the status.
- includeStaysAll: inlcude stay orders.
- includeOrderHistories: include order histories for orders.
- includeOrderShipments: include orderShipments for orders.
- customerUserId: filter orders placed by the customer.
Arguments
businessId
path- string (required)
If-Modified-Since
header- string (optional)
startAt
query- string (optional)
startOffset
query- integer (optional)
endAt
query- string (optional)
timeType
query- string (optional)
limit
query- integer (optional)
cardNumberFirst6
query- string (optional)
cardNumberLast4
query- string (optional)
cardExpirationMonth
query- integer (optional)
cardExpirationYear
query- integer (optional)
cardHolderFirstName
query- string (optional)
cardHolderLastName
query- string (optional)
storeId
query- string (optional)
orderNumber
query- string (optional)
orderStatus
query- string (optional)
includeStaysAll
query- boolean (optional)
includeOrderHistories
query- boolean (optional)
includeOrderShipments
query- boolean (optional)
customerUserId
query- integer (optional)
Response
Returns a OrderList.
Definition
GET /businesses/{businessId}/orders/{orderId}
Sample Request
curl --location --request GET 'https://services.poynt.net/businesses/a3f24e9e-6764-42f2-bbcd-5541e7f3be38/orders/36adbb38-0186-1000-7a36-d7c56ae9c66c' \
--header 'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json'
Sample Response
{
"taxExempted": false,
"valid": true,
"accepted": true,
"createdAt": "2023-02-09T14:57:19Z",
"updatedAt": "2023-02-09T14:57:19Z",
"context": {
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:5312e878-e1a2-3676-bd78-d04640310b02",
"sourceApp": "co.poynt.register",
"transactionInstruction": "NONE",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "89d82d45-5a56-4446-a04e-4340d417b841",
"channelId": "89d82d45-5a56-4446-a04e-4340d417b841"
},
"customer": {
"createdAt": "2023-02-09T14:57:10Z",
"updatedAt": "2023-02-09T14:57:10Z",
"id": 442274438,
"emails": {},
"phones": {}
},
"discounts": [],
"fees": [],
"orderHistories": [
{
"timestamp": "2023-02-09T14:57:19Z",
"id": 41801449,
"event": "ACCEPTED"
}
],
"items": [
{
"taxExempted": false,
"createdAt": "2023-02-09T14:57:19Z",
"updatedAt": "2023-02-09T14:57:19Z",
"quantity": 1,
"id": 1,
"discounts": [],
"fees": [],
"taxes": [
{
"taxExempted": false,
"catalogLevel": true,
"amountPrecision": 6,
"amount": 2000000,
"taxRateFixedAmount": 200,
"id": "e0e66ef1-ac18-40a5-a505-461dcc1c8a25",
"type": "custom"
}
],
"selectedVariants": [],
"unitPrice": 800,
"discount": 0,
"fee": 0,
"tax": 200,
"status": "FULFILLED",
"name": "New-Improved Cheetos!",
"productId": "35fac750-77be-4d8a-b20c-18621bffc038",
"sku": "SK-c368706a-b0b1-4de3-808a-f86e35ff5250",
"unitOfMeasure": "EACH"
},
{
"taxExempted": false,
"createdAt": "2023-02-09T14:57:19Z",
"updatedAt": "2023-02-09T14:57:19Z",
"quantity": 1,
"id": 2,
"discounts": [],
"fees": [],
"taxes": [
{
"taxExempted": false,
"catalogLevel": true,
"amountPrecision": 6,
"amount": 2000000,
"taxRateFixedAmount": 200,
"id": "e0e66ef1-ac18-40a5-a505-461dcc1c8a25",
"type": "custom"
}
],
"selectedVariants": [],
"unitPrice": 500,
"discount": 0,
"fee": 0,
"tax": 200,
"status": "FULFILLED",
"name": "Coffee",
"productId": "b584efda-8509-4183-b62c-dcdc933551fd",
"sku": "coffee",
"unitOfMeasure": "EACH"
}
],
"orderShipments": [
{
"address": {
"countryCode": "USA"
},
"createdAt": "2023-02-09T14:57:19Z",
"updatedAt": "2023-02-09T14:57:19Z",
"id": 55617784,
"deliveryMode": "FOR_HERE",
"status": "NONE",
"shipmentType": "FULFILLMENT"
}
],
"transactions": [
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": false,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2023-02-09T14:57:10Z",
"updatedAt": "2023-02-09T14:57:10Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2023-02-09T14:57:07Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:5312e878-e1a2-3676-bd78-d04640310b02",
"sourceApp": "co.poynt.register",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "89d82d45-5a56-4446-a04e-4340d417b841",
"channelId": "89d82d45-5a56-4446-a04e-4340d417b841"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2024,
"id": 442379758,
"numberFirst6": "441779",
"numberLast4": "1678",
"numberMasked": "441779******1678",
"cardId": "3fc78223-30b9-4152-9cc0-7fae6291cc8f"
},
"emvData": {
"emvTags": {
"0x9F36": "000C",
"0x5F34": "00",
"0x9F37": "7D82DA80",
"0x9F35": "22",
"0x9F10": "1F420132A0000000001003027300000000400000000000000000000000000000",
"0x9F33": "E0F0C8",
"0x4F": "A0000000031010",
"0x84": "A0000000031010",
"0x5F2A": "0840",
"0x82": "0040",
"0x9F6C": "0080",
"0x1F8231": "16",
"0x9F27": "80",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F03": "000000000000",
"0x9B": "0000",
"0x5F24": "241231",
"0x9F26": "580D5F6189497DEB",
"0x9A": "230209",
"0x9F01": "000000000000",
"0x9F02": "000000001700",
"0x9F24": "5630303130303135383230303331323632363337333232313336373630",
"0x9F66": "36804000",
"0x95": "0000000000",
"0x9F1A": "0840",
"0x8A": "3030"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_INTEGRATED_CIRCUIT_CARD"
},
"type": "CREDIT_DEBIT"
},
"references": [
{
"id": "36adbb38-0186-1000-7a36-d7c56ae9c66c",
"type": "POYNT_ORDER"
}
],
"customerUserId": 442274438,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"approvedAmount": 1700,
"emvTags": {
"0x8A": "3030",
"0x89": "454139324645"
},
"processor": "MOCK",
"acquirer": "ELAVON",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "36ae2285-0186-1000-1b52-368cbb5df3ed",
"approvalCode": "419763",
"batchId": "1",
"retrievalRefNum": "36ae2285-0186-1000-1b52-368cbb5df3ed"
},
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "SALE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 1700,
"orderAmount": 1700,
"tipAmount": 0,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "36ae2285-0186-1000-1b52-368cbb5df3ed"
}
],
"customerUserId": 442274438,
"amounts": {
"subTotal": 1300,
"discountTotal": 0,
"feeTotal": 0,
"taxTotal": 400,
"netTotal": 1700,
"currency": "USD",
"capturedTotals": {
"transactionFees": [],
"transactionAmount": 1700,
"orderAmount": 1700,
"tipAmount": 0,
"cashbackAmount": 0,
"currency": "USD"
}
},
"statuses": {
"fulfillmentStatus": "FULFILLED",
"status": "COMPLETED",
"transactionStatusSummary": "COMPLETED"
},
"orderNumber": "2",
"id": "36adbb38-0186-1000-7a36-d7c56ae9c66c"
}
Definition
.exports.getOrders = function getOrders(options, next) { ... }
module/**
* Get all orders at a business.
* @param {String} options.businessId
* @param {String} options.startAt (optional) - the time from which to start fetching orders
* @param {Integer} options.startOffset (optional)
* @param {String} options.endAt (optional) - the time at which to stop fetching orders
* @param {Integer} options.limit (optional) - the number of orders to fetch
* @param {String} options.cardNumberFirst6 (optional) - limit results to orders with transactions done by cards starting with these 6 numbers
* @param {String} options.cardNumberLast4 (optional) - limit results to orders with transactions done by cards ending with these 4 numbers
* @param {Integer} options.cardExpirationMonth (optional) - limit results to orders with transactions done by cards expiring in this month
* @param {Integer} options.cardExpirationYear (optional) - limit results to orders with transactions done by cards expiring in this year
* @param {String} options.cardHolderFirstName (optional) - limit results to orders with transactions done by cards with this card holder first name
* @param {String} options.cardHolderLastName (optional) - limit results to orders with transactions done by cards with this card holder last name
* @param {String} options.storeId (optional) - only fetch orders for this store
* @param {String} options.includeStaysAll (optional)
* @return {OrderList} orders
*/
Sample Request
.getOrder({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
orderId : '01a0c6f9-015a-1000-e3ce-bbbd758e32de'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
})
Sample Response
{"amounts": {
"capturedTotals": {
"cashbackAmount": 0,
"currency": "USD",
"orderAmount": 625,
"tipAmount": 68,
"transactionAmount": 693
,
}"currency": "USD",
"discountTotal": 0,
"feeTotal": 0,
"netTotal": 625,
"subTotal": 625,
"taxTotal": 0
,
}"context": {
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"employeeUserId": 5247870,
"source": "INSTORE",
"storeDeviceId": "urn:tid:48c54303-6d51-39af-bdeb-4af53f621652",
"storeId": "c394627f-4f68-47fb-90a5-684ea801a352",
"transactionInstruction": "NONE"
,
}"createdAt": "2017-02-03T01:40:21Z",
"customerUserId": 5249839,
"discounts": [],
"fees": [],
"id": "01a0c6f9-015a-1000-e3ce-bbbd758e32de",
"items": [
{"createdAt": "2017-02-03T01:40:21Z",
"discount": 0,
"discounts": [],
"fee": 0,
"fees": [],
"id": 1,
"name": "water",
"productId": "f9faac3a-be56-4bff-b60b-84987cf021cb",
"quantity": 5,
"selectedVariants": [],
"sku": "water",
"status": "FULFILLED",
"tax": 0,
"taxExempted": false,
"taxes": [],
"unitOfMeasure": "EACH",
"unitPrice": 125,
"updatedAt": "2017-02-03T01:40:21Z"
},
]"orderNumber": "4",
"statuses": {
"fulfillmentStatus": "FULFILLED",
"status": "OPENED",
"transactionStatusSummary": "COMPLETED"
,
}"taxExempted": false,
"transactions": [
{"action": "AUTHORIZE",
"actionVoid": false,
"adjusted": true,
"amounts": {
"cashbackAmount": 0,
"currency": "USD",
"customerOptedNoTip": false,
"orderAmount": 625,
"tipAmount": 68,
"transactionAmount": 693
,
}"amountsAdjusted": true,
"authOnly": false,
"context": {
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"businessType": "TEST_MERCHANT",
"employeeUserId": 5247870,
"mcc": "5812",
"source": "INSTORE",
"sourceApp": "co.poynt.services",
"storeDeviceId": "urn:tid:48c54303-6d51-39af-bdeb-4af53f621652",
"storeId": "c394627f-4f68-47fb-90a5-684ea801a352",
"transmissionAtLocal": "2017-02-03T01:40:08Z"
,
}"createdAt": "2017-02-03T01:40:09Z",
"customerUserId": 5249839,
"fundingSource": {
"card": {
"cardHolderFirstName": "LAWRENCE",
"cardHolderFullName": "LUK/LAWRENCE",
"cardHolderLastName": "LUK",
"expirationDate": 31,
"expirationMonth": 8,
"expirationYear": 2019,
"id": 244219,
"numberFirst6": "414720",
"numberLast4": "4308",
"status": "ACTIVE",
"type": "VISA"
,
}"debit": false,
"emvData": {
"emvTags": {
"0x50": "5649534120435245444954",
"0x5F20": "4C554B2F4C415752454E434520",
"0x5F24": "190831",
"0x5F2A": "0840",
"0x5F34": "02",
"0x82": "3C00",
"0x84": "A0000000031010",
"0x95": "0080008000",
"0x9A": "170202",
"0x9B": "E800",
"0x9C": "00",
"0x9F02": "000000000625",
"0x9F03": "000000000000",
"0x9F06": "A0000000031010",
"0x9F10": "06010A03A0A802",
"0x9F12": "43484153452056495341",
"0x9F1A": "0840",
"0x9F26": "376393F3F6B156AA",
"0x9F27": "80",
"0x9F33": "E0F0C8",
"0x9F34": "5E0000",
"0x9F35": "21",
"0x9F36": "0036",
"0x9F37": "94F3A4C5"
},
}"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "INTEGRATED_CIRCUIT_CARD"
,
}"type": "CREDIT_DEBIT"
,
}"id": "624fbc0f-794c-4866-a211-0f5be53b8345",
"links": [
{"href": "1b622bd1-c0db-4271-9fe1-4ed9fbf6610c",
"method": "GET",
"rel": "CAPTURE"
},
]"partiallyApproved": false,
"pinCaptured": false,
"processorResponse": {
"acquirer": "CHASE_PAYMENTECH",
"approvalCode": "534216",
"approvedAmount": 625,
"batchId": "1",
"emvTags": {
"0x89": "454139324645",
"0x8A": "3030"
,
}"processor": "MOCK",
"retrievalRefNum": "624fbc0f-794c-4866-a211-0f5be53b8345",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "624fbc0f-794c-4866-a211-0f5be53b8345"
,
}"references": [
{"id": "01a0c6f9-015a-1000-e3ce-bbbd758e32de",
"type": "POYNT_ORDER"
},
]"settled": false,
"signatureCaptured": true,
"signatureRequired": false,
"status": "CAPTURED",
"updatedAt": "2017-02-03T02:34:14Z",
"voided": false
,
}
{"action": "CAPTURE",
"actionVoid": false,
"adjusted": false,
"amounts": {
"cashbackAmount": 0,
"currency": "USD",
"customerOptedNoTip": false,
"orderAmount": 625,
"tipAmount": 68,
"transactionAmount": 693
,
}"amountsAdjusted": false,
"authOnly": false,
"context": {
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"businessType": "TEST_MERCHANT",
"employeeUserId": 5247870,
"mcc": "5812",
"source": "INSTORE",
"storeDeviceId": "urn:tid:48c54303-6d51-39af-bdeb-4af53f621652",
"storeId": "c394627f-4f68-47fb-90a5-684ea801a352"
,
}"createdAt": "2017-02-03T02:34:14Z",
"customerUserId": 5249839,
"fundingSource": {
"card": {
"cardHolderFirstName": "LAWRENCE",
"cardHolderFullName": "LUK/LAWRENCE",
"cardHolderLastName": "LUK",
"expirationDate": 31,
"expirationMonth": 8,
"expirationYear": 2019,
"id": 244219,
"numberFirst6": "414720",
"numberLast4": "4308",
"status": "ACTIVE",
"type": "VISA"
,
}"debit": false,
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "INTEGRATED_CIRCUIT_CARD"
,
}"type": "CREDIT_DEBIT"
,
}"id": "1b622bd1-c0db-4271-9fe1-4ed9fbf6610c",
"links": [
{"href": "624fbc0f-794c-4866-a211-0f5be53b8345",
"method": "GET",
"rel": "AUTHORIZE"
},
]"parentId": "624fbc0f-794c-4866-a211-0f5be53b8345",
"partiallyApproved": false,
"pinCaptured": false,
"processorResponse": {
"acquirer": "CHASE_PAYMENTECH",
"approvalCode": "283042",
"batchId": "1",
"processor": "MOCK",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "1b622bd1-c0db-4271-9fe1-4ed9fbf6610c"
,
}"references": [
{"id": "01a0c6f9-015a-1000-e3ce-bbbd758e32de",
"type": "POYNT_ORDER"
},
]"settled": false,
"signatureCaptured": true,
"signatureRequired": false,
"status": "CAPTURED",
"updatedAt": "2017-02-03T02:34:14Z",
"voided": false
},
]"updatedAt": "2017-02-03T01:40:21Z"
}
Definition
@classmethod
def get_orders(cls, business_id, start_at=None, start_offset=None,
=None, limit=None, card_number_first_6=None,
end_at=None, card_expiration_month=None,
card_number_last_4=None, card_holder_first_name=None,
card_expiration_year=None, store_id=None):
card_holder_last_name"""
Get all orders at a business by various criteria.
Arguments:
business_id (str): the business ID
Keyword arguments:
start_at (int, optional): get orders created after this time in seconds
start_offset (int, optional): the numeric offset to start the list (for pagination)
end_at (int, optional): get orders created before this time in seconds
limit (int, optional): how many orders to return (for pagination)
card_number_first_6 (str, optional): return orders with card numbers starting with this
card_number_last_4 (str, optional): return orders with card numbers ending with this
card_expiration_month (str, optional): return orders with this card expiration month
card_expiration_year (str, optional): return orders with this card expiration year
card_holder_first_name (str, optional): return orders with first name matching this
card_holder_last_name (str, optional): return orders with last name matching this
store_id (str, optional): return orders from this store
"""
Sample Request
= poynt.Order.get_order(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'01a0c6f9-015a-1000-e3ce-bbbd758e32de'
)
if status_code < 300:
print(doc)
Sample Response
u'transactions': [{u'status': u'CAPTURED', u'adjusted': True, u'links': [{u'href': u'1b622bd1-c0db-4271-9fe1-4ed9fbf6610c', u'method': u'GET', u'rel': u'CAPTURE'}], u'voided': False, u'fundingSource': {u'entryDetails': {u'customerPresenceStatus': u'PRESENT', u'entryMode': u'INTEGRATED_CIRCUIT_CARD'}, u'emvData': {u'emvTags': {u'0x5F34': u'02', u'0x9C': u'00', u'0x9B': u'E800', u'0x9F27': u'80', u'0x9F26': u'376393F3F6B156AA', u'0x9F03': u'000000000000', u'0x9F02': u'000000000625', u'0x9F06': u'A0000000031010', u'0x9F37': u'94F3A4C5', u'0x9A': u'170202', u'0x9F33': u'E0F0C8', u'0x84': u'A0000000031010', u'0x5F2A': u'0840', u'0x82': u'3C00', u'0x9F1A': u'0840', u'0x5F24': u'190831', u'0x9F36': u'0036', u'0x5F20': u'4C554B2F4C415752454E434520', u'0x9F34': u'5E0000', u'0x9F35': u'21', u'0x9F10': u'06010A03A0A802', u'0x9F12': u'43484153452056495341', u'0x50': u'5649534120435245444954', u'0x95': u'0080008000'}}, u'type': u'CREDIT_DEBIT', u'card': {u'status': u'ACTIVE', u'expirationMonth': 8, u'cardHolderFullName': u'LUK/LAWRENCE', u'cardHolderLastName': u'LUK', u'cardHolderFirstName': u'LAWRENCE', u'expirationYear': 2019, u'expirationDate': 31, u'numberLast4': u'4308', u'type': u'VISA', u'id': 244219, u'numberFirst6': u'414720'}, u'debit': False}, u'references': [{u'type': u'POYNT_ORDER', u'id': u'01a0c6f9-015a-1000-e3ce-bbbd758e32de'}], u'updatedAt': u'2017-02-03T02:34:14Z', u'processorResponse': {u'status': u'Successful', u'approvalCode': u'534216', u'retrievalRefNum': u'624fbc0f-794c-4866-a211-0f5be53b8345', u'batchId': u'1', u'emvTags': {u'0x8A': u'3030', u'0x89': u'454139324645'}, u'statusMessage': u'Successful', u'approvedAmount': 625, u'transactionId': u'624fbc0f-794c-4866-a211-0f5be53b8345', u'processor': u'MOCK', u'acquirer': u'CHASE_PAYMENTECH', u'statusCode': u'1'}, u'id': u'624fbc0f-794c-4866-a211-0f5be53b8345', u'createdAt': u'2017-02-03T01:40:09Z', u'amounts': {u'tipAmount': 68, u'cashbackAmount': 0, u'currency': u'USD', u'customerOptedNoTip': False, u'orderAmount': 625, u'transactionAmount': 693}, u'customerUserId': 5249839, u'partiallyApproved': False, u'authOnly': False, u'amountsAdjusted': True, u'pinCaptured': False, u'signatureRequired': False, u'actionVoid': False, u'signatureCaptured': True, u'context': {u'businessType': u'TEST_MERCHANT', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'storeId': u'c394627f-4f68-47fb-90a5-684ea801a352', u'mcc': u'5812', u'sourceApp': u'co.poynt.services', u'source': u'INSTORE', u'employeeUserId': 5247870, u'transmissionAtLocal': u'2017-02-03T01:40:08Z', u'storeDeviceId': u'urn:tid:48c54303-6d51-39af-bdeb-4af53f621652'}, u'action': u'AUTHORIZE', u'settled': False}, {u'status': u'CAPTURED', u'adjusted': False, u'links': [{u'href': u'624fbc0f-794c-4866-a211-0f5be53b8345', u'method': u'GET', u'rel': u'AUTHORIZE'}], u'voided': False, u'fundingSource': {u'entryDetails': {u'customerPresenceStatus': u'PRESENT', u'entryMode': u'INTEGRATED_CIRCUIT_CARD'}, u'type': u'CREDIT_DEBIT', u'card': {u'status': u'ACTIVE', u'expirationMonth': 8, u'cardHolderFullName': u'LUK/LAWRENCE', u'cardHolderLastName': u'LUK', u'cardHolderFirstName': u'LAWRENCE', u'expirationYear': 2019, u'expirationDate': 31, u'numberLast4': u'4308', u'type': u'VISA', u'id': 244219, u'numberFirst6': u'414720'}, u'debit': False}, u'references': [{u'type': u'POYNT_ORDER', u'id': u'01a0c6f9-015a-1000-e3ce-bbbd758e32de'}], u'updatedAt': u'2017-02-03T02:34:14Z', u'processorResponse': {u'status': u'Successful', u'approvalCode': u'283042', u'batchId': u'1', u'acquirer': u'CHASE_PAYMENTECH', u'transactionId': u'1b622bd1-c0db-4271-9fe1-4ed9fbf6610c', u'processor': u'MOCK', u'statusMessage': u'Successful', u'statusCode': u'1'}, u'id': u'1b622bd1-c0db-4271-9fe1-4ed9fbf6610c', u'createdAt': u'2017-02-03T02:34:14Z', u'amounts': {u'tipAmount': 68, u'cashbackAmount': 0, u'currency': u'USD', u'customerOptedNoTip': False, u'orderAmount': 625, u'transactionAmount': 693}, u'customerUserId': 5249839, u'partiallyApproved': False, u'parentId': u'624fbc0f-794c-4866-a211-0f5be53b8345', u'authOnly': False, u'amountsAdjusted': False, u'pinCaptured': False, u'signatureRequired': False, u'actionVoid': False, u'signatureCaptured': True, u'context': {u'businessType': u'TEST_MERCHANT', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'storeId': u'c394627f-4f68-47fb-90a5-684ea801a352', u'mcc': u'5812', u'source': u'INSTORE', u'employeeUserId': 5247870, u'storeDeviceId': u'urn:tid:48c54303-6d51-39af-bdeb-4af53f621652'}, u'action': u'CAPTURE', u'settled': False}], u'amounts': {u'netTotal': 625, u'capturedTotals': {u'currency': u'USD', u'tipAmount': 68, u'transactionAmount': 693, u'cashbackAmount': 0, u'orderAmount': 625}, u'currency': u'USD', u'discountTotal': 0, u'feeTotal': 0, u'subTotal': 625, u'taxTotal': 0}, u'items': [{u'status': u'FULFILLED', u'sku': u'water', u'unitOfMeasure': u'EACH', u'fee': 0, u'name': u'water', u'discount': 0, u'selectedVariants': [], u'fees': [], u'tax': 0, u'taxes': [], u'discounts': [], u'updatedAt': u'2017-02-03T01:40:21Z', u'taxExempted': False, u'productId': u'f9faac3a-be56-4bff-b60b-84987cf021cb', u'unitPrice': 125, u'id': 1, u'createdAt': u'2017-02-03T01:40:21Z', u'quantity': 5.0}], u'updatedAt': u'2017-02-03T01:40:21Z', u'customerUserId': 5249839, u'orderNumber': u'4', u'discounts': [], u'statuses': {u'status': u'OPENED', u'transactionStatusSummary': u'COMPLETED', u'fulfillmentStatus': u'FULFILLED'}, u'context': {u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'storeId': u'c394627f-4f68-47fb-90a5-684ea801a352', u'source': u'INSTORE', u'employeeUserId': 5247870, u'storeDeviceId': u'urn:tid:48c54303-6d51-39af-bdeb-4af53f621652', u'transactionInstruction': u'NONE'}, u'fees': [], u'taxExempted': False, u'id': u'01a0c6f9-015a-1000-e3ce-bbbd758e32de', u'createdAt': u'2017-02-03T01:40:21Z'} {
Get an order
Get an order (along with HATEOS links to all immediately related orders).
Arguments
If-Modified-Since
header- string (optional)
businessId
path- string (required)
orderId
path- string (required)
Response
Returns a Order.
Definition
GET /businesses/{businessId}/orders/{orderId}/shipments
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Get order shipments
Get all order shipments for a given order.
Arguments
businessId
path- string (required)
orderId
path- string (required)
Response
Returns a array.
Definition
GET /businesses/{businessId}/orders/{orderId}/histories
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Get order history events
Get all order history events for a given order.
Arguments
businessId
path- string (required)
orderId
path- string (required)
Response
Returns a array.
Definition
PUT /businesses/{businessId}/orders/{orderId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
This method is currently not possible using the Node.js SDK.
Definition
This method is currently not possible using the Python SDK.
Record order
Record an order that has already happened. A full order object must be populated as this operation will simply record what is passed to it.
Arguments
Poynt-Request-Id
header- string (required)
businessId
path- string (required)
orderId
path- string (required)
order
body- Order (optional)
Response
Returns a Order.
Definition
PATCH /businesses/{businessId}/orders/{orderId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
This method is currently not possible using the Node.js SDK.
Definition
This method is currently not possible using the Python SDK.
Update order
Update an order. This takes a JsonPatch object as request. We allow updates to order level amounts and replace on /items and /discounts.
Arguments
Poynt-Request-Id
header- string (required)
businessId
path- string (required)
orderId
path- string (required)
patch
body- JsonPatch (optional)
Response
Returns a Order.
Transactions
Transactions resource represents a financial transaction. It has operations to manage the entire lifecycle of a transaction initiated through MSR, manual key-in, NFC or EMV. It provides operations to:
- save a transaction at the Poynt server for later processing,
- record a transaction that has already happened – maybe offline or through some other path with the acquirer,
- initiate interaction with the acquirer to move funds (for a previously saved transaction or a brand new transaction),
- capture, void or update a previously authorized transaction,
- refund a previously completed transaction,
- record EMV tags associated with an authorized transaction or
- get details about all transactions meeting a certain filter criteria.
Definition
GET /businesses/{businessId}/transactions
Sample Request
curl --location --request GET 'https://services.poynt.net/businesses/a3f24e9e-6764-42f2-bbcd-5541e7f3be38/transactions' \ --header 'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json'
Sample Response
{
"links": [
{
"href": "/businesses/a3f24e9e-6764-42f2-bbcd-5541e7f3be38/transactions?startAt=2022-04-27T18%3A05%3A04Z&endAt=2023-02-10T09%3A08%3A53Z&startOffset=1",
"rel": "next",
"method": "GET"
}
],
"transactions": [
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-14T04:28:02Z",
"updatedAt": "2022-04-14T04:28:02Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-14T04:28:01Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2023,
"id": 369309072,
"numberFirst6": "405413",
"numberLast4": "6357",
"numberMasked": "4054136357",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/",
"serviceCode": "201",
"cardId": "6c08a9cc-b0f6-47a6-aa21-b1c85ab50a35"
},
"emvData": {
"emvTags": {
"0x1F8231": "18",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F37": "5384CD17",
"0x5F24": "231231",
"0x9F02": "000000002300",
"0x9F66": "82804000",
"0x5F20": "202F"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "908e5ea6-750c-4ee0-a067-4c749308ce33",
"type": "POYNT_ORDER"
}
],
"customerUserId": 369327767,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"approvedAmount": 2300,
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "265403b5-0180-1000-3394-854eaade8985",
"approvalCode": "188879",
"batchId": "1",
"retrievalRefNum": "265403b5-0180-1000-3394-854eaade8985"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 2300,
"orderAmount": 2300,
"tipAmount": 0,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "AUTHORIZED",
"id": "265403b5-0180-1000-3394-854eaade8985"
},
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": true,
"amountsAdjusted": true,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-14T20:31:06Z",
"updatedAt": "2022-04-14T20:32:22Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-14T20:31:05Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2023,
"id": 369442365,
"numberFirst6": "405413",
"numberLast4": "6357",
"numberMasked": "4054136357",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/",
"serviceCode": "201",
"cardId": "885f6b75-5e8f-4933-a55d-cc53a8dda6e9"
},
"emvData": {
"emvTags": {
"0x1F8231": "18",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F37": "2F9BD81B",
"0x5F24": "231231",
"0x9F02": "000000002500",
"0x9F66": "82804000",
"0x5F20": "202F"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "71241c6f-ff98-46b2-879f-1805596465ca",
"rel": "CAPTURE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "38caea72-3965-48af-9dfe-ab81145abe4e",
"type": "POYNT_ORDER"
}
],
"customerUserId": 369458304,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"approvedAmount": 2500,
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "29c5b96d-0180-1000-d4b4-c6fb481f142d",
"approvalCode": "219475",
"batchId": "1",
"retrievalRefNum": "29c5b96d-0180-1000-d4b4-c6fb481f142d"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 7500,
"orderAmount": 2500,
"tipAmount": 5000,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "29c5b96d-0180-1000-d4b4-c6fb481f142d"
},
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": true,
"amountsAdjusted": true,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-14T20:36:25Z",
"updatedAt": "2022-04-14T20:37:13Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-14T20:36:25Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2023,
"id": 369444391,
"numberFirst6": "405413",
"numberLast4": "6357",
"numberMasked": "4054136357",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/",
"serviceCode": "201",
"cardId": "3bf41a39-a5f3-4d2d-b660-70373e607556"
},
"emvData": {
"emvTags": {
"0x1F8231": "18",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F37": "402061F8",
"0x5F24": "231231",
"0x9F02": "000000004000",
"0x9F66": "82804000",
"0x5F20": "202F"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "89e33222-1319-4619-84e4-492a08789452",
"rel": "CAPTURE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "e34dff21-1226-4af2-a42b-ca15275bd9fa",
"type": "POYNT_ORDER"
}
],
"customerUserId": 369460286,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"approvedAmount": 4000,
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "29ca99f3-0180-1000-d4b4-c6fb481f142d",
"approvalCode": "158881",
"batchId": "1",
"retrievalRefNum": "29ca99f3-0180-1000-d4b4-c6fb481f142d"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 5500,
"orderAmount": 4000,
"tipAmount": 1500,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "29ca99f3-0180-1000-d4b4-c6fb481f142d"
},
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-14T21:10:55Z",
"updatedAt": "2022-04-14T21:11:29Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-14T21:10:54Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2023,
"id": 369457464,
"numberFirst6": "405413",
"numberLast4": "6357",
"numberMasked": "4054136357",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/",
"serviceCode": "201",
"cardId": "be92c1e4-2fdd-4b28-a2ab-57addb085115"
},
"emvData": {
"emvTags": {
"0x1F8231": "18",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F37": "8CF4ACD8",
"0x5F24": "231231",
"0x9F02": "000000008000",
"0x9F66": "82804000",
"0x5F20": "202F"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "96d6ec95-aae0-45a9-bf29-2735f460ee59",
"rel": "CAPTURE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "ca62c3bb-90aa-4563-bd69-f95d91287627",
"type": "POYNT_ORDER"
}
],
"customerUserId": 369473069,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"approvedAmount": 8000,
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "29ea2e4d-0180-1000-d4b4-c6fb481f142d",
"approvalCode": "302550",
"batchId": "1",
"retrievalRefNum": "29ea2e4d-0180-1000-d4b4-c6fb481f142d"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 8000,
"orderAmount": 5500,
"tipAmount": 2500,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "29ea2e4d-0180-1000-d4b4-c6fb481f142d"
},
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-14T21:12:29Z",
"updatedAt": "2022-04-14T21:12:29Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-14T21:12:28Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2023,
"id": 369458037,
"numberFirst6": "405413",
"numberLast4": "6357",
"numberMasked": "4054136357",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/",
"serviceCode": "201",
"cardId": "831f298b-0622-4e2a-9ad5-3a7cfabbb565"
},
"emvData": {
"emvTags": {
"0x1F8231": "18",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F37": "060F6C27",
"0x5F24": "231231",
"0x9F02": "000000009000",
"0x9F66": "82804000",
"0x5F20": "202F"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "4de10d8c-94b0-40c1-a4c6-d1420a543963",
"type": "POYNT_ORDER"
}
],
"customerUserId": 369473629,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"approvedAmount": 9000,
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "29eb9e2e-0180-1000-d4b4-c6fb481f142d",
"approvalCode": "528437",
"batchId": "1",
"retrievalRefNum": "29eb9e2e-0180-1000-d4b4-c6fb481f142d"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 9000,
"orderAmount": 6000,
"tipAmount": 3000,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "AUTHORIZED",
"id": "29eb9e2e-0180-1000-d4b4-c6fb481f142d"
},
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-14T21:13:45Z",
"updatedAt": "2022-04-22T18:03:53Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-14T21:13:44Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2023,
"id": 369458474,
"numberFirst6": "405413",
"numberLast4": "6357",
"numberMasked": "4054136357",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/",
"serviceCode": "201",
"cardId": "e39a4f40-73f3-40b7-896e-4c4263d2ebb0"
},
"emvData": {
"emvTags": {
"0x1F8231": "18",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F37": "49249314",
"0x5F24": "231231",
"0x9F02": "000000006500",
"0x9F66": "82804000",
"0x5F20": "202F"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "89f9d20a-9b27-41f2-aea7-0c96939caaae",
"rel": "CAPTURE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "1800d850-34ea-4213-81d4-793dc640fe55",
"type": "POYNT_ORDER"
}
],
"customerUserId": 369474054,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"approvedAmount": 6500,
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "29ecc5cf-0180-1000-d4b4-c6fb481f142d",
"approvalCode": "687942",
"batchId": "1",
"retrievalRefNum": "29ecc5cf-0180-1000-d4b4-c6fb481f142d"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 6500,
"orderAmount": 2500,
"tipAmount": 4000,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "REFUNDED",
"id": "29ecc5cf-0180-1000-d4b4-c6fb481f142d"
},
{
"signatureRequired": true,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": false,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-27T18:03:03Z",
"updatedAt": "2022-04-27T18:03:14Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-27T18:03:02Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 7,
"expirationYear": 2020,
"id": 372381668,
"numberFirst6": "473702",
"numberLast4": "2897",
"numberMasked": "4737022897",
"cardHolderFirstName": "KASTUREEMA",
"cardHolderLastName": "SARMA",
"cardHolderFullName": "SARMA/ KASTUREEMA",
"serviceCode": "201",
"cardId": "dde252ec-02f1-462d-9ea4-b61568006cea"
},
"emvData": {
"emvTags": {
"0x5F34": "00",
"0x9F36": "01BF",
"0x9F37": "384802E3",
"0x9F34": "5E0000",
"0x9F35": "21",
"0x9F10": "06010A03600000",
"0x9F33": "E0F0C8",
"0x84": "A0000000031010",
"0x5F2A": "0840",
"0x9F0E": "0000000000",
"0x82": "1800",
"0x9F0F": "FC70BC9800",
"0x9F0D": "FC50AC8800",
"0x9F09": "008C",
"0x9F07": "FF80",
"0x9F08": "0096",
"0x1F8231": "87",
"0x5F28": "0840",
"0x9F27": "40",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9B": "6800",
"0x9F03": "000000000000",
"0x9A": "220427",
"0x9F26": "0CD6F292E998049C",
"0x5F24": "200731",
"0x9F02": "000000002500",
"0x5F20": "5341524D412F204B415354555245454D41202020202020202020",
"0x95": "80C0008000",
"0x50": "56495341204445424954",
"0x9F1A": "0840"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "INTEGRATED_CIRCUIT_CARD"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "ecb82bdc-f355-4f9d-8656-929d238c424f",
"rel": "CAPTURE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "112ea7d1-b821-4579-8afe-3112778596c0",
"type": "POYNT_ORDER"
}
],
"customerUserId": 372348487,
"processorOptions": {},
"processorResponse": {
"approvedAmount": 2500,
"emvTags": {
"0x8A": "3030",
"0x89": "454139324645"
},
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "6c30db9b-0180-1000-7603-9ebe5180647c",
"approvalCode": "870321",
"batchId": "1",
"retrievalRefNum": "6c30db9b-0180-1000-7603-9ebe5180647c"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 2500,
"orderAmount": 2500,
"tipAmount": 0,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "6c30db9b-0180-1000-7603-9ebe5180647c"
},
{
"signatureRequired": true,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-27T18:03:38Z",
"updatedAt": "2022-04-27T18:03:50Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-27T18:03:38Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 7,
"expirationYear": 2020,
"id": 372381880,
"numberFirst6": "473702",
"numberLast4": "2897",
"numberMasked": "4737022897",
"cardHolderFirstName": "KASTUREEMA",
"cardHolderLastName": "SARMA",
"cardHolderFullName": "SARMA/ KASTUREEMA",
"serviceCode": "201",
"cardId": "b19e3be7-138a-4dc5-a468-8b43b5ef8a49"
},
"emvData": {
"emvTags": {
"0x5F34": "00",
"0x9F36": "01C0",
"0x9F37": "19886D6B",
"0x9F34": "5E0000",
"0x9F35": "21",
"0x9F10": "06010A03600000",
"0x9F33": "E0F0C8",
"0x84": "A0000000031010",
"0x5F2A": "0840",
"0x9F0E": "0000000000",
"0x82": "1800",
"0x9F0F": "FC70BC9800",
"0x9F0D": "FC50AC8800",
"0x9F09": "008C",
"0x9F07": "FF80",
"0x9F08": "0096",
"0x1F8231": "87",
"0x5F28": "0840",
"0x9F27": "40",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9B": "6800",
"0x9F03": "000000000000",
"0x9A": "220427",
"0x9F26": "E6822924CDDAB441",
"0x5F24": "200731",
"0x9F02": "000000004300",
"0x5F20": "5341524D412F204B415354555245454D41202020202020202020",
"0x95": "80C0008000",
"0x50": "56495341204445424954",
"0x9F1A": "0840"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "INTEGRATED_CIRCUIT_CARD"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "7f1e5cc7-1dfa-4248-b7a2-3f9317b38a24",
"rel": "CAPTURE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "29198bfb-620b-41ca-940d-cae9fb415bcf",
"type": "POYNT_ORDER"
}
],
"customerUserId": 372348695,
"processorOptions": {},
"processorResponse": {
"approvedAmount": 4300,
"emvTags": {
"0x8A": "3030",
"0x89": "454139324645"
},
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "6c3166d4-0180-1000-7603-9ebe5180647c",
"approvalCode": "166143",
"batchId": "1",
"retrievalRefNum": "6c3166d4-0180-1000-7603-9ebe5180647c"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 4300,
"orderAmount": 4300,
"tipAmount": 0,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "6c3166d4-0180-1000-7603-9ebe5180647c"
},
{
"signatureRequired": true,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": true,
"amountsAdjusted": true,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-27T18:04:08Z",
"updatedAt": "2022-04-27T18:04:31Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-27T18:04:08Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 7,
"expirationYear": 2020,
"id": 372382095,
"numberFirst6": "473702",
"numberLast4": "2897",
"numberMasked": "4737022897",
"cardHolderFirstName": "KASTUREEMA",
"cardHolderLastName": "SARMA",
"cardHolderFullName": "SARMA/ KASTUREEMA",
"serviceCode": "201",
"cardId": "ef0b39f1-116c-4295-9761-a2951314b156"
},
"emvData": {
"emvTags": {
"0x5F34": "00",
"0x9F36": "01C1",
"0x9F37": "0EC12581",
"0x9F34": "5E0000",
"0x9F35": "21",
"0x9F10": "06010A03600000",
"0x9F33": "E0F0C8",
"0x84": "A0000000031010",
"0x5F2A": "0840",
"0x9F0E": "0000000000",
"0x82": "1800",
"0x9F0F": "FC70BC9800",
"0x9F0D": "FC50AC8800",
"0x9F09": "008C",
"0x9F07": "FF80",
"0x9F08": "0096",
"0x1F8231": "87",
"0x5F28": "0840",
"0x9F27": "40",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9B": "6800",
"0x9F03": "000000000000",
"0x9A": "220427",
"0x9F26": "870DF41226E24EAC",
"0x5F24": "200731",
"0x9F02": "000000004500",
"0x5F20": "5341524D412F204B415354555245454D41202020202020202020",
"0x95": "80C0008000",
"0x50": "56495341204445424954",
"0x9F1A": "0840"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "INTEGRATED_CIRCUIT_CARD"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "6b0d6b3d-e9da-419a-937e-f18652a6cac1",
"rel": "CAPTURE",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "8471f591-252b-4112-bae1-02288161911a",
"type": "POYNT_ORDER"
}
],
"customerUserId": 372348900,
"processorOptions": {},
"processorResponse": {
"approvedAmount": 4500,
"emvTags": {
"0x8A": "3030",
"0x89": "454139324645"
},
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "6c31dbef-0180-1000-7603-9ebe5180647c",
"approvalCode": "831188",
"batchId": "1",
"retrievalRefNum": "6c31dbef-0180-1000-7603-9ebe5180647c"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 5000,
"orderAmount": 4500,
"tipAmount": 500,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "6c31dbef-0180-1000-7603-9ebe5180647c"
},
{
"signatureRequired": true,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": true,
"partiallyApproved": false,
"actionVoid": false,
"voided": true,
"settled": false,
"reversalVoid": false,
"createdAt": "2022-04-27T18:04:52Z",
"updatedAt": "2022-04-27T18:05:04Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2022-04-27T18:04:52Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:c1e269b9-5afe-379e-b28c-c63a3373f3af",
"sourceApp": "co.poynt.posconnector",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 7,
"expirationYear": 2020,
"id": 372382389,
"numberFirst6": "473702",
"numberLast4": "2897",
"numberMasked": "4737022897",
"cardHolderFirstName": "KASTUREEMA",
"cardHolderLastName": "SARMA",
"cardHolderFullName": "SARMA/ KASTUREEMA",
"serviceCode": "201",
"cardId": "d5d8f42c-84e5-49ef-8bb2-112604fb0cf1"
},
"emvData": {
"emvTags": {
"0x5F34": "00",
"0x9F36": "01C2",
"0x9F37": "65C9902E",
"0x9F34": "5E0000",
"0x9F35": "21",
"0x9F10": "06010A03600000",
"0x9F33": "E0F0C8",
"0x84": "A0000000031010",
"0x5F2A": "0840",
"0x9F0E": "0000000000",
"0x82": "1800",
"0x9F0F": "FC70BC9800",
"0x9F0D": "FC50AC8800",
"0x9F09": "008C",
"0x9F07": "FF80",
"0x9F08": "0096",
"0x1F8231": "87",
"0x5F28": "0840",
"0x9F27": "40",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9B": "6800",
"0x9F03": "000000000000",
"0x9A": "220427",
"0x9F26": "FC14EB2A11C5B560",
"0x5F24": "200731",
"0x9F02": "000000006000",
"0x5F20": "5341524D412F204B415354555245454D41202020202020202020",
"0x95": "80C0008000",
"0x50": "56495341204445424954",
"0x9F1A": "0840"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "INTEGRATED_CIRCUIT_CARD"
},
"type": "CREDIT_DEBIT"
},
"links": [
{
"href": "ba5953f1-f637-4b2d-8639-4e2887d80d4c",
"rel": "REFUND",
"method": "GET"
}
],
"references": [
{
"id": "",
"customType": "externalReferenceId",
"type": "CUSTOM"
},
{
"id": "afc4a299-7f78-4c4c-964e-c481af333ee5",
"type": "POYNT_ORDER"
}
],
"customerUserId": 372349189,
"processorOptions": {},
"processorResponse": {
"approvedAmount": 6000,
"emvTags": {
"0x8A": "3030",
"0x89": "454139324645"
},
"processor": "MOCK",
"acquirer": "CHASE_PAYMENTECH",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "6c3287cb-0180-1000-7603-9ebe5180647c",
"approvalCode": "373470",
"batchId": "1",
"retrievalRefNum": "6c3287cb-0180-1000-7603-9ebe5180647c"
},
"notes": "",
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "AUTHORIZE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 6000,
"orderAmount": 5500,
"tipAmount": 500,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "VOIDED",
"id": "6c3287cb-0180-1000-7603-9ebe5180647c"
}
],
"count": 315
}
Definition
.exports.getTransactions = function getTransactions(options, next) { ... }
module/**
* Get all transactions that match the specified filters.
* If no filter is specified it will fetch all transactions for the business
* since it started.
* @param {String} options.businessId
* @param {String} options.startAt (optional) - the time from which to start fetching transactions
* @param {Integer} options.startOffset (optional)
* @param {String} options.endAt (optional) - the time at which to stop fetching transactions
* @param {Integer} options.limit (optional) - the number of transactions to fetch
* @param {String} options.storeId (optional) - only fetch transactions for this store
* @param {String} options.deviceId (optional) - only fetch transactions for this device
* @param {String} options.searchKey (optional) - instead of specifying which exact field to look at, the client can simply pass this search key and the server will look at various different fields,
* @param {String} options.cardNumberFirst6 (optional) - limit results to transactions done by cards starting with these 6 numbers
* @param {String} options.cardNumberLast4 (optional) - limit results to transactions done by cards ending with these 4 numbers
* @param {Integer} options.cardExpirationMonth (optional) - limit results to transactions done by cards expiring in this month
* @param {Integer} options.cardExpirationYear (optional) - limit results to transactions done by cards expiring in this year
* @param {String} options.cardHolderFirstName (optional) - limit results to transactions done by cards with this card holder first name
* @param {String} options.cardHolderLastName (optional) - limit results to transactions done by cards with this card holder last name
* @param {String} options.action (optional) - only fetch transactions with this action
* @param {String} options.status (optional) - only fetch transactions with this status
* @param {String} options.transactionIds (optional) - only fetch transactions matching these ids (comma separated)
* @param {Boolean} options.authOnly (optional) - only fetch auth only transactions
* @param {Boolean} options.unsettledOnly (optional) - only fetch unsettled transactions
* @param {Boolean} options.creditDebitOnly (optional) - only fetch credit/debit transactions
* @param {Boolean} options.includePending (optional) - include transactions with PENDING status
* @return {TransactionList} transactions
*/
Sample Request
.getTransactions({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
startOffset : 0,
limit : 1
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
})
Sample Response
{"count": 256,
"links": [
{"href": "/businesses/18f071cc-5ed4-4b33-80c1-305056d42bfb/transactions?startAt=2017-01-04T23%3A10%3A25Z&endAt=2017-08-28T06%3A56%3A17Z&limit=1",
"method": "GET",
"rel": "next"
},
]"transactions": [
{"action": "AUTHORIZE",
"actionVoid": false,
"adjusted": false,
"amounts": {
"cashbackAmount": 0,
"currency": "USD",
"customerOptedNoTip": false,
"orderAmount": 800,
"tipAmount": 0,
"transactionAmount": 800
,
}"amountsAdjusted": false,
"authOnly": false,
"context": {
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"businessType": "TEST_MERCHANT",
"employeeUserId": 5247838,
"mcc": "5812",
"source": "INSTORE",
"sourceApp": "co.poynt.services",
"storeDeviceId": "urn:tid:48c54303-6d51-39af-bdeb-4af53f621652",
"storeId": "c394627f-4f68-47fb-90a5-684ea801a352",
"transmissionAtLocal": "2017-01-04T22:20:40Z"
,
}"createdAt": "2017-01-04T22:20:40Z",
"customerUserId": 5247839,
"fundingSource": {
"card": {
"cardHolderFirstName": "",
"cardHolderFullName": "/",
"cardHolderLastName": "",
"expirationDate": 31,
"expirationMonth": 8,
"expirationYear": 2022,
"id": 243043,
"numberFirst6": "405413",
"numberLast4": "0833",
"status": "ACTIVE",
"type": "VISA"
,
}"debit": false,
"emvData": {
"emvTags": {
"0x5F20": "202F",
"0x5F24": "220831",
"0x9C": "00",
"0x9F02": "000000000800",
"0x9F06": "A0000000031010",
"0x9F37": "7619D4A6"
},
}"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
,
}"type": "CREDIT_DEBIT"
,
}"id": "9ee28946-cab1-48af-b30b-d111716128a2",
"partiallyApproved": false,
"pinCaptured": false,
"processorResponse": {
"acquirer": "CHASE_PAYMENTECH",
"approvalCode": "667149",
"approvedAmount": 800,
"batchId": "1",
"processor": "MOCK",
"retrievalRefNum": "9ee28946-cab1-48af-b30b-d111716128a2",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "9ee28946-cab1-48af-b30b-d111716128a2"
,
}"references": [
{"customType": "referenceId",
"id": "6b91d9a0-0159-1000-6f82-44ed3d4b4a5a",
"type": "CUSTOM"
},
]"settled": false,
"signatureCaptured": true,
"signatureRequired": false,
"status": "AUTHORIZED",
"updatedAt": "2017-01-04T22:20:40Z",
"voided": false
}
] }
Definition
@classmethod
def get_transactions(cls, business_id, start_at=None, start_offset=None,
=None, limit=None, card_number_first_6=None,
end_at=None, card_expiration_month=None,
card_number_last_4=None, card_holder_first_name=None,
card_expiration_year=None, store_id=None, device_id=None,
card_holder_last_name=None, action=None, status=None, transaction_ids=None,
search_key=None, unsettled_only=None, credit_debit_only=None):
auth_only"""
Get all transactions at a business by various criteria.
Arguments:
business_id (str): the business ID
Keyword arguments:
start_at (int, optional): get txns created after this time in seconds
start_offset (int, optional): the numeric offset to start the list (for pagination)
end_at (int, optional): get txns created before this time in seconds
limit (int, optional): how many txns to return (for pagination)
card_number_first_6 (str, optional): return txns with card numbers starting with this
card_number_last_4 (str, optional): return txns with card numbers ending with this
card_expiration_month (str, optional): return txns with this card expiration month
card_expiration_year (str, optional): return txns with this card expiration year
card_holder_first_name (str, optional): return txns with first name matching this
card_holder_last_name (str, optional): return txns with last name matching this
store_id (str, optional): return txns from this store
device_id (str, optional): return txns from this device
search_key (str, optional): instead of specifying which exact field to look at, the
client can simply pass this search key and the server will
look at various different fields,
action (str, optional): only fetch txns with this action
status (str, optional): only fetch txns with this status
transaction_ids (str, optional): only fetch txns matching these ids (comma separated)
auth_only (bool, optional): only fetch auth only txns
unsettled_only (bool, optional): only fetch unsettled txns
credit_debit_only (bool, optional): only fetch credit/debit txns
"""
Sample Request
= poynt.Transaction.get_transactions(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
=0,
start_offset=1
limit
)
if status_code < 300:
print(doc)
Sample Response
u'count': 256, u'links': [{u'href': u'/businesses/18f071cc-5ed4-4b33-80c1-305056d42bfb/transactions?startAt=2017-01-04T23%3A10%3A25Z&endAt=2017-08-28T06%3A57%3A16Z&limit=1', u'method': u'GET', u'rel': u'next'}], u'transactions': [{u'authOnly': False, u'status': u'AUTHORIZED', u'adjusted': False, u'context': {u'businessType': u'TEST_MERCHANT', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'storeId': u'c394627f-4f68-47fb-90a5-684ea801a352', u'mcc': u'5812', u'sourceApp': u'co.poynt.services', u'source': u'INSTORE', u'employeeUserId': 5247838, u'transmissionAtLocal': u'2017-01-04T22:20:40Z', u'storeDeviceId': u'urn:tid:48c54303-6d51-39af-bdeb-4af53f621652'}, u'pinCaptured': False, u'id': u'9ee28946-cab1-48af-b30b-d111716128a2', u'action': u'AUTHORIZE', u'customerUserId': 5247839, u'amountsAdjusted': False, u'partiallyApproved': False, u'voided': False, u'signatureRequired': False, u'fundingSource': {u'entryDetails': {u'customerPresenceStatus': u'PRESENT', u'entryMode': u'CONTACTLESS_MAGSTRIPE'}, u'emvData': {u'emvTags': {u'0x5F24': u'220831', u'0x9C': u'00', u'0x5F20': u'202F', u'0x9F02': u'000000000800', u'0x9F06': u'A0000000031010', u'0x9F37': u'7619D4A6'}}, u'type': u'CREDIT_DEBIT', u'card': {u'status': u'ACTIVE', u'expirationMonth': 8, u'cardHolderFullName': u'/', u'cardHolderLastName': u'', u'cardHolderFirstName': u'', u'expirationYear': 2022, u'expirationDate': 31, u'numberLast4': u'0833', u'type': u'VISA', u'id': 243043, u'numberFirst6': u'405413'}, u'debit': False}, u'amounts': {u'tipAmount': 0, u'cashbackAmount': 0, u'currency': u'USD', u'customerOptedNoTip': False, u'orderAmount': 800, u'transactionAmount': 800}, u'references': [{u'customType': u'referenceId', u'type': u'CUSTOM', u'id': u'6b91d9a0-0159-1000-6f82-44ed3d4b4a5a'}], u'actionVoid': False, u'updatedAt': u'2017-01-04T22:20:40Z', u'processorResponse': {u'status': u'Successful', u'approvalCode': u'667149', u'retrievalRefNum': u'9ee28946-cab1-48af-b30b-d111716128a2', u'batchId': u'1', u'acquirer': u'CHASE_PAYMENTECH', u'approvedAmount': 800, u'transactionId': u'9ee28946-cab1-48af-b30b-d111716128a2', u'processor': u'MOCK', u'statusMessage': u'Successful', u'statusCode': u'1'}, u'settled': False, u'signatureCaptured': True, u'createdAt': u'2017-01-04T22:20:40Z'}]} {
Get all transactions
Get all transactions that match the specified filters. If no filter is specified it will fetch all transactions for the business since it started. We currently return 10 records at a time with a HATEOS link to the next 10. The following optional filters are supported:
- If-Modified-Since: will only return transaction since If-Modified-Since,
- startAt: the time in seconds from which to start fetching transactions,
- startOffset: for all transactions at startAt the offset from where to start,
- endAt: the time in seconds at which to end,
- limit: the number of transactions to fetch in one go,
- storeId: only fetch transactions for this store,
- deviceId: only fetch transactions for this device,
- searchKey: instead of specifying which exact field to look at, the client can simply pass this search key and the server will look at various different fields,
- cardNumberFirst6: limit results to transactions done by cards starting with these 6 numbers,
- cardNumberLast4: limit results to transactions done by cards ending with these 4 numbers,
- cardExpirationMonth: limit results to transactions done by cards expiring in this month,
- cardExpirationYear: limit results to transactions done by cards expiring in this year,
- cardHolderFirstName: limit results to transactions done by cards with this card holder first name,
- cardHolderLastName: limit results to transactions done by cards with this card holder last name,
- transactionIds: only fetch transactions matching these transactionIds,
- authOny: only fetch authOnly transactions,
- unsettledOnly: only fetch unsettled transactions. note: this will limit result to creditDebitOnly,
- creditDebitOnly: only fetch credit-debit transactions. note: if one of the card filters or unsettledOnly filter above are enabled, it automatically limits result to credit-debit only,
- includePending: if true then response can include transactions with PENDING status. By default such transactions excluded from response,
Arguments
businessId
path- string (required)
If-Modified-Since
header- string (optional)
startAt
query- string (optional)
startOffset
query- integer (optional)
endAt
query- string (optional)
timeType
query- string (optional)
limit
query- integer (optional)
storeId
query- string (optional)
deviceId
query- string (optional)
tid
query- string (optional)
searchKey
query- string (optional)
cardNumberFirst6
query- string (optional)
cardNumberLast4
query- string (optional)
cardExpirationMonth
query- integer (optional)
cardExpirationYear
query- integer (optional)
cardHolderFirstName
query- string (optional)
cardHolderLastName
query- string (optional)
action
query- string (optional)
status
query- string (optional)
transactionIds
query- array (optional)
authOnly
query- boolean (optional)
unsettledOnly
query- boolean (optional)
creditDebitOnly
query- boolean (optional)
includePending
query- boolean (optional)
orderBy
query- string (optional)
Response
Returns a TransactionList.
Definition
POST /businesses/{businessId}/transactions
Sample Request
{
"_id": 0,
"_parentId": 0,
"_tcdId": 0,
"action": "AUTHORIZE",
"actionVoid": true,
"adjusted": true,
"adjustmentHistory": [
{
"amountChanges": {
"cashbackAmount": 2147483647,
"currency": "string",
"customerOptedNoTip": true,
"orderAmount": 2147483647,
"tipAmount": 2147483647,
"transactionFees": [
{
"amount": 0,
"feeProgramType": "SURCHARGE",
"feeType": "FIXED",
"required": true,
"signature": "string",
"feeProgramId": "927253be-0294-4a36-80e7-7d0d8c175f0c"
}
],
"transactionAmount": 2147483647
},
"amounts": {
"cashbackAmount": 2147483647,
"currency": "string",
"customerOptedNoTip": true,
"orderAmount": 2147483647,
"tipAmount": 2147483647,
"transactionFees": [
{
"amount": 0,
"feeProgramType": "SURCHARGE",
"feeType": "FIXED",
"required": true,
"signature": "string",
"feeProgramId": "927253be-0294-4a36-80e7-7d0d8c175f0c"
}
],
"transactionAmount": 2147483647
},
"createdAt": "2019-08-24T14:15:22Z",
"exchangeRate": {
"businessId": "50092e54-cd12-4059-9828-e8c4ee9a3cf5",
"cardAmount": 0,
"cardCurrency": "str",
"cardTipAmount": 0,
"disclaimer": "string",
"markupInfo1": "string",
"markupInfo2": "string",
"markupPercentage": "string",
"provider": "string",
"rate": 0,
"ratePrecision": 0,
"requestedAt": "2019-08-24T14:15:22Z",
"signature": "string",
"tipAmount": 0,
"txnAmount": 0,
"txnCurrency": "str"
},
"processorResponse": {
"acquirer": "CHASE_PAYMENTECH",
"adapterId": "string",
"adapterVariant": "string",
"approvalCode": "string",
"approvedAmount": 0,
"avsResult": {
"actualResult": "string",
"addressResult": "MATCH",
"cardHolderNameResult": "MATCH",
"cityResult": "MATCH",
"countryResult": "MATCH",
"phoneResult": "MATCH",
"postalCodeResult": "MATCH",
"stateResult": "MATCH"
},
"batchAutoClosedByHost": "string",
"batchId": "string",
"cardToken": "string",
"cvActualResult": "string",
"cvResult": "MATCH",
"debitResponseCode": "string",
"echeckProcessor": "MOCK",
"emvTags": {
"property1": "string",
"property2": "string"
},
"interacMac": "string",
"pinSessionKey": "string",
"processedAt": "2019-08-24T14:15:22Z",
"processor": "CHASE_PAYMENTECH",
"providerVerification": {
"publicKeyHash": "string",
"signature": "string"
},
"remainingBalance": 0,
"retrievalRefNum": "string",
"scaResult": "ENTER_PIN",
"status": "Successful",
"statusCode": "string",
"statusMessage": "string",
"transactionId": "string"
},
"reason": {
"program": "NO_SHOW",
"programFor": [
"RESTAURANT"
]
},
"sequence": 0,
"signature": [
-128
],
"signatureCaptured": true,
"systemTraceAuditNumber": "string",
"transactionNumber": "string"
}
],
"amounts": {
"cashbackAmount": 2147483647,
"currency": "string",
"customerOptedNoTip": true,
"orderAmount": 2147483647,
"tipAmount": 2147483647,
"transactionFees": [
{
"amount": 0,
"feeProgramType": "SURCHARGE",
"feeType": "FIXED",
"required": true,
"signature": "string",
"feeProgramId": "927253be-0294-4a36-80e7-7d0d8c175f0c"
}
],
"transactionAmount": 2147483647
},
"amountsAdjusted": true,
"approvalCode": "string",
"authOnly": true,
"chargebackStatus": "CREATED",
"context": {
"acquirerId": "string",
"businessId": "50092e54-cd12-4059-9828-e8c4ee9a3cf5",
"businessType": "MERCHANT",
"employeeUserId": 0,
"mcc": "string",
"mid": "string",
"source": "INSTORE",
"sourceApp": "string",
"storeAddressCity": "string",
"storeAddressTerritory": "string",
"storeDeviceId": "string",
"storeId": "b8adcdc8-9238-4168-90f8-77b2d14c211c",
"storeTimezone": "string",
"tid": "string",
"transactionInstruction": "NONE",
"transmissionAtLocal": "2019-08-24T14:15:22Z"
},
"createdAt": "2019-08-24T14:15:22Z",
"customerLanguage": "st",
"customerUserId": 0,
"emailReceipt": true,
"fundingSource": {
"accountType": "EBT",
"bankAccount": {
"accountNumber": "string",
"accountNumberLastFour": "string",
"accountType": "CHECKING",
"accountHolderType": "BUSINESS",
"bankName": "string",
"country": "string",
"currency": "string",
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"ownerName": "string",
"routingNumber": "string"
},
"card": {
"cardAgreement": {
"agreedOn": "2019-08-24T14:15:22Z",
"businessId": "50092e54-cd12-4059-9828-e8c4ee9a3cf5",
"cardId": "f16ba382-eb42-481a-b08f-c57bdc9aae24",
"createdAt": "2019-08-24T14:15:22Z",
"declinedOn": "2019-08-24T14:15:22Z",
"email": "string",
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"status": "string",
"updatedAt": "2019-08-24T14:15:22Z",
"version": 0
},
"cardBrand": {
"brand": "string",
"createdAt": "2019-08-24T14:15:22Z",
"displayName": "string",
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"issuerBank": "string",
"logoUrl": "string",
"scheme": "string"
},
"cardHolderFirstName": "string",
"cardHolderFullName": "string",
"cardHolderLastName": "string",
"cardId": "f16ba382-eb42-481a-b08f-c57bdc9aae24",
"currency": "str",
"encrypted": true,
"encryptedExpirationDate": "string",
"expirationDate": 0,
"expirationMonth": 0,
"expirationYear": 0,
"id": 0,
"issuer": "string",
"key": [
{
"id": "WCK",
"version": "stri"
}
],
"keySerialNumber": "string",
"number": "string",
"numberFirst6": "string",
"numberHashed": "string",
"numberLast4": "string",
"numberMasked": "string",
"sequenceNumber": "string",
"serviceCode": "string",
"source": "DIRECT",
"status": "ACTIVE",
"track1data": "string",
"track2data": "string",
"track3data": "string",
"type": "AMERICAN_EXPRESS"
},
"cardToken": "string",
"customFundingSource": {
"accountId": "string",
"description": "string",
"name": "string",
"processor": "string",
"provider": "string",
"type": "GIFT_CARD"
},
"debit": true,
"debitEBTReEntryDetails": {
"origApprovalCode": "string",
"origAuthSourceCode": "string",
"origCreatedAt": "2019-08-24T14:15:22Z",
"origNetworkId": "string",
"origResponseCode": "string",
"origRetrievalRefNumber": "string",
"origTraceNumber": "string",
"origTransactionId": "string",
"origTransactionNumber": "string"
},
"ebtDetails": {
"electronicVoucherApprovalCode": "string",
"electronicVoucherSerialNumber": "string",
"type": "CASH_BENEFIT"
},
"emvData": {
"emvTags": {
"property1": "string",
"property2": "string"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "KEYED",
"iccFallback": true
},
"exchangeRate": {
"businessId": "50092e54-cd12-4059-9828-e8c4ee9a3cf5",
"cardAmount": 0,
"cardCurrency": "str",
"cardTipAmount": 0,
"disclaimer": "string",
"markupInfo1": "string",
"markupInfo2": "string",
"markupPercentage": "string",
"provider": "string",
"rate": 0,
"ratePrecision": 0,
"requestedAt": "2019-08-24T14:15:22Z",
"signature": "string",
"tipAmount": 0,
"txnAmount": 0,
"txnCurrency": "str"
},
"interacMac": "string",
"interacMacKeySerialNumber": "string",
"nonce": "string",
"paymentToken": "string",
"tokenize": true,
"type": "CHEQUE",
"verificationData": {
"additionalIdRefNumber": "string",
"additionalIdType": "DRIVERS_LICENCE",
"cardHolderBillingAddress": {
"city": "string",
"countryCode": "str",
"createdAt": "2019-08-24T14:15:22Z",
"id": 0,
"line1": "string",
"line2": "string",
"postalCode": "string",
"postalCodeExtension": "string",
"primary": true,
"status": "ADDED",
"territory": "string",
"territoryType": "STATE",
"type": "HOME",
"updatedAt": "2019-08-24T14:15:22Z"
},
"cvData": "string",
"cvSkipReason": "NOT_PRESENT",
"keySerialNumber": "string",
"pin": "string",
"threeDSecureData": {
"cryptogram": "string",
"eci": "string"
}
}
},
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"links": [
{
"href": "string",
"method": "string",
"rel": "string"
}
],
"notes": "string",
"parentId": "70850378-7d3c-4f45-91b7-942d4dfbbd43",
"partialAuthEnabled": true,
"partiallyApproved": true,
"paymentTokenUsed": true,
"pinCaptured": true,
"poyntLoyalty": {
"externalId": "string",
"loyalty": [
{
"businessLoyaltyId": 0,
"campaignDescription": "string",
"campaignName": "string",
"lastIncrement": 0,
"loyaltyType": "string",
"loyaltyUnit": "string",
"nextTier": "string",
"points": 0,
"pointsRequired": 0,
"rewardDescription": "string",
"tier": "string",
"totalPoints": 0,
"totalSpend": 0,
"totalVisits": 0
}
],
"loyaltyId": 0,
"reward": [
{
"businessLoyaltyId": 0,
"expireAt": "2019-08-24T14:15:22Z",
"newReward": true,
"postText": "string",
"preText": "string",
"rewardDescription": "string",
"rewardId": 0,
"status": "string",
"type": "string",
"value": 0
}
]
},
"processorOptions": {
"property1": "string",
"property2": "string"
},
"processorResponse": {
"acquirer": "CHASE_PAYMENTECH",
"adapterId": "string",
"adapterVariant": "string",
"approvalCode": "string",
"approvedAmount": 0,
"avsResult": {
"actualResult": "string",
"addressResult": "MATCH",
"cardHolderNameResult": "MATCH",
"cityResult": "MATCH",
"countryResult": "MATCH",
"phoneResult": "MATCH",
"postalCodeResult": "MATCH",
"stateResult": "MATCH"
},
"batchAutoClosedByHost": "string",
"batchId": "string",
"cardToken": "string",
"cvActualResult": "string",
"cvResult": "MATCH",
"debitResponseCode": "string",
"echeckProcessor": "MOCK",
"emvTags": {
"property1": "string",
"property2": "string"
},
"interacMac": "string",
"pinSessionKey": "string",
"processedAt": "2019-08-24T14:15:22Z",
"processor": "CHASE_PAYMENTECH",
"providerVerification": {
"publicKeyHash": "string",
"signature": "string"
},
"remainingBalance": 0,
"retrievalRefNum": "string",
"scaResult": "ENTER_PIN",
"status": "Successful",
"statusCode": "string",
"statusMessage": "string",
"transactionId": "string"
},
"reason": {
"program": "NO_SHOW",
"programFor": [
"RESTAURANT"
]
},
"receiptEmailAddress": "string",
"receiptPhone": {
"areaCode": "string",
"createdAt": "2019-08-24T14:15:22Z",
"extensionNumber": "string",
"id": 0,
"ituCountryCode": "string",
"localPhoneNumber": "string",
"primaryDayTime": true,
"primaryEvening": true,
"status": "ADDED",
"type": "HOME",
"updatedAt": "2019-08-24T14:15:22Z"
},
"references": [
{
"customType": "string",
"id": "string",
"type": "POYNT_ORDER"
}
],
"reversalVoid": true,
"settled": true,
"shippingAddress": {
"city": "string",
"countryCode": "str",
"createdAt": "2019-08-24T14:15:22Z",
"id": 0,
"line1": "string",
"line2": "string",
"postalCode": "string",
"postalCodeExtension": "string",
"primary": true,
"status": "ADDED",
"territory": "string",
"territoryType": "STATE",
"type": "HOME",
"updatedAt": "2019-08-24T14:15:22Z"
},
"signature": [
-128
],
"signatureCaptured": true,
"signatureRequired": true,
"status": "CREATED",
"stayType": "GENERAL_CONTAINER",
"systemTraceAuditNumber": "string",
"transactionNumber": "string",
"updatedAt": "2019-08-24T14:15:22Z",
"voided": true
}
Sample Response
{
"_id": 0,
"_parentId": 0,
"_tcdId": 0,
"action": "AUTHORIZE",
"actionVoid": true,
"adjusted": true,
"adjustmentHistory": [
{
"amountChanges": {
"cashbackAmount": 2147483647,
"currency": "string",
"customerOptedNoTip": true,
"orderAmount": 2147483647,
"tipAmount": 2147483647,
"transactionFees": [
{
"amount": 0,
"feeProgramType": "SURCHARGE",
"feeType": "FIXED",
"required": true,
"signature": "string",
"feeProgramId": "927253be-0294-4a36-80e7-7d0d8c175f0c"
}
],
"transactionAmount": 2147483647
},
"amounts": {
"cashbackAmount": 2147483647,
"currency": "string",
"customerOptedNoTip": true,
"orderAmount": 2147483647,
"tipAmount": 2147483647,
"transactionFees": [
{
"amount": 0,
"feeProgramType": "SURCHARGE",
"feeType": "FIXED",
"required": true,
"signature": "string",
"feeProgramId": "927253be-0294-4a36-80e7-7d0d8c175f0c"
}
],
"transactionAmount": 2147483647
},
"createdAt": "2019-08-24T14:15:22Z",
"exchangeRate": {
"businessId": "50092e54-cd12-4059-9828-e8c4ee9a3cf5",
"cardAmount": 0,
"cardCurrency": "str",
"cardTipAmount": 0,
"disclaimer": "string",
"markupInfo1": "string",
"markupInfo2": "string",
"markupPercentage": "string",
"provider": "string",
"rate": 0,
"ratePrecision": 0,
"requestedAt": "2019-08-24T14:15:22Z",
"signature": "string",
"tipAmount": 0,
"txnAmount": 0,
"txnCurrency": "str"
},
"processorResponse": {
"acquirer": "CHASE_PAYMENTECH",
"adapterId": "string",
"adapterVariant": "string",
"approvalCode": "string",
"approvedAmount": 0,
"avsResult": {
"actualResult": "string",
"addressResult": "MATCH",
"cardHolderNameResult": "MATCH",
"cityResult": "MATCH",
"countryResult": "MATCH",
"phoneResult": "MATCH",
"postalCodeResult": "MATCH",
"stateResult": "MATCH"
},
"batchAutoClosedByHost": "string",
"batchId": "string",
"cardToken": "string",
"cvActualResult": "string",
"cvResult": "MATCH",
"debitResponseCode": "string",
"echeckProcessor": "MOCK",
"emvTags": {
"property1": "string",
"property2": "string"
},
"interacMac": "string",
"pinSessionKey": "string",
"processedAt": "2019-08-24T14:15:22Z",
"processor": "CHASE_PAYMENTECH",
"providerVerification": {
"publicKeyHash": "string",
"signature": "string"
},
"remainingBalance": 0,
"retrievalRefNum": "string",
"scaResult": "ENTER_PIN",
"status": "Successful",
"statusCode": "string",
"statusMessage": "string",
"transactionId": "string"
},
"reason": {
"program": "NO_SHOW",
"programFor": [
"RESTAURANT"
]
},
"sequence": 0,
"signature": [
-128
],
"signatureCaptured": true,
"systemTraceAuditNumber": "string",
"transactionNumber": "string"
}
],
"amounts": {
"cashbackAmount": 2147483647,
"currency": "string",
"customerOptedNoTip": true,
"orderAmount": 2147483647,
"tipAmount": 2147483647,
"transactionFees": [
{
"amount": 0,
"feeProgramType": "SURCHARGE",
"feeType": "FIXED",
"required": true,
"signature": "string",
"feeProgramId": "927253be-0294-4a36-80e7-7d0d8c175f0c"
}
],
"transactionAmount": 2147483647
},
"amountsAdjusted": true,
"approvalCode": "string",
"authOnly": true,
"chargebackStatus": "CREATED",
"context": {
"acquirerId": "string",
"businessId": "50092e54-cd12-4059-9828-e8c4ee9a3cf5",
"businessType": "MERCHANT",
"employeeUserId": 0,
"mcc": "string",
"mid": "string",
"source": "INSTORE",
"sourceApp": "string",
"storeAddressCity": "string",
"storeAddressTerritory": "string",
"storeDeviceId": "string",
"storeId": "b8adcdc8-9238-4168-90f8-77b2d14c211c",
"storeTimezone": "string",
"tid": "string",
"transactionInstruction": "NONE",
"transmissionAtLocal": "2019-08-24T14:15:22Z"
},
"createdAt": "2019-08-24T14:15:22Z",
"customerLanguage": "st",
"customerUserId": 0,
"emailReceipt": true,
"fundingSource": {
"accountType": "EBT",
"bankAccount": {
"accountNumber": "string",
"accountNumberLastFour": "string",
"accountType": "CHECKING",
"accountHolderType": "BUSINESS",
"bankName": "string",
"country": "string",
"currency": "string",
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"ownerName": "string",
"routingNumber": "string"
},
"card": {
"cardAgreement": {
"agreedOn": "2019-08-24T14:15:22Z",
"businessId": "50092e54-cd12-4059-9828-e8c4ee9a3cf5",
"cardId": "f16ba382-eb42-481a-b08f-c57bdc9aae24",
"createdAt": "2019-08-24T14:15:22Z",
"declinedOn": "2019-08-24T14:15:22Z",
"email": "string",
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"status": "string",
"updatedAt": "2019-08-24T14:15:22Z",
"version": 0
},
"cardBrand": {
"brand": "string",
"createdAt": "2019-08-24T14:15:22Z",
"displayName": "string",
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"issuerBank": "string",
"logoUrl": "string",
"scheme": "string"
},
"cardHolderFirstName": "string",
"cardHolderFullName": "string",
"cardHolderLastName": "string",
"cardId": "f16ba382-eb42-481a-b08f-c57bdc9aae24",
"currency": "str",
"encrypted": true,
"encryptedExpirationDate": "string",
"expirationDate": 0,
"expirationMonth": 0,
"expirationYear": 0,
"id": 0,
"issuer": "string",
"key": [
{
"id": "WCK",
"version": "stri"
}
],
"keySerialNumber": "string",
"number": "string",
"numberFirst6": "string",
"numberHashed": "string",
"numberLast4": "string",
"numberMasked": "string",
"sequenceNumber": "string",
"serviceCode": "string",
"source": "DIRECT",
"status": "ACTIVE",
"track1data": "string",
"track2data": "string",
"track3data": "string",
"type": "AMERICAN_EXPRESS"
},
"cardToken": "string",
"customFundingSource": {
"accountId": "string",
"description": "string",
"name": "string",
"processor": "string",
"provider": "string",
"type": "GIFT_CARD"
},
"debit": true,
"debitEBTReEntryDetails": {
"origApprovalCode": "string",
"origAuthSourceCode": "string",
"origCreatedAt": "2019-08-24T14:15:22Z",
"origNetworkId": "string",
"origResponseCode": "string",
"origRetrievalRefNumber": "string",
"origTraceNumber": "string",
"origTransactionId": "string",
"origTransactionNumber": "string"
},
"ebtDetails": {
"electronicVoucherApprovalCode": "string",
"electronicVoucherSerialNumber": "string",
"type": "CASH_BENEFIT"
},
"emvData": {
"emvTags": {
"property1": "string",
"property2": "string"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "KEYED",
"iccFallback": true
},
"exchangeRate": {
"businessId": "50092e54-cd12-4059-9828-e8c4ee9a3cf5",
"cardAmount": 0,
"cardCurrency": "str",
"cardTipAmount": 0,
"disclaimer": "string",
"markupInfo1": "string",
"markupInfo2": "string",
"markupPercentage": "string",
"provider": "string",
"rate": 0,
"ratePrecision": 0,
"requestedAt": "2019-08-24T14:15:22Z",
"signature": "string",
"tipAmount": 0,
"txnAmount": 0,
"txnCurrency": "str"
},
"interacMac": "string",
"interacMacKeySerialNumber": "string",
"nonce": "string",
"paymentToken": "string",
"tokenize": true,
"type": "CHEQUE",
"verificationData": {
"additionalIdRefNumber": "string",
"additionalIdType": "DRIVERS_LICENCE",
"cardHolderBillingAddress": {
"city": "string",
"countryCode": "str",
"createdAt": "2019-08-24T14:15:22Z",
"id": 0,
"line1": "string",
"line2": "string",
"postalCode": "string",
"postalCodeExtension": "string",
"primary": true,
"status": "ADDED",
"territory": "string",
"territoryType": "STATE",
"type": "HOME",
"updatedAt": "2019-08-24T14:15:22Z"
},
"cvData": "string",
"cvSkipReason": "NOT_PRESENT",
"keySerialNumber": "string",
"pin": "string",
"threeDSecureData": {
"cryptogram": "string",
"eci": "string"
}
}
},
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"links": [
{
"href": "string",
"method": "string",
"rel": "string"
}
],
"notes": "string",
"parentId": "70850378-7d3c-4f45-91b7-942d4dfbbd43",
"partialAuthEnabled": true,
"partiallyApproved": true,
"paymentTokenUsed": true,
"pinCaptured": true,
"poyntLoyalty": {
"externalId": "string",
"loyalty": [
{
"businessLoyaltyId": 0,
"campaignDescription": "string",
"campaignName": "string",
"lastIncrement": 0,
"loyaltyType": "string",
"loyaltyUnit": "string",
"nextTier": "string",
"points": 0,
"pointsRequired": 0,
"rewardDescription": "string",
"tier": "string",
"totalPoints": 0,
"totalSpend": 0,
"totalVisits": 0
}
],
"loyaltyId": 0,
"reward": [
{
"businessLoyaltyId": 0,
"expireAt": "2019-08-24T14:15:22Z",
"newReward": true,
"postText": "string",
"preText": "string",
"rewardDescription": "string",
"rewardId": 0,
"status": "string",
"type": "string",
"value": 0
}
]
},
"processorOptions": {
"property1": "string",
"property2": "string"
},
"processorResponse": {
"acquirer": "CHASE_PAYMENTECH",
"adapterId": "string",
"adapterVariant": "string",
"approvalCode": "string",
"approvedAmount": 0,
"avsResult": {
"actualResult": "string",
"addressResult": "MATCH",
"cardHolderNameResult": "MATCH",
"cityResult": "MATCH",
"countryResult": "MATCH",
"phoneResult": "MATCH",
"postalCodeResult": "MATCH",
"stateResult": "MATCH"
},
"batchAutoClosedByHost": "string",
"batchId": "string",
"cardToken": "string",
"cvActualResult": "string",
"cvResult": "MATCH",
"debitResponseCode": "string",
"echeckProcessor": "MOCK",
"emvTags": {
"property1": "string",
"property2": "string"
},
"interacMac": "string",
"pinSessionKey": "string",
"processedAt": "2019-08-24T14:15:22Z",
"processor": "CHASE_PAYMENTECH",
"providerVerification": {
"publicKeyHash": "string",
"signature": "string"
},
"remainingBalance": 0,
"retrievalRefNum": "string",
"scaResult": "ENTER_PIN",
"status": "Successful",
"statusCode": "string",
"statusMessage": "string",
"transactionId": "string"
},
"reason": {
"program": "NO_SHOW",
"programFor": [
"RESTAURANT"
]
},
"receiptEmailAddress": "string",
"receiptPhone": {
"areaCode": "string",
"createdAt": "2019-08-24T14:15:22Z",
"extensionNumber": "string",
"id": 0,
"ituCountryCode": "string",
"localPhoneNumber": "string",
"primaryDayTime": true,
"primaryEvening": true,
"status": "ADDED",
"type": "HOME",
"updatedAt": "2019-08-24T14:15:22Z"
},
"references": [
{
"customType": "string",
"id": "string",
"type": "POYNT_ORDER"
}
],
"reversalVoid": true,
"settled": true,
"shippingAddress": {
"city": "string",
"countryCode": "str",
"createdAt": "2019-08-24T14:15:22Z",
"id": 0,
"line1": "string",
"line2": "string",
"postalCode": "string",
"postalCodeExtension": "string",
"primary": true,
"status": "ADDED",
"territory": "string",
"territoryType": "STATE",
"type": "HOME",
"updatedAt": "2019-08-24T14:15:22Z"
},
"signature": [
-128
],
"signatureCaptured": true,
"signatureRequired": true,
"status": "CREATED",
"stayType": "GENERAL_CONTAINER",
"systemTraceAuditNumber": "string",
"transactionNumber": "string",
"updatedAt": "2019-08-24T14:15:22Z",
"voided": true
}
Create transaction
Create a new authorize, sale or refund transaction. If process query parameter is set to true or not provided, the processor will be called to actually process the transaction. If the process query parameter is set to false, the transaction will just be saved, but the processor will not be called for processing. This operation returns the Transaction just created. If the transaction is declined before the processor is called, the client will get an error. If the transaction is declined by the processor, the Transaction object is returned with status set to DECLINED and processor response object containing the response from the processor.
Referenced Refunds are only supported for a limited time, up to 180 days from the original authorization. To execute a refund beyond the referenced refund duration limit, please use an Unreferenced Refund.
Arguments
Poynt-Request-Id
header- string (required)
process
query- boolean (optional)
businessId
path- string (required)
transaction
body- Transaction (optional)
Response
Returns a CompletableFuture«Transaction».
Definition
GET /businesses/{businessId}/transactions/archive/{queryId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Get archive query status
Get query status and return resource URL for fetching archived transaction (.csv format)
Arguments
businessId
path- string (required)
queryId
path- string (required)
Response
Returns a QueryStatus.
Definition
POST /businesses/{businessId}/transactions/cancel
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
: Add definition TODO
Sample Request
: Add request TODO
Sample Response
: Add response TODO
Definition
TODO: Add definition
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Cancel transaction
Cancel and void are similar. They can both undo auth, sale, capture or refund transactions (if the acquirer supports it). A Cancel operation carries a CancelReason request parameter where the caller can specify the exact reason for the undo. Also note that if the Cancel operation is unable to perform an undo, and the original transaction is a sale or capture, it will proceed to issue a refund.
Arguments
Poynt-Request-Id
header- string (required)
businessId
path- string (required)
original-request-id
query- string (optional)
transaction-id
query- string (optional)
cancelRequest
body- CancelRequest (optional)
Response
Returns a ResponseEntity«Transaction».
Definition
GET /businesses/{businessId}/transactions/{transactionId}
Sample Request
curl --location --request GET 'https://services.poynt.net/businesses/a3f24e9e-6764-42f2-bbcd-5541e7f3be38/transactions/3a8acc83-0186-1000-8ba1-0c7ecdce3d15' \
--header 'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json'
Sample Response
{
"signatureRequired": false,
"signatureCaptured": false,
"pinCaptured": false,
"adjusted": false,
"amountsAdjusted": false,
"authOnly": false,
"partiallyApproved": false,
"actionVoid": false,
"voided": false,
"settled": false,
"reversalVoid": false,
"createdAt": "2023-02-10T08:57:03Z",
"updatedAt": "2023-02-10T08:57:03Z",
"context": {
"businessType": "TEST_MERCHANT",
"transmissionAtLocal": "2023-02-10T08:57:00Z",
"employeeUserId": 369299726,
"storeDeviceId": "urn:tid:5312e878-e1a2-3676-bd78-d04640310b02",
"sourceApp": "co.poynt.register",
"mcc": "5812",
"transactionInstruction": "ONLINE_AUTH_REQUIRED",
"source": "INSTORE",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38",
"storeId": "89d82d45-5a56-4446-a04e-4340d417b841",
"channelId": "89d82d45-5a56-4446-a04e-4340d417b841"
},
"fundingSource": {
"debit": false,
"card": {
"cardBrand": {
"createdAt": "2020-05-27T16:14:32Z",
"scheme": "VISA",
"displayName": "Visa",
"id": "c74ab71f-9024-4de0-910d-54c6a9c2bbc5"
},
"type": "VISA",
"source": "DIRECT",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 12,
"expirationYear": 2024,
"id": 442586255,
"numberFirst6": "441779",
"numberLast4": "1678",
"numberMasked": "441779******1678",
"cardId": "377cad8d-96f7-4d0e-a515-55a76e3f591b"
},
"emvData": {
"emvTags": {
"0x9F36": "000D",
"0x5F34": "00",
"0x9F37": "7E78C8BF",
"0x9F35": "22",
"0x9F10": "1F420132A0000000001003027300000000400000000000000000000000000000",
"0x9F33": "E0F0C8",
"0x4F": "A0000000031010",
"0x84": "A0000000031010",
"0x5F2A": "0840",
"0x82": "0040",
"0x9F6C": "0080",
"0x1F8231": "16",
"0x9F27": "80",
"0x9F06": "A0000000031010",
"0x9C": "00",
"0x9F03": "000000000000",
"0x9B": "0000",
"0x5F24": "241231",
"0x9F26": "6CB4DAFDF50EB4FC",
"0x9A": "230210",
"0x9F01": "000000000000",
"0x9F02": "000000004500",
"0x9F24": "5630303130303135383230303331323632363337333232313336373630",
"0x9F66": "36C04000",
"0x95": "0000000000",
"0x9F1A": "0840",
"0x8A": "3030"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_INTEGRATED_CIRCUIT_CARD"
},
"type": "CREDIT_DEBIT"
},
"references": [
{
"id": "3a8a7727-0186-1000-7fed-1c1d6817967a",
"type": "POYNT_ORDER"
}
],
"customerUserId": 442485772,
"processorOptions": {
"scaIndicator": "supported"
},
"processorResponse": {
"approvedAmount": 4500,
"emvTags": {
"0x8A": "3030",
"0x89": "454139324645"
},
"processor": "MOCK",
"acquirer": "ELAVON",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "3a8acc83-0186-1000-8ba1-0c7ecdce3d15",
"approvalCode": "419763",
"batchId": "1",
"retrievalRefNum": "3a8acc83-0186-1000-8ba1-0c7ecdce3d15"
},
"customerLanguage": "en",
"settlementStatus": "UNSETTLED",
"action": "SALE",
"amounts": {
"customerOptedNoTip": false,
"transactionAmount": 4500,
"orderAmount": 4500,
"tipAmount": 0,
"cashbackAmount": 0,
"currency": "USD"
},
"status": "CAPTURED",
"id": "3a8acc83-0186-1000-8ba1-0c7ecdce3d15"
}
Definition
.exports.getTransaction = function getTransaction(options, next) { ... }
module/**
* Get a single transaction at a business.
* @param {String} options.businessId
* @param {String} options.transactionId
* @return {Transaction} transaction
*/
Sample Request
.getTransaction({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
transactionId : '9ee28946-cab1-48af-b30b-d111716128a2'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"action": "AUTHORIZE",
"actionVoid": false,
"adjusted": false,
"amounts": {
"cashbackAmount": 0,
"currency": "USD",
"customerOptedNoTip": false,
"orderAmount": 800,
"tipAmount": 0,
"transactionAmount": 800
,
}"amountsAdjusted": false,
"authOnly": false,
"context": {
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"businessType": "TEST_MERCHANT",
"employeeUserId": 5247838,
"mcc": "5812",
"source": "INSTORE",
"sourceApp": "co.poynt.services",
"storeDeviceId": "urn:tid:48c54303-6d51-39af-bdeb-4af53f621652",
"storeId": "c394627f-4f68-47fb-90a5-684ea801a352",
"transmissionAtLocal": "2017-01-04T22:20:40Z"
,
}"createdAt": "2017-01-04T22:20:40Z",
"customerUserId": 5247839,
"fundingSource": {
"card": {
"cardHolderFirstName": "",
"cardHolderFullName": "/",
"cardHolderLastName": "",
"expirationDate": 31,
"expirationMonth": 8,
"expirationYear": 2022,
"id": 243043,
"numberFirst6": "405413",
"numberLast4": "0833",
"status": "ACTIVE",
"type": "VISA"
,
}"debit": false,
"emvData": {
"emvTags": {
"0x5F20": "202F",
"0x5F24": "220831",
"0x9C": "00",
"0x9F02": "000000000800",
"0x9F06": "A0000000031010",
"0x9F37": "7619D4A6"
},
}"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "CONTACTLESS_MAGSTRIPE"
,
}"type": "CREDIT_DEBIT"
,
}"id": "9ee28946-cab1-48af-b30b-d111716128a2",
"partiallyApproved": false,
"pinCaptured": false,
"processorResponse": {
"acquirer": "CHASE_PAYMENTECH",
"approvalCode": "667149",
"approvedAmount": 800,
"batchId": "1",
"processor": "MOCK",
"retrievalRefNum": "9ee28946-cab1-48af-b30b-d111716128a2",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "9ee28946-cab1-48af-b30b-d111716128a2"
,
}"references": [
{"customType": "referenceId",
"id": "6b91d9a0-0159-1000-6f82-44ed3d4b4a5a",
"type": "CUSTOM"
},
]"settled": false,
"signatureCaptured": true,
"signatureRequired": false,
"status": "AUTHORIZED",
"updatedAt": "2017-01-04T22:20:40Z",
"voided": false
}
Definition
@classmethod
def get_transaction(cls, business_id, transaction_id):
"""
Get a single transaction at a business.
Arguments:
business_id (str): the business ID
transaction_id (str): the transaction ID
"""
Sample Request
= poynt.Transaction.get_transaction(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'9ee28946-cab1-48af-b30b-d111716128a2'
)
if status_code < 300:
print(doc)
Sample Response
u'authOnly': False, u'status': u'AUTHORIZED', u'adjusted': False, u'context': {u'businessType': u'TEST_MERCHANT', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'storeId': u'c394627f-4f68-47fb-90a5-684ea801a352', u'mcc': u'5812', u'sourceApp': u'co.poynt.services', u'source': u'INSTORE', u'employeeUserId': 5247838, u'transmissionAtLocal': u'2017-01-04T22:20:40Z', u'storeDeviceId': u'urn:tid:48c54303-6d51-39af-bdeb-4af53f621652'}, u'pinCaptured': False, u'id': u'9ee28946-cab1-48af-b30b-d111716128a2', u'action': u'AUTHORIZE', u'customerUserId': 5247839, u'amountsAdjusted': False, u'partiallyApproved': False, u'voided': False, u'signatureRequired': False, u'fundingSource': {u'entryDetails': {u'customerPresenceStatus': u'PRESENT', u'entryMode': u'CONTACTLESS_MAGSTRIPE'}, u'emvData': {u'emvTags': {u'0x5F24': u'220831', u'0x9C': u'00', u'0x5F20': u'202F', u'0x9F02': u'000000000800', u'0x9F06': u'A0000000031010', u'0x9F37': u'7619D4A6'}}, u'type': u'CREDIT_DEBIT', u'card': {u'status': u'ACTIVE', u'expirationMonth': 8, u'cardHolderFullName': u'/', u'cardHolderLastName': u'', u'cardHolderFirstName': u'', u'expirationYear': 2022, u'expirationDate': 31, u'numberLast4': u'0833', u'type': u'VISA', u'id': 243043, u'numberFirst6': u'405413'}, u'debit': False}, u'amounts': {u'tipAmount': 0, u'cashbackAmount': 0, u'currency': u'USD', u'customerOptedNoTip': False, u'orderAmount': 800, u'transactionAmount': 800}, u'references': [{u'customType': u'referenceId', u'type': u'CUSTOM', u'id': u'6b91d9a0-0159-1000-6f82-44ed3d4b4a5a'}], u'actionVoid': False, u'updatedAt': u'2017-01-04T22:20:40Z', u'processorResponse': {u'status': u'Successful', u'approvalCode': u'667149', u'retrievalRefNum': u'9ee28946-cab1-48af-b30b-d111716128a2', u'batchId': u'1', u'acquirer': u'CHASE_PAYMENTECH', u'approvedAmount': 800, u'transactionId': u'9ee28946-cab1-48af-b30b-d111716128a2', u'processor': u'MOCK', u'statusMessage': u'Successful', u'statusCode': u'1'}, u'settled': False, u'signatureCaptured': True, u'createdAt': u'2017-01-04T22:20:40Z'} {
Get a transaction
Get a transaction (along with HATEOS links to all immediately related transactions).
Arguments
If-Modified-Since
header- string (optional)
businessId
path- string (required)
transactionId
path- string (required)
Response
Returns a Transaction.
Definition
PATCH /businesses/{businessId}/transactions/{transactionId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
This method is currently not possible using the Node.js SDK.
Definition
This method is currently not possible using the Python SDK.
Update transaction
Update a transaction. This only works on an authorization transaction. This takes a JsonPatch object as request. We currently support replacing all the fields in the /amounts object and adding on /receiptEmailAddress and /signature.
Arguments
Poynt-Request-Id
header- string (required)
businessId
path- string (required)
transactionId
path- string (required)
patch
body- JsonPatch (optional)
Response
Returns a Transaction.
Definition
PUT /businesses/{businessId}/transactions/{transactionId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
This method is currently not possible using the Node.js SDK.
Definition
This method is currently not possible using the Python SDK.
Record transaction
Record a transaction that has already happened. A full transaction object must be populated as this operation will simply record what is passed to it. If the references field in the body includes a POYNT_ORDER, this transaction will be attached to it.
Arguments
Poynt-Request-Id
header- string (required)
businessId
path- string (required)
transactionId
path- string (required)
transaction
body- Transaction (optional)
Response
Returns a Transaction.
Definition
POST /businesses/{businessId}/transactions/{transactionId}/capture
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
: Add definition TODO
Sample Request
: Add request TODO
Sample Response
: Add response TODO
Definition
TODO: Add definition
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Capture transaction
Capture a previously authorized transaction. Multiple captures are allowed on a single authorization. This return the original authorization transaction with its status updated to CAPTURED if the capture operation succeeded and set to AUTHORIZED if the capture operation failed. The authorization transaction returned will also have a HATEOS link to the capture. In order to get capture specific details (e.g. the processor response), the client will have to perform a GET on the capture transaction from the HATEOS link.
Arguments
Poynt-Request-Id
header- string (required)
businessId
path- string (required)
transactionId
path- string (required)
capture
body- AdjustTransactionRequest (optional)
allowDuplicates
query- boolean (optional)
Response
Returns a CompletableFuture«Transaction».
Definition
POST /businesses/{businessId}/transactions/{transactionId}/void
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
: Add definition TODO
Sample Request
: Add request TODO
Sample Response
: Add response TODO
Definition
TODO: Add definition
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Void transaction
Void and Cancel are similar. They can both undo auth, sale, capture or refund transactions (if the acquirer supports it). A Void is always for the full amount. Hence there can only be one void for a transaction and no amount needs to be specified in the void request. This will result in the original transaction being marked as voided. In order to get to the specific void details (e.g. the specific response received from the processor for the void request), the client will have to follow the HATEOS links. Also note that for a Void operation is always assumed to have been MERCHANT_INITIATED.
Arguments
Poynt-Request-Id
header- string (required)
businessId
path- string (required)
transactionId
path- string (required)
voidRequest
body- VoidRequest (optional)
Response
Returns a Transaction.
Definition
GET /businesses/{businessId}/transactions/{transactionId}/receipt
Sample Request
TODO: Add request
Sample Response
TODO: Add response
renderReceipt
renderReceipt
Arguments
businessId
path- string (required)
transactionId
path- string (required)
receiptTemplateId
query- string (optional, default is default_txn_receipt_template_id)
Response
Returns a TransactionReceipt.
Surcharge
The surcharge API provides an ability to onboard merchants into the surcharging fee program, which allows them to apply additional charges to credit card transactions to offset the cost of credit card payment proccessing.
Definition
POST /businesses/{businessId}/transactions/eligible-fees
Sample Request
{
"_id": 0,
"_parentId": 0,
"_tcdId": 0,
"action": "AUTHORIZE",
"actionVoid": true,
"adjusted": true,
"adjustmentHistory": [
{
"amountChanges": {
"cashbackAmount": 2147483647,
"currency": "string",
"customerOptedNoTip": true,
"orderAmount": 2147483647,
"tipAmount": 2147483647,
"transactionFees": [
{
"amount": 0,
"feeProgramType": "SURCHARGE",
"feeType": "FIXED",
"required": true,
"signature": "string",
"feeProgramId": "927253be-0294-4a36-80e7-7d0d8c175f0c"
}
],
"transactionAmount": 2147483647
},
"amounts":{
"cashbackAmount": 2147483647,
"currency": "string",
"customerOptedNoTip": true,
"orderAmount": 2147483647,
"tipAmount": 2147483647,
"transactionFees": [
{
"amount": 0,
"feeProgramType": "SURCHARGE",
"feeType": "FIXED",
"required": true,
"signature": "string",
"feeProgramId": "927253be-0294-4a36-80e7-7d0d8c175f0c"
}
],
"transactionAmount": 2147483647
},
"createdAt": "2019-08-24T14:15:22Z",
"exchangeRate": {
"businessId": "50092e54-cd12-4059-9828-e8c4ee9a3cf5",
"cardAmount": 0,
"cardCurrency": "str",
"cardTipAmount": 0,
"disclaimer": "string",
"markupInfo1": "string",
"markupInfo2": "string",
"markupPercentage": "string",
"provider": "string",
"rate": 0,
"ratePrecision": 0,
"requestedAt": "2019-08-24T14:15:22Z",
"signature": "string",
"tipAmount": 0,
"txnAmount": 0,
"txnCurrency": "str"
},
"processorResponse": {
"acquirer": "CHASE_PAYMENTECH",
"adapterId": "string",
"adapterVariant": "string",
"approvalCode": "string",
"approvedAmount": 0,
"avsResult": {
"actualResult": "string",
"addressResult": "MATCH",
"cardHolderNameResult": "MATCH",
"cityResult": "MATCH",
"countryResult": "MATCH",
"phoneResult": "MATCH",
"postalCodeResult": "MATCH",
"stateResult": "MATCH"
},
"batchAutoClosedByHost": "string",
"batchId": "string",
"cardToken": "string",
"cvActualResult": "string",
"cvResult": "MATCH",
"debitResponseCode": "string",
"echeckProcessor": "MOCK",
"emvTags": {
"property1": "string",
"property2": "string"
},
"interacMac": "string",
"pinSessionKey": "string",
"processedAt": "2019-08-24T14:15:22Z",
"processor": "CHASE_PAYMENTECH",
"providerVerification": {
"publicKeyHash": "string",
"signature": "string"
},
"remainingBalance": 0,
"retrievalRefNum": "string",
"scaResult": "ENTER_PIN",
"status": "Successful",
"statusCode": "string",
"statusMessage": "string",
"transactionId": "string"
},
"reason": {
"program": "NO_SHOW",
"programFor": [
"RESTAURANT"
]
},
"sequence": 0,
"signature": [
-128
],
"signatureCaptured": true,
"systemTraceAuditNumber": "string",
"transactionNumber": "string"
}
],
"amounts": {
"cashbackAmount": 2147483647,
"currency": "string",
"customerOptedNoTip": true,
"orderAmount": 2147483647,
"tipAmount": 2147483647,
"transactionFees": [
{
"amount": 0,
"feeProgramType": "SURCHARGE",
"feeType": "FIXED",
"required": true,
"signature": "string",
"feeProgramId": "927253be-0294-4a36-80e7-7d0d8c175f0c"
}
],
"transactionAmount": 2147483647
},
"amountsAdjusted": true,
"approvalCode": "string",
"authOnly": true,
"chargebackStatus": "CREATED",
"context": {
"acquirerId": "string",
"businessId": "50092e54-cd12-4059-9828-e8c4ee9a3cf5",
"businessType": "MERCHANT",
"employeeUserId": 0,
"mcc": "string",
"mid": "string",
"source": "INSTORE",
"sourceApp": "string",
"storeAddressCity": "string",
"storeAddressTerritory": "string",
"storeDeviceId": "string",
"storeId": "b8adcdc8-9238-4168-90f8-77b2d14c211c",
"storeTimezone": "string",
"tid": "string",
"transactionInstruction": "NONE",
"transmissionAtLocal": "2019-08-24T14:15:22Z"
},
"createdAt": "2019-08-24T14:15:22Z",
"customerLanguage": "st",
"customerUserId": 0,
"emailReceipt": true,
"fundingSource": {
"accountType": "EBT",
"bankAccount": {
"accountNumber": "string",
"accountNumberLastFour": "string",
"accountType": "CHECKING",
"accountHolderType": "BUSINESS",
"bankName": "string",
"country": "string",
"currency": "string",
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"ownerName": "string",
"routingNumber": "string"
},
"card": {
"cardAgreement": {
"agreedOn": "2019-08-24T14:15:22Z",
"businessId": "50092e54-cd12-4059-9828-e8c4ee9a3cf5",
"cardId": "f16ba382-eb42-481a-b08f-c57bdc9aae24",
"createdAt": "2019-08-24T14:15:22Z",
"declinedOn": "2019-08-24T14:15:22Z",
"email": "string",
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"status": "string",
"updatedAt": "2019-08-24T14:15:22Z",
"version": 0
},
"cardBrand": {
"brand": "string",
"createdAt": "2019-08-24T14:15:22Z",
"displayName": "string",
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"issuerBank": "string",
"logoUrl": "string",
"scheme": "string"
},
"cardHolderFirstName": "string",
"cardHolderFullName": "string",
"cardHolderLastName": "string",
"cardId": "f16ba382-eb42-481a-b08f-c57bdc9aae24",
"currency": "str",
"encrypted": true,
"encryptedExpirationDate": "string",
"expirationDate": 0,
"expirationMonth": 0,
"expirationYear": 0,
"id": 0,
"issuer": "string",
"key": [
{
"id": "WCK",
"version": "stri"
}
],
"keySerialNumber": "string",
"number": "string",
"numberFirst6": "string",
"numberHashed": "string",
"numberLast4": "string",
"numberMasked": "string",
"sequenceNumber": "string",
"serviceCode": "string",
"source": "DIRECT",
"status": "ACTIVE",
"track1data": "string",
"track2data": "string",
"track3data": "string",
"type": "AMERICAN_EXPRESS"
},
"cardToken": "string",
"customFundingSource": {
"accountId": "string",
"description": "string",
"name": "string",
"processor": "string",
"provider": "string",
"type": "GIFT_CARD"
},
"debit": true,
"debitEBTReEntryDetails": {
"origApprovalCode": "string",
"origAuthSourceCode": "string",
"origCreatedAt": "2019-08-24T14:15:22Z",
"origNetworkId": "string",
"origResponseCode": "string",
"origRetrievalRefNumber": "string",
"origTraceNumber": "string",
"origTransactionId": "string",
"origTransactionNumber": "string"
},
"ebtDetails": {
"electronicVoucherApprovalCode": "string",
"electronicVoucherSerialNumber": "string",
"type": "CASH_BENEFIT"
},
"emvData": {
"emvTags": {
"property1": "string",
"property2": "string"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "KEYED",
"iccFallback": true
},
"exchangeRate": {
"businessId": "50092e54-cd12-4059-9828-e8c4ee9a3cf5",
"cardAmount": 0,
"cardCurrency": "str",
"cardTipAmount": 0,
"disclaimer": "string",
"markupInfo1": "string",
"markupInfo2": "string",
"markupPercentage": "string",
"provider": "string",
"rate": 0,
"ratePrecision": 0,
"requestedAt": "2019-08-24T14:15:22Z",
"signature": "string",
"tipAmount": 0,
"txnAmount": 0,
"txnCurrency": "str"
},
"interacMac": "string",
"interacMacKeySerialNumber": "string",
"nonce": "string",
"paymentToken": "string",
"tokenize": true,
"type": "CHEQUE",
"verificationData": {
"additionalIdRefNumber": "string",
"additionalIdType": "DRIVERS_LICENCE",
"cardHolderBillingAddress": {
"city": "string",
"countryCode": "str",
"createdAt": "2019-08-24T14:15:22Z",
"id": 0,
"line1": "string",
"line2": "string",
"postalCode": "string",
"postalCodeExtension": "string",
"primary": true,
"status": "ADDED",
"territory": "string",
"territoryType": "STATE",
"type": "HOME",
"updatedAt": "2019-08-24T14:15:22Z"
},
"cvData": "string",
"cvSkipReason": "NOT_PRESENT",
"keySerialNumber": "string",
"pin": "string",
"threeDSecureData": {
"cryptogram": "string",
"eci": "string"
}
}
},
"id": "497f6eca-6276-4993-bfeb-53cbbbba6f08",
"links": [
{
"href": "string",
"method": "string",
"rel": "string"
}
],
"notes": "string",
"parentId": "70850378-7d3c-4f45-91b7-942d4dfbbd43",
"partialAuthEnabled": true,
"partiallyApproved": true,
"paymentTokenUsed": true,
"pinCaptured": true,
"poyntLoyalty": {
"externalId": "string",
"loyalty": [
{
"businessLoyaltyId": 0,
"campaignDescription": "string",
"campaignName": "string",
"lastIncrement": 0,
"loyaltyType": "string",
"loyaltyUnit": "string",
"nextTier": "string",
"points": 0,
"pointsRequired": 0,
"rewardDescription": "string",
"tier": "string",
"totalPoints": 0,
"totalSpend": 0,
"totalVisits": 0
}
],
"loyaltyId": 0,
"reward": [
{
"businessLoyaltyId": 0,
"expireAt": "2019-08-24T14:15:22Z",
"newReward": true,
"postText": "string",
"preText": "string",
"rewardDescription": "string",
"rewardId": 0,
"status": "string",
"type": "string",
"value": 0
}
]
},
"processorOptions": {
"property1": "string",
"property2": "string"
},
"processorResponse": {
"acquirer": "CHASE_PAYMENTECH",
"adapterId": "string",
"adapterVariant": "string",
"approvalCode": "string",
"approvedAmount": 0,
"avsResult": {
"actualResult": "string",
"addressResult": "MATCH",
"cardHolderNameResult": "MATCH",
"cityResult": "MATCH",
"countryResult": "MATCH",
"phoneResult": "MATCH",
"postalCodeResult": "MATCH",
"stateResult": "MATCH"
},
"batchAutoClosedByHost": "string",
"batchId": "string",
"cardToken": "string",
"cvActualResult": "string",
"cvResult": "MATCH",
"debitResponseCode": "string",
"echeckProcessor": "MOCK",
"emvTags": {
"property1": "string",
"property2": "string"
},
"interacMac": "string",
"pinSessionKey": "string",
"processedAt": "2019-08-24T14:15:22Z",
"processor": "CHASE_PAYMENTECH",
"providerVerification": {
"publicKeyHash": "string",
"signature": "string"
},
"remainingBalance": 0,
"retrievalRefNum": "string",
"scaResult": "ENTER_PIN",
"status": "Successful",
"statusCode": "string",
"statusMessage": "string",
"transactionId": "string"
},
"reason": {
"program": "NO_SHOW",
"programFor": [
"RESTAURANT"
]
},
"receiptEmailAddress": "string",
"receiptPhone": {
"areaCode": "string",
"createdAt": "2019-08-24T14:15:22Z",
"extensionNumber": "string",
"id": 0,
"ituCountryCode": "string",
"localPhoneNumber": "string",
"primaryDayTime": true,
"primaryEvening": true,
"status": "ADDED",
"type": "HOME",
"updatedAt": "2019-08-24T14:15:22Z"
},
"references": [
{
"customType": "string",
"id": "string",
"type": "POYNT_ORDER"
}
],
"reversalVoid": true,
"settled": true,
"shippingAddress": {
"city": "string",
"countryCode": "str",
"createdAt": "2019-08-24T14:15:22Z",
"id": 0,
"line1": "string",
"line2": "string",
"postalCode": "string",
"postalCodeExtension": "string",
"primary": true,
"status": "ADDED",
"territory": "string",
"territoryType": "STATE",
"type": "HOME",
"updatedAt": "2019-08-24T14:15:22Z"
},
"signature": [
-128
],
"signatureCaptured": true,
"signatureRequired": true,
"status": "CREATED",
"stayType": "GENERAL_CONTAINER",
"systemTraceAuditNumber": "string",
"transactionNumber": "string",
"updatedAt": "2019-08-24T14:15:22Z",
"voided": true
}
Sample Response
{
"fees": [
{
"amount": 0,
"feeProgramType": "SURCHARGE",
"feeType": "FIXED",
"required": true,
"signature": "string",
"feeProgramId": "927253be-0294-4a36-80e7-7d0d8c175f0c"
}
]
}
Check Elegible Fees
This endpoint should be called to check if the transaction is eligible for any fees. A 200 response means the call was successful and a list of those eligible fees will be returned in the response body, otherwise a 404 is returned if no resource is found.
Once you have the list of eligibleFees, it can then be used in the POST call to the transaction endpoint to create the transaction with the corresponding surcharge. The transaction model allows incorporating such fees into the amounts property. Be sure to include each elible fee in its entirety (including any signatures) in the eligibleFees that you pass to the POST to the transaction endpoint.
Path Parameters
businessId
path- string (required)
Headers
- Content-Type: application/json’
- Authorization: Bearer {appAccessToken}’
- requestId: string
Request Body Schema
Customers
Operations for customers of a business
Definition
GET /businesses/{businessId}/customers
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.getCustomers = function getCustomers(options, next) { ... }
module/**
* Get all customers of a business by various criteria.
* @param {String} options.businessId
* @param {String} options.startAt (optional)
* @param {Integer} options.startOffset (optional)
* @param {String} options.endAt (optional)
* @param {Integer} options.limit (optional)
* @param {String} options.cardNumberFirst6 (optional)
* @param {String} options.cardNumberLast4 (optional)
* @param {Integer} options.cardExpirationMonth (optional)
* @param {Integer} options.cardExpirationYear (optional)
* @param {String} options.cardHolderFirstName (optional)
* @param {String} options.cardHolderLastName (optional)
* @return {CustomerList} customers
*/
Sample Request
.getCustomers({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
startOffset : 0,
limit : 1
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"count": 37,
"customers": [
{"attributes": {},
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"businessPreferences": {
"emailReceipt": false,
"preferredCardId": 0,
"printPaperReceipt": false,
"useCardOnFile": false
,
}"cards": [
{"cardHolderFirstName": "",
"cardHolderFullName": "/",
"cardHolderLastName": "",
"expirationDate": 31,
"expirationMonth": 8,
"expirationYear": 2022,
"id": 243043,
"numberFirst6": "405413",
"numberLast4": "0833",
"status": "ACTIVE",
"type": "VISA"
},
]"createdAt": "2017-01-04T22:20:40Z",
"emails": {},
"firstName": "",
"id": 5247839,
"lastName": "",
"loyaltyCustomers": [],
"phones": {},
"updatedAt": "2017-01-04T22:20:40Z"
},
]"links": [
{"href": "/businesses/18f071cc-5ed4-4b33-80c1-305056d42bfb/customers?startAt=2017-01-06T01%3A23%3A10Z&endAt=2017-08-28T07%3A03%3A20Z&limit=1",
"method": "GET",
"rel": "next"
}
] }
Definition
@classmethod
def get_customers(cls, business_id, start_at=None, start_offset=None,
=None, limit=None, card_number_first_6=None,
end_at=None, card_expiration_month=None,
card_number_last_4=None, card_holder_first_name=None,
card_expiration_year=None):
card_holder_last_name"""
Get all customers of a business by various criteria.
Arguments:
business_id (str): the business ID
Keyword arguments:
start_at (int, optional): get customers created after this time in seconds
start_offset (int, optional): the numeric offset to start the list (for pagination)
end_at (int, optional): get customers created before this time in seconds
limit (int, optional): how many customers to return (for pagination)
card_number_first_6 (str, optional): return customers with card numbers starting with this
card_number_last_4 (str, optional): return customers with card numbers ending with this
card_expiration_month (str, optional): return customers with this card expiration month
card_expiration_year (str, optional): return customers with this card expiration year
card_holder_first_name (str, optional): return customers with first name matching this
card_holder_last_name (str, optional): return customers with last name matching this
"""
Sample Request
= poynt.Customer.get_customers(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
=0,
start_offset=1
limit
)
if status_code < 300:
print(doc)
Sample Response
u'count': 37, u'customers': [{u'lastName': u'', u'loyaltyCustomers': [], u'firstName': u'', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'phones': {}, u'businessPreferences': {u'emailReceipt': False, u'preferredCardId': 0, u'printPaperReceipt': False, u'useCardOnFile': False}, u'emails': {}, u'updatedAt': u'2017-01-04T22:20:40Z', u'cards': [{u'status': u'ACTIVE', u'expirationMonth': 8, u'cardHolderFullName': u'/', u'cardHolderLastName': u'', u'cardHolderFirstName': u'', u'expirationYear': 2022, u'expirationDate': 31, u'numberLast4': u'0833', u'type': u'VISA', u'id': 243043, u'numberFirst6': u'405413'}], u'attributes': {}, u'id': 5247839, u'createdAt': u'2017-01-04T22:20:40Z'}], u'links': [{u'href': u'/businesses/18f071cc-5ed4-4b33-80c1-305056d42bfb/customers?startAt=2017-01-06T01%3A23%3A10Z&endAt=2017-08-28T07%3A03%3A53Z&limit=1', u'method': u'GET', u'rel': u'next'}]} {
Search customers
Get all customers of a business by various criterias.
Arguments
businessId
path- string (required)
If-Modified-Since
header- string (optional)
startAt
query- string (optional)
startOffset
query- integer (optional)
endAt
query- string (optional)
limit
query- integer (optional)
cardNumberFirst6
query- string (optional)
cardNumberLast4
query- string (optional)
cardExpirationMonth
query- integer (optional)
cardExpirationYear
query- integer (optional)
cardHolderFirstName
query- string (optional)
cardHolderLastName
query- string (optional)
cardOnFile
query- boolean (optional)
Response
Returns a CustomerList.
Definition
GET /businesses/{businessId}/customers/{customerId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.getCustomer = function getCustomer(options, next) { ... }
module/**
* Get a single customer at a business.
* @param {String} options.businessId
* @param {String} options.customerId
* @return {Customer} customer
*/
Sample Request
.getCustomer({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
customerId : 5247839
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"createdAt": "2017-01-04T22:20:40Z",
"updatedAt": "2017-01-04T22:20:40Z",
"businessPreferences": {
"useCardOnFile": false,
"emailReceipt": false,
"printPaperReceipt": false,
"preferredCardId": 0
,
}"insights": {
"since": "2017-01-04T22:20:40Z",
"scores": [
{"score": 8.59,
"type": "OVERALL"
},
]"totalOrders": 0
,
}"cards": [
{"type": "VISA",
"status": "ACTIVE",
"expirationDate": 31,
"expirationMonth": 8,
"expirationYear": 2022,
"id": 243043,
"numberFirst6": "405413",
"numberLast4": "0833",
"cardHolderFirstName": "",
"cardHolderLastName": "",
"cardHolderFullName": "/"
},
]"loyaltyCustomers": [],
"id": 5247839,
"emails": {},
"phones": {},
"attributes": {},
"firstName": "",
"lastName": "",
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb"
}
Definition
@classmethod
def get_customer(cls, business_id, customer_id):
"""
Get a single customer at a business.
Arguments:
business_id (str): the business ID
customer_id (str): the customer ID
"""
Sample Request
= poynt.Customer.get_customer(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
5247839
)
if status_code < 300:
print(doc)
Sample Response
u'lastName': u'', u'loyaltyCustomers': [], u'firstName': u'', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'phones': {}, u'businessPreferences': {u'emailReceipt': False, u'preferredCardId': 0, u'printPaperReceipt': False, u'useCardOnFile': False}, u'emails': {}, u'updatedAt': u'2017-01-04T22:20:40Z', u'cards': [{u'status': u'ACTIVE', u'expirationMonth': 8, u'cardHolderFullName': u'/', u'cardHolderLastName': u'', u'cardHolderFirstName': u'', u'expirationYear': 2022, u'expirationDate': 31, u'numberLast4': u'0833', u'type': u'VISA', u'id': 243043, u'numberFirst6': u'405413'}], u'attributes': {}, u'id': 5247839, u'createdAt': u'2017-01-04T22:20:40Z', u'insights': {u'totalOrders': 0, u'since': u'2017-01-04T22:20:40Z', u'scores': [{u'score': 3.09, u'type': u'OVERALL'}]}} {
Get By Id
Get customer by id.
Arguments
If-Modified-Since
header- string (optional)
businessId
path- string (required)
customerId
path- integer (required)
Response
Returns a Customer.
Definition
POST /businesses/{businessId}/customers
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
This method is currently not possible using the Node.js SDK.
Definition
This method is currently not possible using the Python SDK.
Create a customer
Create a customer of a business.
Arguments
businessId
path- string (required)
customer
body- Customer (optional)
Response
Returns a Customer.
Definition
POST /businesses/{businessId}/customers/lookup
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
This method is currently not possible using the Node.js SDK.
Definition
This method is currently not possible using the Python SDK.
Lookup by card
Find a customer by his card.
Arguments
businessId
path- string (required)
card
body- Card (optional)
Response
Returns a array.
Definition
PATCH /businesses/{businessId}/customers/{customerId}
Sample Request
curl --location --request PATCH 'https://services.poynt.net/businesses/a3f24e9e-6764-42f2-bbcd-5541e7f3be38/customers/380847254' \
--header 'Authorization: Bearer {access_token}' \
--data-raw '[ { "op": "add", "path": "/firstName", "value": "Shiny" }, { "op": "replace", "path": "/emails", "value": { "PERSONAL": { "emailAddress": "xyz@gmail.com" }, "WORK": { "emailAddress": "xyz@godaddy.com" } }
}, { "op": "replace", "path": "/phones", "value": { "WORK": { "ituCountryCode":"1", "areaCode":"650", "localPhoneNumber":"3226872", "status":"ADDED", "type":"BUSINESS" } } } ]'
Sample Response
{
"createdAt": "2022-06-02T01:42:27Z",
"updatedAt": "2023-02-13T14:00:35Z",
"businessPreferences": {
"useCardOnFile": false,
"emailReceipt": false,
"printPaperReceipt": false,
"preferredCardId": 0
},
"insights": {
"since": "2022-06-02T01:42:27Z",
"scores": [
{
"score": 3.09,
"type": "OVERALL"
}
],
"poyntLoyalty": {
"loyalty": [],
"reward": []
}
},
"cards": [],
"loyaltyCustomers": [],
"userIdentities": [],
"id": 380847254,
"emails": {
"PERSONAL": {
"emailAddress": "xyz@gmail.com"
},
"WORK": {
"emailAddress": "xyz@godaddy.com"
}
},
"phones": {
"WORK": {
"createdAt": "2023-02-13T14:20:33Z",
"updatedAt": "2023-02-13T14:20:33Z",
"id": 9789886,
"status": "ADDED",
"type": "BUSINESS",
"ituCountryCode": "1",
"areaCode": "650",
"localPhoneNumber": "3226872"
}
},
"attributes": {},
"firstName": "Shiny",
"lastName": "Sarma",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38"
}
Definition
: Add definition TODO
Sample Request
var request = require('request');
var options = {
'method': 'PATCH',
'url': 'https://services.poynt.net/businesses/a3f24e9e-6764-42f2-bbcd-5541e7f3be38/customers/380847254',
'headers': {
'Authorization': 'Bearer {access_token}',
'Content-Type': 'application/json',
'Poynt-Request-Id': 'abbe8d66-a2ad-45ec-877e-c894d1b3edf3'
,
}body: JSON.stringify([{
"op": "add",
"path": "/firstName",
"value": "Shiny"
, {
}"op": "replace",
"path": "/emails",
"value": {
"PERSONAL": {
"emailAddress": "xyz@gmail.com"
,
}"WORK": {
"emailAddress": "xyz@godaddy.com"
}
}, {
}"op": "replace",
"path": "/phones",
"value": {
"WORK": {
"ituCountryCode": "1",
"areaCode": "650",
"localPhoneNumber": "3226872",
"status": "ADDED",
"type": "BUSINESS"
}
}
}])
;
}request(options, function(error, response) {
if (error) throw new Error(error);
console.log(response.body);
; })
Sample Response
{
"createdAt": "2022-06-02T01:42:27Z",
"updatedAt": "2023-02-13T14:00:35Z",
"businessPreferences": {
"useCardOnFile": false,
"emailReceipt": false,
"printPaperReceipt": false,
"preferredCardId": 0
},
"insights": {
"since": "2022-06-02T01:42:27Z",
"scores": [
{
"score": 3.09,
"type": "OVERALL"
}
],
"poyntLoyalty": {
"loyalty": [],
"reward": []
}
},
"cards": [],
"loyaltyCustomers": [],
"userIdentities": [],
"id": 380847254,
"emails": {
"PERSONAL": {
"emailAddress": "xyz@gmail.com"
},
"WORK": {
"emailAddress": "xyz@godaddy.com"
}
},
"phones": {
"WORK": {
"createdAt": "2023-02-13T14:20:33Z",
"updatedAt": "2023-02-13T14:20:33Z",
"id": 9789886,
"status": "ADDED",
"type": "BUSINESS",
"ituCountryCode": "1",
"areaCode": "650",
"localPhoneNumber": "3226872"
}
},
"attributes": {},
"firstName": "Shiny",
"lastName": "Sarma",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38"
}
Definition
TODO: Add definition
Sample Request
import requests
import json
= "https://services.poynt.net/businesses/a3f24e9e-6764-42f2-bbcd-5541e7f3be38/customers/380847254"
url
= json.dumps([{
payload "op": "add",
"path": "/firstName",
"value": "Shiny"
}, {"op": "replace",
"path": "/emails",
"value": {
"PERSONAL": {
"emailAddress": "xyz@gmail.com"
},"WORK": {
"emailAddress": "xyz@godaddy.com"
}
}
}, {"op": "replace",
"path": "/phones",
"value": {
"WORK": {
"ituCountryCode": "1",
"areaCode": "650",
"localPhoneNumber": "3226872",
"status": "ADDED",
"type": "BUSINESS"
}
}= {
}]) headers 'Authorization': 'Bearer {access_token}',
'Content-Type': 'application/json',
'Poynt-Request-Id': '4229430d-9bd3-40eb-9f47-81a3f49d67cb'
}
= requests.request("PATCH", url, headers = headers, data = payload)
response
print(response.text)
Sample Response
{
"createdAt": "2022-06-02T01:42:27Z",
"updatedAt": "2023-02-13T14:00:35Z",
"businessPreferences": {
"useCardOnFile": false,
"emailReceipt": false,
"printPaperReceipt": false,
"preferredCardId": 0
},
"insights": {
"since": "2022-06-02T01:42:27Z",
"scores": [
{
"score": 3.09,
"type": "OVERALL"
}
],
"poyntLoyalty": {
"loyalty": [],
"reward": []
}
},
"cards": [],
"loyaltyCustomers": [],
"userIdentities": [],
"id": 380847254,
"emails": {
"PERSONAL": {
"emailAddress": "xyz@gmail.com"
},
"WORK": {
"emailAddress": "xyz@godaddy.com"
}
},
"phones": {
"WORK": {
"createdAt": "2023-02-13T14:20:33Z",
"updatedAt": "2023-02-13T14:20:33Z",
"id": 9789886,
"status": "ADDED",
"type": "BUSINESS",
"ituCountryCode": "1",
"areaCode": "650",
"localPhoneNumber": "3226872"
}
},
"attributes": {},
"firstName": "Shiny",
"lastName": "Sarma",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38"
}
Update customer
Update a customer. This takes a JsonPatch object as request. We allow updates to all fields except id, businessId, createdAt, cards, devices and insights.
Arguments
Poynt-Request-Id
header- string (required)
businessId
path- string (required)
customerId
path- integer (required)
patch
body- JsonPatch (optional)
Response
Returns a Customer.
Merchants
Businesses
Business resource represents the merchant business. The Poynt system assumes the following business hierarchy. There is the parent business at the root. Each business could have 0 or more stores. Each store could have 0 or more terminals. This resource provides operations to create, update and view this entire hierarchy.
Definition
GET /businesses
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Get by Parameters
Get a business by various parameters.
Arguments
acquirer
query- string (optional)
storeDeviceId
query- string (optional)
mid
query- string (optional)
isBusinessLevelMid
query- boolean (optional, default is false)
tid
query- string (optional)
ignoreDeactivatedDevices
query- boolean (optional, default is true)
includeField
query- Set (optional)
If-Modified-Since
header- string (optional)
Response
Returns a Business.
Definition
GET /businesses/{businessId}
Sample Request
curl --location --request GET 'https://services.poynt.net/businesses/a3f24e9e-6764-42f2-bbcd-5541e7f3be38' \
--header 'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json'
Sample Response
{
"address": {
"status": "ADDED",
"type": "BUSINESS",
"createdAt": "2021-01-08T01:51:13Z",
"updatedAt": "2023-02-06T13:30:00Z",
"id": 1336635,
"line1": "3032 Bunker Hill Ln",
"line2": "",
"city": "Santa Clara",
"territory": "CA",
"postalCode": "95054",
"countryCode": "US",
"territoryType": "STATE"
},
"mockProcessor": true,
"scrubbed": false,
"status": "ACTIVATED",
"type": "TEST_MERCHANT",
"activeSince": "1970-01-01T00:00:00Z",
"createdAt": "2021-01-08T01:51:13Z",
"updatedAt": "2023-02-06T13:30:01Z",
"attributes": {
"enableCheck": "0",
"enableCatalogV2": "0",
"enableDepositChangedBy": "",
"alipayEligible": "0",
"enableSmartTip": "0",
"overrideTimezoneName": "",
"enableDebitCardCustomization": "0",
"disableEMVCT": "0",
"defaultScreenBrightness": "-1",
"settlementMode": "HOST",
"enablePoyntTerminalApp": "1",
"disableEMVCL": "0",
"useDeviceLogin": "1",
"firstTaxAmount": "0",
"distributorId": "87b7aa4c-598f-450f-bd27-a4a6d5848de4",
"enableManualEntryDiners": "1",
"tipType2": "P",
"tipType1": "P",
"enablePreApproved": "0",
"tipType3": "P",
"dinersCommercialCode": "",
"enablePoyntInvoicesApp": "1",
"roleInventoryPermissions": "13",
"tutorialWebOnboarded": "0",
"vtDeviceId": "",
"showToSBanner": "1",
"enableRefundChangedBy": "",
"merchantContactFirstName": "Kastureema",
"tipScreenTimeoutInMilliSecs": "10000",
"enableMenuBoard": "0",
"enablePaymentChangeReason": "",
"enableYolotea": "0",
"roleSupportPermissions": "1,2,3,19",
"distributorName": "Kastureema's organization",
"fundomateToken": "",
"enableOrderInboxApp": "0",
"enableDepositChangeReason": "",
"jcbCardBinList": "",
"disableForceTransactions": "0",
"enableManualEntry": "1",
"enableAddCardVerify": "0",
"businessBundleIds": "635bd79a-64a7-43a2-aee7-2a8f0ccdd8d9",
"enableManualEntryMastercard": "1",
"enablePoyntStoreApp": "1",
"maestroBankFundedIndicator": "0",
"requireDeviceLogin": "R",
"removeCardWaitTime": "60",
"disableLegacyScannerFlow": "0",
"pcAppKey": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38=urn:aid:a7cc7e72-a402-4b81-9a31-323288c19737",
"enableOffline": "0",
"enableLocalSettlement": "0",
"showPayfacVirtualCard": "0",
"visaBankFundedIndicator": "1",
"autoCapture": "0",
"roleSalesPermissions": "1,41,14,15",
"showDebitCardBanner": "0",
"dccRebatePercentage": "0",
"disableSMSReceipt": "0",
"getAdditionalSiftInfo": "0",
"cardPresentPercentage": "100",
"merchantBundlesCohort": "",
"PUSH_TEST": "1",
"payfacCaseActivityId": "",
"enableManualEntryDiscover": "1",
"enableGratuity": "1",
"deployMode": "0",
"enableActivationEmails": "1",
"enableAutomaticSettlement": "0",
"receiptService": "co.poynt.services",
"enableEBT": "0",
"bankId": "",
"socialTwitter": "",
"enableVisa": "1",
"showLargerFontOnReceipts": "0",
"enableCashback": "0",
"enableManualEntryAmex": "1",
"enablePayfacDashboard": "0",
"fundomateOfferAccepted": "0",
"enablePayment": "1",
"enableScanOnlyPayment": "0",
"paymentOptionsImageUrl": "",
"newPremium": "0",
"pcDomainWhiteList": "",
"tutorialsCompleted": "0",
"payLinksDomainSslStatus": "",
"ivu": "0",
"customScreenBrightness": "0",
"enableTutorialFlow": "0",
"lodgingPromptTotalStayAmount": "1",
"crap": "0",
"enableFulfillment": "1",
"firstTaxLabel": "Tax 1",
"riskStatus": "",
"customerWelcomeMessage": "",
"mastercardCommercialCode": "",
"overrideTimezone": "0",
"payfacOrganizationId": "",
"disableCustomContactPhone": "0",
"enableVoiceAuth": "0",
"enableDCC": "0",
"godaddyVentureId": "",
"disableEmailReceipt": "0",
"enableCatalogApp": "1",
"supportContactPhone": "",
"enablePoyntCardTransactionAlert": "1",
"skipSignatureAmount": "0",
"logoImageUrl": "",
"underwritingLevel": "FULL",
"maxOfflineTransactions": "1000",
"noPlanMode": "0",
"displayProcessorTxnId": "0",
"useLegacyReceipts": "0",
"autoSettleAuths": "0",
"showBatchNumberReceipt": "0",
"smartTipThreshold": "1000",
"localeCountry": "US",
"enableOnlineStore": "0",
"debitCardCustomizationChanged": "0",
"godaddyPayments": "0",
"enablePoyntRegisterApp": "1",
"enableSecondScreenLanguagePref": "0",
"salesAgentEmail": "",
"defaultScreenOffTimeoutInSecs": "-1",
"dinersSENumber": "",
"enableLoyalty": "0",
"enableSettlementSweep": "1",
"salesManagerName": "",
"existingPremium": "0",
"disableDebit": "1",
"enableMSM": "0",
"automaticReportsTypes": "TRANSACTIONS,ITEMS",
"unionpayBankFundedIndicator": "0",
"enableVerifyCard": "0",
"enableTerminalAppTax": "0",
"pcApiKey": "3afc8257-b898-4925-8a4f-9ef847eeaa91",
"transactionListLimitSize": "1000",
"ecommerceBusinessName": "",
"dinersCardBinList": "",
"enableCustomerFeedback": "1",
"stopAfterCardReadRecords": "0",
"signoutAfterTransaction": "0",
"enableIosTransactionNotification": "0",
"enableMerchantPresentQrCode": "0",
"enableUserRoles": "0",
"showVTCardPresenceForManualEntry": "0",
"enableNotTransactedEmails": "1",
"fulfillmentOrderProcess": "MANUAL",
"disablePaperReceipt": "0",
"enableCalculatorApp": "1",
"newSettlementPath": "",
"enableDebitCards": "0",
"enableCampaignCreation": "0",
"defaultTaxRate": "0",
"enableWeeklyInsightsEmails": "1",
"mastercardBankFundedIndicator": "1",
"localeLanguage": "en-us",
"firstTaxRate": "0",
"forceSettlementOne": "0",
"godaddyCustomerId": "",
"paymentTimeoutInSecs": "-1",
"decisionFulfill": "",
"existingBasic": "0",
"disableNonRefRefunds": "0",
"enableCustomPaymentsImage": "1",
"mastercardCardBinList": "",
"presentBundleDiscount": "0",
"enableAuthOnly": "1",
"enableSiftTracking": "0",
"showOnlyCityAndStateOnReceipts": "0",
"enableFulfillmentChangedBy": "",
"maestroCommercialCode": "",
"useVTForManualEntry": "1",
"disableEMVCTDebit": "0",
"debitAID2": "",
"debitCardsChanged": "0",
"debitAID3": "",
"enableInventory": "0",
"debitAID1": "A0000000042203,A0000000980840,A0000001524010,A0000006200620,A000000333010108",
"taxDisplayOrder": "",
"newAdvanced": "0",
"enableCustomerPresentQrCode": "0",
"godaddyServiceId": "",
"salesAgentName": "",
"visaCommercialCode": "",
"referralUrlId": "",
"amexBankFundedIndicator": "0",
"enableHqSettlement": "0",
"enableEBTCashCashback": "0",
"enableAdvancedCatalog": "0",
"lodgingTaxRate": "0",
"closingTime": "",
"customContactEmail": "",
"seenTransitionMessage": "0",
"autoInventoryUpdate": "0",
"fraudServiceType": "",
"autoSelectCommonDebitAID": "1",
"newBasic": "0",
"enableHqAdjustments": "0",
"enableManualEntryVisa": "1",
"terminalRequireInvoiceId": "0",
"autoForwardTipScreen": "0",
"enableBalanceInquiry": "0",
"maestroCardBinList": "",
"enableMastercard": "1",
"jcbCommercialCode": "",
"enablePoyntSettlementApp": "1",
"customFooterText": "",
"operatingHours": "",
"enableManualEntryUnionPay": "1",
"enableRefund": "1",
"roleAccountingPermissions": "0,1,11,12,17,34",
"validatePANForManualEntry": "0",
"enableADA": "0",
"preventApiAttributesChange": "0",
"secondScreenLanguages": "",
"secondTaxAmount": "0",
"enableDiscover": "1",
"enableReportEmails": "1",
"existingAdvanced": "0",
"socialYelp": "",
"supportedCustomFunding": "",
"parentMid": "",
"enableAlphanumericZip": "0",
"enableAccounting": "0",
"salesManagerId": "",
"enablePushLowBatteryNotification": "1",
"enableLocalVt": "0",
"enabledAppTutorial": "",
"excludeTaxesFromTip": "0",
"socialFacebook": "",
"enableAddCard": "0",
"blockPoyntCollect": "0",
"showBankFundedOnSettlement": "0",
"alacarteLoyaltyTrialUsed": "0",
"amexCommercialCode": "",
"payfacCaseId": "",
"enableManualCVV": "1",
"defaultPayLinkId": "",
"enableCustomTaxAmount": "0",
"enableEmployeeSettlementView": "0",
"disableEBTFSRefund": "0",
"enableDiners": "1",
"enableNewSettlementApp": "0",
"acceptedOfflineAgreement": "0",
"payLinksDomainDnsStatus": "",
"reportingContactEmail": "",
"enablePoyntHQApp": "1",
"voucherAID1": "",
"enablePaymentChangedBy": "",
"hideCustomerPaymentIcons": "1",
"enableNavbar": "0",
"smartTipAmount2": "200",
"unionpayCommercialCode": "",
"smartTipAmount3": "300",
"twiyoContactEmail": "",
"webOnboarded": "0",
"decisionEnableProducts": "",
"enableOther": "0",
"alwaysShowAddCard": "0",
"disableWaitForCardRemoval": "0",
"enableEBTCreditBalInq": "0",
"smartTipAmount1": "100",
"godaddyServiceType": "",
"enableFirstTax": "0",
"enableManualEntryNRR": "0",
"disableCustomContactEmail": "0",
"autoForwardReceiptScreen": "0",
"enableAmex": "1",
"enableAutomaticReports": "0",
"enableTerminalTaxEditing": "0",
"discoverSENumber": "",
"enableGDPHQ": "0",
"merchantBackgroundImageUrl": "",
"amexCardBinList": "",
"enableNonADASounds": "0",
"alacarteInvoicingTrialUsed": "0",
"disableCCRefund": "0",
"alwaysSkipSignature": "0",
"enableEBTCashPurchase": "0",
"alacarteQuickbooksTrialUsed": "0",
"enableSecondTax": "0",
"socialWebsite": "",
"customerScreenMessage": "",
"showDebitCardBlockedModal": "0",
"disableEBTForceRefund": "0",
"visaCardBinList": "",
"enableIosReportNotification": "1",
"enableWebAdjustments": "0",
"salesManagerEmail": "",
"visaSENumber": "",
"enableFulfillmentChangeReason": "",
"tipAmount1": "100",
"receiptScreenTimeoutInMilliSecs": "10000",
"tipAmount2": "200",
"eCheckStoreProcessor": "1",
"verifySignature": "0",
"viewScope": "TERMINAL",
"ecommerceCheckoutButtonColor": "",
"automaticSettlementTime": "",
"secondTaxLabel": "Tax 2",
"enableInvoicesWithCof": "0",
"enableManualEntryTerminalApp": "1",
"disableOrderInbox": "0",
"enableOrderTaxForTxn": "0",
"customContactPhone": "",
"signoutAfterSuspend": "0",
"tutorialsSkipped": "0",
"enableAddCardAuthOnly": "0",
"payfacCampaignId": "",
"enableNewHQ": "0",
"creditAID3": "",
"creditAID4": "",
"creditAID5": "",
"tipPercent3": "20",
"tipPercent2": "18",
"tipPercent1": "15",
"useNewHQ": "1",
"paymentProcessor": "co.poynt.services",
"riskStatusChangeReason": "",
"tipBeforeCardRead": "0",
"godaddyShopperId": "",
"enableManualZipStreet": "1",
"showPoyntCollect": "0",
"creditAID1": "",
"creditAID2": "",
"disableEMVCLForCashback": "0",
"purchaseAction": "AUTHORIZE",
"npsWasShown": "0",
"showDCCRebateOnSettlement": "0",
"tipAmount3": "300",
"skipCVMAmount": "2500",
"enableOfflineFeature": "0",
"payfacApplicationId": "",
"dinersBankFundedIndicator": "0",
"payfacProfileId": "",
"enableNotSettledEmails": "1",
"payfacAgentId": "",
"secondTaxRate": "0",
"enableInsertCardAPI": "0",
"disableEMVCLDebit": "0",
"appInvoiceFailedPaymentReminder": "0",
"showAddressOnReceipts": "1",
"enableIosTransactionVolumeYDay": "1",
"enablePaidScanner": "0",
"enableCash": "1",
"disableLCDR": "0",
"freeTrialSubscriptionId": "5b9c24a1-28ac-4142-8770-423ff37b046f",
"grantAppPermissions": "undefined",
"smartTipPercent3": "20",
"enableRefundChangeReason": "",
"amexSENumber": "",
"cashbackAmountLimit": "30000",
"showCustomTipByDefault": "0",
"thirdTaxLabel": "Tax 3",
"enableJcb": "1",
"preferredStoreId": "",
"customerBackgroundImageUrl": "",
"enableHqVirtualTerminal": "0",
"skipCVM": "0",
"godaddyProjectId": "",
"fundomateLoanFunded": "0",
"enableManualEntryJcb": "1",
"processorStatus": "",
"ecommerceCheckoutLogoUrl": "",
"maxOfflineTransactionValue": "10000",
"showDebitCardSuspendModal": "0",
"enableFulfillmentType": "",
"preinstallApks": "",
"enableAgeVerification": "0",
"disallowEditing": "0",
"enableManualZip": "1",
"salesAgentId": "",
"alacarteVirtualTerminalTrialUsed": "0",
"cardPreferenceType": "",
"roleMarketingPermissions": "7,8,9,29,30,43",
"bankName": "",
"customScreenOffTimeoutInSecs": "0",
"disableCCForce": "0",
"maxOfflineTotal": "1000000",
"enablePoyntTransactionsApp": "1",
"payLinksDomain": "",
"smartTipType": "A",
"unionpayCardBinList": "",
"automaticReportsTime": "0000",
"supportContactEmail": "",
"enableEBTFoodStamp": "0",
"hideCustomerName": "0",
"enableBaseAmountAdjust": "0",
"merchantContactLastName": "Sarma",
"smartTipPercent2": "18",
"hideCustomerWelcomeMessage": "0",
"smartTipPercent1": "15",
"showPoyntCapital": "0",
"enableInvoices": "0",
"disableOrderAutoClose": "0",
"lodgingEnableFolio": "0",
"enableEBTCashOnly": "0",
"jcbBankFundedIndicator": "0",
"riskStatusChangedBy": "",
"enablePoyntLodgingApp": "0",
"excludeTaxesFromCashback": "0"
},
"phone": {
"createdAt": "2021-01-08T01:51:13Z",
"updatedAt": "2023-02-06T13:30:00Z",
"id": 1348710,
"status": "ADDED",
"type": "BUSINESS",
"ituCountryCode": "1",
"areaCode": "653",
"localPhoneNumber": "6889630"
},
"acquirer": "CHASE_PAYMENTECH",
"processor": "CREDITCALL",
"externalMerchantId": "reemad45d",
"legalName": "Reema Chai Cafe",
"businessUrl": "http://reema chai cafe.com",
"emailAddress": "kastureema@poynt.co",
"doingBusinessAs": "Reema Chai Cafe",
"description": "Just another test merchant",
"industryType": "Restaurant",
"mcc": "5812",
"sic": "5812",
"timezone": "America/Los_Angeles",
"underwritingLevel": "FULL",
"id": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38"
}
Definition
.exports.getBusiness = function getBusiness(options, next) { ... }
module/**
* Gets a business by ID.
* @param {String} options.businessId
* @return {Business} business
*/
Sample Request
.getBusiness({
poyntbusinessId: '18f071cc-5ed4-4b33-80c1-305056d42bfb'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"acquirer": "ELAVON",
"activeSince": "1970-01-01T00:00:00Z",
"address": {
"city": "Palo Alto",
"countryCode": "USA",
"createdAt": "2017-01-04T22:04:33Z",
"id": 48119,
"line1": "4151 Middlefield Road",
"line2": "Level 2",
"postalCode": "94303",
"status": "ADDED",
"territory": "California",
"territoryType": "STATE",
"type": "BUSINESS",
"updatedAt": "2017-08-25T01:05:33Z"
,
}"attributes": { ... },
"businessUrl": "http://lawrence-s-last-straw.com",
"createdAt": "2017-01-04T22:04:34Z",
"description": "Just another test merchant",
"doingBusinessAs": "Lawrence's Last Straw",
"emailAddress": "lawrence-s-last-straw@gmail.com",
"externalMerchantId": "lawrence's0226",
"id": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"industryType": "Restaurant",
"legalName": "Lawrence's Last Straw",
"mcc": "5812",
"mockProcessor": false,
"phone": {
"areaCode": "575",
"createdAt": "2017-01-04T22:04:33Z",
"id": 50700,
"ituCountryCode": "1",
"localPhoneNumber": "2040375",
"status": "ADDED",
"type": "BUSINESS",
"updatedAt": "2017-08-25T01:05:33Z"
,
}"processor": "ELAVON",
"sic": "5812",
"status": "ACTIVATED",
"stores": [
{"acquirer": "ELAVON",
"address": {
"city": "Palo Alto",
"countryCode": "USA",
"createdAt": "2017-01-04T22:04:33Z",
"id": 48120,
"line1": "10617 Business Drive",
"line2": "Suite 424",
"postalCode": "94301",
"status": "ADDED",
"territory": "California",
"territoryType": "STATE",
"type": "BUSINESS",
"updatedAt": "2017-08-16T02:59:42Z"
,
}"attributes": { ... },
"currency": "USD",
"displayName": "Lawrence's Last Straw 488",
"externalStoreId": "161779901711",
"fixedLocation": true,
"id": "c394627f-4f68-47fb-90a5-684ea801a352",
"latitude": 37.4457,
"longitude": -122.162,
"mockProcessor": false,
"phone": {
"areaCode": "575",
"createdAt": "2017-01-04T22:04:33Z",
"id": 50701,
"ituCountryCode": "1",
"localPhoneNumber": "2040375",
"status": "ADDED",
"type": "BUSINESS",
"updatedAt": "2017-08-16T02:59:42Z"
,
}"processor": "ELAVON",
"processorData": {},
"status": "ACTIVE",
"storeDevices": [
{"businessAgreements": {
"EULA": {
"acceptedAt": "2017-07-25T01:36:14Z",
"current": true,
"type": "EULA",
"userId": 5247838,
"version": "1f",
"versionOutdated": false
},
}"catalogId": "9deca670-8761-4133-b1a9-b05b9fb01cb6",
"createdAt": "2017-01-04T22:10:00Z",
"deviceId": "urn:tid:48c54303-6d51-39af-bdeb-4af53f621652",
"externalTerminalId": "8024696638530",
"name": "LAWRENCE'S LAST STRAW DON'T MESS",
"publicKey": "-----BEGIN PUBLIC KEY-----\nMIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAyl+Sa2C1E6xcv9d9Mm9N\ngtzDtSsjWd9k7PZPDLy3JRq3dhGU9rQtopfEUrtqRUDRS7xpP3842enns39Z427+\nwOuAq7aqVLHzCIio8+D0m21mmsKpE8rSH54dCKciSypuj/MF4o+jIMA43+ov6dba\nN/6WFH/+c4MzTqz673XldP9RAvrK8k55lKoZW5bvX5Bx0xmFbtey17Uyby+y6kT+\nTyQArYwzEFBA2RQ+LKf/XwIgoh6RcdMVlNahe5LNW2rl5FQGm+HxnvYG6ZQGQ4oX\nFEGClsvOQabdjVXNNA6rVmJeeKmSekVNFjA3ZqEcmq8E6ijmT/H1upmiZ5fI0Eag\nSwIDAQAB\n-----END PUBLIC KEY-----\n",
"serialNumber": "P61SWA231FS000416",
"status": "ACTIVATED",
"storeId": "c394627f-4f68-47fb-90a5-684ea801a352",
"type": "TERMINAL",
"updatedAt": "2017-08-16T04:26:19Z"
},
]"timezone": "America/Los_Angeles"
},
]"timezone": "America/Los_Angeles",
"type": "TEST_MERCHANT",
"updatedAt": "2017-08-25T01:05:33Z"
}
Definition
@classmethod
def get_business(cls, business_id):
"""
Gets a business by ID.
Arguments:
business_id (str): the business ID to get
"""
Sample Request
= poynt.Business.get_business(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb'
)
if status_code < 300:
print(doc)
Sample Response
u'stores': [{u'status': u'ACTIVE', u'processorData': {}, u'externalStoreId': u'161779901711', u'displayName': u"Lawrence's Last Straw 488", u'currency': u'USD', u'mockProcessor': False, u'longitude': -122.162, u'id': u'c394627f-4f68-47fb-90a5-684ea801a352', u'storeDevices': [{u'status': u'ACTIVATED', u'storeId': u'c394627f-4f68-47fb-90a5-684ea801a352', u'name': u"LAWRENCE'S LAST STRAW DON'T MESS", u'serialNumber': u'P61SWA231FS000416', u'businessAgreements': {u'EULA': {u'userId': 5247838, u'versionOutdated': False, u'current': True, u'version': u'1f', u'acceptedAt': u'2017-07-25T01:36:14Z', u'type': u'EULA'}}, u'externalTerminalId': u'8024696638530', u'publicKey': u'-----BEGIN PUBLIC KEY-----\nMIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAyl+Sa2C1E6xcv9d9Mm9N\ngtzDtSsjWd9k7PZPDLy3JRq3dhGU9rQtopfEUrtqRUDRS7xpP3842enns39Z427+\nwOuAq7aqVLHzCIio8+D0m21mmsKpE8rSH54dCKciSypuj/MF4o+jIMA43+ov6dba\nN/6WFH/+c4MzTqz673XldP9RAvrK8k55lKoZW5bvX5Bx0xmFbtey17Uyby+y6kT+\nTyQArYwzEFBA2RQ+LKf/XwIgoh6RcdMVlNahe5LNW2rl5FQGm+HxnvYG6ZQGQ4oX\nFEGClsvOQabdjVXNNA6rVmJeeKmSekVNFjA3ZqEcmq8E6ijmT/H1upmiZ5fI0Eag\nSwIDAQAB\n-----END PUBLIC KEY-----\n', u'deviceId': u'urn:tid:48c54303-6d51-39af-bdeb-4af53f621652', u'updatedAt': u'2017-08-16T04:26:19Z', u'catalogId': u'9deca670-8761-4133-b1a9-b05b9fb01cb6', u'type': u'TERMINAL', u'createdAt': u'2017-01-04T22:10:00Z'}], u'phone': {u'status': u'ADDED', u'areaCode': u'575', u'localPhoneNumber': u'2040375', u'updatedAt': u'2017-08-16T02:59:42Z', u'ituCountryCode': u'1', u'type': u'BUSINESS', u'id': 50701, u'createdAt': u'2017-01-04T22:04:33Z'}, u'timezone': u'America/Los_Angeles', u'acquirer': u'ELAVON', u'address': {u'status': u'ADDED', u'city': u'Palo Alto', u'countryCode': u'USA', u'line2': u'Suite 424', u'line1': u'10617 Business Drive', u'territoryType': u'STATE', u'updatedAt': u'2017-08-16T02:59:42Z', u'postalCode': u'94301', u'territory': u'California', u'type': u'BUSINESS', u'id': 48120, u'createdAt': u'2017-01-04T22:04:33Z'}, u'latitude': 37.4457, u'attributes': {}, u'processor': u'ELAVON', u'fixedLocation': True}], u'businessUrl': u'http://lawrence-s-last-straw.com', u'updatedAt': u'2017-08-25T01:05:33Z', u'timezone': u'America/Los_Angeles', u'id': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'industryType': u'Restaurant', u'sic': u'5812', u'acquirer': u'ELAVON', u'type': u'TEST_MERCHANT', u'status': u'ACTIVATED', u'description': u'Just another test merchant', u'mockProcessor': False, u'mcc': u'5812', u'activeSince': u'1970-01-01T00:00:00Z', u'phone': {u'status': u'ADDED', u'areaCode': u'575', u'localPhoneNumber': u'2040375', u'updatedAt': u'2017-08-25T01:05:33Z', u'ituCountryCode': u'1', u'type': u'BUSINESS', u'id': 50700, u'createdAt': u'2017-01-04T22:04:33Z'}, u'emailAddress': u'lawrence-s-last-straw@gmail.com', u'address': {u'status': u'ADDED', u'city': u'Palo Alto', u'countryCode': u'USA', u'line2': u'Level 2', u'line1': u'4151 Middlefield Road', u'territoryType': u'STATE', u'updatedAt': u'2017-08-25T01:05:33Z', u'postalCode': u'94303', u'territory': u'California', u'type': u'BUSINESS', u'id': 48119, u'createdAt': u'2017-01-04T22:04:33Z'}, u'externalMerchantId': u"lawrence's0226", u'doingBusinessAs': u"Lawrence's Last Straw", u'createdAt': u'2017-01-04T22:04:34Z', u'legalName': u"Lawrence's Last Straw", u'attributes': {}, u'processor': u'ELAVON'} {
Get by businessId
Get a business by id.
Arguments
businessId
path- string (required)
storeId
query- string (optional)
storeDeviceId
query- string (optional)
includeField
query- Set (optional)
ignoreDeactivatedDevices
query- boolean (optional, default is true)
Response
Returns a Business.
Definition
GET /businesses/{businessId}/stores/{storeId}
Sample Request
curl --location --request GET 'https://services.poynt.net/businesses/a3f24e9e-6764-42f2-bbcd-5541e7f3be38/stores/0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700' \
--header 'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json'
Sample Response
{
"address": {
"status": "ADDED",
"type": "BUSINESS",
"createdAt": "2021-01-08T01:51:13Z",
"updatedAt": "2023-01-24T05:04:28Z",
"id": 1336636,
"line1": "3032 Bunker Hill Ln",
"line2": "",
"city": "Santa Clara",
"territory": "CA",
"postalCode": "95054",
"countryCode": "US",
"territoryType": "STATE"
},
"fixedLocation": true,
"mockProcessor": true,
"longitude": -121.98188,
"latitude": 37.404964,
"storeDevices": [
{
"createdAt": "2021-01-08T01:51:13Z",
"updatedAt": "2022-09-09T19:00:03Z",
"status": "ACTIVATED",
"type": "TERMINAL",
"externalTerminalId": "wjbq",
"deviceId": "urn:tid:faf60b63-16d7-3cd6-bdab-bc7fc5b2310a",
"serialNumber": "P6EMUA91051723",
"name": "Kastureema's Emulator",
"publicKey": "-----BEGIN PUBLIC KEY-----\nMIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAtX9KIg4/0S9foDYYpXoD\n/7nB14rFbGa+DSzJjHh6wuaANnoyrHdbnvwCxHdBjum+M2kTc4LpebHDY8tYpbAg\nAD7V09nMzCtYji6x77wmCTQS5BhHxBaQmPUAld4wNG2cAjY5gXQ4Jt0ERuiJf6P+\n9W9T/dtQwh5CgH4hBwTRf4Co2bAiHp78o4cPBTp1KFy2FkxzneTLLSck1esDnddP\nOqA0kLKSANttZxjKn0ybEafpzWmN77Hy+xNWAz8FvZdm7NK90z5GmoscpTKnaGlS\n+n5OMAIzlljm4SS1H+V0SSJG6U7f04bI/xJIN3CUykVnaT8jjO7pU847vJ0iGnWO\nNwIDAQAB\n-----END PUBLIC KEY-----\n",
"catalogId": "7eba8d76-b08e-4137-95c7-deabe4b89cc3",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
{
"createdAt": "2022-04-28T03:35:31Z",
"updatedAt": "2022-12-29T21:28:43Z",
"status": "CREATED",
"type": "TERMINAL",
"externalTerminalId": "123",
"catalogId": "7eba8d76-b08e-4137-95c7-deabe4b89cc3",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
}
],
"attributes": {
"enableCheck": "0",
"enableCatalogV2": "0",
"enableDepositChangedBy": "",
"alipayEligible": "0",
"enableSmartTip": "0",
"overrideTimezoneName": "",
"enableDebitCardCustomization": "0",
"disableEMVCT": "0",
"defaultScreenBrightness": "-1",
"settlementMode": "HOST",
"enablePoyntTerminalApp": "1",
"disableEMVCL": "0",
"useDeviceLogin": "1",
"firstTaxAmount": "0",
"distributorId": "87b7aa4c-598f-450f-bd27-a4a6d5848de4",
"enableManualEntryDiners": "1",
"tipType2": "P",
"tipType1": "P",
"enablePreApproved": "0",
"tipType3": "P",
"dinersCommercialCode": "",
"enablePoyntInvoicesApp": "1",
"roleInventoryPermissions": "13",
"tutorialWebOnboarded": "0",
"vtDeviceId": "",
"showToSBanner": "1",
"enableRefundChangedBy": "",
"merchantContactFirstName": "Kastureema",
"tipScreenTimeoutInMilliSecs": "10000",
"enableMenuBoard": "0",
"enablePaymentChangeReason": "",
"enableYolotea": "0",
"roleSupportPermissions": "1,2,3,19",
"distributorName": "Kastureema's organization",
"fundomateToken": "",
"enableOrderInboxApp": "0",
"enableDepositChangeReason": "",
"jcbCardBinList": "",
"disableForceTransactions": "0",
"enableManualEntry": "1",
"enableAddCardVerify": "0",
"businessBundleIds": "635bd79a-64a7-43a2-aee7-2a8f0ccdd8d9",
"enableManualEntryMastercard": "1",
"enablePoyntStoreApp": "1",
"maestroBankFundedIndicator": "0",
"requireDeviceLogin": "R",
"removeCardWaitTime": "60",
"disableLegacyScannerFlow": "0",
"pcAppKey": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38=urn:aid:a7cc7e72-a402-4b81-9a31-323288c19737",
"feeValuePercentage": "0",
"enableOffline": "0",
"enableLocalSettlement": "0",
"showPayfacVirtualCard": "0",
"visaBankFundedIndicator": "1",
"autoCapture": "0",
"roleSalesPermissions": "1,41,14,15",
"showDebitCardBanner": "0",
"dccRebatePercentage": "0",
"disableSMSReceipt": "0",
"getAdditionalSiftInfo": "0",
"cardPresentPercentage": "100",
"merchantBundlesCohort": "",
"PUSH_TEST": "1",
"payfacCaseActivityId": "",
"enableManualEntryDiscover": "1",
"enableGratuity": "1",
"payLinksDomainDnsErrorCode": "",
"deployMode": "0",
"enableActivationEmails": "1",
"enableAutomaticSettlement": "0",
"receiptService": "co.poynt.services",
"enableEBT": "0",
"bankId": "",
"socialTwitter": "",
"enableVisa": "1",
"showLargerFontOnReceipts": "0",
"enableCashback": "0",
"enableManualEntryAmex": "1",
"enablePayfacDashboard": "0",
"fundomateOfferAccepted": "0",
"enablePayment": "1",
"enableScanOnlyPayment": "0",
"paymentOptionsImageUrl": "https://d2olcuyf08j3e6.cloudfront.net/banners/1586447430824_AMEX,APPLEPAY,DISCOVER,GOOGLEPAY,JCB,MASTERCARD,VISA.png",
"newPremium": "0",
"pcDomainWhiteList": "",
"tutorialsCompleted": "0",
"payLinksDomainSslStatus": "",
"ivu": "0",
"customScreenBrightness": "0",
"enableTutorialFlow": "0",
"lodgingPromptTotalStayAmount": "1",
"crap": "0",
"enableFulfillment": "1",
"firstTaxLabel": "Tax 1",
"riskStatus": "",
"customerWelcomeMessage": "",
"mastercardCommercialCode": "",
"overrideTimezone": "0",
"payfacOrganizationId": "",
"disableCustomContactPhone": "0",
"enableVoiceAuth": "0",
"enableDCC": "0",
"godaddyVentureId": "",
"disableEmailReceipt": "0",
"enableCatalogApp": "1",
"supportContactPhone": "",
"enablePoyntCardTransactionAlert": "1",
"skipSignatureAmount": "0",
"logoImageUrl": "",
"underwritingLevel": "FULL",
"maxOfflineTransactions": "1000",
"noPlanMode": "0",
"displayProcessorTxnId": "0",
"useLegacyReceipts": "0",
"autoSettleAuths": "0",
"showBatchNumberReceipt": "0",
"smartTipThreshold": "1000",
"localeCountry": "US",
"enableOnlineStore": "0",
"debitCardCustomizationChanged": "0",
"godaddyPayments": "0",
"enablePoyntRegisterApp": "1",
"enableSecondScreenLanguagePref": "0",
"salesAgentEmail": "",
"defaultScreenOffTimeoutInSecs": "-1",
"dinersSENumber": "",
"enableLoyalty": "0",
"enableSettlementSweep": "1",
"salesManagerName": "",
"existingPremium": "0",
"disableDebit": "0",
"enableMSM": "0",
"automaticReportsTypes": "TRANSACTIONS,ITEMS",
"unionpayBankFundedIndicator": "0",
"enableVerifyCard": "0",
"enableTerminalAppTax": "0",
"pcApiKey": "3afc8257-b898-4925-8a4f-9ef847eeaa91",
"transactionListLimitSize": "1000",
"ecommerceBusinessName": "",
"dinersCardBinList": "",
"enableCustomerFeedback": "1",
"stopAfterCardReadRecords": "0",
"signoutAfterTransaction": "0",
"enableIosTransactionNotification": "0",
"enableMerchantPresentQrCode": "0",
"enableUserRoles": "0",
"showVTCardPresenceForManualEntry": "0",
"enableNotTransactedEmails": "1",
"fulfillmentOrderProcess": "MANUAL",
"disablePaperReceipt": "0",
"enableCalculatorApp": "1",
"newSettlementPath": "",
"enableDebitCards": "0",
"enableNewOrderSoundNotification": "0",
"enableCampaignCreation": "0",
"defaultTaxRate": "0",
"enableWeeklyInsightsEmails": "1",
"mastercardBankFundedIndicator": "1",
"payLinksDomainSslErrorText": "",
"localeLanguage": "en-us",
"firstTaxRate": "0",
"forceSettlementOne": "0",
"godaddyCustomerId": "",
"paymentTimeoutInSecs": "-1",
"decisionFulfill": "",
"existingBasic": "0",
"disableNonRefRefunds": "0",
"enableCustomPaymentsImage": "1",
"mastercardCardBinList": "",
"presentBundleDiscount": "0",
"enableAuthOnly": "1",
"enableSiftTracking": "0",
"showOnlyCityAndStateOnReceipts": "0",
"enableFulfillmentChangedBy": "",
"maestroCommercialCode": "",
"useVTForManualEntry": "1",
"disableEMVCTDebit": "0",
"debitAID2": "",
"debitCardsChanged": "0",
"debitAID3": "",
"enableInventory": "0",
"debitAID1": "A0000000042203,A0000000980840,A0000001524010,A0000006200620,A000000333010108",
"taxDisplayOrder": "",
"newAdvanced": "0",
"payLinksDomainDnsErrorText": "",
"enableCustomerPresentQrCode": "0",
"godaddyServiceId": "",
"salesAgentName": "",
"visaCommercialCode": "",
"referralUrlId": "",
"amexBankFundedIndicator": "0",
"enableHqSettlement": "0",
"enableEBTCashCashback": "0",
"enableAdvancedCatalog": "0",
"lodgingTaxRate": "0",
"closingTime": "",
"customContactEmail": "",
"seenTransitionMessage": "0",
"feeValueFixed": "0",
"autoInventoryUpdate": "0",
"fraudServiceType": "",
"autoSelectCommonDebitAID": "1",
"newBasic": "0",
"enableHqAdjustments": "0",
"enableManualEntryVisa": "1",
"terminalRequireInvoiceId": "0",
"autoForwardTipScreen": "0",
"enableBalanceInquiry": "0",
"maestroCardBinList": "",
"enableMastercard": "1",
"jcbCommercialCode": "",
"enablePoyntSettlementApp": "1",
"customFooterText": "",
"operatingHours": "",
"enableManualEntryUnionPay": "1",
"enableRefund": "1",
"roleAccountingPermissions": "0,1,11,12,17,34",
"validatePANForManualEntry": "0",
"enableADA": "0",
"preventApiAttributesChange": "0",
"secondScreenLanguages": "",
"secondTaxAmount": "0",
"enableDiscover": "1",
"enableReportEmails": "1",
"existingAdvanced": "0",
"socialYelp": "",
"supportedCustomFunding": "",
"parentMid": "",
"enableAlphanumericZip": "0",
"enableAccounting": "0",
"salesManagerId": "",
"enablePushLowBatteryNotification": "1",
"enableLocalVt": "0",
"enabledAppTutorial": "",
"requireCardholderConfirmation": "1",
"excludeTaxesFromTip": "0",
"socialFacebook": "",
"enableAddCard": "0",
"blockPoyntCollect": "0",
"showBankFundedOnSettlement": "0",
"alacarteLoyaltyTrialUsed": "0",
"amexCommercialCode": "",
"payfacCaseId": "",
"enableManualCVV": "1",
"defaultPayLinkId": "",
"enableCustomTaxAmount": "0",
"enableConvenienceFee": "0",
"enableEmployeeSettlementView": "0",
"disableEBTFSRefund": "0",
"enableDiners": "1",
"enableNewSettlementApp": "0",
"acceptedOfflineAgreement": "0",
"payLinksDomainDnsStatus": "",
"reportingContactEmail": "",
"enablePoyntHQApp": "1",
"voucherAID1": "",
"enablePaymentChangedBy": "",
"hideCustomerPaymentIcons": "1",
"enableNavbar": "1",
"smartTipAmount2": "200",
"unionpayCommercialCode": "",
"smartTipAmount3": "300",
"twiyoContactEmail": "",
"webOnboarded": "0",
"decisionEnableProducts": "",
"enableOther": "0",
"alwaysShowAddCard": "0",
"disableWaitForCardRemoval": "0",
"enableEBTCreditBalInq": "0",
"smartTipAmount1": "100",
"godaddyServiceType": "",
"enableFirstTax": "0",
"enableManualEntryNRR": "0",
"disableCustomContactEmail": "0",
"autoForwardReceiptScreen": "0",
"enableAmex": "1",
"enableAutomaticReports": "0",
"enableTerminalTaxEditing": "0",
"discoverSENumber": "",
"enableGDPHQ": "0",
"merchantBackgroundImageUrl": "",
"amexCardBinList": "",
"enableNonADASounds": "0",
"alacarteInvoicingTrialUsed": "0",
"disableCCRefund": "0",
"alwaysSkipSignature": "0",
"enableEBTCashPurchase": "0",
"alacarteQuickbooksTrialUsed": "0",
"enableSecondTax": "0",
"socialWebsite": "",
"payLinksDomainSslErrorCode": "",
"customerScreenMessage": "",
"showDebitCardBlockedModal": "0",
"disableEBTForceRefund": "0",
"visaCardBinList": "",
"enableIosReportNotification": "1",
"enableWebAdjustments": "0",
"salesManagerEmail": "",
"visaSENumber": "",
"enableFulfillmentChangeReason": "",
"tipAmount1": "100",
"receiptScreenTimeoutInMilliSecs": "10000",
"tipAmount2": "200",
"eCheckStoreProcessor": "1",
"verifySignature": "0",
"viewScope": "TERMINAL",
"ecommerceCheckoutButtonColor": "",
"automaticSettlementTime": "",
"secondTaxLabel": "Tax 2",
"enableInvoicesWithCof": "0",
"enableManualEntryTerminalApp": "1",
"disableOrderInbox": "0",
"enableOrderTaxForTxn": "0",
"customContactPhone": "",
"signoutAfterSuspend": "0",
"tutorialsSkipped": "0",
"enableAddCardAuthOnly": "0",
"payfacCampaignId": "",
"enableNewHQ": "0",
"payLinksDomainAttachErrorText": "",
"creditAID3": "",
"disableCardOnFile": "0",
"creditAID4": "",
"creditAID5": "",
"tipPercent3": "20",
"tipPercent2": "18",
"tipPercent1": "15",
"useNewHQ": "1",
"paymentProcessor": "co.poynt.services",
"riskStatusChangeReason": "",
"tipBeforeCardRead": "1",
"godaddyShopperId": "",
"enableManualZipStreet": "1",
"showPoyntCollect": "0",
"creditAID1": "",
"creditAID2": "",
"disableEMVCLForCashback": "0",
"purchaseAction": "AUTHORIZE",
"npsWasShown": "0",
"showDCCRebateOnSettlement": "0",
"tipAmount3": "300",
"skipCVMAmount": "2500",
"enableOfflineFeature": "0",
"payfacApplicationId": "",
"dinersBankFundedIndicator": "0",
"payfacProfileId": "",
"enableNotSettledEmails": "1",
"payfacAgentId": "",
"secondTaxRate": "0",
"enableInsertCardAPI": "0",
"disableEMVCLDebit": "0",
"appInvoiceFailedPaymentReminder": "0",
"showAddressOnReceipts": "1",
"enableIosTransactionVolumeYDay": "1",
"enablePaidScanner": "0",
"enableCash": "1",
"disableLCDR": "0",
"freeTrialSubscriptionId": "5b9c24a1-28ac-4142-8770-423ff37b046f",
"grantAppPermissions": "undefined",
"smartTipPercent3": "20",
"enableRefundChangeReason": "",
"amexSENumber": "",
"cashbackAmountLimit": "30000",
"enableImmediatePrintOrderReceipt": "0",
"showCustomTipByDefault": "0",
"thirdTaxLabel": "Tax 3",
"enableNewRegisterAppFlow": "0",
"enableJcb": "1",
"preferredStoreId": "",
"customerBackgroundImageUrl": "",
"enableHqVirtualTerminal": "0",
"skipCVM": "0",
"godaddyProjectId": "",
"fundomateLoanFunded": "0",
"enableManualEntryJcb": "1",
"processorStatus": "",
"ecommerceCheckoutLogoUrl": "",
"feeCalculation": "",
"maxOfflineTransactionValue": "10000",
"showDebitCardSuspendModal": "0",
"enableFulfillmentType": "",
"preinstallApks": "",
"enableAgeVerification": "0",
"disallowEditing": "0",
"enableManualZip": "1",
"salesAgentId": "",
"alacarteVirtualTerminalTrialUsed": "0",
"cardPreferenceType": "",
"roleMarketingPermissions": "7,8,9,29,30,43",
"bankName": "",
"customScreenOffTimeoutInSecs": "0",
"disableCCForce": "0",
"maxOfflineTotal": "1000000",
"enablePoyntTransactionsApp": "1",
"enableNewOrderNotification": "0",
"payLinksDomain": "",
"smartTipType": "A",
"unionpayCardBinList": "",
"automaticReportsTime": "0000",
"supportContactEmail": "",
"enableEBTFoodStamp": "0",
"hideCustomerName": "0",
"enableBaseAmountAdjust": "0",
"merchantContactLastName": "Sarma",
"smartTipPercent2": "18",
"hideCustomerWelcomeMessage": "0",
"smartTipPercent1": "15",
"showPoyntCapital": "0",
"enableInvoices": "0",
"disableOrderAutoClose": "0",
"lodgingEnableFolio": "0",
"enableEBTCashOnly": "0",
"jcbBankFundedIndicator": "0",
"riskStatusChangedBy": "",
"enablePoyntLodgingApp": "0",
"excludeTaxesFromCashback": "0"
},
"processorData": {},
"phone": {
"createdAt": "2021-01-08T01:51:13Z",
"updatedAt": "2023-01-24T05:04:28Z",
"id": 1348711,
"status": "ADDED",
"type": "BUSINESS",
"ituCountryCode": "1",
"areaCode": "653",
"localPhoneNumber": "6889630"
},
"acquirer": "CHASE_PAYMENTECH",
"processor": "CREDITCALL",
"status": "ACTIVE",
"externalStoreId": "a07u39smq0",
"displayName": "Reema Chai Cafe 504",
"currency": "USD",
"timezone": "America/Los_Angeles",
"id": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
}
Definition
: Add definition TODO
Sample Request
var request = require('request');
var options = {
'method': 'GET',
'url': 'https://services.poynt.net/businesses/a3f24e9e-6764-42f2-bbcd-5541e7f3be38/stores/0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700',
'headers': {
'Authorization': 'Bearer {access_token}',
'Content-Type': 'application/json'
};
}request(options, function(error, response) {
if (error) throw new Error(error);
console.log(response.body);
; })
Sample Response
{
"address": {
"status": "ADDED",
"type": "BUSINESS",
"createdAt": "2021-01-08T01:51:13Z",
"updatedAt": "2023-01-24T05:04:28Z",
"id": 1336636,
"line1": "3032 Bunker Hill Ln",
"line2": "",
"city": "Santa Clara",
"territory": "CA",
"postalCode": "95054",
"countryCode": "US",
"territoryType": "STATE"
},
"fixedLocation": true,
"mockProcessor": true,
"longitude": -121.98188,
"latitude": 37.404964,
"storeDevices": [
{
"createdAt": "2021-01-08T01:51:13Z",
"updatedAt": "2022-09-09T19:00:03Z",
"status": "ACTIVATED",
"type": "TERMINAL",
"externalTerminalId": "wjbq",
"deviceId": "urn:tid:faf60b63-16d7-3cd6-bdab-bc7fc5b2310a",
"serialNumber": "P6EMUA91051723",
"name": "Kastureema's Emulator",
"publicKey": "-----BEGIN PUBLIC KEY-----\nMIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAtX9KIg4/0S9foDYYpXoD\n/7nB14rFbGa+DSzJjHh6wuaANnoyrHdbnvwCxHdBjum+M2kTc4LpebHDY8tYpbAg\nAD7V09nMzCtYji6x77wmCTQS5BhHxBaQmPUAld4wNG2cAjY5gXQ4Jt0ERuiJf6P+\n9W9T/dtQwh5CgH4hBwTRf4Co2bAiHp78o4cPBTp1KFy2FkxzneTLLSck1esDnddP\nOqA0kLKSANttZxjKn0ybEafpzWmN77Hy+xNWAz8FvZdm7NK90z5GmoscpTKnaGlS\n+n5OMAIzlljm4SS1H+V0SSJG6U7f04bI/xJIN3CUykVnaT8jjO7pU847vJ0iGnWO\nNwIDAQAB\n-----END PUBLIC KEY-----\n",
"catalogId": "7eba8d76-b08e-4137-95c7-deabe4b89cc3",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
{
"createdAt": "2022-04-28T03:35:31Z",
"updatedAt": "2022-12-29T21:28:43Z",
"status": "CREATED",
"type": "TERMINAL",
"externalTerminalId": "123",
"catalogId": "7eba8d76-b08e-4137-95c7-deabe4b89cc3",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
}
],
"attributes": {
"enableCheck": "0",
"enableCatalogV2": "0",
"enableDepositChangedBy": "",
"alipayEligible": "0",
"enableSmartTip": "0",
"overrideTimezoneName": "",
"enableDebitCardCustomization": "0",
"disableEMVCT": "0",
"defaultScreenBrightness": "-1",
"settlementMode": "HOST",
"enablePoyntTerminalApp": "1",
"disableEMVCL": "0",
"useDeviceLogin": "1",
"firstTaxAmount": "0",
"distributorId": "87b7aa4c-598f-450f-bd27-a4a6d5848de4",
"enableManualEntryDiners": "1",
"tipType2": "P",
"tipType1": "P",
"enablePreApproved": "0",
"tipType3": "P",
"dinersCommercialCode": "",
"enablePoyntInvoicesApp": "1",
"roleInventoryPermissions": "13",
"tutorialWebOnboarded": "0",
"vtDeviceId": "",
"showToSBanner": "1",
"enableRefundChangedBy": "",
"merchantContactFirstName": "Kastureema",
"tipScreenTimeoutInMilliSecs": "10000",
"enableMenuBoard": "0",
"enablePaymentChangeReason": "",
"enableYolotea": "0",
"roleSupportPermissions": "1,2,3,19",
"distributorName": "Kastureema's organization",
"fundomateToken": "",
"enableOrderInboxApp": "0",
"enableDepositChangeReason": "",
"jcbCardBinList": "",
"disableForceTransactions": "0",
"enableManualEntry": "1",
"enableAddCardVerify": "0",
"businessBundleIds": "635bd79a-64a7-43a2-aee7-2a8f0ccdd8d9",
"enableManualEntryMastercard": "1",
"enablePoyntStoreApp": "1",
"maestroBankFundedIndicator": "0",
"requireDeviceLogin": "R",
"removeCardWaitTime": "60",
"disableLegacyScannerFlow": "0",
"pcAppKey": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38=urn:aid:a7cc7e72-a402-4b81-9a31-323288c19737",
"feeValuePercentage": "0",
"enableOffline": "0",
"enableLocalSettlement": "0",
"showPayfacVirtualCard": "0",
"visaBankFundedIndicator": "1",
"autoCapture": "0",
"roleSalesPermissions": "1,41,14,15",
"showDebitCardBanner": "0",
"dccRebatePercentage": "0",
"disableSMSReceipt": "0",
"getAdditionalSiftInfo": "0",
"cardPresentPercentage": "100",
"merchantBundlesCohort": "",
"PUSH_TEST": "1",
"payfacCaseActivityId": "",
"enableManualEntryDiscover": "1",
"enableGratuity": "1",
"payLinksDomainDnsErrorCode": "",
"deployMode": "0",
"enableActivationEmails": "1",
"enableAutomaticSettlement": "0",
"receiptService": "co.poynt.services",
"enableEBT": "0",
"bankId": "",
"socialTwitter": "",
"enableVisa": "1",
"showLargerFontOnReceipts": "0",
"enableCashback": "0",
"enableManualEntryAmex": "1",
"enablePayfacDashboard": "0",
"fundomateOfferAccepted": "0",
"enablePayment": "1",
"enableScanOnlyPayment": "0",
"paymentOptionsImageUrl": "https://d2olcuyf08j3e6.cloudfront.net/banners/1586447430824_AMEX,APPLEPAY,DISCOVER,GOOGLEPAY,JCB,MASTERCARD,VISA.png",
"newPremium": "0",
"pcDomainWhiteList": "",
"tutorialsCompleted": "0",
"payLinksDomainSslStatus": "",
"ivu": "0",
"customScreenBrightness": "0",
"enableTutorialFlow": "0",
"lodgingPromptTotalStayAmount": "1",
"crap": "0",
"enableFulfillment": "1",
"firstTaxLabel": "Tax 1",
"riskStatus": "",
"customerWelcomeMessage": "",
"mastercardCommercialCode": "",
"overrideTimezone": "0",
"payfacOrganizationId": "",
"disableCustomContactPhone": "0",
"enableVoiceAuth": "0",
"enableDCC": "0",
"godaddyVentureId": "",
"disableEmailReceipt": "0",
"enableCatalogApp": "1",
"supportContactPhone": "",
"enablePoyntCardTransactionAlert": "1",
"skipSignatureAmount": "0",
"logoImageUrl": "",
"underwritingLevel": "FULL",
"maxOfflineTransactions": "1000",
"noPlanMode": "0",
"displayProcessorTxnId": "0",
"useLegacyReceipts": "0",
"autoSettleAuths": "0",
"showBatchNumberReceipt": "0",
"smartTipThreshold": "1000",
"localeCountry": "US",
"enableOnlineStore": "0",
"debitCardCustomizationChanged": "0",
"godaddyPayments": "0",
"enablePoyntRegisterApp": "1",
"enableSecondScreenLanguagePref": "0",
"salesAgentEmail": "",
"defaultScreenOffTimeoutInSecs": "-1",
"dinersSENumber": "",
"enableLoyalty": "0",
"enableSettlementSweep": "1",
"salesManagerName": "",
"existingPremium": "0",
"disableDebit": "0",
"enableMSM": "0",
"automaticReportsTypes": "TRANSACTIONS,ITEMS",
"unionpayBankFundedIndicator": "0",
"enableVerifyCard": "0",
"enableTerminalAppTax": "0",
"pcApiKey": "3afc8257-b898-4925-8a4f-9ef847eeaa91",
"transactionListLimitSize": "1000",
"ecommerceBusinessName": "",
"dinersCardBinList": "",
"enableCustomerFeedback": "1",
"stopAfterCardReadRecords": "0",
"signoutAfterTransaction": "0",
"enableIosTransactionNotification": "0",
"enableMerchantPresentQrCode": "0",
"enableUserRoles": "0",
"showVTCardPresenceForManualEntry": "0",
"enableNotTransactedEmails": "1",
"fulfillmentOrderProcess": "MANUAL",
"disablePaperReceipt": "0",
"enableCalculatorApp": "1",
"newSettlementPath": "",
"enableDebitCards": "0",
"enableNewOrderSoundNotification": "0",
"enableCampaignCreation": "0",
"defaultTaxRate": "0",
"enableWeeklyInsightsEmails": "1",
"mastercardBankFundedIndicator": "1",
"payLinksDomainSslErrorText": "",
"localeLanguage": "en-us",
"firstTaxRate": "0",
"forceSettlementOne": "0",
"godaddyCustomerId": "",
"paymentTimeoutInSecs": "-1",
"decisionFulfill": "",
"existingBasic": "0",
"disableNonRefRefunds": "0",
"enableCustomPaymentsImage": "1",
"mastercardCardBinList": "",
"presentBundleDiscount": "0",
"enableAuthOnly": "1",
"enableSiftTracking": "0",
"showOnlyCityAndStateOnReceipts": "0",
"enableFulfillmentChangedBy": "",
"maestroCommercialCode": "",
"useVTForManualEntry": "1",
"disableEMVCTDebit": "0",
"debitAID2": "",
"debitCardsChanged": "0",
"debitAID3": "",
"enableInventory": "0",
"debitAID1": "A0000000042203,A0000000980840,A0000001524010,A0000006200620,A000000333010108",
"taxDisplayOrder": "",
"newAdvanced": "0",
"payLinksDomainDnsErrorText": "",
"enableCustomerPresentQrCode": "0",
"godaddyServiceId": "",
"salesAgentName": "",
"visaCommercialCode": "",
"referralUrlId": "",
"amexBankFundedIndicator": "0",
"enableHqSettlement": "0",
"enableEBTCashCashback": "0",
"enableAdvancedCatalog": "0",
"lodgingTaxRate": "0",
"closingTime": "",
"customContactEmail": "",
"seenTransitionMessage": "0",
"feeValueFixed": "0",
"autoInventoryUpdate": "0",
"fraudServiceType": "",
"autoSelectCommonDebitAID": "1",
"newBasic": "0",
"enableHqAdjustments": "0",
"enableManualEntryVisa": "1",
"terminalRequireInvoiceId": "0",
"autoForwardTipScreen": "0",
"enableBalanceInquiry": "0",
"maestroCardBinList": "",
"enableMastercard": "1",
"jcbCommercialCode": "",
"enablePoyntSettlementApp": "1",
"customFooterText": "",
"operatingHours": "",
"enableManualEntryUnionPay": "1",
"enableRefund": "1",
"roleAccountingPermissions": "0,1,11,12,17,34",
"validatePANForManualEntry": "0",
"enableADA": "0",
"preventApiAttributesChange": "0",
"secondScreenLanguages": "",
"secondTaxAmount": "0",
"enableDiscover": "1",
"enableReportEmails": "1",
"existingAdvanced": "0",
"socialYelp": "",
"supportedCustomFunding": "",
"parentMid": "",
"enableAlphanumericZip": "0",
"enableAccounting": "0",
"salesManagerId": "",
"enablePushLowBatteryNotification": "1",
"enableLocalVt": "0",
"enabledAppTutorial": "",
"requireCardholderConfirmation": "1",
"excludeTaxesFromTip": "0",
"socialFacebook": "",
"enableAddCard": "0",
"blockPoyntCollect": "0",
"showBankFundedOnSettlement": "0",
"alacarteLoyaltyTrialUsed": "0",
"amexCommercialCode": "",
"payfacCaseId": "",
"enableManualCVV": "1",
"defaultPayLinkId": "",
"enableCustomTaxAmount": "0",
"enableConvenienceFee": "0",
"enableEmployeeSettlementView": "0",
"disableEBTFSRefund": "0",
"enableDiners": "1",
"enableNewSettlementApp": "0",
"acceptedOfflineAgreement": "0",
"payLinksDomainDnsStatus": "",
"reportingContactEmail": "",
"enablePoyntHQApp": "1",
"voucherAID1": "",
"enablePaymentChangedBy": "",
"hideCustomerPaymentIcons": "1",
"enableNavbar": "1",
"smartTipAmount2": "200",
"unionpayCommercialCode": "",
"smartTipAmount3": "300",
"twiyoContactEmail": "",
"webOnboarded": "0",
"decisionEnableProducts": "",
"enableOther": "0",
"alwaysShowAddCard": "0",
"disableWaitForCardRemoval": "0",
"enableEBTCreditBalInq": "0",
"smartTipAmount1": "100",
"godaddyServiceType": "",
"enableFirstTax": "0",
"enableManualEntryNRR": "0",
"disableCustomContactEmail": "0",
"autoForwardReceiptScreen": "0",
"enableAmex": "1",
"enableAutomaticReports": "0",
"enableTerminalTaxEditing": "0",
"discoverSENumber": "",
"enableGDPHQ": "0",
"merchantBackgroundImageUrl": "",
"amexCardBinList": "",
"enableNonADASounds": "0",
"alacarteInvoicingTrialUsed": "0",
"disableCCRefund": "0",
"alwaysSkipSignature": "0",
"enableEBTCashPurchase": "0",
"alacarteQuickbooksTrialUsed": "0",
"enableSecondTax": "0",
"socialWebsite": "",
"payLinksDomainSslErrorCode": "",
"customerScreenMessage": "",
"showDebitCardBlockedModal": "0",
"disableEBTForceRefund": "0",
"visaCardBinList": "",
"enableIosReportNotification": "1",
"enableWebAdjustments": "0",
"salesManagerEmail": "",
"visaSENumber": "",
"enableFulfillmentChangeReason": "",
"tipAmount1": "100",
"receiptScreenTimeoutInMilliSecs": "10000",
"tipAmount2": "200",
"eCheckStoreProcessor": "1",
"verifySignature": "0",
"viewScope": "TERMINAL",
"ecommerceCheckoutButtonColor": "",
"automaticSettlementTime": "",
"secondTaxLabel": "Tax 2",
"enableInvoicesWithCof": "0",
"enableManualEntryTerminalApp": "1",
"disableOrderInbox": "0",
"enableOrderTaxForTxn": "0",
"customContactPhone": "",
"signoutAfterSuspend": "0",
"tutorialsSkipped": "0",
"enableAddCardAuthOnly": "0",
"payfacCampaignId": "",
"enableNewHQ": "0",
"payLinksDomainAttachErrorText": "",
"creditAID3": "",
"disableCardOnFile": "0",
"creditAID4": "",
"creditAID5": "",
"tipPercent3": "20",
"tipPercent2": "18",
"tipPercent1": "15",
"useNewHQ": "1",
"paymentProcessor": "co.poynt.services",
"riskStatusChangeReason": "",
"tipBeforeCardRead": "1",
"godaddyShopperId": "",
"enableManualZipStreet": "1",
"showPoyntCollect": "0",
"creditAID1": "",
"creditAID2": "",
"disableEMVCLForCashback": "0",
"purchaseAction": "AUTHORIZE",
"npsWasShown": "0",
"showDCCRebateOnSettlement": "0",
"tipAmount3": "300",
"skipCVMAmount": "2500",
"enableOfflineFeature": "0",
"payfacApplicationId": "",
"dinersBankFundedIndicator": "0",
"payfacProfileId": "",
"enableNotSettledEmails": "1",
"payfacAgentId": "",
"secondTaxRate": "0",
"enableInsertCardAPI": "0",
"disableEMVCLDebit": "0",
"appInvoiceFailedPaymentReminder": "0",
"showAddressOnReceipts": "1",
"enableIosTransactionVolumeYDay": "1",
"enablePaidScanner": "0",
"enableCash": "1",
"disableLCDR": "0",
"freeTrialSubscriptionId": "5b9c24a1-28ac-4142-8770-423ff37b046f",
"grantAppPermissions": "undefined",
"smartTipPercent3": "20",
"enableRefundChangeReason": "",
"amexSENumber": "",
"cashbackAmountLimit": "30000",
"enableImmediatePrintOrderReceipt": "0",
"showCustomTipByDefault": "0",
"thirdTaxLabel": "Tax 3",
"enableNewRegisterAppFlow": "0",
"enableJcb": "1",
"preferredStoreId": "",
"customerBackgroundImageUrl": "",
"enableHqVirtualTerminal": "0",
"skipCVM": "0",
"godaddyProjectId": "",
"fundomateLoanFunded": "0",
"enableManualEntryJcb": "1",
"processorStatus": "",
"ecommerceCheckoutLogoUrl": "",
"feeCalculation": "",
"maxOfflineTransactionValue": "10000",
"showDebitCardSuspendModal": "0",
"enableFulfillmentType": "",
"preinstallApks": "",
"enableAgeVerification": "0",
"disallowEditing": "0",
"enableManualZip": "1",
"salesAgentId": "",
"alacarteVirtualTerminalTrialUsed": "0",
"cardPreferenceType": "",
"roleMarketingPermissions": "7,8,9,29,30,43",
"bankName": "",
"customScreenOffTimeoutInSecs": "0",
"disableCCForce": "0",
"maxOfflineTotal": "1000000",
"enablePoyntTransactionsApp": "1",
"enableNewOrderNotification": "0",
"payLinksDomain": "",
"smartTipType": "A",
"unionpayCardBinList": "",
"automaticReportsTime": "0000",
"supportContactEmail": "",
"enableEBTFoodStamp": "0",
"hideCustomerName": "0",
"enableBaseAmountAdjust": "0",
"merchantContactLastName": "Sarma",
"smartTipPercent2": "18",
"hideCustomerWelcomeMessage": "0",
"smartTipPercent1": "15",
"showPoyntCapital": "0",
"enableInvoices": "0",
"disableOrderAutoClose": "0",
"lodgingEnableFolio": "0",
"enableEBTCashOnly": "0",
"jcbBankFundedIndicator": "0",
"riskStatusChangedBy": "",
"enablePoyntLodgingApp": "0",
"excludeTaxesFromCashback": "0"
},
"processorData": {},
"phone": {
"createdAt": "2021-01-08T01:51:13Z",
"updatedAt": "2023-01-24T05:04:28Z",
"id": 1348711,
"status": "ADDED",
"type": "BUSINESS",
"ituCountryCode": "1",
"areaCode": "653",
"localPhoneNumber": "6889630"
},
"acquirer": "CHASE_PAYMENTECH",
"processor": "CREDITCALL",
"status": "ACTIVE",
"externalStoreId": "a07u39smq0",
"displayName": "Reema Chai Cafe 504",
"currency": "USD",
"timezone": "America/Los_Angeles",
"id": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
}
Definition
TODO: Add definition
Sample Request
import requests
import json
= "https://services.poynt.net/businesses/a3f24e9e-6764-42f2-bbcd-5541e7f3be38/stores/0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
url
= {}
payload = {
headers 'Authorization': 'Bearer {access_token}',
'Content-Type': 'application/json'
}
= requests.request("GET", url, headers = headers, data = payload)
response
print(response.text)
Sample Response
{
"address": {
"status": "ADDED",
"type": "BUSINESS",
"createdAt": "2021-01-08T01:51:13Z",
"updatedAt": "2023-01-24T05:04:28Z",
"id": 1336636,
"line1": "3032 Bunker Hill Ln",
"line2": "",
"city": "Santa Clara",
"territory": "CA",
"postalCode": "95054",
"countryCode": "US",
"territoryType": "STATE"
},
"fixedLocation": true,
"mockProcessor": true,
"longitude": -121.98188,
"latitude": 37.404964,
"storeDevices": [
{
"createdAt": "2021-01-08T01:51:13Z",
"updatedAt": "2022-09-09T19:00:03Z",
"status": "ACTIVATED",
"type": "TERMINAL",
"externalTerminalId": "wjbq",
"deviceId": "urn:tid:faf60b63-16d7-3cd6-bdab-bc7fc5b2310a",
"serialNumber": "P6EMUA91051723",
"name": "Kastureema's Emulator",
"publicKey": "-----BEGIN PUBLIC KEY-----\nMIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAtX9KIg4/0S9foDYYpXoD\n/7nB14rFbGa+DSzJjHh6wuaANnoyrHdbnvwCxHdBjum+M2kTc4LpebHDY8tYpbAg\nAD7V09nMzCtYji6x77wmCTQS5BhHxBaQmPUAld4wNG2cAjY5gXQ4Jt0ERuiJf6P+\n9W9T/dtQwh5CgH4hBwTRf4Co2bAiHp78o4cPBTp1KFy2FkxzneTLLSck1esDnddP\nOqA0kLKSANttZxjKn0ybEafpzWmN77Hy+xNWAz8FvZdm7NK90z5GmoscpTKnaGlS\n+n5OMAIzlljm4SS1H+V0SSJG6U7f04bI/xJIN3CUykVnaT8jjO7pU847vJ0iGnWO\nNwIDAQAB\n-----END PUBLIC KEY-----\n",
"catalogId": "7eba8d76-b08e-4137-95c7-deabe4b89cc3",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
},
{
"createdAt": "2022-04-28T03:35:31Z",
"updatedAt": "2022-12-29T21:28:43Z",
"status": "CREATED",
"type": "TERMINAL",
"externalTerminalId": "123",
"catalogId": "7eba8d76-b08e-4137-95c7-deabe4b89cc3",
"storeId": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
}
],
"attributes": {
"enableCheck": "0",
"enableCatalogV2": "0",
"enableDepositChangedBy": "",
"alipayEligible": "0",
"enableSmartTip": "0",
"overrideTimezoneName": "",
"enableDebitCardCustomization": "0",
"disableEMVCT": "0",
"defaultScreenBrightness": "-1",
"settlementMode": "HOST",
"enablePoyntTerminalApp": "1",
"disableEMVCL": "0",
"useDeviceLogin": "1",
"firstTaxAmount": "0",
"distributorId": "87b7aa4c-598f-450f-bd27-a4a6d5848de4",
"enableManualEntryDiners": "1",
"tipType2": "P",
"tipType1": "P",
"enablePreApproved": "0",
"tipType3": "P",
"dinersCommercialCode": "",
"enablePoyntInvoicesApp": "1",
"roleInventoryPermissions": "13",
"tutorialWebOnboarded": "0",
"vtDeviceId": "",
"showToSBanner": "1",
"enableRefundChangedBy": "",
"merchantContactFirstName": "Kastureema",
"tipScreenTimeoutInMilliSecs": "10000",
"enableMenuBoard": "0",
"enablePaymentChangeReason": "",
"enableYolotea": "0",
"roleSupportPermissions": "1,2,3,19",
"distributorName": "Kastureema's organization",
"fundomateToken": "",
"enableOrderInboxApp": "0",
"enableDepositChangeReason": "",
"jcbCardBinList": "",
"disableForceTransactions": "0",
"enableManualEntry": "1",
"enableAddCardVerify": "0",
"businessBundleIds": "635bd79a-64a7-43a2-aee7-2a8f0ccdd8d9",
"enableManualEntryMastercard": "1",
"enablePoyntStoreApp": "1",
"maestroBankFundedIndicator": "0",
"requireDeviceLogin": "R",
"removeCardWaitTime": "60",
"disableLegacyScannerFlow": "0",
"pcAppKey": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38=urn:aid:a7cc7e72-a402-4b81-9a31-323288c19737",
"feeValuePercentage": "0",
"enableOffline": "0",
"enableLocalSettlement": "0",
"showPayfacVirtualCard": "0",
"visaBankFundedIndicator": "1",
"autoCapture": "0",
"roleSalesPermissions": "1,41,14,15",
"showDebitCardBanner": "0",
"dccRebatePercentage": "0",
"disableSMSReceipt": "0",
"getAdditionalSiftInfo": "0",
"cardPresentPercentage": "100",
"merchantBundlesCohort": "",
"PUSH_TEST": "1",
"payfacCaseActivityId": "",
"enableManualEntryDiscover": "1",
"enableGratuity": "1",
"payLinksDomainDnsErrorCode": "",
"deployMode": "0",
"enableActivationEmails": "1",
"enableAutomaticSettlement": "0",
"receiptService": "co.poynt.services",
"enableEBT": "0",
"bankId": "",
"socialTwitter": "",
"enableVisa": "1",
"showLargerFontOnReceipts": "0",
"enableCashback": "0",
"enableManualEntryAmex": "1",
"enablePayfacDashboard": "0",
"fundomateOfferAccepted": "0",
"enablePayment": "1",
"enableScanOnlyPayment": "0",
"paymentOptionsImageUrl": "https://d2olcuyf08j3e6.cloudfront.net/banners/1586447430824_AMEX,APPLEPAY,DISCOVER,GOOGLEPAY,JCB,MASTERCARD,VISA.png",
"newPremium": "0",
"pcDomainWhiteList": "",
"tutorialsCompleted": "0",
"payLinksDomainSslStatus": "",
"ivu": "0",
"customScreenBrightness": "0",
"enableTutorialFlow": "0",
"lodgingPromptTotalStayAmount": "1",
"crap": "0",
"enableFulfillment": "1",
"firstTaxLabel": "Tax 1",
"riskStatus": "",
"customerWelcomeMessage": "",
"mastercardCommercialCode": "",
"overrideTimezone": "0",
"payfacOrganizationId": "",
"disableCustomContactPhone": "0",
"enableVoiceAuth": "0",
"enableDCC": "0",
"godaddyVentureId": "",
"disableEmailReceipt": "0",
"enableCatalogApp": "1",
"supportContactPhone": "",
"enablePoyntCardTransactionAlert": "1",
"skipSignatureAmount": "0",
"logoImageUrl": "",
"underwritingLevel": "FULL",
"maxOfflineTransactions": "1000",
"noPlanMode": "0",
"displayProcessorTxnId": "0",
"useLegacyReceipts": "0",
"autoSettleAuths": "0",
"showBatchNumberReceipt": "0",
"smartTipThreshold": "1000",
"localeCountry": "US",
"enableOnlineStore": "0",
"debitCardCustomizationChanged": "0",
"godaddyPayments": "0",
"enablePoyntRegisterApp": "1",
"enableSecondScreenLanguagePref": "0",
"salesAgentEmail": "",
"defaultScreenOffTimeoutInSecs": "-1",
"dinersSENumber": "",
"enableLoyalty": "0",
"enableSettlementSweep": "1",
"salesManagerName": "",
"existingPremium": "0",
"disableDebit": "0",
"enableMSM": "0",
"automaticReportsTypes": "TRANSACTIONS,ITEMS",
"unionpayBankFundedIndicator": "0",
"enableVerifyCard": "0",
"enableTerminalAppTax": "0",
"pcApiKey": "3afc8257-b898-4925-8a4f-9ef847eeaa91",
"transactionListLimitSize": "1000",
"ecommerceBusinessName": "",
"dinersCardBinList": "",
"enableCustomerFeedback": "1",
"stopAfterCardReadRecords": "0",
"signoutAfterTransaction": "0",
"enableIosTransactionNotification": "0",
"enableMerchantPresentQrCode": "0",
"enableUserRoles": "0",
"showVTCardPresenceForManualEntry": "0",
"enableNotTransactedEmails": "1",
"fulfillmentOrderProcess": "MANUAL",
"disablePaperReceipt": "0",
"enableCalculatorApp": "1",
"newSettlementPath": "",
"enableDebitCards": "0",
"enableNewOrderSoundNotification": "0",
"enableCampaignCreation": "0",
"defaultTaxRate": "0",
"enableWeeklyInsightsEmails": "1",
"mastercardBankFundedIndicator": "1",
"payLinksDomainSslErrorText": "",
"localeLanguage": "en-us",
"firstTaxRate": "0",
"forceSettlementOne": "0",
"godaddyCustomerId": "",
"paymentTimeoutInSecs": "-1",
"decisionFulfill": "",
"existingBasic": "0",
"disableNonRefRefunds": "0",
"enableCustomPaymentsImage": "1",
"mastercardCardBinList": "",
"presentBundleDiscount": "0",
"enableAuthOnly": "1",
"enableSiftTracking": "0",
"showOnlyCityAndStateOnReceipts": "0",
"enableFulfillmentChangedBy": "",
"maestroCommercialCode": "",
"useVTForManualEntry": "1",
"disableEMVCTDebit": "0",
"debitAID2": "",
"debitCardsChanged": "0",
"debitAID3": "",
"enableInventory": "0",
"debitAID1": "A0000000042203,A0000000980840,A0000001524010,A0000006200620,A000000333010108",
"taxDisplayOrder": "",
"newAdvanced": "0",
"payLinksDomainDnsErrorText": "",
"enableCustomerPresentQrCode": "0",
"godaddyServiceId": "",
"salesAgentName": "",
"visaCommercialCode": "",
"referralUrlId": "",
"amexBankFundedIndicator": "0",
"enableHqSettlement": "0",
"enableEBTCashCashback": "0",
"enableAdvancedCatalog": "0",
"lodgingTaxRate": "0",
"closingTime": "",
"customContactEmail": "",
"seenTransitionMessage": "0",
"feeValueFixed": "0",
"autoInventoryUpdate": "0",
"fraudServiceType": "",
"autoSelectCommonDebitAID": "1",
"newBasic": "0",
"enableHqAdjustments": "0",
"enableManualEntryVisa": "1",
"terminalRequireInvoiceId": "0",
"autoForwardTipScreen": "0",
"enableBalanceInquiry": "0",
"maestroCardBinList": "",
"enableMastercard": "1",
"jcbCommercialCode": "",
"enablePoyntSettlementApp": "1",
"customFooterText": "",
"operatingHours": "",
"enableManualEntryUnionPay": "1",
"enableRefund": "1",
"roleAccountingPermissions": "0,1,11,12,17,34",
"validatePANForManualEntry": "0",
"enableADA": "0",
"preventApiAttributesChange": "0",
"secondScreenLanguages": "",
"secondTaxAmount": "0",
"enableDiscover": "1",
"enableReportEmails": "1",
"existingAdvanced": "0",
"socialYelp": "",
"supportedCustomFunding": "",
"parentMid": "",
"enableAlphanumericZip": "0",
"enableAccounting": "0",
"salesManagerId": "",
"enablePushLowBatteryNotification": "1",
"enableLocalVt": "0",
"enabledAppTutorial": "",
"requireCardholderConfirmation": "1",
"excludeTaxesFromTip": "0",
"socialFacebook": "",
"enableAddCard": "0",
"blockPoyntCollect": "0",
"showBankFundedOnSettlement": "0",
"alacarteLoyaltyTrialUsed": "0",
"amexCommercialCode": "",
"payfacCaseId": "",
"enableManualCVV": "1",
"defaultPayLinkId": "",
"enableCustomTaxAmount": "0",
"enableConvenienceFee": "0",
"enableEmployeeSettlementView": "0",
"disableEBTFSRefund": "0",
"enableDiners": "1",
"enableNewSettlementApp": "0",
"acceptedOfflineAgreement": "0",
"payLinksDomainDnsStatus": "",
"reportingContactEmail": "",
"enablePoyntHQApp": "1",
"voucherAID1": "",
"enablePaymentChangedBy": "",
"hideCustomerPaymentIcons": "1",
"enableNavbar": "1",
"smartTipAmount2": "200",
"unionpayCommercialCode": "",
"smartTipAmount3": "300",
"twiyoContactEmail": "",
"webOnboarded": "0",
"decisionEnableProducts": "",
"enableOther": "0",
"alwaysShowAddCard": "0",
"disableWaitForCardRemoval": "0",
"enableEBTCreditBalInq": "0",
"smartTipAmount1": "100",
"godaddyServiceType": "",
"enableFirstTax": "0",
"enableManualEntryNRR": "0",
"disableCustomContactEmail": "0",
"autoForwardReceiptScreen": "0",
"enableAmex": "1",
"enableAutomaticReports": "0",
"enableTerminalTaxEditing": "0",
"discoverSENumber": "",
"enableGDPHQ": "0",
"merchantBackgroundImageUrl": "",
"amexCardBinList": "",
"enableNonADASounds": "0",
"alacarteInvoicingTrialUsed": "0",
"disableCCRefund": "0",
"alwaysSkipSignature": "0",
"enableEBTCashPurchase": "0",
"alacarteQuickbooksTrialUsed": "0",
"enableSecondTax": "0",
"socialWebsite": "",
"payLinksDomainSslErrorCode": "",
"customerScreenMessage": "",
"showDebitCardBlockedModal": "0",
"disableEBTForceRefund": "0",
"visaCardBinList": "",
"enableIosReportNotification": "1",
"enableWebAdjustments": "0",
"salesManagerEmail": "",
"visaSENumber": "",
"enableFulfillmentChangeReason": "",
"tipAmount1": "100",
"receiptScreenTimeoutInMilliSecs": "10000",
"tipAmount2": "200",
"eCheckStoreProcessor": "1",
"verifySignature": "0",
"viewScope": "TERMINAL",
"ecommerceCheckoutButtonColor": "",
"automaticSettlementTime": "",
"secondTaxLabel": "Tax 2",
"enableInvoicesWithCof": "0",
"enableManualEntryTerminalApp": "1",
"disableOrderInbox": "0",
"enableOrderTaxForTxn": "0",
"customContactPhone": "",
"signoutAfterSuspend": "0",
"tutorialsSkipped": "0",
"enableAddCardAuthOnly": "0",
"payfacCampaignId": "",
"enableNewHQ": "0",
"payLinksDomainAttachErrorText": "",
"creditAID3": "",
"disableCardOnFile": "0",
"creditAID4": "",
"creditAID5": "",
"tipPercent3": "20",
"tipPercent2": "18",
"tipPercent1": "15",
"useNewHQ": "1",
"paymentProcessor": "co.poynt.services",
"riskStatusChangeReason": "",
"tipBeforeCardRead": "1",
"godaddyShopperId": "",
"enableManualZipStreet": "1",
"showPoyntCollect": "0",
"creditAID1": "",
"creditAID2": "",
"disableEMVCLForCashback": "0",
"purchaseAction": "AUTHORIZE",
"npsWasShown": "0",
"showDCCRebateOnSettlement": "0",
"tipAmount3": "300",
"skipCVMAmount": "2500",
"enableOfflineFeature": "0",
"payfacApplicationId": "",
"dinersBankFundedIndicator": "0",
"payfacProfileId": "",
"enableNotSettledEmails": "1",
"payfacAgentId": "",
"secondTaxRate": "0",
"enableInsertCardAPI": "0",
"disableEMVCLDebit": "0",
"appInvoiceFailedPaymentReminder": "0",
"showAddressOnReceipts": "1",
"enableIosTransactionVolumeYDay": "1",
"enablePaidScanner": "0",
"enableCash": "1",
"disableLCDR": "0",
"freeTrialSubscriptionId": "5b9c24a1-28ac-4142-8770-423ff37b046f",
"grantAppPermissions": "undefined",
"smartTipPercent3": "20",
"enableRefundChangeReason": "",
"amexSENumber": "",
"cashbackAmountLimit": "30000",
"enableImmediatePrintOrderReceipt": "0",
"showCustomTipByDefault": "0",
"thirdTaxLabel": "Tax 3",
"enableNewRegisterAppFlow": "0",
"enableJcb": "1",
"preferredStoreId": "",
"customerBackgroundImageUrl": "",
"enableHqVirtualTerminal": "0",
"skipCVM": "0",
"godaddyProjectId": "",
"fundomateLoanFunded": "0",
"enableManualEntryJcb": "1",
"processorStatus": "",
"ecommerceCheckoutLogoUrl": "",
"feeCalculation": "",
"maxOfflineTransactionValue": "10000",
"showDebitCardSuspendModal": "0",
"enableFulfillmentType": "",
"preinstallApks": "",
"enableAgeVerification": "0",
"disallowEditing": "0",
"enableManualZip": "1",
"salesAgentId": "",
"alacarteVirtualTerminalTrialUsed": "0",
"cardPreferenceType": "",
"roleMarketingPermissions": "7,8,9,29,30,43",
"bankName": "",
"customScreenOffTimeoutInSecs": "0",
"disableCCForce": "0",
"maxOfflineTotal": "1000000",
"enablePoyntTransactionsApp": "1",
"enableNewOrderNotification": "0",
"payLinksDomain": "",
"smartTipType": "A",
"unionpayCardBinList": "",
"automaticReportsTime": "0000",
"supportContactEmail": "",
"enableEBTFoodStamp": "0",
"hideCustomerName": "0",
"enableBaseAmountAdjust": "0",
"merchantContactLastName": "Sarma",
"smartTipPercent2": "18",
"hideCustomerWelcomeMessage": "0",
"smartTipPercent1": "15",
"showPoyntCapital": "0",
"enableInvoices": "0",
"disableOrderAutoClose": "0",
"lodgingEnableFolio": "0",
"enableEBTCashOnly": "0",
"jcbBankFundedIndicator": "0",
"riskStatusChangedBy": "",
"enablePoyntLodgingApp": "0",
"excludeTaxesFromCashback": "0"
},
"processorData": {},
"phone": {
"createdAt": "2021-01-08T01:51:13Z",
"updatedAt": "2023-01-24T05:04:28Z",
"id": 1348711,
"status": "ADDED",
"type": "BUSINESS",
"ituCountryCode": "1",
"areaCode": "653",
"localPhoneNumber": "6889630"
},
"acquirer": "CHASE_PAYMENTECH",
"processor": "CREDITCALL",
"status": "ACTIVE",
"externalStoreId": "a07u39smq0",
"displayName": "Reema Chai Cafe 504",
"currency": "USD",
"timezone": "America/Los_Angeles",
"id": "0ac7c74b-d88e-4bd8-8d6b-ddf8db6ec700"
}
Get store by id
Get a store by businessId and storeId.
Arguments
businessId
path- string (required)
storeId
path- string (required)
includeField
query- Set (optional)
Response
Returns a Store.
Definition
GET /businesses
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.getBusinessByDeviceId = function getBusinessByDeviceId(options, next) { ... }
module/**
* Gets a business by device ID.
* @param {String} options.deviceId
* @return {Business} business
*/
Sample Request
.getBusinessByDeviceId({
poyntdeviceId: 'urn:tid:48c54303-6d51-39af-bdeb-4af53f621652'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"acquirer": "ELAVON",
"activeSince": "1970-01-01T00:00:00Z",
"address": {
"city": "Palo Alto",
"countryCode": "USA",
"createdAt": "2017-01-04T22:04:33Z",
"id": 48119,
"line1": "4151 Middlefield Road",
"line2": "Level 2",
"postalCode": "94303",
"status": "ADDED",
"territory": "California",
"territoryType": "STATE",
"type": "BUSINESS",
"updatedAt": "2017-08-25T01:05:33Z"
,
}"attributes": { ... },
"businessUrl": "http://lawrence-s-last-straw.com",
"createdAt": "2017-01-04T22:04:34Z",
"description": "Just another test merchant",
"doingBusinessAs": "Lawrence's Last Straw",
"emailAddress": "lawrence-s-last-straw@gmail.com",
"externalMerchantId": "lawrence's0226",
"id": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"industryType": "Restaurant",
"legalName": "Lawrence's Last Straw",
"mcc": "5812",
"mockProcessor": false,
"phone": {
"areaCode": "575",
"createdAt": "2017-01-04T22:04:33Z",
"id": 50700,
"ituCountryCode": "1",
"localPhoneNumber": "2040375",
"status": "ADDED",
"type": "BUSINESS",
"updatedAt": "2017-08-25T01:05:33Z"
,
}"processor": "ELAVON",
"sic": "5812",
"status": "ACTIVATED",
"stores": [
{"acquirer": "ELAVON",
"address": {
"city": "Palo Alto",
"countryCode": "USA",
"createdAt": "2017-01-04T22:04:33Z",
"id": 48120,
"line1": "10617 Business Drive",
"line2": "Suite 424",
"postalCode": "94301",
"status": "ADDED",
"territory": "California",
"territoryType": "STATE",
"type": "BUSINESS",
"updatedAt": "2017-08-16T02:59:42Z"
,
}"attributes": { ... },
"currency": "USD",
"displayName": "Lawrence's Last Straw 488",
"externalStoreId": "161779901711",
"fixedLocation": true,
"id": "c394627f-4f68-47fb-90a5-684ea801a352",
"latitude": 37.4457,
"longitude": -122.162,
"mockProcessor": false,
"phone": {
"areaCode": "575",
"createdAt": "2017-01-04T22:04:33Z",
"id": 50701,
"ituCountryCode": "1",
"localPhoneNumber": "2040375",
"status": "ADDED",
"type": "BUSINESS",
"updatedAt": "2017-08-16T02:59:42Z"
,
}"processor": "ELAVON",
"processorData": {},
"status": "ACTIVE",
"storeDevices": [
{"businessAgreements": {
"EULA": {
"acceptedAt": "2017-07-25T01:36:14Z",
"current": true,
"type": "EULA",
"userId": 5247838,
"version": "1f",
"versionOutdated": false
},
}"catalogId": "9deca670-8761-4133-b1a9-b05b9fb01cb6",
"createdAt": "2017-01-04T22:10:00Z",
"deviceId": "urn:tid:48c54303-6d51-39af-bdeb-4af53f621652",
"externalTerminalId": "8024696638530",
"name": "LAWRENCE'S LAST STRAW DON'T MESS",
"publicKey": "-----BEGIN PUBLIC KEY-----\nMIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAyl+Sa2C1E6xcv9d9Mm9N\ngtzDtSsjWd9k7PZPDLy3JRq3dhGU9rQtopfEUrtqRUDRS7xpP3842enns39Z427+\nwOuAq7aqVLHzCIio8+D0m21mmsKpE8rSH54dCKciSypuj/MF4o+jIMA43+ov6dba\nN/6WFH/+c4MzTqz673XldP9RAvrK8k55lKoZW5bvX5Bx0xmFbtey17Uyby+y6kT+\nTyQArYwzEFBA2RQ+LKf/XwIgoh6RcdMVlNahe5LNW2rl5FQGm+HxnvYG6ZQGQ4oX\nFEGClsvOQabdjVXNNA6rVmJeeKmSekVNFjA3ZqEcmq8E6ijmT/H1upmiZ5fI0Eag\nSwIDAQAB\n-----END PUBLIC KEY-----\n",
"serialNumber": "P61SWA231FS000416",
"status": "ACTIVATED",
"storeId": "c394627f-4f68-47fb-90a5-684ea801a352",
"type": "TERMINAL",
"updatedAt": "2017-08-16T04:26:19Z"
},
]"timezone": "America/Los_Angeles"
},
]"timezone": "America/Los_Angeles",
"type": "TEST_MERCHANT",
"updatedAt": "2017-08-25T01:05:33Z"
}
Definition
@classmethod
def get_business_by_device_id(cls, device_id):
"""
Get a business by a device id.
Arguments:
device_id (str): the device ID to get a business for
"""
Sample Request
= poynt.Business.get_business_by_device_id(
doc, status_code 'urn:tid:48c54303-6d51-39af-bdeb-4af53f621652'
)
if status_code < 300:
print(doc)
Sample Response
u'stores': [{u'status': u'ACTIVE', u'processorData': {}, u'externalStoreId': u'161779901711', u'displayName': u"Lawrence's Last Straw 488", u'currency': u'USD', u'mockProcessor': False, u'longitude': -122.162, u'id': u'c394627f-4f68-47fb-90a5-684ea801a352', u'storeDevices': [{u'status': u'ACTIVATED', u'storeId': u'c394627f-4f68-47fb-90a5-684ea801a352', u'name': u"LAWRENCE'S LAST STRAW DON'T MESS", u'serialNumber': u'P61SWA231FS000416', u'businessAgreements': {u'EULA': {u'userId': 5247838, u'versionOutdated': False, u'current': True, u'version': u'1f', u'acceptedAt': u'2017-07-25T01:36:14Z', u'type': u'EULA'}}, u'externalTerminalId': u'8024696638530', u'publicKey': u'-----BEGIN PUBLIC KEY-----\nMIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAyl+Sa2C1E6xcv9d9Mm9N\ngtzDtSsjWd9k7PZPDLy3JRq3dhGU9rQtopfEUrtqRUDRS7xpP3842enns39Z427+\nwOuAq7aqVLHzCIio8+D0m21mmsKpE8rSH54dCKciSypuj/MF4o+jIMA43+ov6dba\nN/6WFH/+c4MzTqz673XldP9RAvrK8k55lKoZW5bvX5Bx0xmFbtey17Uyby+y6kT+\nTyQArYwzEFBA2RQ+LKf/XwIgoh6RcdMVlNahe5LNW2rl5FQGm+HxnvYG6ZQGQ4oX\nFEGClsvOQabdjVXNNA6rVmJeeKmSekVNFjA3ZqEcmq8E6ijmT/H1upmiZ5fI0Eag\nSwIDAQAB\n-----END PUBLIC KEY-----\n', u'deviceId': u'urn:tid:48c54303-6d51-39af-bdeb-4af53f621652', u'updatedAt': u'2017-08-16T04:26:19Z', u'catalogId': u'9deca670-8761-4133-b1a9-b05b9fb01cb6', u'type': u'TERMINAL', u'createdAt': u'2017-01-04T22:10:00Z'}], u'phone': {u'status': u'ADDED', u'areaCode': u'575', u'localPhoneNumber': u'2040375', u'updatedAt': u'2017-08-16T02:59:42Z', u'ituCountryCode': u'1', u'type': u'BUSINESS', u'id': 50701, u'createdAt': u'2017-01-04T22:04:33Z'}, u'timezone': u'America/Los_Angeles', u'acquirer': u'ELAVON', u'address': {u'status': u'ADDED', u'city': u'Palo Alto', u'countryCode': u'USA', u'line2': u'Suite 424', u'line1': u'10617 Business Drive', u'territoryType': u'STATE', u'updatedAt': u'2017-08-16T02:59:42Z', u'postalCode': u'94301', u'territory': u'California', u'type': u'BUSINESS', u'id': 48120, u'createdAt': u'2017-01-04T22:04:33Z'}, u'latitude': 37.4457, u'attributes': {}, u'processor': u'ELAVON', u'fixedLocation': True}], u'businessUrl': u'http://lawrence-s-last-straw.com', u'updatedAt': u'2017-08-25T01:05:33Z', u'timezone': u'America/Los_Angeles', u'id': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'industryType': u'Restaurant', u'sic': u'5812', u'acquirer': u'ELAVON', u'type': u'TEST_MERCHANT', u'status': u'ACTIVATED', u'description': u'Just another test merchant', u'mockProcessor': False, u'mcc': u'5812', u'activeSince': u'1970-01-01T00:00:00Z', u'phone': {u'status': u'ADDED', u'areaCode': u'575', u'localPhoneNumber': u'2040375', u'updatedAt': u'2017-08-25T01:05:33Z', u'ituCountryCode': u'1', u'type': u'BUSINESS', u'id': 50700, u'createdAt': u'2017-01-04T22:04:33Z'}, u'emailAddress': u'lawrence-s-last-straw@gmail.com', u'address': {u'status': u'ADDED', u'city': u'Palo Alto', u'countryCode': u'USA', u'line2': u'Level 2', u'line1': u'4151 Middlefield Road', u'territoryType': u'STATE', u'updatedAt': u'2017-08-25T01:05:33Z', u'postalCode': u'94303', u'territory': u'California', u'type': u'BUSINESS', u'id': 48119, u'createdAt': u'2017-01-04T22:04:33Z'}, u'externalMerchantId': u"lawrence's0226", u'doingBusinessAs': u"Lawrence's Last Straw", u'createdAt': u'2017-01-04T22:04:34Z', u'legalName': u"Lawrence's Last Straw", u'attributes': {}, u'processor': u'ELAVON'} {
Get by device Id
Get a business by a device id.
Arguments
storeDeviceId
query- string (required)
Response
Returns a Business.
Business Users
Operations for owners and employees of a business
Definition
GET /businesses/{businessId}/businessUsers
Sample Request
curl --location --request GET 'https://services.poynt.net/businesses/a3f24e9e-6764-42f2-bbcd-5541e7f3be38/businessUsers' \
--header 'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json'
Sample Response
[
{
"status": "EMPLOYED",
"employmentDetails": {
"role": "OWNER",
"startAt": 1649894941,
"endAt": 0
},
"credentials": [
{
"publicCredentialType": "USERNAME",
"id": 592678,
"publicCredentialValue": "Reema"
}
],
"userId": 369299726,
"firstName": "Reema",
"lastName": "",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38"
},
{
"status": "EMPLOYED",
"employmentDetails": {
"role": "MANAGER",
"startAt": 0,
"endAt": 0
},
"credentials": [
{
"publicCredentialType": "USERNAME",
"id": 692777,
"publicCredentialValue": "Shiny"
}
],
"userId": 402258359,
"firstName": "Kastureema",
"lastName": "Sarma",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38"
}
]
Definition
.exports.getBusinessUsers = function getBusinessUsers(options, next) { ... }
module/**
* Get all users at a business.
* @param {String} options.businessId
* @return {BusinessUser[]} businessusers
*/
Sample Request
.getBusinessUsers({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
[
{"status": "EMPLOYED",
"employmentDetails": {
"role": "OWNER",
"startAt": 1483568424,
"endAt": 0
,
}"credentials": [
{"publicCredentialType": "USERNAME",
"id": 33250,
"publicCredentialValue": "law"
},
]"userId": 5247838,
"firstName": "law",
"lastName": "",
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb"
,
}
{"status": "EMPLOYED",
"employmentDetails": {
"role": "EMPLOYEE",
"startAt": 0,
"endAt": 0
,
}"credentials": [
{"publicCredentialType": "USERNAME",
"id": 33266,
"publicCredentialValue": "sample"
},
]"userId": 5247869,
"firstName": "Sample",
"lastName": "Person",
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb"
,
}
{"status": "EMPLOYED",
"employmentDetails": {
"role": "EMPLOYEE",
"startAt": 0,
"endAt": 0
,
}"credentials": [
{"publicCredentialType": "USERNAME",
"id": 33267,
"publicCredentialValue": "Test"
},
]"userId": 5247870,
"firstName": "Test",
"lastName": "Person",
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb"
,
}
{"status": "EMPLOYED",
"employmentDetails": {
"role": "OWNER",
"startAt": 1487789772,
"endAt": 0
,
}"credentials": [
{"publicCredentialType": "USERNAME",
"id": 35355,
"publicCredentialValue": "yan"
},
]"userId": 5253478,
"firstName": "yan",
"lastName": "",
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb"
} ]
Definition
@classmethod
def get_business_users(cls, business_id):
"""
Get all users at a business.
Arguments:
business_id (str): the business ID
"""
Sample Request
= poynt.BusinessUser.get_business_users(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb'
)
if status_code < 300:
print(doc)
Sample Response
u'status': u'EMPLOYED', u'firstName': u'law', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'lastName': u'', u'userId': 5247838, u'employmentDetails': {u'startAt': 1483568424, u'role': u'OWNER', u'endAt': 0}, u'credentials': [{u'publicCredentialType': u'USERNAME', u'publicCredentialValue': u'law', u'id': 33250}]}, {u'status': u'EMPLOYED', u'firstName': u'Sample', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'lastName': u'Person', u'userId': 5247869, u'employmentDetails': {u'startAt': 0, u'role': u'EMPLOYEE', u'endAt': 0}, u'credentials': [{u'publicCredentialType': u'USERNAME', u'publicCredentialValue': u'sample', u'id': 33266}]}, {u'status': u'EMPLOYED', u'firstName': u'Test', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'lastName': u'Person', u'userId': 5247870, u'employmentDetails': {u'startAt': 0, u'role': u'EMPLOYEE', u'endAt': 0}, u'credentials': [{u'publicCredentialType': u'USERNAME', u'publicCredentialValue': u'Test', u'id': 33267}]}, {u'status': u'EMPLOYED', u'firstName': u'yan', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'lastName': u'', u'userId': 5253478, u'employmentDetails': {u'startAt': 1487789772, u'role': u'OWNER', u'endAt': 0}, u'credentials': [{u'publicCredentialType': u'USERNAME', u'publicCredentialValue': u'yan', u'id': 35355}]}] [{
Get All Users
Get all users at a business.
Arguments
businessId
path- string (required)
api-version
header- string (optional)
Response
Returns a array.
Definition
GET /businesses/{businessId}/businessUsers/{businessUserId}
Sample Request
curl --location --request GET 'https://services.poynt.net/businesses/a3f24e9e-6764-42f2-bbcd-5541e7f3be38/businessUsers/369299726' \
--header 'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json'
Sample Response
{
"status": "EMPLOYED",
"employmentDetails": {
"role": "OWNER",
"startAt": 1649894941,
"endAt": 0
},
"credentials": [
{
"publicCredentialType": "USERNAME",
"id": 592678,
"publicCredentialValue": "Reema"
}
],
"userId": 369299726,
"firstName": "Reema",
"lastName": "",
"businessId": "a3f24e9e-6764-42f2-bbcd-5541e7f3be38"
}
Definition
.exports.getBusinessUser = function getBusinessUser(options, next) { ... }
module/**
* Get a single user at a business.
* @param {String} options.businessId
* @param {String} options.businessUserId
* @return {BusinessUser} businessuser
*/
Sample Request
.getBusinessUser({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
businessUserId : 5247838
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"credentials": [
{"id": 33250,
"publicCredentialType": "USERNAME",
"publicCredentialValue": "law"
},
]"employmentDetails": {
"endAt": 0,
"role": "OWNER",
"startAt": 1483568424
,
}"firstName": "law",
"lastName": "",
"status": "EMPLOYED",
"userId": 5247838
}
Definition
@classmethod
def get_business_user(cls, business_id, business_user_id):
"""
Get a single user at a business.
Arguments:
business_id (str): the business ID
business_user_id (str): the user ID
"""
Sample Request
= poynt.BusinessUser.get_business_user(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
5247838
)
if status_code < 300:
print(doc)
Sample Response
u'status': u'EMPLOYED', u'firstName': u'law', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'lastName': u'', u'userId': 5247838, u'employmentDetails': {u'startAt': 1483568424, u'role': u'OWNER', u'endAt': 0}, u'credentials': [{u'publicCredentialType': u'USERNAME', u'publicCredentialValue': u'law', u'id': 33250}]} {
Get User
Get a user at a business.
Arguments
businessId
path- string (required)
businessUserId
path- integer (required)
api-version
header- string (optional)
Response
Returns a BusinessUser.
Inventory
Operations for inventory
Definition
GET /businesses/{businessId}/inventory
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
: Add definition TODO
Sample Request
: Add request TODO
Sample Response
: Add response TODO
Definition
TODO: Add definition
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Get Inventory Summary List
Get a list of inventory summary.
Arguments
If-Modified-Since
header- string (optional)
startAt
query- string (optional)
startOffset
query- integer (optional)
endAt
query- string (optional)
limit
query- integer (optional)
storeId
query- string (optional)
productId
query- string (optional)
sku
query- string (optional)
businessId
path- string (required)
Response
Returns a InventorySummaryList.
Definition
PUT /businesses/{businessId}/products/{productId}/inventory
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
: Add definition TODO
Sample Request
: Add request TODO
Sample Response
: Add response TODO
Definition
TODO: Add definition
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Add Inventory
Add a new inventory at a store.
Arguments
businessId
path- string (required)
productId
path- string (required)
inventory
body- Inventory (optional)
Response
Returns a Inventory.
Definition
GET /businesses/{businessId}/products/{productId}/inventory/{storeId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
: Add definition TODO
Sample Request
: Add request TODO
Sample Response
: Add response TODO
Definition
TODO: Add definition
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Get Inventory
Get an inventory at a store.
Arguments
businessId
path- string (required)
productId
path- string (required)
storeId
path- string (required)
Response
Returns a Inventory.
Definition
POST /businesses/{businessId}/products/{productId}/inventory/{storeId}/decrement
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
: Add definition TODO
Sample Request
: Add request TODO
Sample Response
: Add response TODO
Definition
TODO: Add definition
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Decrement inventory
Decrement an inventory.
Arguments
businessId
path- string (required)
productId
path- string (required)
storeId
path- string (required)
delta
query- number (required)
Response
Returns a Inventory.
Definition
POST /businesses/{businessId}/products/{productId}/inventory/{storeId}/increment
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
: Add definition TODO
Sample Request
: Add request TODO
Sample Response
: Add response TODO
Definition
TODO: Add definition
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Increment inventory
Increment an inventory.
Arguments
businessId
path- string (required)
productId
path- string (required)
storeId
path- string (required)
delta
query- number (required)
Response
Returns a Inventory.
Definition
PUT /businesses/{businessId}/products/{productId}/variants/{sku}/inventory
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
: Add definition TODO
Sample Request
: Add request TODO
Sample Response
: Add response TODO
Definition
TODO: Add definition
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Add Variant Inventory
Add a new variant inventory at a store.
Arguments
businessId
path- string (required)
productId
path- string (required)
sku
path- string (required)
inventory
body- Inventory (optional)
Response
Returns a Inventory.
Definition
GET /businesses/{businessId}/products/{productId}/variants/{sku}/inventory/{storeId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
: Add definition TODO
Sample Request
: Add request TODO
Sample Response
: Add response TODO
Definition
TODO: Add definition
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Get Variant Inventory
Get a variant inventory at a store.
Arguments
businessId
path- string (required)
productId
path- string (required)
sku
path- string (required)
storeId
path- string (required)
Response
Returns a Inventory.
Definition
POST /businesses/{businessId}/products/{productId}/variants/{sku}/inventory/{storeId}/decrement
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
: Add definition TODO
Sample Request
: Add request TODO
Sample Response
: Add response TODO
Definition
TODO: Add definition
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Decrement variant inventory
Decrement a variant inventory.
Arguments
businessId
path- string (required)
productId
path- string (required)
sku
path- string (required)
storeId
path- string (required)
delta
query- number (required)
Response
Returns a Inventory.
Definition
POST /businesses/{businessId}/products/{productId}/variants/{sku}/inventory/{storeId}/increment
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
: Add definition TODO
Sample Request
: Add request TODO
Sample Response
: Add response TODO
Definition
TODO: Add definition
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Increment variant inventory
Increment a variant inventory.
Arguments
businessId
path- string (required)
productId
path- string (required)
sku
path- string (required)
storeId
path- string (required)
delta
query- number (required)
Response
Returns a Inventory.
Products
Products are what a merchant sell at their business. Products have a
name
, shortCode
, sku
, and
price
. sku
should be unique for tracking
inventory purpose. shortCode
should be unique and
represents an easily remembered identifier for the business user.
Definition
GET /businesses/{businessId}/products
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.getProducts = function getProducts(options, next) { ... }
module/**
* Get a list of products
* @param {String} options.businessId
* @param {String} options.startAt (optional)
* @param {Integer} options.startOffset (optional)
* @param {String} options.endAt (optional)
* @param {Integer} options.limit (optional)
* @return {ProductList} products
*/
Sample Request
.getProducts({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
startOffset : 0,
limit : 1
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"links": [
{"href": "/businesses/18f071cc-5ed4-4b33-80c1-305056d42bfb/products?startAt=2017-01-04T22%3A04%3A34Z&endAt=2017-08-28T07%3A23%3A30Z&startOffset=1&limit=1",
"method": "GET",
"rel": "next"
},
]"products": [
{"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-01-04T22:04:34Z",
"description": "Cappuccino",
"id": "080aae58-93fe-4754-93f4-8fbf9420b49d",
"name": "Cappuccino",
"price": {
"amount": 350,
"currency": "USD"
,
}"shortCode": "Cappu",
"sku": "082011500008",
"status": "ACTIVE",
"type": "SIMPLE",
"updatedAt": "2017-01-04T22:04:34Z"
}
] }
Definition
@classmethod
def get_products(cls, business_id, start_at=None, start_offset=None,
=None, limit=None):
end_at"""
Get a list of products at a business.
Arguments:
business_id (str): the business ID
Keyword arguments:
start_at (int, optional): get products created after this time in seconds
start_offset (int, optional): the numeric offset to start the list (for pagination)
end_at (int, optional): get products created before this time in seconds
limit (int, optional): how many products to return (for pagination)
"""
Sample Request
= poynt.Product.get_products(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
=0,
start_offset=1
limit
)
if status_code < 300:
print(doc)
Sample Response
u'products': [{u'status': u'ACTIVE', u'sku': u'082011500008', u'name': u'Cappuccino', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'type': u'SIMPLE', u'price': {u'currency': u'USD', u'amount': 350}, u'updatedAt': u'2017-01-04T22:04:34Z', u'shortCode': u'Cappu', u'id': u'080aae58-93fe-4754-93f4-8fbf9420b49d', u'createdAt': u'2017-01-04T22:04:34Z', u'description': u'Cappuccino'}], u'links': [{u'href': u'/businesses/18f071cc-5ed4-4b33-80c1-305056d42bfb/products?startAt=2017-01-04T22%3A04%3A34Z&endAt=2017-08-28T07%3A24%3A08Z&startOffset=1&limit=1', u'method': u'GET', u'rel': u'next'}]} {
Get List
Get all product since time. Result is paginated
Arguments
If-Modified-Since
header- string (optional)
startAt
query- string (optional)
startOffset
query- integer (optional)
endAt
query- string (optional)
limit
query- integer (optional)
businessId
path- string (required)
Response
Returns a ProductList.
Definition
POST /businesses/{businessId}/products
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.createProduct = function createProduct(options, product, next) { ... }
module/**
* Creates a product on a business.
* @param {String} options.businessId
* @param {Product} product
* @return {Product} product
*/
Sample Request
.createProduct({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb'
, {
}price: {
currency: 'USD',
amount: 500
,
}status: 'ACTIVE',
shortCode: 'FOO',
sku: 'FOO',
name: 'Foo Product'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-08-28T07:26:29Z",
"id": "e989e7eb-5c59-4c4d-9a80-006147f0740f",
"name": "Foo Product",
"price": {
"amount": 500,
"currency": "USD"
,
}"shortCode": "FOO",
"sku": "FOO",
"status": "ACTIVE",
"type": "SIMPLE",
"updatedAt": "2017-08-28T07:26:29Z"
}
Definition
@classmethod
def create_product(cls, business_id, product):
"""
Creates a product on a business.
Arguments:
business_id (str): the business ID
product (dict): the full product object
"""
Sample Request
= poynt.Product.create_product(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
{'price': {
'currency': 'USD',
'amount': 500
},'status': 'ACTIVE',
'shortCode': 'FOO',
'sku': 'FOO',
'name': 'Foo Product'
}
)
if status_code < 300:
print(doc)
Sample Response
u'status': u'ACTIVE', u'sku': u'FOO', u'name': u'Foo Product', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'type': u'SIMPLE', u'price': {u'currency': u'USD', u'amount': 500}, u'updatedAt': u'2017-08-28T07:25:23Z', u'shortCode': u'FOO', u'id': u'd92cc4d6-0298-47cf-9537-b45b1870454c', u'createdAt': u'2017-08-28T07:25:23Z'} {
Create
Create a product definition for a business.
Arguments
businessId
path- string (required)
product
body- Product (optional)
Response
Returns a Product.
Definition
GET /businesses/{businessId}/products/lookup
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.lookupProducts = function lookupProducts(options, next) { ... }
module/**
* Get a list of products by ID.
* @param {String} options.businessId
* @param {String[]} options.ids
* @return {ProductList} products
*/
Sample Request
.lookupProducts({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
ids : ['e989e7eb-5c59-4c4d-9a80-006147f0740f']
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
[
{"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-08-28T07:26:29Z",
"id": "e989e7eb-5c59-4c4d-9a80-006147f0740f",
"name": "Foo Product",
"price": {
"amount": 500,
"currency": "USD"
,
}"shortCode": "FOO",
"sku": "FOO",
"status": "ACTIVE",
"type": "SIMPLE",
"updatedAt": "2017-08-28T07:26:29Z"
} ]
Definition
@classmethod
def lookup_products(cls, business_id, product_ids):
"""
Get a list of products by ID.
Arguments:
business_id (str): the business ID
product_ids (list of str): a list of product ids
"""
Sample Request
= poynt.Product.lookup_products(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'e989e7eb-5c59-4c4d-9a80-006147f0740f']
[
)
if status_code < 300:
print(doc)
Sample Response
u'products': [{u'status': u'ACTIVE', u'sku': u'FOO', u'name': u'Foo Product', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'type': u'SIMPLE', u'price': {u'currency': u'USD', u'amount': 500}, u'updatedAt': u'2017-08-28T07:26:29Z', u'shortCode': u'FOO', u'id': u'e989e7eb-5c59-4c4d-9a80-006147f0740f', u'createdAt': u'2017-08-28T07:26:29Z'}]} {
Get Multiple Id
Get multiple products by ids.
Arguments
businessId
path- string (required)
ids
query- string (required)
Response
Returns a ProductList.
Definition
GET /businesses/{businessId}/products/summary
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.getProductsSummary = function getProductsSummary(options, next) { ... }
module/**
* Get a list of summaries at a business. Product summaries contain product
* shortCode, price, businessId, name, and id.
* @param {String} options.businessId
* @param {String} options.startAt (optional)
* @param {Integer} options.startOffset (optional)
* @param {String} options.endAt (optional)
* @param {Integer} options.limit (optional)
* @return {ProductList} products
*/
Sample Request
.getProductsSummary({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
startOffset : 0,
limit : 1
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"links": [
{"href": "/businesses/18f071cc-5ed4-4b33-80c1-305056d42bfb/products/summary?startAt=2017-01-04T22%3A04%3A34Z&endAt=2017-08-28T07%3A30%3A34Z&startOffset=1&limit=1",
"method": "GET",
"rel": "next"
},
]"products": [
{"id": "080aae58-93fe-4754-93f4-8fbf9420b49d",
"name": "Cappuccino",
"price": {
"amount": 350,
"currency": "USD"
,
}"shortCode": "Cappu"
}
] }
Definition
@classmethod
def get_products_summary(cls, business_id, start_at=None, start_offset=None,
=None, limit=None):
end_at"""
Get a list of product summaries at a business. Product summaries contain
product shortCode, price, businessId, name, and id.
Arguments:
business_id (str): the business ID
Keyword arguments:
start_at (int, optional): get products created after this time in seconds
start_offset (int, optional): the numeric offset to start the list (for pagination)
end_at (int, optional): get products created before this time in seconds
limit (int, optional): how many products to return (for pagination)
"""
Sample Request
= poynt.Product.get_products_summary(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
=0,
start_offset=1
limit
)
if status_code < 300:
print(doc)
Sample Response
u'products': [{u'price': {u'currency': u'USD', u'amount': 350}, u'shortCode': u'Cappu', u'id': u'080aae58-93fe-4754-93f4-8fbf9420b49d', u'name': u'Cappuccino'}], u'links': [{u'href': u'/businesses/18f071cc-5ed4-4b33-80c1-305056d42bfb/products/summary?startAt=2017-01-04T22%3A04%3A34Z&endAt=2017-08-28T07%3A30%3A01Z&startOffset=1&limit=1', u'method': u'GET', u'rel': u'next'}]} {
Get Summary
Get all product summary. Result is paginated
Arguments
If-Modified-Since
header- string (optional)
startAt
query- string (optional)
startOffset
query- integer (optional)
endAt
query- string (optional)
limit
query- integer (optional)
businessId
path- string (required)
Response
Returns a ProductSummaryList.
Definition
DELETE /businesses/{businessId}/products/{productId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.deleteProduct = function deleteProduct(options, next) { ... }
module/**
* Deactivates a product. Deactivated products will be removed from all catalog
* references.
* @param {String} options.businessId
* @param {String} options.productId
* @return {Product} product
*/
Sample Request
.deleteProduct({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
productId : '080aae58-93fe-4754-93f4-8fbf9420b49d'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{}
Definition
@classmethod
def delete_product(cls, business_id, product_id):
"""
Deactivates a product. Deactivated products will be removed from all
catalog references.
Arguments:
business_id (str): the business ID
product_id (str): the product ID
"""
Sample Request
= poynt.Product.delete_product(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'080aae58-93fe-4754-93f4-8fbf9420b49d'
)
if status_code < 300:
print(doc)
Sample Response
None
Deactivate
Deactivate a product. Deactivated product will be removed from all catalog references.
Arguments
businessId
path- string (required)
productId
path- string (required)
Definition
GET /businesses/{businessId}/products/{productId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.getProduct = function getProduct(options, next) { ... }
module/**
* Get a single product for a business.
* @param {String} options.businessId
* @param {String} options.productId
* @return {Product} product
*/
Sample Request
.getProduct({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
productId : '0cb54922-0ef2-4509-8735-72ccb373c464'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-01-04T22:04:34Z",
"description": "Apple Pie",
"id": "0cb54922-0ef2-4509-8735-72ccb373c464",
"name": "Apple Pie",
"price": {
"amount": 300,
"currency": "USD"
,
}"shortCode": "ApPie",
"sku": "082011500001",
"status": "ACTIVE",
"type": "SIMPLE",
"updatedAt": "2017-01-04T22:04:34Z"
}
Definition
@classmethod
def get_product(cls, business_id, product_id):
"""
Get a single product for a business.
Arguments:
business_id (str): the business ID
product_id (str): the product ID
"""
Sample Request
= poynt.Product.get_product(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'0cb54922-0ef2-4509-8735-72ccb373c464'
)
if status_code < 300:
print(doc)
Sample Response
u'status': u'ACTIVE', u'sku': u'082011500001', u'name': u'Apple Pie', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'type': u'SIMPLE', u'price': {u'currency': u'USD', u'amount': 300}, u'updatedAt': u'2017-01-04T22:04:34Z', u'shortCode': u'ApPie', u'id': u'0cb54922-0ef2-4509-8735-72ccb373c464', u'createdAt': u'2017-01-04T22:04:34Z', u'description': u'Apple Pie'} {
Get By Id
Get a business product by id.
Arguments
businessId
path- string (required)
If-Modified-Since
header- string (optional)
productId
path- string (required)
Response
Returns a Product.
Definition
PATCH /businesses/{businessId}/products/{productId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.updateProduct = function updateProduct(options, productOrPatch, next) { ... }
module/**
* Updates a product by ID. Can either specify the whole product, or an array
* of JSON Patch instructions.
* @param {String} options.businessId - the business ID
* @param {String} options.productId - the product ID
* @param {Boolean} options.noRemove - don't remove any keys from old product in
* the patch. safer this way. defaults to true
* @param {Object} productOrPatch - if is an array, will treat as JSON patch;
* if object, will treat as product
* @return {Product} product
*/
Sample Request
.updateProduct({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
productId : '0cb54922-0ef2-4509-8735-72ccb373c464'
, {
}price : {
currency : 'USD',
amount : 600
}, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-01-04T22:04:34Z",
"description": "Apple Pie",
"id": "0cb54922-0ef2-4509-8735-72ccb373c464",
"name": "Apple Pie",
"price": {
"amount": 600,
"currency": "USD"
,
}"shortCode": "ApPie",
"sku": "082011500001",
"status": "ACTIVE",
"type": "SIMPLE",
"updatedAt": "2017-08-28T07:35:51Z"
}
Definition
@classmethod
def update_product(cls, business_id, product_id, product=None, patch=None, no_remove=True):
"""
Updates a product by ID. Can either specify the whole product, or an array
of JSON Patch instructions.
Arguments:
business_id (str): the business ID
product_id (str): the product ID
Keyword arguments:
product (dict): the full product object
patch (list of dict): JSON Patch update instructions
no_remove (boolean, optional): don't remove any keys from old product in the patch.
safer this way. defaults to True
"""
Sample Request
= poynt.Product.update_product(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'0cb54922-0ef2-4509-8735-72ccb373c464',
={
product'price': {
'currency': 'USD',
'amount': 600
}
}
)
if status_code < 300:
print(doc)
Sample Response
u'status': u'ACTIVE', u'sku': u'082011500001', u'name': u'Apple Pie', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'type': u'SIMPLE', u'price': {u'currency': u'USD', u'amount': 600}, u'updatedAt': u'2017-08-28T07:36:15Z', u'shortCode': u'ApPie', u'id': u'0cb54922-0ef2-4509-8735-72ccb373c464', u'createdAt': u'2017-01-04T22:04:34Z', u'description': u'Apple Pie'} {
Update By Id
Update a product by id.
Arguments
businessId
path- string (required)
productId
path- string (required)
patch
body- JsonPatch (optional)
Response
Returns a Product.
Definition
PUT /businesses/{businessId}/products/{productId}/variants
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
This method is currently not possible using the Node.js SDK.
Definition
This method is currently not possible using the Python SDK.
Upsert variant
Upsert a variant of a product, retaining its inventory, if any.
Arguments
businessId
path- string (required)
productId
path- string (required)
variant
body- Variant (optional)
Response
Returns a Variant.
Definition
GET /businesses/{businessId}/products/{productId}/variants
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
This method is currently not possible using the Node.js SDK.
Definition
This method is currently not possible using the Python SDK.
Get all variants
Get all variants of this product.
Arguments
businessId
path- string (required)
productId
path- string (required)
Response
Returns a array.
Definition
DELETE /businesses/{businessId}/products/{productId}/variants/{sku}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
This method is currently not possible using the Node.js SDK.
Definition
This method is currently not possible using the Python SDK.
Delete variant
Delete a variant of a product
Arguments
businessId
path- string (required)
productId
path- string (required)
sku
path- string (required)
Catalogs
A catalog is a complete list of products for sale by the merchant. Catalog can contain products and/or categories of products organized in a hierarchy. Catalogs, once defined, can be assigned to a store or individual Poynt Terminal.
Definition
GET /businesses/{businessId}/catalogs
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.getCatalogs = function getCatalogs(options, next) { ... }
module/**
* Get a list of catalogs
* @param {String} options.businessId
* @param {String} options.startAt (optional)
* @param {Integer} options.startOffset (optional)
* @param {String} options.endAt (optional)
* @param {Integer} options.limit (optional)
* @return {CatalogList} catalogs
*/
Sample Request
.getCatalogs({
poyntbusinessId: '18f071cc-5ed4-4b33-80c1-305056d42bfb'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"catalogs": [
{"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-08-16T04:25:59Z",
"displayMetadata": [
{"horizontalScroll": "false",
"numberOfColumns": 4,
"numberOfRows": 6,
"optimizedForPackage": "poynt-smart-terminal",
"verticalScroll": "true"
},
]"id": "9deca670-8761-4133-b1a9-b05b9fb01cb6",
"name": "Leech Catalog",
"products": [
{"displayOrder": 1,
"id": "74e9594a-4de0-40dd-a955-af2155dc10e3"
,
}
{"displayOrder": 7,
"id": "8b86b625-b209-44cb-8e3e-c6a09af4e88e"
,
}
{"displayOrder": 10,
"id": "c8bbc0f0-c7ad-4925-8a5e-1ab8eec22fcb"
,
}
{"displayOrder": 11,
"id": "909b0361-79d8-4fd9-a6c7-85391787769e"
,
}
{"displayOrder": 12,
"id": "c77ef1c5-9895-4515-9035-209d579a3af3"
,
}
{"displayOrder": 13,
"id": "54127174-2b69-4cff-ad2d-5893795a12f2"
,
}
{"displayOrder": 14,
"id": "691989f4-e610-4a3f-94b3-3b191fc68d40"
,
}
{"displayOrder": 17,
"id": "6c34a14b-8184-4da4-bb5f-c87c78bdce8e"
,
}
{"displayOrder": 18,
"id": "f77346e8-172d-43d8-9a62-c18ed9b4f787"
,
}
{"displayOrder": 23,
"id": "6eec1971-5eab-4410-b124-27e1356ea6f7"
},
]"updatedAt": "2017-08-19T02:28:14Z"
,
}
{"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-08-28T07:41:48Z",
"id": "3301546f-aa10-404c-8dd2-44894db70f56",
"name": "Foo catalog",
"updatedAt": "2017-08-28T07:41:48Z"
,
}
{"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-08-28T07:42:19Z",
"id": "ee85312d-7449-4003-8222-a22415fdfed9",
"name": "Foo catalog",
"updatedAt": "2017-08-28T07:42:19Z"
}
] }
Definition
@classmethod
def get_catalogs(cls, business_id, start_at=None, start_offset=None,
=None, limit=None):
end_at"""
Get all catalogs at a business.
Arguments:
business_id (str): the business ID
Keyword arguments:
start_at (int, optional): get catalogs created after this time in seconds
start_offset (int, optional): the numeric offset to start the list (for pagination)
end_at (int, optional): get catalogs created before this time in seconds
limit (int, optional): how many catalogs to return (for pagination)
"""
Sample Request
= poynt.Catalog.get_catalogs(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb'
)
if status_code < 300:
print(doc)
Sample Response
u'catalogs': [{u'name': u'Leech Catalog', u'displayMetadata': [{u'verticalScroll': u'true', u'numberOfColumns': 4, u'optimizedForPackage': u'poynt-smart-terminal', u'horizontalScroll': u'false', u'numberOfRows': 6}], u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'products': [{u'id': u'74e9594a-4de0-40dd-a955-af2155dc10e3', u'displayOrder': 1}, {u'id': u'8b86b625-b209-44cb-8e3e-c6a09af4e88e', u'displayOrder': 7}, {u'id': u'c8bbc0f0-c7ad-4925-8a5e-1ab8eec22fcb', u'displayOrder': 10}, {u'id': u'909b0361-79d8-4fd9-a6c7-85391787769e', u'displayOrder': 11}, {u'id': u'c77ef1c5-9895-4515-9035-209d579a3af3', u'displayOrder': 12}, {u'id': u'54127174-2b69-4cff-ad2d-5893795a12f2', u'displayOrder': 13}, {u'id': u'691989f4-e610-4a3f-94b3-3b191fc68d40', u'displayOrder': 14}, {u'id': u'6c34a14b-8184-4da4-bb5f-c87c78bdce8e', u'displayOrder': 17}, {u'id': u'f77346e8-172d-43d8-9a62-c18ed9b4f787', u'displayOrder': 18}, {u'id': u'6eec1971-5eab-4410-b124-27e1356ea6f7', u'displayOrder': 23}], u'updatedAt': u'2017-08-19T02:28:14Z', u'id': u'9deca670-8761-4133-b1a9-b05b9fb01cb6', u'createdAt': u'2017-08-16T04:25:59Z'}, {u'name': u'Foo catalog', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'id': u'3301546f-aa10-404c-8dd2-44894db70f56', u'createdAt': u'2017-08-28T07:41:48Z', u'updatedAt': u'2017-08-28T07:41:48Z'}, {u'name': u'Foo catalog', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'id': u'ee85312d-7449-4003-8222-a22415fdfed9', u'createdAt': u'2017-08-28T07:42:19Z', u'updatedAt': u'2017-08-28T07:42:19Z'}]} {
Get List
Get all catalog since time. Result is paginated
Arguments
If-Modified-Since
header- string (optional)
startAt
query- string (optional)
startOffset
query- integer (optional)
endAt
query- string (optional)
limit
query- integer (optional)
businessId
path- string (required)
Response
Returns a CatalogList.
Definition
GET /businesses/{businessId}/catalogs/{catalogId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.getCatalog = function getCatalog(options, next) { ... }
module/**
* Get a single catalog for a business.
* @param {String} options.businessId
* @param {String} options.catalogId
* @return {Catalog} catalog
*/
Sample Request
.getCatalog({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
catalogId : '365febc2-28b6-411e-85d7-7a57f72ff597'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-08-28T07:47:14Z",
"id": "365febc2-28b6-411e-85d7-7a57f72ff597",
"name": "Foo catalog",
"products": [
{"displayOrder": 1,
"id": "74c89006-ea3c-4c28-8a13-408b77cbe16c"
},
]"updatedAt": "2017-08-28T07:47:14Z"
}
Definition
@classmethod
def get_catalog(cls, business_id, catalog_id):
"""
Get a single catalog for a business.
Arguments:
business_id (str): the business ID
catalog_id (str): the catalog ID
"""
Sample Request
= poynt.Catalog.get_catalog(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'365febc2-28b6-411e-85d7-7a57f72ff597'
)
if status_code < 300:
print(doc)
Sample Response
u'name': u'Foo catalog', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'products': [{u'id': u'74c89006-ea3c-4c28-8a13-408b77cbe16c', u'displayOrder': 1}], u'updatedAt': u'2017-08-28T07:47:14Z', u'id': u'365febc2-28b6-411e-85d7-7a57f72ff597', u'createdAt': u'2017-08-28T07:47:14Z'} {
Get By Id
Get a catalog by id.
Arguments
If-Modified-Since
header- string (optional)
businessId
path- string (required)
catalogId
path- string (required)
Response
Returns a Catalog.
Definition
GET /businesses/{businessId}/catalogs/{catalogId}/full
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.getFullCatalog = function getFullCatalog(options, next) { ... }
module/**
* Get a catalog by id with all product details info embedded in the Catalog.
* @param {String} options.businessId
* @param {String} options.catalogId
* @return {Catalog} catalog
*/
Sample Request
.getFullCatalog({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
catalogId : '365febc2-28b6-411e-85d7-7a57f72ff597'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"categories": [
{"id": "d2c335c9-a771-469f-a0b3-633977aea290",
"name": "Category 2",
"shortCode": "Cat"
},
]"createdAt": "2017-08-28T07:47:14Z",
"id": "365febc2-28b6-411e-85d7-7a57f72ff597",
"name": "Foo catalog",
"products": [
{"displayOrder": 1,
"product": {
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-08-28T07:47:14Z",
"id": "74c89006-ea3c-4c28-8a13-408b77cbe16c",
"name": "Foo 2",
"price": {
"amount": 600,
"currency": "USD"
,
}"shortCode": "Foo2",
"sku": "Foo2",
"status": "ACTIVE",
"type": "SIMPLE",
"updatedAt": "2017-08-28T07:47:14Z"
}
},
]"updatedAt": "2017-08-28T08:08:35Z"
}
Definition
@classmethod
def get_full_catalog(cls, business_id, catalog_id):
"""
Get a catalog by id with all product details info embedded in
the Catalog.
Arguments:
business_id (str): the business ID
catalog_id (str): the catalog ID
"""
Sample Request
= poynt.Catalog.get_full_catalog(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'365febc2-28b6-411e-85d7-7a57f72ff597'
)
if status_code < 300:
print(doc)
Sample Response
u'name': u'Foo catalog', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'products': [{u'product': {u'status': u'ACTIVE', u'sku': u'Foo2', u'name': u'Foo 2', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'type': u'SIMPLE', u'price': {u'currency': u'USD', u'amount': 600}, u'updatedAt': u'2017-08-28T07:47:14Z', u'shortCode': u'Foo2', u'id': u'74c89006-ea3c-4c28-8a13-408b77cbe16c', u'createdAt': u'2017-08-28T07:47:14Z'}, u'displayOrder': 1}], u'createdAt': u'2017-08-28T07:47:14Z', u'updatedAt': u'2017-08-28T08:08:35Z', u'id': u'365febc2-28b6-411e-85d7-7a57f72ff597', u'categories': [{u'shortCode': u'Cat', u'id': u'd2c335c9-a771-469f-a0b3-633977aea290', u'name': u'Category 2'}]} {
Get Full Catalog By Id
Get a catalog by id with all product details info embedded in the Catalog.
Arguments
If-Modified-Since
header- string (optional)
businessId
path- string (required)
catalogId
path- string (required)
Response
Returns a CatalogWithProduct.
Definition
POST /businesses/{businessId}/catalogs
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.createCatalog = function createCatalog(options, catalog, next) { ... }
module/**
* Creates a catalog for a business.
* @param {String} options.businessId
* @param {Catalog} catalog
* @return {Catalog} catalog
*/
Sample Request
.createCatalog({
poyntbusinessId: '18f071cc-5ed4-4b33-80c1-305056d42bfb'
, {
}name: 'Foo catalog'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-08-28T07:42:19Z",
"id": "ee85312d-7449-4003-8222-a22415fdfed9",
"name": "Foo catalog",
"updatedAt": "2017-08-28T07:42:19Z"
}
Definition
@classmethod
def create_catalog(cls, business_id, catalog):
"""
Creates a catalog on a business.
Arguments:
business_id (str): the business ID
catalog (dict): the catalog object
"""
Sample Request
= poynt.Catalog.create_catalog(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
{'name': 'Foo catalog'
}
)
if status_code < 300:
print(doc)
Sample Response
u'name': u'Foo catalog', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'id': u'3301546f-aa10-404c-8dd2-44894db70f56', u'createdAt': u'2017-08-28T07:41:48Z', u'updatedAt': u'2017-08-28T07:41:48Z'} {
Create
Create a catalog for a business.
Arguments
catalog
body- Catalog (optional)
businessId
path- string (required)
Response
Returns a Catalog.
Definition
POST /businesses/{businessId}/catalogs/full
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.createFullCatalog = function createFullCatalog(options, catalog, next) { ... }
module/**
* Creates a catalog for a business. This differs from createCatalog as you can
* create products and catalogs at the same time.
* @param {String} options.businessId
* @param {Catalog} catalog
* @return {Catalog} catalog
*/
Sample Request
.createFullCatalog({
poyntbusinessId: '18f071cc-5ed4-4b33-80c1-305056d42bfb'
, {
}name: 'Foo catalog',
products: [{
product: {
businessId: '18f071cc-5ed4-4b33-80c1-305056d42bfb',
name: 'Foo 2',
price: {
currency: 'USD',
amount: 600
,
}sku: 'Foo2',
shortCode: 'Foo2'
,
}displayOrder: 1
}], function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-08-28T07:48:28Z",
"id": "e3e16774-321e-463f-8ed9-8a996094b1d9",
"name": "Foo catalog",
"products": [
{"displayOrder": 1,
"product": {
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-08-28T07:48:28Z",
"id": "0968d410-cb86-41a1-838c-c9a868ad0335",
"name": "Foo 2",
"price": {
"amount": 600,
"currency": "USD"
,
}"shortCode": "Foo2",
"sku": "Foo2",
"status": "ACTIVE",
"type": "SIMPLE",
"updatedAt": "2017-08-28T07:48:28Z"
}
},
]"updatedAt": "2017-08-28T07:48:28Z"
}
Definition
@classmethod
def create_full_catalog(cls, business_id, full_catalog):
"""
Creates a catalog on a business with embedded products.
This differs from create_catalog as you can create products
and catalogs at the same time.
Arguments:
business_id (str): the business ID
full_catalog (dict): the full catalog object
"""
Sample Request
= poynt.Catalog.create_full_catalog(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
{'name': 'Foo catalog',
'products': [{
'product': {
'businessId': '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'name': 'Foo 2',
'price': {
'currency': 'USD',
'amount': 600
},'sku': 'Foo2',
'shortCode': 'Foo2'
},'displayOrder': 1
}]
}
)
if status_code < 300:
print(doc)
Sample Response
u'name': u'Foo catalog', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'products': [{u'product': {u'status': u'ACTIVE', u'sku': u'Foo2', u'name': u'Foo 2', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'type': u'SIMPLE', u'price': {u'currency': u'USD', u'amount': 600}, u'updatedAt': u'2017-08-28T07:47:14Z', u'shortCode': u'Foo2', u'id': u'74c89006-ea3c-4c28-8a13-408b77cbe16c', u'createdAt': u'2017-08-28T07:47:14Z'}, u'displayOrder': 1}], u'updatedAt': u'2017-08-28T07:47:14Z', u'id': u'365febc2-28b6-411e-85d7-7a57f72ff597', u'createdAt': u'2017-08-28T07:47:14Z'} {
Create Full
Create a catalog with all its embedded products.
Arguments
catalog
body- CatalogWithProduct (optional)
businessId
path- string (required)
Response
Returns a CatalogWithProduct.
Definition
GET /businesses/{businessId}/catalogs/search
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
This method is currently not possible using the Node.js SDK.
Definition
This method is currently not possible using the Python SDK.
Search Catalog
Search for a catalog by name or device id.
Arguments
businessId
path- string (required)
name
query- string (optional)
deviceId
query- string (optional)
Response
Returns a Catalog.
Definition
PATCH /businesses/{businessId}/catalogs/{catalogId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.updateCatalog = function updateCatalog(options, catalogOrPatch, next) { ... }
module/**
* Updates a catalog by ID. Can either specify the whole catalog, or an array
* of JSON Patch instructions.
* @param {String} options.businessId - the business ID
* @param {String} options.catalogId - the catalog ID
* @param {Boolean} options.noRemove - don't remove any keys from old catalog in
* the patch. safer this way. defaults to true
* @param {Object} catalogOrPatch - if is an array, will treat as JSON patch;
* if object, will treat as catalog
* @return {Catalog} catalog
*/
Sample Request
.updateCatalog({
poyntbusinessId: '18f071cc-5ed4-4b33-80c1-305056d42bfb',
catalogId: 'e3e16774-321e-463f-8ed9-8a996094b1d9'
, {
}name: 'New foo catalog'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-08-28T07:48:28Z",
"id": "e3e16774-321e-463f-8ed9-8a996094b1d9",
"name": "New foo catalog",
"products": [
{"displayOrder": 1,
"id": "0968d410-cb86-41a1-838c-c9a868ad0335"
},
]"updatedAt": "2017-08-28T07:50:17Z"
}
Definition
@classmethod
def update_catalog(cls, business_id, catalog_id, catalog=None, patch=None, no_remove=True):
"""
Updates a catalog by ID. Can either specify the whole catalog, or an array
of JSON Patch instructions.
Arguments:
business_id (str): the business ID
catalog_id (str): the catalog ID
Keyword arguments:
catalog (dict): the catalog object. this should be a Catalog, not a CatalogWithProduct.
patch (list of dict): JSON Patch update instructions
no_remove (boolean, optional): don't remove any keys from old catalog in the patch.
safer this way. defaults to True
"""
Sample Request
= poynt.Catalog.update_catalog(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'e3e16774-321e-463f-8ed9-8a996094b1d9',
={
catalog'name': 'New foo catalog'
}
)
if status_code < 300:
print(doc)
Sample Response
u'name': u'Foo catalog', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'products': [{u'product': {u'status': u'ACTIVE', u'sku': u'Foo2', u'name': u'Foo 2', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'type': u'SIMPLE', u'price': {u'currency': u'USD', u'amount': 600}, u'updatedAt': u'2017-08-28T07:47:14Z', u'shortCode': u'Foo2', u'id': u'74c89006-ea3c-4c28-8a13-408b77cbe16c', u'createdAt': u'2017-08-28T07:47:14Z'}, u'displayOrder': 1}], u'updatedAt': u'2017-08-28T07:47:14Z', u'id': u'365febc2-28b6-411e-85d7-7a57f72ff597', u'createdAt': u'2017-08-28T07:47:14Z'} {
Update catalog
Update a catalog.
Arguments
businessId
path- string (required)
catalogId
path- string (required)
patch
body- JsonPatch (optional)
Response
Returns a Catalog.
Definition
DELETE /businesses/{businessId}/catalogs/{catalogId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.deleteCatalog = function deleteCatalog(options, next) { ... }
module/**
* Deletes a single catalog for a business.
* @param {String} options.businessId
* @param {String} options.catalogId
*/
Sample Request
.deleteCatalog({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
catalogId : 'e3e16774-321e-463f-8ed9-8a996094b1d9'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{}
Definition
@classmethod
def delete_catalog(cls, business_id, catalog_id):
"""
Deletes a single catalog for a business.
Arguments:
business_id (str): the business ID
catalog_id (str): the catalog ID
"""
Sample Request
= poynt.Catalog.delete_catalog(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'e3e16774-321e-463f-8ed9-8a996094b1d9'
)
if status_code < 300:
print(doc)
Sample Response
None
Delete catalog
Delete a catalog.
Arguments
businessId
path- string (required)
catalogId
path- string (required)
Definition
POST /businesses/{businessId}/catalogs/{catalogId}/categories
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.createCategory = function createCategory(options, category, next) { ... }
module/**
* Creates a category on a catalog for a business.
* @param {String} options.businessId
* @param {String} options.catalogId
* @param {Category} category
* @return {Category} category
*/
Sample Request
.createCategory({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
catalogId : '365febc2-28b6-411e-85d7-7a57f72ff597'
, {
}name : 'Category',
shortCode : 'Cat'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"id": "d2c335c9-a771-469f-a0b3-633977aea290",
"name": "Category",
"shortCode": "Cat"
}
Definition
@classmethod
def create_category(cls, business_id, catalog_id, category):
"""
Creates a category on a catalog on a business.
Arguments:
business_id (str): the business ID
catalog_id (str): the catalog ID
category (dict): the category object
"""
Sample Request
= poynt.Catalog.create_category(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'365febc2-28b6-411e-85d7-7a57f72ff597',
{'name': 'Category',
'shortCode': 'Cat'
}
)
if status_code < 300:
print(doc)
Sample Response
u'shortCode': u'Cat', u'id': u'4ab8a489-a3fc-4124-87f0-91f8b6044407', u'name': u'Category'} {
Create category
Create a category for a catalog.
Arguments
businessId
path- string (required)
catalogId
path- string (required)
category
body- Category (optional)
Response
Returns a Category.
Definition
GET /businesses/{businessId}/catalogs/{catalogId}/categories/lookup
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.lookupCategories = function lookupCategories(options, next) { ... }
module/**
* Gets multiple categories on a catalog by IDs.
* @param {String} options.businessId
* @param {String} options.catalogId
* @param {String[]} options.ids
* @return {Category[]} categories
*/
Sample Request
.lookupCategories({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
catalogId : '365febc2-28b6-411e-85d7-7a57f72ff597',
ids : ['4ab8a489-a3fc-4124-87f0-91f8b6044407']
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"categories": [
{"id": "4ab8a489-a3fc-4124-87f0-91f8b6044407",
"name": "Category",
"shortCode": "Cat"
}
] }
Definition
@classmethod
def lookup_categories(cls, business_id, catalog_id, category_ids):
"""
Gets multiple categories on a catalog by IDs.
Arguments:
business_id (str): the business ID
catalog_id (str): the catalog ID
category_ids (list of str): a list of category ids
"""
Sample Request
= poynt.Catalog.lookup_categories(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'365febc2-28b6-411e-85d7-7a57f72ff597',
'4ab8a489-a3fc-4124-87f0-91f8b6044407']
[
)
if status_code < 300:
print(doc)
Sample Response
u'categories': [{u'shortCode': u'Cat', u'id': u'4ab8a489-a3fc-4124-87f0-91f8b6044407', u'name': u'Category'}]} {
Get Multiple Id
Get multiple category by ids.
Arguments
businessId
path- string (required)
catalogId
path- string (required)
ids
query- string (required)
Response
Returns a CategoryList.
Definition
GET /businesses/{businessId}/catalogs/{catalogId}/categories/{categoryId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.getCategory = function getCategory(options, next) { ... }
module/**
* Get a single category in a catalog for a business.
* @param {String} options.businessId
* @param {String} options.catalogId
* @param {String} options.categoryId
* @return {Category} category
*/
Sample Request
.getCategory({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
catalogId : '365febc2-28b6-411e-85d7-7a57f72ff597',
categoryId : '4ab8a489-a3fc-4124-87f0-91f8b6044407'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"id": "4ab8a489-a3fc-4124-87f0-91f8b6044407",
"name": "Category",
"shortCode": "Cat"
}
Definition
@classmethod
def get_category(cls, business_id, catalog_id, category_id):
"""
Get a single category in a catalog for a business.
Arguments:
business_id (str): the business ID
catalog_id (str): the catalog ID
category_id (str): the category ID
"""
Sample Request
= poynt.Catalog.get_category(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'365febc2-28b6-411e-85d7-7a57f72ff597',
'4ab8a489-a3fc-4124-87f0-91f8b6044407'
)
if status_code < 300:
print(doc)
Sample Response
u'shortCode': u'Cat', u'id': u'4ab8a489-a3fc-4124-87f0-91f8b6044407', u'name': u'Category'} {
Get category by id
Getall a category by id.
Arguments
businessId
path- string (required)
catalogId
path- string (required)
categoryId
path- string (required)
Response
Returns a Category.
Definition
DELETE /businesses/{businessId}/catalogs/{catalogId}/categories/{categoryId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.deleteCategory = function deleteCategory(options, next) { ... }
module/**
* Deletes a category by ID.
* @param {String} options.businessId
* @param {String} options.catalogId
* @param {String} options.categoryId
* @return {Category} category
*/
Sample Request
.deleteCategory({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
catalogId : '365febc2-28b6-411e-85d7-7a57f72ff597',
categoryId : '4ab8a489-a3fc-4124-87f0-91f8b6044407'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{}
Definition
@classmethod
def delete_category(cls, business_id, catalog_id, category_id):
"""
Deletes a category by ID.
Arguments:
business_id (str): the business ID
catalog_id (str): the catalog ID
category_id (str): the category ID
"""
Sample Request
= poynt.Catalog.delete_category(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'365febc2-28b6-411e-85d7-7a57f72ff597',
'4ab8a489-a3fc-4124-87f0-91f8b6044407'
)
if status_code < 300:
print(doc)
Sample Response
None
Delete category
Delete a category.
Arguments
businessId
path- string (required)
catalogId
path- string (required)
categoryId
path- string (required)
Definition
PATCH /businesses/{businessId}/catalogs/{catalogId}/categories/{categoryId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.updateCategory = function updateCategory(options, categoryOrPatch, next) { ... }
module/**
* Updates a category by ID. Can either specify the whole category, or an array
* of JSON Patch instructions.
* @param {String} options.businessId - the business ID
* @param {String} options.catalogId - the catalog ID
* @param {String} options.categoryId - the category ID
* @param {Boolean} options.noRemove - don't remove any keys from old category in
* the patch. safer this way. defaults to true
* @param {Object} categoryOrPatch - if is an array, will treat as JSON patch;
* if object, will treat as category
* @return {Category} category
*/
Sample Request
.updateCategory({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
catalogId : '365febc2-28b6-411e-85d7-7a57f72ff597',
categoryId : 'd2c335c9-a771-469f-a0b3-633977aea290'
, {
}name : 'Category 2'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"id": "d2c335c9-a771-469f-a0b3-633977aea290",
"name": "Category 2",
"shortCode": "Cat"
}
Definition
@classmethod
def update_category(cls, business_id, catalog_id, category_id, category=None, patch=None, no_remove=True):
"""
Updates a category by ID. Can either specify the whole category, or an array
of JSON Patch instructions.
Arguments:
business_id (str): the business ID
catalog_id (str): the catalog ID
category_id (str): the category ID
Keyword arguments:
category (dict): the category object
patch (list of dict): JSON Patch update instructions
no_remove (boolean, optional): don't remove any keys from old category in the patch.
safer this way. defaults to True
"""
Sample Request
= poynt.Catalog.update_category(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'365febc2-28b6-411e-85d7-7a57f72ff597',
'd2c335c9-a771-469f-a0b3-633977aea290',
={
category'name': 'Category 2'
}
)
if status_code < 300:
print(doc)
Sample Response
u'shortCode': u'Cat', u'id': u'd2c335c9-a771-469f-a0b3-633977aea290', u'name': u'Category 2'} {
Update category
Update a category.
Arguments
businessId
path- string (required)
catalogId
path- string (required)
categoryId
path- string (required)
patch
body- JsonPatch (optional)
Response
Returns a Category.
Taxes
Merchants must collect appropriate taxes based on the location their business and the products they sell. A tax, once defined, can be associated at the catalog, category, or individual product level.
Definition
GET /businesses/{businessId}/taxes
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.getTaxes = function getTaxes(options, next) { ... }
module/**
* Get a list of taxes
* @param {String} options.businessId
* @param {String} options.startAt (optional)
* @param {Integer} options.startOffset (optional)
* @param {String} options.endAt (optional)
* @param {Integer} options.limit (optional)
* @return {TaxList} taxes
*/
Sample Request
.getTaxes({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
startOffset : 0,
limit : 1
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"links": [
{"href": "/businesses/18f071cc-5ed4-4b33-80c1-305056d42bfb/taxes?startAt=2017-07-28T17%3A31%3A38Z&endAt=2017-08-28T08%3A09%3A46Z&limit=1",
"method": "GET",
"rel": "next"
},
]"taxes": [
{"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-04-05T00:18:39Z",
"id": "0f60100b-5332-44b3-9e40-8cb3106be1bc",
"name": "CF Sales",
"rate": 8.25,
"type": "sales",
"updatedAt": "2017-04-05T00:18:39Z"
}
] }
Definition
@classmethod
def get_taxes(cls, business_id, start_at=None, start_offset=None,
=None, limit=None):
end_at"""
Get a list of taxes at a business.
Arguments:
business_id (str): the business ID
Keyword arguments:
start_at (int, optional): get taxes created after this time in seconds
start_offset (int, optional): the numeric offset to start the list (for pagination)
end_at (int, optional): get taxes created before this time in seconds
limit (int, optional): how many taxes to return (for pagination)
"""
Sample Request
= poynt.Tax.get_taxes(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
=0,
start_offset=1
limit
)
if status_code < 300:
print(doc)
Sample Response
u'taxes': [{u'name': u'CF Sales', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'rate': 8.25, u'updatedAt': u'2017-04-05T00:18:39Z', u'type': u'sales', u'id': u'0f60100b-5332-44b3-9e40-8cb3106be1bc', u'createdAt': u'2017-04-05T00:18:39Z'}], u'links': [{u'href': u'/businesses/18f071cc-5ed4-4b33-80c1-305056d42bfb/taxes?startAt=2017-07-28T17%3A31%3A38Z&endAt=2017-08-28T08%3A10%3A24Z&limit=1', u'method': u'GET', u'rel': u'next'}]} {
Get List
Get all tax rate for this business.
Arguments
If-Modified-Since
header- string (optional)
startAt
query- string (optional)
startOffset
query- integer (optional)
endAt
query- string (optional)
limit
query- integer (optional)
businessId
path- string (required)
Response
Returns a TaxList.
Definition
POST /businesses/{businessId}/taxes
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.createTax = function createTax(options, tax, next) { ... }
module/**
* Creates a tax on a business.
* @param {String} options.businessId
* @param {Tax} tax
* @return {Tax} tax
*/
Sample Request
.createTax({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb'
, {
}businessId: '18f071cc-5ed4-4b33-80c1-305056d42bfb',
name: 'Sales tax',
description: 'California sales tax',
type: 'SALES',
rate: 8.25
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-08-28T08:13:02Z",
"description": "California sales tax",
"id": "a0e9a94f-5821-4511-8966-7f8ca3c42167",
"name": "Sales tax",
"rate": 8.25,
"type": "SALES",
"updatedAt": "2017-08-28T08:13:02Z"
}
Definition
@classmethod
def create_tax(cls, business_id, tax):
"""
Creates a tax on a business.
Arguments:
business_id (str): the business ID
tax (dict): the full tax object
"""
Sample Request
= poynt.Tax.create_tax(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
{'businessId': '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'name': 'Sales tax',
'description': 'California sales tax',
'type': 'SALES',
'rate': 8.25
}
)
if status_code < 300:
print(doc)
Sample Response
u'name': u'Sales tax', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'rate': 8.25, u'updatedAt': u'2017-08-28T08:12:19Z', u'type': u'SALES', u'id': u'de581e36-fd76-433d-b104-416978d8d01b', u'createdAt': u'2017-08-28T08:12:19Z', u'description': u'California sales tax'} {
Create
Create a tax rate definition for a business.
Arguments
businessId
path- string (required)
tax
body- Tax (optional)
Response
Returns a Tax.
Definition
DELETE /businesses/{businessId}/taxes/{taxId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.deleteTax = function deleteTax(options, next) { ... }
module/**
* Deactivates a tax. Deactivated taxes will be removed from all catalog
* references.
* @param {String} options.businessId
* @param {String} options.taxId
* @return {Tax} tax
*/
Sample Request
.deleteTax({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
taxId : 'a0e9a94f-5821-4511-8966-7f8ca3c42167'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{}
Definition
@classmethod
def delete_tax(cls, business_id, tax_id):
"""
Deletes a tax.
Arguments:
business_id (str): the business ID
tax_id (str): the tax ID
"""
Sample Request
= poynt.Tax.delete_tax(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'a0e9a94f-5821-4511-8966-7f8ca3c42167'
)
if status_code < 300:
print(doc)
Sample Response
None
Delete tax
Delete a tax.
Arguments
businessId
path- string (required)
taxId
path- string (required)
Definition
GET /businesses/{businessId}/taxes/{taxId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.getTax = function getTax(options, next) { ... }
module/**
* Get a single tax for a business.
* @param {String} options.businessId
* @param {String} options.taxId
* @return {Tax} tax
*/
Sample Request
.getTax({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
taxId : 'de581e36-fd76-433d-b104-416978d8d01b'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-08-28T08:12:19Z",
"description": "California sales tax",
"id": "de581e36-fd76-433d-b104-416978d8d01b",
"name": "Sales tax",
"rate": 8.25,
"type": "SALES",
"updatedAt": "2017-08-28T08:12:19Z"
}
Definition
@classmethod
def get_tax(cls, business_id, tax_id):
"""
Get a single tax for a business.
Arguments:
business_id (str): the business ID
tax_id (str): the tax ID
"""
Sample Request
= poynt.Tax.get_tax(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'de581e36-fd76-433d-b104-416978d8d01b'
)
if status_code < 300:
print(doc)
Sample Response
u'name': u'Sales tax', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'rate': 8.25, u'updatedAt': u'2017-08-28T08:12:19Z', u'type': u'SALES', u'id': u'de581e36-fd76-433d-b104-416978d8d01b', u'createdAt': u'2017-08-28T08:12:19Z', u'description': u'California sales tax'} {
Get
Get a tax rate detail.
Arguments
If-Modified-Since
header- string (optional)
businessId
path- string (required)
taxId
path- string (required)
Response
Returns a Tax.
Definition
PATCH /businesses/{businessId}/taxes/{taxId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.updateTax = function updateTax(options, taxOrPatch, next) { ... }
module/**
* Updates a tax by ID. Can either specify the whole tax, or an array
* of JSON Patch instructions.
* @param {String} options.businessId - the business ID
* @param {String} options.taxId - the tax ID
* @param {Boolean} options.noRemove - don't remove any keys from old tax in
* the patch. safer this way. defaults to true
* @param {Object} taxOrPatch - if is an array, will treat as JSON patch;
* if object, will treat as tax
* @return {Tax} tax
*/
Sample Request
.updateTax({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
taxId : 'de581e36-fd76-433d-b104-416978d8d01b'
, {
}name : 'New sales tax',
rate : 8.3
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"createdAt": "2017-08-28T08:12:19Z",
"updatedAt": "2017-08-28T08:17:47Z",
"rate": 8.3,
"id": "de581e36-fd76-433d-b104-416978d8d01b",
"name": "New sales tax",
"description": "California sales tax",
"type": "SALES",
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb"
}
Definition
@classmethod
def update_tax(cls, business_id, tax_id, tax=None, patch=None, no_remove=True):
"""
Updates a tax by ID. Can either specify the whole tax, or an array
of JSON Patch instructions.
Arguments:
business_id (str): the business ID
tax_id (str): the tax ID
Keyword arguments:
tax (dict): the full tax object
patch (list of dict): JSON Patch update instructions
no_remove (boolean, optional): don't remove any keys from old tax in the patch.
safer this way. defaults to True
"""
Sample Request
= poynt.Tax.update_tax(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'de581e36-fd76-433d-b104-416978d8d01b',
={
tax'name': 'New sales tax',
'rate': 8.3
}
)
if status_code < 300:
print(doc)
Sample Response
u'name': u'Sales tax', u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'rate': 8.25, u'updatedAt': u'2017-08-28T08:12:19Z', u'type': u'SALES', u'id': u'de581e36-fd76-433d-b104-416978d8d01b', u'createdAt': u'2017-08-28T08:12:19Z', u'description': u'California sales tax'} {
Update
Update tax details.
Arguments
businessId
path- string (required)
taxId
path- string (required)
patch
body- JsonPatch (optional)
Response
Returns a Tax.
Receipt Template
This class contains receipt templates resources. It can be used to perform various operations on a receipt template. For example we can store customer transaction receipt template from this resource. Currently we support only Handlebar templates https://github.com/jknack/handlebars.java. The template context will have following objects available: * transaction * business * customerSignatureUrl Once you are ready with the Handlebar template follow these final steps for better results: * JSON encode the template * Put inline CSS to support the final html receipt in all major Email clients.
Definition
POST /business/{businessId}/transactions/receipt-template
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Upsert receipt template
Update or insert a receipt template.
Arguments
businessId
path- string (required)
Poynt-Request-Id
header- string (required)
receiptTemplate
body- ReceiptTemplate (optional)
Response
Returns a ReceiptTemplate.
Notifications
Cloud Messages can be sent from the cloud applications running on the Poynt Smart Terminal.
Webhooks allow developers to register their application to receive callback events from Poynt or replay missed events.
To register for an event type, the developer can create a hook specifying the business and event type they would like to receive callbacks on. This can be done programmatically or via our developer portal UI.
When an event matching the criterias defined in a hook happens, a delivery is made to the developer application endpoint. Webhook deliveries can be redelivered or lookup based on time range as needed.
Cloudmessages
A message sent from the cloud through the Poynt Cloud Messaging to applications running on the Poynt Smart Terminal.
Definition
POST /cloudMessages
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.sendCloudMessage = function sendCloudMessage(options, next) { ... }
module/**
* Send a message from the cloud to your application running at a Poynt terminal.
* @param {String} options.businessId
* @param {String} options.storeId
* @param {String} options.recipientClassName
* @param {String} options.recipientPackageName
* @param {String} options.deviceId
* @param {String} options.serialNumber
* @param {String} options.data (optional) - defaults to "{}"
* @param {String} options.ttl (optional) - defaults to 900 seconds or 15 min
* @param {String} options.collapseKey (optional)
*/
Sample Request
.sendCloudMessage({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
storeId : 'c394627f-4f68-47fb-90a5-684ea801a352',
deviceId : 'urn:tid:48c54303-6d51-39af-bdeb-4af53f621652',
recipientClassName : 'FooClass',
recipientPackageName : 'co.poynt.testapp',
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{}
Definition
@classmethod
def send_cloud_message(cls, business_id=None, store_id=None,
=None, package_name=None, device_id=None,
class_name=None, data='{}', collapse_key=None,
serial_number=900):
ttl"""
Send a message from the cloud to your application running at a Poynt terminal.
Keyword arguments:
business_id (str): the business ID
store_id (str): the store ID
class_name (str): the class name of the receiver in your app
package_name (str): the package name of your app
device_id (str): the device ID
serial_number (str): the serial number
data (str, optional): the data to send. defaults to {}
collapse_key (str, optional): dedupe messages on this key
ttl (int, optional): how long until the cloud message expires. defaults
to 900 seconds
"""
Sample Request
= poynt.CloudMessage.send_cloud_message(
doc, status_code ='18f071cc-5ed4-4b33-80c1-305056d42bfb',
business_id='c394627f-4f68-47fb-90a5-684ea801a352',
store_id='urn:tid:48c54303-6d51-39af-bdeb-4af53f621652',
device_id='foo',
class_name='co.poynt.testapp',
package_name
)
if status_code < 300:
print(doc)
Sample Response
None
Send cloud message
Send a message from the cloud to your application running at a Poynt terminal.
Arguments
message
body- CloudMessage (optional)
Hooks
A hook represent an application’s registration to be notified should a particular resource event occurs.
Definition
POST /hooks
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.createHook = function createHook(options, next) { ... }
module/**
* Subscribes to a webhook.
* @param {String} options.eventType
* @param {String[]} options.eventTypes
* @param {String} options.businessId
* @param {String} options.deliveryUrl
* @param {String} options.secret
*/
Sample Request
.createHook({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb',
eventTypes : ['TRANSACTION_AUTHORIZED', 'TRANSACTION_PENDING', 'TRANSACTION_CAPTURED', 'TRANSACTION_REFUNDED', 'TRANSACTION_UPDATED', 'TRANSACTION_VOIDED'],
deliveryUrl : 'https://poynt.com/hooks',
secret : 'poynt123'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"active": true,
"applicationId": "urn:aid:cb2c55f6-7dfe-482e-a6da-e27c683edf0a",
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-08-28T08:25:46Z",
"deliveryUrl": "https://poynt.com/hooks",
"eventTypes": [
"TRANSACTION_AUTHORIZED",
"TRANSACTION_PENDING",
"TRANSACTION_CAPTURED",
"TRANSACTION_REFUNDED",
"TRANSACTION_UPDATED",
"TRANSACTION_VOIDED"
,
]"id": "4852ebb4-36bf-4060-9ed4-1c3150ddfab6",
"secret": "********",
"updatedAt": "2017-08-28T08:25:46Z"
}
Definition
@classmethod
def create_hook(cls, business_id, delivery_url, secret=None, event_type=None,
=None):
event_types"""
Subscribes to a webhook.
Arguments:
business_id (str): the business ID to subscribe to a hook for. Use a merchant
business ID to subscribe to their e.g. transaction hooks;
use your own organization ID to subscribe to app billing, etc.
delivery_url (str): the URL to deliver webhooks to.
Keyword arguments:
secret (str, optional): used to sign the webhook event, so you can verify.
event_type (str, optional): a single event type to subscribe to webhooks for.
event_types (list of str, optional): a list of event types to subscribe to webhooks for.
"""
Sample Request
= poynt.Hook.create_hook(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb',
'https://poynt.com/hooks',
='poynt123',
secret=['TRANSACTION_AUTHORIZED', 'TRANSACTION_PENDING', 'TRANSACTION_CAPTURED',
event_types'TRANSACTION_REFUNDED', 'TRANSACTION_UPDATED', 'TRANSACTION_VOIDED']
)
if status_code < 300:
print(doc)
Sample Response
u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'deliveryUrl': u'https://poynt.com/hooks', u'secret': u'********', u'updatedAt': u'2017-08-28T08:28:55Z', u'active': True, u'eventTypes': [u'TRANSACTION_AUTHORIZED', u'TRANSACTION_PENDING', u'TRANSACTION_CAPTURED', u'TRANSACTION_REFUNDED', u'TRANSACTION_UPDATED', u'TRANSACTION_VOIDED'], u'applicationId': u'urn:aid:cb2c55f6-7dfe-482e-a6da-e27c683edf0a', u'id': u'0f9bc70f-1de4-4cb7-83a5-248fc358ad56', u'createdAt': u'2017-08-28T08:28:55Z'} {
Create
Create a web hook. Client application MUST have already have been authorized by the business to access the resource of the events in this hook. The following webhooks event are available:
- APPLICATION_SUBSCRIPTION_START - published when a business starts a subscription
- APPLICATION_SUBSCRIPTION_END - published when a business ends a subscription
- APPLICATION_SUBSCRIPTION_PAYMENT_SUCCESS - published when a subscription payment succeeds
- APPLICATION_SUBSCRIPTION_PAYMENT_FAIL - published when a subscription payment fails
- APPLICATION_SUBSCRIPTION_PHASE_CHANGE - published when a subscription phase changes
- APPLICATION_SUBSCRIPTION_REFUND_SUCCESS - published when a subscription refund succeeds
- BUSINESS_USER_CREATED - published when a business (terminal) user is created
- BUSINESS_USER_UPDATED - published when a business (terminal) user is updated
- CATALOG_CREATED - published when a catalog is created
- CATALOG_UPDATED - published when a catalog is updated
- CATALOG_DELETED - published when a catalog is deleted
- CATEGORY_CREATED - published when a category is created
- CATEGORY_UPDATED - published when a category is updated
- CATEGORY_DELETED - published when a category is deleted
- INVENTORY_UPDATED - published when inventory is updated
- ORDER_OPENED - published when a new order is OPENED
- ORDER_CANCELLED - published when an order is CANCELLED
- ORDER_COMPLETED - published when an order is COMPLETED
- ORDER_UPDATED - published when anything other than status changes in the order
- ORDER_ITEM_ORDERED - published when a new item is ORDERED
- ORDER_ITEM_FULFILLED - published when an item is FULFILLED
- ORDER_ITEM_RETURNED - published when an item is RETURNED
- ORDER_ITEM_DELETED - published when an item is DELETED
- ORDER_ITEM_UPDATED - published when anything other than status changes in the item
- PRODUCT_CREATED - published when a new product is created
- PRODUCT_UPDATED - published when product is updated
- PRODUCT_DELETED - published when product is deleted
- STORE_CREATED - published when a store is created
- STORE_UPDATED - published when a store is updated
- TAX_CREATED - published when a new tax is created
- TAX_UPDATED - published when a tax is updated
- TAX_DELETED - published when a tax is deleted
- TRANSACTION_AUTHORIZED - published when a transaction is authorized
- TRANSACTION_PENDING - published when a transaction is pending
- TRANSACTION_CAPTURED - published when a transaction is captured
- TRANSACTION_REFUNDED - published when a captured transaction is refunded
- TRANSACTION_UPDATED - published when a transaction is updated
- TRANSACTION_VOIDED - published when a transaction is voided
- USER_CREATED - published when a web/HQ user is created
- USER_JOIN_ORG - published when a web/HQ user joins a developer organization
- USER_LEAVE_ORG - published when a web/HQ user leaves a developer organization
- USER_JOIN_BIZ - published when a web/HQ user joins a business
- USER_LEAVE_BIZ - published when a web/HQ user leaves a business
- USER_UPDATED - published when a web/HQ user is updated
Arguments
hook
body- Hook (optional)
Response
Returns a Hook.
Definition
GET /hooks
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.getHooks = function getHooks(options, next) { ... }
module/**
* Gets a list of hooks currently subscribed to.
* @param {String} options.businessId
*/
Sample Request
.getHooks({
poyntbusinessId : '18f071cc-5ed4-4b33-80c1-305056d42bfb'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"hooks": [
{"active": true,
"applicationId": "urn:aid:cb2c55f6-7dfe-482e-a6da-e27c683edf0a",
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-08-28T08:25:46Z",
"deliveryUrl": "https://poynt.com/hooks",
"eventTypes": [
"TRANSACTION_AUTHORIZED",
"TRANSACTION_PENDING",
"TRANSACTION_CAPTURED",
"TRANSACTION_REFUNDED",
"TRANSACTION_UPDATED",
"TRANSACTION_VOIDED"
,
]"id": "4852ebb4-36bf-4060-9ed4-1c3150ddfab6",
"secret": "********",
"updatedAt": "2017-08-28T08:25:46Z"
}
] }
Definition
@classmethod
def get_hooks(cls, business_id):
"""
Gets a list of hooks currently subscribed to.
Arguments:
business_id (str): use a merchant business ID to see what webhooks your app
is subscribed to for that merchant. use your own organization
ID to see your app billing webhooks, etc.
"""
Sample Request
= poynt.Hook.get_hooks(
doc, status_code '18f071cc-5ed4-4b33-80c1-305056d42bfb'
)
if status_code < 300:
print(doc)
Sample Response
u'hooks': [{u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'deliveryUrl': u'https://poynt.com/hooks', u'secret': u'********', u'updatedAt': u'2017-08-28T08:25:46Z', u'active': True, u'eventTypes': [u'TRANSACTION_AUTHORIZED', u'TRANSACTION_PENDING', u'TRANSACTION_CAPTURED', u'TRANSACTION_REFUNDED', u'TRANSACTION_UPDATED', u'TRANSACTION_VOIDED'], u'applicationId': u'urn:aid:cb2c55f6-7dfe-482e-a6da-e27c683edf0a', u'id': u'4852ebb4-36bf-4060-9ed4-1c3150ddfab6', u'createdAt': u'2017-08-28T08:25:46Z'}]} {
Get List
Get all webhooks since time. Result is paginated
Arguments
If-Modified-Since
header- string (optional)
startAt
query- string (optional)
startOffset
query- integer (optional)
endAt
query- string (optional)
limit
query- integer (optional)
businessId
query- string (required)
Response
Returns a HookList.
Definition
PATCH /hooks/{hookId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Update a hook
Update a hook
Arguments
hookId
path- string (required)
patch
body- JsonPatch (optional)
Response
Returns a Hook.
Definition
DELETE /hooks/{hookId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.deleteHook = function deleteHook(options, next) { ... }
module/**
* Deletes a hook.
* @param {String} options.hookId
*/
Sample Request
.deleteHook({
poynthookId : '0f9bc70f-1de4-4cb7-83a5-248fc358ad56'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{}
Definition
@classmethod
def delete_hook(cls, hook_id):
"""
Deletes a hook.
Arguments:
hook_id (str): hook ID
"""
Sample Request
= poynt.Hook.delete_hook(
doc, status_code '0f9bc70f-1de4-4cb7-83a5-248fc358ad56'
)
if status_code < 300:
print(doc)
Sample Response
None
Delete hook
Delete a hook.
Arguments
hookId
path- string (required)
Definition
GET /hooks/{hookId}
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
.exports.getHook = function getHook(options, next) { ... }
module/**
* Gets a hook by ID.
* @param {String} options.hookId
*/
Sample Request
.getHook({
poynthookId : '0f9bc70f-1de4-4cb7-83a5-248fc358ad56'
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
; })
Sample Response
{"active": true,
"applicationId": "urn:aid:cb2c55f6-7dfe-482e-a6da-e27c683edf0a",
"businessId": "18f071cc-5ed4-4b33-80c1-305056d42bfb",
"createdAt": "2017-08-28T08:28:55Z",
"deliveryUrl": "https://poynt.com/hooks",
"eventTypes": [
"TRANSACTION_AUTHORIZED",
"TRANSACTION_PENDING",
"TRANSACTION_CAPTURED",
"TRANSACTION_REFUNDED",
"TRANSACTION_UPDATED",
"TRANSACTION_VOIDED"
,
]"id": "0f9bc70f-1de4-4cb7-83a5-248fc358ad56",
"secret": "********",
"updatedAt": "2017-08-28T08:28:55Z"
}
Definition
@classmethod
def get_hook(cls, hook_id):
"""
Gets a hook by ID.
Arguments:
hook_id (str): hook ID
"""
Sample Request
= poynt.Hook.get_hook(
doc, status_code '0f9bc70f-1de4-4cb7-83a5-248fc358ad56'
)
if status_code < 300:
print(doc)
Sample Response
u'businessId': u'18f071cc-5ed4-4b33-80c1-305056d42bfb', u'deliveryUrl': u'https://poynt.com/hooks', u'secret': u'********', u'updatedAt': u'2017-08-28T08:28:55Z', u'active': True, u'eventTypes': [u'TRANSACTION_AUTHORIZED', u'TRANSACTION_PENDING', u'TRANSACTION_CAPTURED', u'TRANSACTION_REFUNDED', u'TRANSACTION_UPDATED', u'TRANSACTION_VOIDED'], u'applicationId': u'urn:aid:cb2c55f6-7dfe-482e-a6da-e27c683edf0a', u'id': u'0f9bc70f-1de4-4cb7-83a5-248fc358ad56', u'createdAt': u'2017-08-28T08:28:55Z'} {
Get By Id
Get a hook by id.
Arguments
If-Modified-Since
header- string (optional)
hookId
path- string (required)
Response
Returns a Hook.
Delivery
When a event occurs which matches the registered Hooks of an application, a delivery is made to the registered delivery url. Delivery are attempted up to 10 times with exponential backoff should there be a failure reaching the delivery url.
Definition
GET /businesses/{businessId}/deliveries
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
This method is currently not possible using the Node.js SDK.
Definition
This method is currently not possible using the Python SDK.
Get List
Get all webhook delivery since time. Result is paginated
Arguments
If-Modified-Since
header- string (optional)
startAt
query- string (optional)
startOffset
query- integer (optional)
endAt
query- string (optional)
limit
query- integer (optional)
businessId
path- string (required)
Response
Returns a DeliveryList.
Definition
POST /businesses/{businessId}/deliveries/redeliver
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
This method is currently not possible using the Node.js SDK.
Definition
This method is currently not possible using the Python SDK.
Redeliver By Time
Redeliver all webhook within a time window.
Arguments
businessId
path- string (required)
Poynt-Request-Id
header- string (optional)
startTime
query- string (required)
endTime
query- string (required)
Definition
POST /businesses/{businessId}/deliveries/{deliveryId}/redeliver
Sample Request
TODO: Add request
Sample Response
TODO: Add response
Definition
This method is currently not possible using the Node.js SDK.
Definition
This method is currently not possible using the Python SDK.
Redeliver Event
Redeliver a webhook delivery.
Arguments
businessId
path- string (required)
deliveryId
path- string (required)
Response
Returns a Delivery.
Apple Pay
Operations for apple pay
Definition
GET /businesses/{businessId}/apple-pay/domain-association-file
Sample Request
curl --location 'https://services.poynt.net/businesses/2989f251-147f-4acf-ad8f-4b6795523d43/apple-pay/domain-association-file' \
'Content-Type: text/plain' --header
Sample Response
7B227073704964223A2231413031344232454330394442333830454531443531464534443131364338303146363246323944373446324439333236394645353534434132453334363536222C2276657273696F6E223A312C22637265617465644F6E223A313632333933303630303032312C227369676E6174757265223A22333038303036303932613836343838366637306430313037303261303830333038303032303130313331306633303064303630393630383634383031363530333034303230313035303033303830303630393261383634383836663730643031303730313030303061303830333038323033653333303832303338386130303330323031303230323038346333303431343935313964353433363330306130363038326138363438636533643034303330323330376133313265333032633036303335353034303330633235343137303730366336353230343137303730366336393633363137343639366636653230343936653734363536373732363137343639366636653230343334313230326432303437333333313236333032343036303335353034306230633164343137303730366336353230343336353732373436393636363936333631373436393666366532303431373537343638366637323639373437393331313333303131303630333535303430613063306134313730373036633635323034393665363332653331306233303039303630333535303430363133303235353533333031653137306433313339333033353331333833303331333333323335333735613137306433323334333033353331333633303331333333323335333735613330356633313235333032333036303335353034303330633163363536333633326437333664373032643632373236663662363537323264373336393637366535663535343333343264353035323466343433313134333031323036303335353034306230633062363934663533323035333739373337343635366437333331313333303131303630333535303430613063306134313730373036633635323034393665363332653331306233303039303630333535303430363133303235353533333035393330313330363037326138363438636533643032303130363038326138363438636533643033303130373033343230303034633231353737656465626436633762323231386636386464373039306131323138646337623062643666326332383364383436303935643934616634613534313162383334323065643831316633343037653833333331663163353463336637656233323230643662616435643465666634393238393839336537633066313361333832303231313330383230323064333030633036303335353164313330313031666630343032333030303330316630363033353531643233303431383330313638303134323366323439633434663933653465663237653663346636323836633366613262626664326534623330343530363038326230363031303530353037303130313034333933303337333033353036303832623036303130353035303733303031383632393638373437343730336132663266366636333733373032653631373037303663363532653633366636643266366636333733373033303334326436313730373036633635363136393633363133333330333233303832303131643036303335353164323030343832303131343330383230313130333038323031306330363039326138363438383666373633363430353031333038316665333038316333303630383262303630313035303530373032303233303831623630633831623335323635366336393631366536333635323036663665323037343638363937333230363336353732373436393636363936333631373436353230363237393230363136653739323037303631373237343739323036313733373337353664363537333230363136333633363537303734363136653633363532303666363632303734363836353230373436383635366532303631373037303663363936333631363236633635323037333734363136653634363137323634323037343635373236643733323036313665363432303633366636653634363937343639366636653733323036663636323037353733363532633230363336353732373436393636363936333631373436353230373036663663363936333739323036313665363432303633363537323734363936363639363336313734363936663665323037303732363136333734363936333635323037333734363137343635366436353665373437333265333033363036303832623036303130353035303730323031313632613638373437343730336132663266373737373737326536313730373036633635326536333666366432663633363537323734363936363639363336313734363536313735373436383666373236393734373932663330333430363033353531643166303432643330326233303239613032376130323538363233363837343734373033613266326636333732366332653631373037303663363532653633366636643266363137303730366336353631363936333631333332653633373236633330316430363033353531643065303431363034313439343537646236666435373438313836383938393736326637653537383530376537396235383234333030653036303335353164306630313031666630343034303330323037383033303066303630393261383634383836663736333634303631643034303230353030333030613036303832613836343863653364303430333032303334393030333034363032323130306265303935373166653731653165373335623535653561666163623463373266656234343566333031383532323263373235313030326236316562643666353530323231303064313862333530613564643664643665623137343630333562313165623263653837636661336536616636636264383338303839306463383263646461613633333038323032656533303832303237356130303330323031303230323038343936643266626633613938646139373330306130363038326138363438636533643034303330323330363733313162333031393036303335353034303330633132343137303730366336353230353236663666373432303433343132303264323034373333333132363330323430363033353530343062306331643431373037303663363532303433363537323734363936363639363336313734363936663665323034313735373436383666373236393734373933313133333031313036303335353034306130633061343137303730366336353230343936653633326533313062333030393036303335353034303631333032353535333330316531373064333133343330333533303336333233333334333633333330356131373064333233393330333533303336333233333334333633333330356133303761333132653330326330363033353530343033306332353431373037303663363532303431373037303663363936333631373436393666366532303439366537343635363737323631373436393666366532303433343132303264323034373333333132363330323430363033353530343062306331643431373037303663363532303433363537323734363936363639363336313734363936663665323034313735373436383666373236393734373933313133333031313036303335353034306130633061343137303730366336353230343936653633326533313062333030393036303335353034303631333032353535333330353933303133303630373261383634386365336430323031303630383261383634386365336430333031303730333432303030346630313731313834313964373634383564353161356532353831303737366538383061326566646537626165346465303864666334623933653133333536643536363562333561653232643039373736306432323465376262613038666437363137636538386362373662623636373062656338653832393834666635343435613338316637333038316634333034363036303832623036303130353035303730313031303433613330333833303336303630383262303630313035303530373330303138363261363837343734373033613266326636663633373337303265363137303730366336353265363336663664326636663633373337303330333432643631373037303663363537323666366637343633363136373333333031643036303335353164306530343136303431343233663234396334346639336534656632376536633466363238366333666132626266643265346233303066303630333535316431333031303166663034303533303033303130316666333031663036303335353164323330343138333031363830313462626230646561313538333338383961613438613939646562656264656261666461636232346162333033373036303335353164316630343330333032653330326361303261613032383836323636383734373437303361326632663633373236633265363137303730366336353265363336663664326636313730373036633635373236663666373436333631363733333265363337323663333030653036303335353164306630313031666630343034303330323031303633303130303630613261383634383836663736333634303630323065303430323035303033303061303630383261383634386365336430343033303230333637303033303634303233303361636637323833353131363939623138366662333563333536636136326266663431376564643930663735346461323865626566313963383135653432623738396638393866373962353939663938643534313064386639646539633266653032333033323264643534343231623061333035373736633564663333383362393036376664313737633263323136643936346663363732363938323132366635346638376137643162393963623962303938393231363130363939306630393932316430303030333138323031386233303832303138373032303130313330383138363330376133313265333032633036303335353034303330633235343137303730366336353230343137303730366336393633363137343639366636653230343936653734363536373732363137343639366636653230343334313230326432303437333333313236333032343036303335353034306230633164343137303730366336353230343336353732373436393636363936333631373436393666366532303431373537343638366637323639373437393331313333303131303630333535303430613063306134313730373036633635323034393665363332653331306233303039303630333535303430363133303235353533303230383463333034313439353139643534333633303064303630393630383634383031363530333034303230313035303061303831393533303138303630393261383634383836663730643031303930333331306230363039326138363438383666373064303130373031333031633036303932613836343838366637306430313039303533313066313730643332333133303336333133373331333133353330333033303561333032613036303932613836343838366637306430313039333433313164333031623330306430363039363038363438303136353033303430323031303530306131306130363038326138363438636533643034303330323330326630363039326138363438383666373064303130393034333132323034323039333631633661633961306163616435333864393063336633326262346262383239333063663832613762366533333933326661303936363235316163663132333030613036303832613836343863653364303430333032303434363330343430323230353038366566656663356664623737383634336166366561643530343737653263393031323831376538363234636234363231333665393339313133303538383032323033333764316639313037333562663138646532346366623363363039646366303339343134643837376136616137656565353163336330373336386131396365303030303030303030303030227D
Definition
/**
* Get domain association file
* @param {String} options.businessId - the business ID
*/
.exports.getApplePayDomainAssociationFile = function (options, next) { ... } module
Sample Request
.getApplePayDomainAssociationFile({
poyntbusinessId : "2989f251-147f-4acf-ad8f-4b6795523d43"
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
})
Sample Response
7B227073704964223A2231413031344232454330394442333830454531443531464534443131364338303146363246323944373446324439333236394645353534434132453334363536222C2276657273696F6E223A312C22637265617465644F6E223A313632333933303630303032312C227369676E6174757265223A22333038303036303932613836343838366637306430313037303261303830333038303032303130313331306633303064303630393630383634383031363530333034303230313035303033303830303630393261383634383836663730643031303730313030303061303830333038323033653333303832303338386130303330323031303230323038346333303431343935313964353433363330306130363038326138363438636533643034303330323330376133313265333032633036303335353034303330633235343137303730366336353230343137303730366336393633363137343639366636653230343936653734363536373732363137343639366636653230343334313230326432303437333333313236333032343036303335353034306230633164343137303730366336353230343336353732373436393636363936333631373436393666366532303431373537343638366637323639373437393331313333303131303630333535303430613063306134313730373036633635323034393665363332653331306233303039303630333535303430363133303235353533333031653137306433313339333033353331333833303331333333323335333735613137306433323334333033353331333633303331333333323335333735613330356633313235333032333036303335353034303330633163363536333633326437333664373032643632373236663662363537323264373336393637366535663535343333343264353035323466343433313134333031323036303335353034306230633062363934663533323035333739373337343635366437333331313333303131303630333535303430613063306134313730373036633635323034393665363332653331306233303039303630333535303430363133303235353533333035393330313330363037326138363438636533643032303130363038326138363438636533643033303130373033343230303034633231353737656465626436633762323231386636386464373039306131323138646337623062643666326332383364383436303935643934616634613534313162383334323065643831316633343037653833333331663163353463336637656233323230643662616435643465666634393238393839336537633066313361333832303231313330383230323064333030633036303335353164313330313031666630343032333030303330316630363033353531643233303431383330313638303134323366323439633434663933653465663237653663346636323836633366613262626664326534623330343530363038326230363031303530353037303130313034333933303337333033353036303832623036303130353035303733303031383632393638373437343730336132663266366636333733373032653631373037303663363532653633366636643266366636333733373033303334326436313730373036633635363136393633363133333330333233303832303131643036303335353164323030343832303131343330383230313130333038323031306330363039326138363438383666373633363430353031333038316665333038316333303630383262303630313035303530373032303233303831623630633831623335323635366336393631366536333635323036663665323037343638363937333230363336353732373436393636363936333631373436353230363237393230363136653739323037303631373237343739323036313733373337353664363537333230363136333633363537303734363136653633363532303666363632303734363836353230373436383635366532303631373037303663363936333631363236633635323037333734363136653634363137323634323037343635373236643733323036313665363432303633366636653634363937343639366636653733323036663636323037353733363532633230363336353732373436393636363936333631373436353230373036663663363936333739323036313665363432303633363537323734363936363639363336313734363936663665323037303732363136333734363936333635323037333734363137343635366436353665373437333265333033363036303832623036303130353035303730323031313632613638373437343730336132663266373737373737326536313730373036633635326536333666366432663633363537323734363936363639363336313734363536313735373436383666373236393734373932663330333430363033353531643166303432643330326233303239613032376130323538363233363837343734373033613266326636333732366332653631373037303663363532653633366636643266363137303730366336353631363936333631333332653633373236633330316430363033353531643065303431363034313439343537646236666435373438313836383938393736326637653537383530376537396235383234333030653036303335353164306630313031666630343034303330323037383033303066303630393261383634383836663736333634303631643034303230353030333030613036303832613836343863653364303430333032303334393030333034363032323130306265303935373166653731653165373335623535653561666163623463373266656234343566333031383532323263373235313030326236316562643666353530323231303064313862333530613564643664643665623137343630333562313165623263653837636661336536616636636264383338303839306463383263646461613633333038323032656533303832303237356130303330323031303230323038343936643266626633613938646139373330306130363038326138363438636533643034303330323330363733313162333031393036303335353034303330633132343137303730366336353230353236663666373432303433343132303264323034373333333132363330323430363033353530343062306331643431373037303663363532303433363537323734363936363639363336313734363936663665323034313735373436383666373236393734373933313133333031313036303335353034306130633061343137303730366336353230343936653633326533313062333030393036303335353034303631333032353535333330316531373064333133343330333533303336333233333334333633333330356131373064333233393330333533303336333233333334333633333330356133303761333132653330326330363033353530343033306332353431373037303663363532303431373037303663363936333631373436393666366532303439366537343635363737323631373436393666366532303433343132303264323034373333333132363330323430363033353530343062306331643431373037303663363532303433363537323734363936363639363336313734363936663665323034313735373436383666373236393734373933313133333031313036303335353034306130633061343137303730366336353230343936653633326533313062333030393036303335353034303631333032353535333330353933303133303630373261383634386365336430323031303630383261383634386365336430333031303730333432303030346630313731313834313964373634383564353161356532353831303737366538383061326566646537626165346465303864666334623933653133333536643536363562333561653232643039373736306432323465376262613038666437363137636538386362373662623636373062656338653832393834666635343435613338316637333038316634333034363036303832623036303130353035303730313031303433613330333833303336303630383262303630313035303530373330303138363261363837343734373033613266326636663633373337303265363137303730366336353265363336663664326636663633373337303330333432643631373037303663363537323666366637343633363136373333333031643036303335353164306530343136303431343233663234396334346639336534656632376536633466363238366333666132626266643265346233303066303630333535316431333031303166663034303533303033303130316666333031663036303335353164323330343138333031363830313462626230646561313538333338383961613438613939646562656264656261666461636232346162333033373036303335353164316630343330333032653330326361303261613032383836323636383734373437303361326632663633373236633265363137303730366336353265363336663664326636313730373036633635373236663666373436333631363733333265363337323663333030653036303335353164306630313031666630343034303330323031303633303130303630613261383634383836663736333634303630323065303430323035303033303061303630383261383634386365336430343033303230333637303033303634303233303361636637323833353131363939623138366662333563333536636136326266663431376564643930663735346461323865626566313963383135653432623738396638393866373962353939663938643534313064386639646539633266653032333033323264643534343231623061333035373736633564663333383362393036376664313737633263323136643936346663363732363938323132366635346638376137643162393963623962303938393231363130363939306630393932316430303030333138323031386233303832303138373032303130313330383138363330376133313265333032633036303335353034303330633235343137303730366336353230343137303730366336393633363137343639366636653230343936653734363536373732363137343639366636653230343334313230326432303437333333313236333032343036303335353034306230633164343137303730366336353230343336353732373436393636363936333631373436393666366532303431373537343638366637323639373437393331313333303131303630333535303430613063306134313730373036633635323034393665363332653331306233303039303630333535303430363133303235353533303230383463333034313439353139643534333633303064303630393630383634383031363530333034303230313035303061303831393533303138303630393261383634383836663730643031303930333331306230363039326138363438383666373064303130373031333031633036303932613836343838366637306430313039303533313066313730643332333133303336333133373331333133353330333033303561333032613036303932613836343838366637306430313039333433313164333031623330306430363039363038363438303136353033303430323031303530306131306130363038326138363438636533643034303330323330326630363039326138363438383666373064303130393034333132323034323039333631633661633961306163616435333864393063336633326262346262383239333063663832613762366533333933326661303936363235316163663132333030613036303832613836343863653364303430333032303434363330343430323230353038366566656663356664623737383634336166366561643530343737653263393031323831376538363234636234363231333665393339313133303538383032323033333764316639313037333562663138646532346366623363363039646366303339343134643837376136616137656565353163336330373336386131396365303030303030303030303030227D
Get domain association file for ApplePay
Get domain association filebased on the environment that client is calling from.
Arguments
businessId
path- string (required)
Definition
POST /businesses/{businessId}/apple-pay/registration
Sample Request
curl --location 'https://services.poynt.net/businesses/2989f251-147f-4acf-ad8f-4b6795523d43/apple-pay/registration' \
'Authorization: BEARER {access_token}' \
--header 'Content-Type: application/json' \
--header '{
--data "unregisterDomains": [
"my1.domain.com"
],
"registerDomains": [
"my2.domain.com",
"my3.domain.com"
],
"merchantName":"Business DBA",
"merchantUrl":"https://my1.domain.com"
}'
Sample Response
{
"domains": [
"my2.domain.com",
"my3.domain.com"
],
"merchantName": "Business DBA",
"merchantUrl": "https://my1.domain.com"
}
Definition
/**
* Update merchant domain from Apple Pay server by business id
* @param {String} options.businessId - the business ID
* @param {String[]} options.unregisterDomains - List of fully qualified domain names where Apple Pay button is displayed
* @param {String[]} options.registerDomains - List of fully qualified domain names where Apple Pay button is displayed
* @param {String} options.reason - Human readable reason of unregistration
* @param {String} options.merchantName - Merchant's e-commerce name
* @param {String} options.merchantUrl - Merchant site url where e-commerce store is hosted
*/
.exports.updateApplePayRegistration = function (options, next) { ... } module
Sample Request
.updateApplePayRegistration({
poyntbusinessId : "2989f251-147f-4acf-ad8f-4b6795523d43",
unregisterDomains : ["my1.domain.com"],
registerDomains : ["my2.domain.com","my3.domain.com"],
merchantName : "Business DBA",
merchantUrl : "https://my1.domain.com"
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
})
Sample Response
{
"domains": [
"my2.domain.com",
"my3.domain.com"
],
"merchantName": "Business DBA",
"merchantUrl": "https://my1.domain.com"
}
Update domain registration for ApplePay
Update merchant registration including domain names, merchant name, and merchant url to ApplePay server
Arguments
request
body- ApplePayRegistrationRequest (optional)
businessId
path- string (required)
Response
Returns a ApplePayRegistrationResponse.
Definition
GET /businesses/{businessId}/apple-pay/registration
Sample Request
curl --location 'https://services.poynt.net/businesses/2989f251-147f-4acf-ad8f-4b6795523d43/apple-pay/registration' \
'Authorization: BEARER {access_token}' \
--header 'Content-Type: application/json' --header
Sample Response
{
"domains": [
"my2.domain.com",
"my3.domain.com"
],
"merchantName": "Business DBA",
"merchantUrl": "https://my1.domain.com"
}
Definition
/**
* Get merchant details by business id
* @param {String} options.businessId - the business ID
*/
.exports.getApplePayRegistration = function (options, next) { ... } module
Sample Request
.getApplePayRegistration({
poyntbusinessId : "2989f251-147f-4acf-ad8f-4b6795523d43"
, function (err, doc) {
}if (err) {
throw err;
}console.log(JSON.stringify(doc));
})
Sample Response
{
"domains": [
"my2.domain.com",
"my3.domain.com"
],
"merchantName": "Business DBA",
"merchantUrl": "https://my1.domain.com"
}
Get merchant details
Get merchant details based on the informationsent during registration.
Arguments
businessId
path- string (required)
Response
Returns a ApplePayRegistrationResponse.
Subscriptions API
In some cases, developer organizations and their appplications also have a web application to increase visibility online as well as bringing in more subscribers to the App Center.
In order to integrate these new subscribers into the GoDaddy Poynt ecosystem, we have designed an API that allows developers to include a subscription route on their web application.
Definition
GET 'https://billing.poynt.net/organizations/{orgId}/apps/{appId}/plans'
Sample Request
curl --location --request GET 'https://billing.poynt.net/organizations/{orgId}/apps/{appId}/plans' \
'Authorization: Bearer {app_access_token}' \
--header 'Content-Type: application/json' --header
Sample Response
{
"list": [
{
"description": "plan test auth",
"scope": "BUSINESS",
"status": "ACTIVE",
"createdAt": "2022-11-27T23:33:51Z",
"updatedAt": "2022-11-27T23:33:51Z",
"appId": "urn:aid:39b5deb9-3bac-49de-8456-22c59415c470",
"startPolicy": "IMMEDIATE",
"amount": 1000,
"payer": "MERCHANT",
"planId": "5ebac9e0-a81d-413c-a61e-4337c993e309",
"trialPeriodDays": 10,
"amounts": [
{
"country": "US",
"currency": "USD",
"value": 1000
}
],
"cancelPolicy": "BILLING",
"interval": "MONTH",
"distributors": [],
"distributorPlan": false,
"merchantActionable": true,
"name": "Plan Teste Auth",
"type": "APP"
},
{
"description": "123123123123",
"scope": "BUSINESS",
"status": "ACTIVE",
"createdAt": "2022-12-08T17:44:43Z",
"updatedAt": "2022-12-08T17:44:43Z",
"appId": "urn:aid:39b5deb9-3bac-49de-8456-22c59415c470",
"startPolicy": "IMMEDIATE",
"amount": 2222,
"payer": "MERCHANT",
"planId": "ffaee414-6ab8-466c-a668-048c42406f49",
"trialPeriodDays": 22,
"amounts": [
{
"country": "US",
"currency": "USD",
"value": 2222
}
],
"cancelPolicy": "BILLING",
"interval": "MONTH",
"distributors": [],
"distributorPlan": false,
"merchantActionable": true,
"name": "123123123",
"type": "APP"
}
],
"start": 0,
"total": 2,
"count": 2
}
Get Plans
In order to provide GoDaddy Poynt merchants with the ability to subscribe to your app directly from your web application, you must connect with the billing system first to fetch your active billing plans.
Headers
- Content-Type: application/json’
- Authorization: Bearer {appAccessToken}’
Query Parameters
- currency=USD - Optional
- businessId={businessId} - Optional
- status={status} - Optional
Definition
POST 'https://billing.poynt.net/organizations/{orgId}/apps/{appId}/subscriptions'
Sample Request
curl --location --request POST 'https://billing.poynt.net/organizations/{orgId}/apps/{appId}/subscriptions' \
'Authorization: Bearer {merchant_access_token}' \
--header 'Content-Type: application/json' \
--header '{
--data "businessId": "5c51b42c-bdcf-42de-b986-06da00955e96",
"orgId": "25a81a4e-f76a-4e42-88fd-6d4890089600",
"planId": "ffaee414-6ab8-466c-a668-048c42406f49",
"replaceV2": false
"startAt": "YYY-MM-DDT19:41:24.649Z",
"addOns": [
{
"planId": "string",
"count": 0,
"action": "ADD",
"addOnId": "string"
}
],
"channelAttribution": "string"
}
Sample Response
{
"scope": "BUSINESS",
"status": "ACTIVE",
"phase": "FULL",
"createdAt": "2023-03-30T04:26:39.716Z",
"updatedAt": "2023-03-30T04:26:39.716Z",
"bundleId": "f1bed559-376e-433e-97b8-91c7f76da127",
"subscriptionId": "9528a26d-410d-4ea4-9e49-f9c71ae610f5",
"appId": "urn:aid:39b5deb9-3bac-49de-8456-22c59415c470",
"payer": "MERCHANT",
"businessId": "5c51b42c-bdcf-42de-b986-06da00955e96",
"planId": "ffaee414-6ab8-466c-a668-048c42406f49",
"startAt": "2023-03-30T00:00:00Z",
"paymentStatus": "OVERDUE"
}
Post Subscriptions
Once you have obtained the merchant authorization, you can use the POST endpoint to create, downgrade, and upgrade subscriptions on behalf of the merchant.
Headers
- Authorization: Bearer {merchantAccessToken}
- Content-Type: application/json
Definition
GET 'https://billing.poynt.net/organizations/{orgId}/apps/{appId}/subscriptions?businessId={businessId}'
Sample Request
curl --location --request GET 'https://billing.poynt.net/organizations/{orgId}/apps/{appId}/subscriptions?businessId={businessId}' \
'Authorization: Bearer {merchant_access_token}' \
--header 'Content-Type: application/json' --header
Sample Response
{
"list": [
{
"scope": "BUSINESS",
"status": "ACTIVE",
"phase": "TRIAL",
"createdAt": "2023-03-29T16:02:47Z",
"updatedAt": "2023-03-29T16:02:47Z",
"bundleId": "90542ebf-076b-4cf4-961f-5a4c24bb2afc",
"subscriptionId": "6b1e433c-eaff-4b3f-a303-db893cebb0bb",
"appId": "urn:aid:39b5deb9-3bac-49de-8456-22c59415c470",
"payer": "MERCHANT",
"businessId": "5c51b42c-bdcf-42de-b986-06da00955e96",
"planId": "5ebac9e0-a81d-413c-a61e-4337c993e309",
"startAt": "2023-03-29T00:00:00Z",
"planName": "Plan Teste Auth"
},
{
"scope": "BUSINESS",
"status": "ACTIVE",
"phase": "FULL",
"createdAt": "2023-03-30T04:26:40Z",
"updatedAt": "2023-03-30T04:26:40Z",
"bundleId": "f1bed559-376e-433e-97b8-91c7f76da127",
"subscriptionId": "9528a26d-410d-4ea4-9e49-f9c71ae610f5",
"appId": "urn:aid:39b5deb9-3bac-49de-8456-22c59415c470",
"payer": "MERCHANT",
"businessId": "5c51b42c-bdcf-42de-b986-06da00955e96",
"planId": "ffaee414-6ab8-466c-a668-048c42406f49",
"startAt": "2023-03-30T00:00:00Z",
"planName": "123123123",
"paymentStatus": "PAID"
}
],
"start": 0,
"total": 2,
"count": 2
}
Get Subscriptions
Once you are able to subscribe on behalf of the merchants, you can also list all the subscriptions for any of your applications using the GET endpoint.
Headers
- Authorization: Bearer {merchantAccessToken}
- Content-Type: application/json
Query Parameters
- businessId={businessId}
- storeId={storeId} - Optional
- deviceId={deviceId} - Optional
- status={status} - Optional
Definition
DELETE 'https://billing.poynt.net/organizations/{orgId}/apps/{appId}/subscriptions/{subscriptionId}'
Sample Request
curl --location --request DELETE 'https://billing.poynt.net/organizations/{orgId}/apps/{appId}/subscriptions/{subscriptionId}' \
'Authorization: Bearer {merchant_access_token}' \
--header 'Content-Type: application/json' --header
Sample Response
{
"scope": "BUSINESS",
"status": "ACTIVE",
"phase": "FULL",
"bundleId": "5c06f5cb-b001-4264-a84c-5de8342948ed",
"planId": "ffaee414-6ab8-466c-a668-048c42406f49",
"businessId": "5c51b42c-bdcf-42de-b986-06da00955e96",
"appId": "urn:aid:39b5deb9-3bac-49de-8456-22c59415c470",
"subscriptionId": "de20ffaf-aff7-4486-93fa-8b11af55c758",
"planName": "123123123",
"createdAt": "2023-03-29T16:04:07Z",
"updatedAt": "2023-04-26T19:24:40Z",
"endAt": "2023-05-01T00:00:00Z",
"paymentStatus": "PAID",
"payer": "MERCHANT",
"startAt": "2023-03-29T00:00:00Z"
}
Deleting Subscriptions
If you decide to delete one of the active subscriptions for a business, you can do so by using the DELETE endpoint as outlined below.
Headers
- Authorization: Bearer {merchant_access_token}
- Content-Type: application/json
POS Bridge
POS Bridge is a GoDaddy Poynt Smart Terminal application that provides a lightweight interface for POS applications running on Android devices, to communicate with the GoDaddy Poynt Terminal using JSON over HTTP.
This is a synchronous mode of communication where POS applications make HTTP requests and wait for a response. If the response time exceeds the timeout value provided by the POS application, POS Bridge will return a timeout error.
Definition
POST http://{terminalIp}/devices/cat/pair
Sample Request
POST /devices/cat/pair HTTP/1.1
Host: 10.255.140.195:55555
User-Agent: curl/7.43.0
Accept: */*
Poynt-Client: Awesome POS
Content-Type: text/plain
Signature: f214750ee34bc658522039f8f94419feed55adbe726e3fa77184f8ba145737dd
Content-Length: 57
{
"poyntRequestId": "A6A8E65B-9790-4B91-9528-CD2A50F8E907"
}
Sample Response
HTTP/1.1 200 OK
Connection: Keep-Alive
Signature: CBB0356E7C7D20958FFBC6C6FC52BCC17E22079AB3CBE5A3843C9A10260C7652
Content-Length: 112
Content-Type: application/json
{
"status":"SUCCESS",
"poyntRequestId":"A6A8E65B-9790-4B91-9528-CD2A50F8E907",
"serialNumber":"P61SWA231FS000383"
}
Pair Device
This endpoint can be used to establish a pair connection between a POS App and a GoDaddy Poynt Smart Terminal.
Headers
- Poynt-Client - String: The name of the POS application. This name will show in the POS Bridge UI.
- Siganture - String: Sha-256 hmac of the JSON payload.
- Content-Type - text/plain: Even if the payload is a JSON object, POS Bridge parses it as a string, therefore it is important to pass content-type as “text/plain” otherwise the authentication will fail.
Request Body
- poyntRequestId - String: uuid
Definition
POST http://{terminalIp}/devices/cat/ping
Sample Request
POST /devices/cat/ping HTTP/1.1
Host: 10.255.140.195:55555
User-Agent: curl/7.43.0
Accept: */*
Content-Type: text/plain
Signature: f214750ee34bc658522039f8f94419feed55adbe726e3fa77184f8ba145737dd
Content-Length: 57
{
"poyntRequestId": "A6A8E65B-9790-4B91-9528-CD2A50F8E907"
}
Sample Response
200 OK
Ping Device
This enpoint is designed to ping the device to check the connectivity.
Headers
- Siganture: String: Sha-256 hmac of the JSON payload.
- Content-Type: text/plain: Even if the payload is a JSON object, POS Bridge parses it as a string, therefore it is important to pass content-type as “text/plain” or the authentication will fail.
Request Body
- poyntRequestId - String: uuid
Response
The response for this endpoint will be empty and it will show an HTTP Status Code according to the connection status.
- 200 - The connection is successful
- 500 - The pairing code on the client (POS application) and server (POS Bridge) do not match
Definition
POST http://{terminalIp}/devices/cat/authorizeSales
Sample Request
POST /devices/cat/authorizeSales HTTP/1.1
Host: 10.255.140.195:55555
User-Agent: curl/7.43.0
Accept: */*
Content-Type: text/plain
Signature: 5421c0acc92fc510c38f5e85e152c3a5545ea1216c46012eca00bd2083e3c4fc
Content-Length: 856
{
"timeout": 60000,
"poyntRequestId": "467A5CCD-5F0A-4C23-8E39-1D43E07D39A1",
"payment":{
"currency": "USD",
"referenceId": "0665DF40-4049-4B0E-8C76-A39F434E01A7",
"orderId": "984B3CFD-0C19-4D27-999F-D1B4B4D75AE1",
"order":{
"amounts":{
"currency": "USD",
"subTotal": 1000
}
},
"id": "984B3CFD-0C19-4D27-999F-D1B4B4D75AE1",
"items": [
{
"name": "Item1",
"quantity": 1,
"status": "ORDERED",
"tax": 0,
"unitOfMeasure": "EACH",
"unitPrice": 100
},
{
"name": "Item2",
"quantity": 1,
"status": "ORDERED",
"tax": 0,
"unitOfMeasure": "EACH",
"unitPrice": 100
},
{
"name": "Item3",
"quantity": 8,
"status": "ORDERED",
"tax": 0,
"unitOfMeasure": "EACH",
"unitPrice": 100
}
],
"statuses":{
"status": "OPENED"
}
},
"amount": 1000,
"tipAmount": 0,
"cashbackAmount": 0,
"disableDebitCards": false,
"disableCash": false,
"debit": false,
"disableTip": false,
"skipReceiptScreen": false,
"cashOnly": false,
"nonReferencedCredit": false,
"multiTender": false
}
Sample Response
HTTP/1.1 200 OK
Connection: Keep-Alive
Signature: F2AD208276FDCDA2B70C08A53FB5FD6DF3AE9D38C7D5C69003EE31A25D91ED19
Content-Length: 4436
Content-Type: application/json
{
"catResponse": {
"accountNumber": "371740******7008",
"approvalCode": "415554",
"cardCompanyID": "A",
"cardHolderFirstName": "JOHSN",
"cardHolderLastName": "SMITH",
"centerResultCode": "",
"sequenceNumber": 752,
"paymentCondition": 0,
"transactionType": 10
},
"payment": {
"transactions": [
{
"action": "SALE",
"amounts": {
"cashbackAmount": 0,
"currency": "USD",
"orderAmount": 1000,
"tipAmount": 0,
"transactionAmount": 1000
},
"authOnly": false,
"context": {
"businessId": "469e957c-57a7-4d54-a72a-9e8f3296adad",
"businessType": "TEST_MERCHANT",
"employeeUserId": 1526454,
"mcc": "5812",
"source": "INSTORE",
"sourceApp": "co.poynt.services",
"storeAddressCity": "Palo Alto",
"storeAddressTerritory": "CA",
"storeDeviceId": "urn:tid:df0f23b3-1d90-3466-91a9-0f2157da5687",
"storeId": "c2855b41-1dd5-4ecc-8258-f0c89ae40338",
"storeTimezone": "America/Los_Angeles",
"transmissionAtLocal": "2017-03-10T23:56:28Z"
},
"createdAt": "2017-03-10T23:56:29Z",
"customerUserId": 2196430,
"fundingSource": {
"card": {
"cardHolderFirstName": "JOSHN",
"cardHolderFullName": "SMITH/JOHN",
"cardHolderLastName": "SMITH",
"encrypted": false,
"expirationDate": 31,
"expirationMonth": 5,
"expirationYear": 2020,
"id": 674974,
"numberFirst6": "371740",
"numberLast4": "7008",
"numberMasked": "371740******7008",
"serviceCode": "201",
"type": "AMERICAN_EXPRESS"
},
"debit": false,
"emvData": {
"emvTags": {
"0x5F24": "XXXXXX",
"0x95": "000000XXXX",
"0x5F20": "XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"0x9F34": "XXXXXX",
"0x9F35": "XX",
"0x9F33": "XXXXXX",
"0x50": "XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"0x9A": "XXXXXX",
"0x9B": "XXXX",
"0x9F36": "XXXX",
"0x9C": "00",
"0x9F37": "XXXXXXXX",
"0x9F10": "XXXXXXXXXXXX",
"0x84": "XXXXXXXXXXXXX",
"0x82": "XXXX",
"0x9F1A": "XXXX",
"0x5F2A": "XXXX",
"0x9F26": "XXXXXXXXXXXXXX",
"0x9F27": "XX",
"0x9F03": "000000000000",
"0x9F06": "XXXXXXXXXXXXXX",
"0x9F02": "XXXXXXXXXX",
"0x5F34": "00",
"0x9F0E": "0000000000",
"0x9F0F": "XXXXXXXXX",
"0x5A": "XXXXXXXXXXXXXX",
"0x9F0D": "XXXXXXXX",
"0x5F28": "XXXX",
"0x5F25": "XXXXXX",
"0x9F16": "XXXXXXXXXXXXX",
"0x9F15": "XXXX",
"0x57": "XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"0x9F39": "XX",
"0x9F1E": "XXXXXXXXXXXXX",
"0x87": "XX",
"0x1F8104": "XXXXXXXXX",
"0x1F8103": "XXXXXXXXXX",
"0x1F815E": "XX",
"0x9F1C": "XXXXXXX",
"0x1F8102": "XXXXXXXXXXXXXX",
"0x9F08": "XXXX",
"0x5F30": "XXXX",
"0x9F07": "XXXX",
"0x9F09": "XXXX",
"0x9F40": "XXXXXXXXX",
"0x9F41": "XXXXXXX",
"0x1F8160": "XX",
"0x1F8162": "XX",
"0x9F21": "XXXXXX"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "INTEGRATED_CIRCUIT_CARD"
},
"type": "CREDIT_DEBIT"
},
"id": "30d3978a-da81-455c-91d6-c7c79ee378d2",
"processorResponse": {
"acquirer": "CHASE_PAYMENTECH",
"approvalCode": "415554",
"approvedAmount": 1000,
"batchId": "1",
"emvTags": {
"0x8A": "XXXX",
"0x89": "XXXXXXXX"
},
"processor": "MOCK",
"retrievalRefNum": "30d3978a-da81-455c-91d6-c7c79ee378d2",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "30d3978a-da81-455c-91d6-c7c79ee378d2"
},
"references": [
{
"id": "984b3cfd-0c19-4d27-999f-d1b4b4d75ae1",
"type": "POYNT_ORDER"
}
],
"signatureCaptured": false,
"status": "CAPTURED",
"updatedAt": "2017-03-10T23:56:29Z"
}
],
"tipAmounts": {
"30d3978a-da81-455c-91d6-c7c79ee378d2": 220
},
"status": "COMPLETED",
"currency": "USD",
"referenceId": "0665DF40-4049-4B0E-8C76-A39F434E01A7",
"orderId": "984b3cfd-0c19-4d27-999f-d1b4b4d75ae1",
"order": {
"amounts": {
"currency": "USD",
"subTotal": 1000
},
"id": "984b3cfd-0c19-4d27-999f-d1b4b4d75ae1",
"items": [
{
"name": "Item1",
"quantity": 1.0,
"status": "ORDERED",
"tax": 0,
"unitOfMeasure": "EACH",
"unitPrice": 100
},
{
"name": "Item2",
"quantity": 1.0,
"status": "ORDERED",
"tax": 0,
"unitOfMeasure": "EACH",
"unitPrice": 100
},
{
"name": "Item3",
"quantity": 8.0,
"status": "ORDERED",
"tax": 0,
"unitOfMeasure": "EACH",
"unitPrice": 100
}
],
"statuses": {
"status": "OPENED"
}
},
"amount": 1000,
"tipAmount": 220,
"cashbackAmount": 0,
"disableEMVCT": false,
"disableEbtCashBenefits": false,
"disableEbtFoodStamps": false,
"disableEbtVoucher": false,
"disableMSR": false,
"disableManual": false,
"disableOther": false,
"disablePaymentOptions": false,
"disableTip": false,
"disableDCC": false,
"isBalanceInquiry": false,
"manualEntry": false,
"multiTender": false,
"nonReferencedCredit": false,
"disableCheck": false,
"disableChangeAmount": false,
"disableCash": false,
"readCardDataOnly": false,
"debitOnly": false,
"debit": false,
"skipReceiptScreen": false,
"creditOnly": false,
"disableEMVCL": false,
"disableDebitCards": false,
"cashOnly": false,
"authzOnly": false,
"disableDebit": false,
"adjustToAddCharges": false,
"verifyOnly": false,
"voucher": false
},
"poyntRequestId": "467A5CCD-5F0A-4C23-8E39-1D43E07D39A1"
}
Sale / Authorization
Headers
- Siganture - String: Sha-256 hmac of the JSON payload.
- Content-Type - text/plain: Even if the payload is a JSON object, POS Bridge parses it as a string, therefore it is important to pass content-type as “text/plain” otherwise the authentication will fail.
Request Body
- poyntRequestId - String: UUID of the request.
- timeout - int: Client timeout in milliseconds. Once client timeout is reached, POS Bridge will return an error.
- payment - JSON Object: Contains options for the payment session. Encloses all the properties below.
- currency - String: 3 digit currency code. This property will be inside the payment object.
- referenceId - String: Optional. Reference ID that can be set by the POS. If not set, POS Bridge sets it by default to a UUID.
- orderId - String: Optional. UUID. If there is an order Object created in the Poynt cloud, setting orderId will link this payment with the order object.
- order - JSON Object: Optional. For more information please refer to Orders.
- amount - int: Transaction amount in cents.
- tipAmount - int: Optional. Tip amount in cents (this is in addition to amount).
- cashbackAmount - int: Optional. Cashback amount in cents (can only be used for debit transactions.)
- disableCash - boolean: Optional. If set to true, cash won’t be an option for this payment session.
- disableDebitCards - boolean: Optional. If set to true, debit won’t be an option on Poynt for this payment session.
- disableTip - boolean: Optional. Tip screen will be disabled for this payment session. Tip screen only shows if the merchant account is configured for tip adjustment.
- skipReceiptScreen - boolean: Optional. If set to true, the receipt screen is skipped on the terminal. Receipt screen allows customers to request printed receipts or receipts via email and SMS.
- cashOnly - boolean: Optional. If set to true, payment session will default to cash tender.
- multiTender - boolean: Optional. If set to true, merchant will be able to do a split tender transaction if needed.
- creditOnly - boolean: Optional. Disable any other tender options except credit.
- manualEntry - boolean: Optional. Terminal prompts for card to be entered manually.
Definition
POST http://{terminalIp}/devices/cat/authorizePreSales
Sample Request
Sample Response
Pre-sale / Pre-authorization
Calling this endpoint will automatically set authOnly flag in the Payment object to “true”. However, debit is not possible for this type of transaction.
For more details on this endpoint, please refer to the Sale/Authorization endpoint.
Definition
POST http://{terminalIp}/devices/cat/authorizeAdjustment
Sample Request
POST /devices/cat/authorizeAdjustment HTTP/1.1
Host: 127.0.0.1:55555
User-Agent: curl/7.43.0
Accept: */*
Content-Type: text/plain
Signature: 5306cda8c3871bcfc49371c3449b8444729aee90d9f29f73227cf98f9dfb063a
Content-Length: 250
{
"poyntRequestId": "095DA086-B511-48B8-8A0B-95E61BFD010D",
"transactionId": "29616c32-998c-4383-8002-34dec0249a8b",
"timeout": 60000,
"payment":{
"amount": 3000,
"tipAmount": 1000
}
}
Sample Response
HTTP/1.1 200 OK
Signature: 6B9D0D17228867D60F5729DC940EA1F888B07034C88B7EB410F60BDB7C376277
Content-Length: 2969
Content-Type: application/json
{
"payment":{
"transactions":[
{
"action": "AUTHORIZE",
"adjusted": true,
"amounts":{
"cashbackAmount": 0,
"currency": "USD",
"customerOptedNoTip": false,
"orderAmount": 3000,
"tipAmount": 1000,
"transactionAmount": 4000
},
"amountsAdjusted": true,
"authOnly": false,
},
Adjustment
The adjustment operation allows adjusting base sale amount, tip amount or both.
Headers
- Siganture - String: Sha-256 hmac of the JSON payload.
- Content-Type - text/plain: Even if the payload is a JSON object, POS Bridge parses it as a string, therefore it is important to pass content-type as “text/plain” otherwise the authentication will fail.
Request Body
- poyntRequestId - String: UUID of the request.
- transactionId - String: Poynt transaction ID of the previously created authorization.
- timeout - int: Client timeout in milliseconds.
- payment - JSON Object: Contains options for the payment session. Encloses all the properties below.
- amount - int: Optional. Adjusted sale amount in cents.
- tipAmount - int: Optional. Adjusted tip amount in cents.
Definition
POST http://{terminalIp}/devices/cat/authorizeCompletion
Sample Request
POST /devices/cat/authorizeCompletion HTTP/1.1
Host: 127.0.0.1:55555
User-Agent: curl/7.43.0
Accept: */*
Content-Type: text/plain
Signature: a0e0131cfefc4c622baadd0ad24301afcdbf1862608fb11ade9ef3cc7de60b62
Content-Length: 232
{
"poyntRequestId": "06C12A34-BE38-497D-B6F0-F4F4C193B746",
"transactionId": "29616c32-998c-4383-8002-34dec0249a8b",
"timeout": 60000,
"payment":{
"amount": 3000,
"tipAmount":1000
},
"timeout": 60000,
"transactionId": "29616c32-998c-4383-8002-34dec0249a8b"
}
Sample Response
HTTP/1.1 200 OK
Connection: Keep-Alive
Signature: 1D4CBE0807D5DA60E3DDE461B05D5A87886379A135D0D53234FCF4EDCCAC7D8A
Content-Length: 2771
Content-Type: application/json
{
"payment":{
"transactions":[
{
"action": "CAPTURE",
"links":[
{
"href": "29616c32-998c-4383-8002-34dec0249a8b",
"method": "GET",
"rel": "AUTHORIZE"
}
],
"status": "CAPTURED",
Capture
The adjustment operation allows adjusting base sale amount, tip amount or both.
Headers
- Siganture - String: Sha-256 hmac of the JSON payload.
- Content-Type - text/plain: Even if the payload is a JSON object, POS Bridge parses it as a string, therefore it is important to pass content-type as “text/plain” otherwise the authentication will fail.
Request Body
- poyntRequestId - String: UUID of the request.
- transactionId - String: Poynt transaction ID of the previously created authorization.
- timeout - int: Client timeout in milliseconds.
- payment - JSON Object: Contains options for the payment session. Encloses all the properties below.
- amount - int: Optional. Amount to capture in cents. If not provided full amount will be captured.
- tipAmount - int: Optional. Tip amount in cents. If not provided, full tipAmount will be captured.
Definition
POST http://{terminalIp}/devices/cat/authorizeRefund
Sample Request
POST /devices/cat/authorizeRefund HTTP/1.1
Host: 127.0.0.1:55555
User-Agent: curl/7.43.0
Accept: */*
Content-Type: text/plain
Signature: 8ffebf07e1d224588205934d2c535dcd6d7fac9daf0bf4387d102f4c09e76bb1
Content-Length: 231
{
"poyntRequestId": "7B108C92-BD31-4DDF-B94B-0500F715726F",
"transactionId": "402876d3-79d7-4794-a4bf-99bfbaac896c",
"timeout": 60000,
"payment":{
"amount": 500
}
}
Sample Response
HTTP/1.1 200 OK
Connection: Keep-Alive
Signature: 7201C30B174448960F39AFEE2CD676FBE207FA3B5E4ADC79518C985CB94019C9
Content-Length: 2701
Content-Type: application/json
{
"payment":{
"transactions":[
{
"action": "REFUND",
"status": "REFUNDED",
"links":[
{
"href": "402876d3-79d7-4794-a4bf-99bfbaac896c",
"method": "GET",
"rel": "CAPTURE"
}
],
Refund
The adjustment operation allows adjusting base sale amount, tip amount or both.
Headers
- Siganture - String: Sha-256 hmac of the JSON payload.
- Content-Type - text/plain: Even if the payload is a JSON object, POS Bridge parses it as a string, therefore it is important to pass content-type as “text/plain” otherwise the authentication will fail.
Request Body
- poyntRequestId - String: UUID of the request.
- transactionId - String: Poynt transaction id of the previous capture or sale transaction.
- timeout - int: Client timeout in milliseconds.
- payment - JSON Object: Required. Contains options for the payment session. Encloses all the properties below. This can be an empty object if amount is not specified.
- amount - int: Optional. Amount to refund in cents. If not provided full amount will be refunded
Definition
POST http://{terminalIp}/devices/cat/authorizeSales
Sample Request
POST /devices/cat/authorizeSales HTTP/1.1
Host: 127.0.0.1:55555
User-Agent: curl/7.43.0
Accept: */*
Content-Type: text/plain
Signature: c9fa862e1e1ee49c112dabcb81d06be7b27ef3661c5d551f1ebac6b4d555be68
Content-Length: 933
{
"timeout": 70000,
"poyntRequestId": "B985B5CC-F8E9-47CD-85E9-4F3171FF31B0",
"payment":{
"currency": "USD",
"referenceId": "586DFE74-5CB6-4692-9FF3-048CA049673C",
"orderId": "91D4FAA4-FA5B-45E9-BB54-7ACB1438079D",
"order":{
"amounts":{
"currency": "USD",
"subTotal": 1000
},
"id": "91D4FAA4-FA5B-45E9-BB54-7ACB1438079D",
"items":[
{
"name": "Item1",
"quantity": 1,
"status": "ORDERED",
"tax": 0,
"unitOfMeasure": "EACH",
"unitPrice": 100
},
{
"name": "Item2", "quantity": 1,
"status": "ORDERED",
"tax": 0,
"unitOfMeasure": "EACH",
"unitPrice": 100
},
{
"name": "Item3",
"quantity": 8,
"status": "ORDERED",
"tax": 0,
"unitOfMeasure": "EACH",
"unitPrice": 100
},
],
"statuses":{
"status": "OPENED"
}
},
"amount": 1000,
"tipAmount": 0,
"skipReceiptScreen": true,
"offlineAuth": true,
"offlineApprovalCode": "qwerty"
}
}
Sample Response
HTTP/1.1 200 OK
Connection: Keep-Alive
Signature: 3F57A635AAD46F9B19C9B2FC440B54E884ECFBE9B2657FFE704D9C3E4689450A
Content-Length: 3264
Content-Type: application/json
{
"catResponse":{
"approvalCode": "",
"cardCompanyID": "V",
"cardHolderFirstName": "",
"cardHolderLastName": "07675006903$00000$",
"centerResultCode": "",
"sequenceNumber": 77,
"paymentCondition": 0,
"transactionType": 10
},
"payment":{
"transactions":[
{
"action": "SALE",
"amounts":{
"currency": "USD",
"orderAmount": 1000,
"tipAmount": 0,
"transactionAmount": 1000
},
"approvalCode": "qwerty",
"authOnly": false,
"createdAt": "2017-03-27T22:23:07Z",
"fundingSource":{
"card":{
"cardHolderFirstName": "",
"cardHolderFullName": "07675006903$00000$",
"cardHolderLastName": "07675006903$00000$",
"encrypted": true,
"expirationDate": 31,
"expirationMonth": 5,
"expirationYear": 2021,
"keySerialNumber": "FFFF9876543210E000D7", "numberFirst6": "435880",
"numberLast4": "3536",
"track1data":"E1ADBE420D2462373856574FB593CDF5446824682BB8F04433EA1C97613BF67D85E6E4E0956D12AE6AFB1079E7A7FB9A216B C373004E082143468FE6EFF93C5FB1C16FB586AE6A13",
"track2data": "F53B2BB0FCAD19E96E846F39C603CDBC8FE558218584B9DB37AC4479F943DF00287D2C55A6331059",
"type": "VISA"
},
"emvData":{
"emvTags":{
"0x5F24": "210531",
"0x1F815D": "47",
"0x5F20": "303736373530303639303324303030303024",
"0x1F8104": "33353336",
"0x1F815F": "01",
"0x1F8103": "343335383830",
"0x5F2A": "0840",
"0x1F815E": "26",
"0x1F8102": "FFFF9876543210E000D7",
"0x5F30": "121F",
"0x1F8160": "02",
"0x1F8161": "00",
"0x1F8162": "00",
"0x5F36": "02",
"0x57": "F53B2BB0FCAD19E96E846F39C603CDBC8FE558218584B9DB37AC4479F943DF00287D2C55A6331059",
"0x58": "",
"0x9F39": "02",
"0x1F8153": "28E578F2",
"0x56": "E1ADBE420D2462373856574FB593CDF5446824682BB8F04433EA1C97613BF67D85E6E4E0956D12AE6AFB1079E7A7FB9A216B C373004E082143468FE6EFF93C5FB1C16FB586AE6A13"
}
},
"entryDetails":{
"customerPresenceStatus": "PRESENT",
"entryMode": "TRACK_DATA_FROM_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"id": "11dd9a34-015b-1000-fc08-2b6e519177d8",
"references":[
{
"id": "91d4faa4-fa5b-45e9-bb54-7acb1438079d",
"type": "POYNT_ORDER"
}
],
"status": "CAPTURED"
}
],
"status": "COMPLETED",
"currency": "USD",
"referenceId": "586DFE74-5CB6-4692-9FF3-048CA049673C",
"orderId": "91d4faa4-fa5b-45e9-bb54-7acb1438079d",
"order":{
"amounts":{
"currency": "USD",
"subTotal": 1000
},
"id": "91d4faa4-fa5b-45e9-bb54-7acb1438079d",
"items":[
{
"name": "Item1",
"quantity": 1,
"status": "ORDERED",
"tax": 0,
"unitOfMeasure": "EACH",
"unitPrice": 100
},
{
"name": "Item2",
"quantity": 1,
"status": "ORDERED",
"tax": 0,
"unitOfMeasure": "EACH",
"unitPrice": 100
},
{
"name": "Item3",
"quantity": 8,
"status": "ORDERED",
"tax": 0,
"unitOfMeasure": "EACH",
"unitPrice": 100
}
],
"statuses":{
"status": "OPENED"
}
},
"offlineApprovalCode": "qwerty",
"amount": 1000,
"tipAmount": 0,
"cashbackAmount": 0,
"disableEMVCT": false,
"disableEbtCashBenefits": false,
"disableEbtFoodStamps": false,
"disableEbtVoucher": false,
"disableMSR": false,
"disableManual": false,
"disableOther": false,
"disablePaymentOptions": false,
"disableTip": false,
"disableDCC": false,
"isBalanceInquiry": false,
"manualEntry": false,
"multiTender": false,
"nonReferencedCredit": false,
"disableCheck": false,
"disableChangeAmount": false,
"offlineAuth": true,
"disableCash": false,
"debitOnly": false,
"readCardDataOnly": false,
"debit": false,
"creditOnly": false,
"skipReceiptScreen": true,
"disableEMVCL": false,
"cashOnly": false,
"disableDebitCards": false,
"authzOnly": false,
"applicationIndex": 0,
"disableDebit": false,
"adjustToAddCharges": false,
"verifyOnly": false,
"voucher": false
},
"poyntRequestId": "B985B5CC-F8E9-47CD-85E9-4F3171FF31B0"
}
Voice Authorization
The adjustment operation allows adjusting base sale amount, tip amount or both.
Headers
- Siganture - String: Sha-256 hmac of the JSON payload.
- Content-Type - text/plain: Even if the payload is a JSON object, POS Bridge parses it as a string, therefore it is important to pass content-type as “text/plain” otherwise the authentication will fail.
Request Body
- poyntRequestId - String: UUID of the request.
- timeout - int: Client timeout in milliseconds. Once client timeout is reached POS Bridge will return an error.
- payment - JSON Object: Contains options for the payment session. Encloses all the properties below.
- currency - String: 3 digit currency code. Property inside payment object.
- referenceId - String: Optional. Reference id that can be set by the POS. If not set, POS Bridge sets it by default to a UUID.
- orderId - String: Optional. UUID. If there is an order Object created in the Poynt cloud, setting orderId will link this payment with the order object.
- order - JSON Object: Optional. For more information please refer to the Orders section.
- amount - int: Transaction amount in cents.
- tipAmount - int: Optional. Tip amount in cents (this is in addition to amount).
- skipReceiptScreen - boolean: Optional. If set to true, receipt screen is skipped on the terminal. Receipt screen allows customer to request printed receipt or receipt via email and SMS.
- manualEntry - boolean: Optional. Terminal prompts for card to be entered manually. This option will only work if the terminal is configured to process manual entry on the second screen.
- offlineAuth - boolean: Required. Forces the terminal to go into voice auth mode.
- offlineApprovalCode - String: Optional. If not provided the terminal operator will be prompted to enter the auth code in the payment fragment.
Definition
POST http://{terminalIp}/devices/cat/authorizeVoid
Sample Request
POST /devices/cat/authorizeVoid HTTP/1.1
Host: 127.0.0.1:55555
User-Agent: curl/7.43.0
Accept: */*
Content-Type: text/plain
Content-Type: application/json
Signature: 9e16609e210eb48d31fbb512965e868a163801ef33223fb582ecfa4b60821e88
Content-Length: 131
{
"poyntRequestId": "7982DC19-274C-44B2-8DEB-7016B62C26E1",
"transactionId": "3fbdb67d-b010-4ee9-8f20-af2f2f6bbc60",
"timeout": 60000
}
Sample Response
HTTP/1.1 200 OK
Connection: Keep-Alive
Signature: C3291676F8489CB00541BBFF44EB2B0324FCBD2270BE0E4F3BC90876D12044C3
Content-Length: 2817
Content-Type: application/json
{
"catResponse": {
"accountNumber": "525107******1301",
"approvalCode": "630339",
"cardCompanyID": "M",
"cardHolderFirstName": "JAMES",
"cardHolderLastName": "SMITH",
"centerResultCode": "",
"sequenceNumber": 5415,
"paymentCondition": 0,
"transactionType": 29
},
"payment": {
"transactions": [
{
"action": "AUTHORIZE",
"adjusted": true,
"amounts": {
"cashbackAmount": 0,
"currency": "USD",
"customerOptedNoTip": false,
"orderAmount": 9753100,
"tipAmount": 2438275,
"transactionAmount": 12191375
},
"authOnly": false,
"context": {
"businessId": "469e957c-57a7-4d54-a72a-9e8f3296adad",
"businessType": "TEST_MERCHANT",
"employeeUserId": 1526454,
"mcc": "5812",
"source": "INSTORE",
"sourceApp": "co.poynt.services",
"storeDeviceId": "urn:tid:df0f23b3-1d90-3466-91a9-0f2157da5687",
"storeId": "c2855b41-1dd5-4ecc-8258-f0c89ae40338",
"transmissionAtLocal": "2017-03-11T18:18:53Z"
},
"createdAt": "2017-03-11T18:18:54Z",
"customerUserId": 1543964,
"fundingSource": {
"card": {
"cardHolderFirstName": "JAMES",
"cardHolderFullName": "SMITH/JAMES",
"cardHolderLastName": "SMITH",
"expirationMonth": 7,
"expirationYear": 2018,
"id": 34541,
"numberFirst6": "525107",
"numberLast4": "1301",
"numberMasked": "525107******1301",
"status": "ACTIVE",
"type": "MASTERCARD"
},
"debit": false,
"emvData": {
"emvTags": {
"0x5F2A": "0840",
"0x5F24": "180731"
}
},
"entryDetails": {
"customerPresenceStatus": "PRESENT",
"entryMode": "TRACK_DATA_FROM_MAGSTRIPE"
},
"type": "CREDIT_DEBIT"
},
"id": "3fbdb67d-b010-4ee9-8f20-af2f2f6bbc60",
"links": [
{
"href": "ed56ab6a-ee35-4c88-ac51-e3e2f53f220b",
"method": "GET",
"rel": "REFUND"
}
],
"partiallyApproved": false,
"processorResponse": {
"acquirer": "CHASE_PAYMENTECH",
"approvalCode": "630339",
"approvedAmount": 9753100,
"batchId": "1",
"processor": "MOCK",
"retrievalRefNum": "3fbdb67d-b010-4ee9-8f20-af2f2f6bbc60",
"status": "Successful",
"statusCode": "1",
"statusMessage": "Successful",
"transactionId": "3fbdb67d-b010-4ee9-8f20-af2f2f6bbc60"
},
"references": [
{
"customType": "referenceId",
"id": "be97ff20-015a-1000-dd69-f169c19f49b0",
"type": "CUSTOM"
}
],
"signatureCaptured": true,
"status": "VOIDED",
"updatedAt": "2017-03-11T22:59:33Z",
"voided": true
}
],
"status": "COMPLETED",
"amount": 0,
"tipAmount": 0,
"cashbackAmount": 0,
"disableEMVCT": false,
"disableEbtCashBenefits": false,
"disableEbtFoodStamps": false,
"disableEbtVoucher": false,
"disableMSR": false,
"disableManual": false,
"disableOther": false,
"disablePaymentOptions": false,
"disableTip": false,
"disableDCC": false,
"isBalanceInquiry": false,
"manualEntry": false,
"multiTender": false,
"nonReferencedCredit": false,
"disableCheck": false,
"disableChangeAmount": false,
"disableCash": false,
"readCardDataOnly": false,
"debitOnly": false,
"debit": false,
"skipReceiptScreen": false,
"creditOnly": false,
"disableEMVCL": false,
"disableDebitCards": false,
"cashOnly": false,
"authzOnly": false,
"disableDebit": false,
"adjustToAddCharges": false,
"verifyOnly": false,
"voucher": false
},
"poyntRequestId": "7982DC19-274C-44B2-8DEB-7016B62C26E1"
}
Void
Headers
- Siganture - String: Sha-256 hmac of the JSON payload.
- Content-Type - text/plain: Even if the payload is a JSON object, POS Bridge parses it as a string, therefore it is important to pass content-type as “text/plain” otherwise the authentication will fail.
Request Body
- poyntRequestId - String: UUID of the request.
- transactionId - String: Poynt transaction ID of the previous authorization or pre-authorization.
- poyntRequestId - String: Client timeout in milliseconds.
Definition
POST http://{terminalIp}/devices/imagescanner/getScanData
Sample Request
POST /devices/cat/getScanData HTTP/1.1
Poynt-Client: Java Sample
Poynt-Request-Id: 4950c723-e340-4b2f-a206-262bf60e3594
Signature: 908A49D6BE5BCE7C007FA56B17B9ED09AFA7878BBC40A15AB89B8D1D458D79F2
Content-Type: text/plain; charset=utf-8
Content-Length: 57
Host: 127.0.0.1:55555
Connection: Keep-Alive
Accept-Encoding: gzip
User-Agent: okhttp/3.5.0 =>
{
"poyntRequestId": "c6194f2b-9fab-4bc8-a0b5-7a272120d905"
}
Sample Response
HTTP/1.1 200 OK
Connection: Keep-Alive
Signature: BE133A911686A36E11C895E9F8E009D96D948BDE65CDA5A6B4F13EEB12182789
Content-Length: 105
Content-Type: application/json <=
{
"status":"SUCCESS",
"poyntRequestId":"c6194f2b-9fab-4bc8-a0b5-7a272120d905",
"scanResult":"759751002497"
}
Scan Data
Headers
- Siganture - String: Sha-256 hmac of the JSON payload.
- Content-Type - text/plain: Even if the payload is a JSON object, POS Bridge parses it as a string, therefore it is important to pass content-type as “text/plain” otherwise the authentication will fail.
Request Body
- poyntRequestId - String: UUID of the request.
Definition
POST http://{terminalIp}/devices/posprinter/printNormal
Sample Request
POST /devices/posprinter/printNormal HTTP/1.1
Host: 127.0.0.1:55555
User-Agent: curl/7.43.0
Accept: */*
Content-Type: text/plain
Signature: d81b8b51cbe18381e225120720e2a59d9973d01d04e338212bc8b65d9f2d8e96
Content-Length: 135 =>
{
"timeout": 70000,
"poyntRequestId": "3B35ED17-0812-4AF2-942C-17ADBF89EA74",
"printingRequest":{
"content": "Your order#:\n553\n\n\n\n\n"
}
}
Sample Response
HTTP/1.1 200 OK
Connection: Keep-Alive
Signature: 4709EC46B966C73CF8EE03CB106C1215D4734DD542DC37E73755EB14658EA815
Content-Length: 57
Content-Type: application/json <=
{
"poyntRequestId":"3B35ED17-0812-4AF2-942C-17ADBF89EA74"
}
Print Receipt
This endpoint can be used to print receipts as necessary
Headers
- Siganture - String: Sha-256 hmac of the JSON payload.
- Content-Type - text/plain: Content-type of the payload.
Request Body
- poyntRequestId - String: UUID of the request.
- tiemout - int: Client timeout in milliseconds.
- printingRequest - Object: Encloses the property below.
- content - String: Text to print. Property inside the printingRequest object.
Definition
POST http://{terminalIp}/devices/secondscreen/showItems
Sample Request
POST /devices/secondscreen/showItems HTTP/1.1
Host: 127.0.0.1:55555
User-Agent: curl/7.43.0
Accept: */*
Content-Type: text/plain
Signature: 3d3719747a9a7f5b08454f6a7a1441990adb9674d83f7c5e8223533652c2ea16
Content-Length: 239
{
"timeout": 70000,
"poyntRequestId": "123525C7-D6A5-4FE2-BAA8-2278E10D5C5F",
"secondScreenRequest":{
"totalAmount": 100,
"currency": "USD",
"items":[
{
"name": "Milk tea",
"quantity": 1,
"status": "ORDERED",
"tax": 0,
"unitOfMeasure": "EACH",
"unitPrice": 100
}
]
}
}
Sample Response
HTTP/1.1 200 OK
Connection: Keep-Alive
Signature: D5FC3608A12FA5D1EC0D79AD96EECB52987C3B80A44B9243275DAB7DD0D89EEC
Content-Length: 57
Content-Type: application/json <=
{
"poyntRequestId":"123525C7-D6A5-4FE2-BAA8-2278E10D5C5F"
}
Items on Second Screeen
This endpoint can be used to display itewms on the second screen of the device.
Headers
- Siganture - String: Sha-256 hmac of the JSON payload.
- Content-Type: text/plain: Even if the payload is a JSON object, POS Bridge parses it as a string, therefore it is important to pass content-type as “text/plain” or the authentication will fail.
Request Body
- poyntRequestId - String: UUID of the request.
- timeout - int: Client timeout in milliseconds.
- secondScreenRequest - Object: Encloses the properties below.
- items - JSON Array: Property inside
printingRequest object. This is an array of OrderItem objects which
required fields:
- name
- quantity
- status
- tax
- unifOfMeasure
- unitPrice
- currency - String: 3 character currency code which determines the currency symbol shown on the second screen.
- totalAmount - int: Amount in cents which should be calculated as the sum of items times their quantity.
Paylinks
Pay Links are a fast and versatile way to get paid online. Merchants can get up and running with payments with no setup time with a Pay Link included with their GoDaddy account. They can also event connect their domain for a more personalized experience.
Pay Links can accept wallet and credit card payments and can easily be customized to include the merchant’s brand info and request info from their customers.
Definition
GET https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks/recentSales
Sample Request
curl --location --request GET 'https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks/recentSales' \
'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json' --header
Sample Response
{
"recentSales": [
{
"amount": 1625,
"currency": null,
"date": "2023-12-04T15:50:09.000Z",
"title": "Google Pay Demo"
},
{
"amount": 1625,
"currency": null,
"date": "2023-11-30T18:05:18.000Z",
"title": "Google Pay Demo"
},
{
"amount": 1625,
"currency": null,
"date": "2023-11-29T23:26:55.000Z",
"title": "Google Pay Demo"
}
]
}
Recent Sales
This endpoint is designed to obtain the 3 most recent sales carried out through paylinks.
Headers
- ‘Authorization: Bearer {access_token}’
- ‘Content-Type: application/json’
Query Parameters
- storeId - String: This is the ID of the store for which you wish to obtain the recent sales.
Definition
GET https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks/totalSales
Sample Request
curl --location --request GET 'https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks/totalSales' \
'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json' --header
Sample Response
{
"items": {
"amount": 0,
"count": 0,
"currency": "USD"
},
"payments": {
"amount": 7075215,
"count": 180,
"currency": "USD"
},
"totalSales": {
"amount": 7075215,
"currency": "USD"
}
}
Total Sales
This endpoint is designed to obtain the total sales carried out through paylinks.
Headers
- ‘Authorization: Bearer {access_token}’
- ‘Content-Type: application/json’
Query Parameters
- storeId - String: This is the ID of the store for which you wish to obtain the total sales.
Definition
GET https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks
Sample Request
curl --location --request GET https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks \
'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json' --header
Sample Response
{
"checkoutUrls": [
{
"checkoutUrlId": "6da6d6e2-40ba-4c86-84e1-fa6aecf625f6",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Testing store id df",
"shortName": "6da6d6e2-40ba-4c86-84e1-fa6",
"description": "",
"buttonText": null,
"picture": "",
"amount": 500,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-12-19T17:04:30.000Z",
"updatedAt": "2023-12-19T17:04:30.000Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": null,
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "aa41b55b-5aff-44d6-8105-677399281c1e",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "test",
"shortName": "edy",
"description": "dfvdsvdfcvdsv",
"buttonText": null,
"picture": "",
"amount": 11111,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-12-18T13:57:32.000Z",
"updatedAt": "2023-12-18T13:57:32.000Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": null,
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "c2c7e8ca-ec1b-465a-acee-de28dc0a6712",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "New Link",
"shortName": "c2c7e8ca-ec1b-465a-acee-de2",
"description": "",
"buttonText": null,
"picture": "",
"amount": 0,
"currency": null,
"isCustomPrice": 1,
"status": "A",
"createdAt": "2023-11-29T16:18:20.000Z",
"updatedAt": "2023-11-29T16:18:20.000Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":true,\"collectBillingAddress\":true,\"isAddressRequired\":false,\"allowTips\":true}",
"productId": null,
"domain": null,
"isDefault": null,
"totalSalesAmount": 7250,
"totalSalesCount": 4
},
{
"checkoutUrlId": "f8c9fb08-b3ce-431e-87a1-3f4a20486575",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "opt shipping test",
"shortName": "f8c9fb08-b3ce-431e-87a1-3f4",
"description": "",
"buttonText": null,
"picture": "",
"amount": 1111,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-11-27T22:39:25.000Z",
"updatedAt": "2023-11-28T15:38:50.745Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":true,\"collectBillingAddress\":true,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": "null",
"isDefault": null,
"totalSalesAmount": 6789,
"totalSalesCount": 7
},
{
"checkoutUrlId": "e7604b60-0345-4003-8408-c6782c6b0e46",
"urlType": "L",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Google Pay Demo",
"shortName": "e7604b60-0345-4003-8408-c67",
"description": "",
"buttonText": null,
"picture": "",
"amount": 0,
"currency": null,
"isCustomPrice": 1,
"status": "A",
"createdAt": "2023-11-22T00:16:45.000Z",
"updatedAt": "2023-12-04T15:49:42.267Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false,\"options\":[{\"amount\":1625,\"index\":0,\"title\":\"op1\"},{\"index\":1,\"amount\":349},{\"amount\":0,\"index\":2,\"disabled\":true,\"allowCustomPrice\":true,\"title\":\"custom1\"}]}",
"productId": null,
"domain": "null",
"isDefault": null,
"totalSalesAmount": 8125,
"totalSalesCount": 5
},
{
"checkoutUrlId": "2c106020-026d-49df-9d2e-0f9ac19cb55b",
"urlType": "L",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "test amount options",
"shortName": "testing-katy22",
"description": "",
"buttonText": null,
"picture": "",
"amount": null,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-11-14T00:01:24.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false,\"options\":[{\"index\":0,\"amount\":100},{\"index\":1,\"amount\":200},{\"index\":2,\"amount\":300}]}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 200,
"totalSalesCount": 1
},
{
"checkoutUrlId": "4613c1ce-b684-4e0d-99a5-bb56e39961b5",
"urlType": "L",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "The Options Are Endless",
"shortName": "optionz",
"description": "",
"buttonText": null,
"picture": "",
"amount": null,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-11-09T23:44:56.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false,\"options\":[{\"index\":0,\"amount\":1000,\"title\":\"First Option Yo\"},{\"index\":1,\"amount\":2000,\"title\":\"Second Option Yo\"},{\"index\":2,\"amount\":3000,\"title\":\"Third Option Man\"}]}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 2000,
"totalSalesCount": 1
},
{
"checkoutUrlId": "bd80845c-f46a-4ffd-b07f-50848ef06e4b",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Apple Pay with Shipping",
"shortName": "testing-katy12",
"description": "",
"buttonText": null,
"picture": "",
"amount": 1000,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-11-08T18:38:20.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":true,\"collectBillingAddress\":true,\"isAddressRequired\":true,\"allowTips\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 26000,
"totalSalesCount": 26
},
{
"checkoutUrlId": "83ca2909-372e-4ddb-8391-309fc836a948",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "deactivate2",
"shortName": "83ca2909-372e-4ddb-8391-309",
"description": "test",
"buttonText": null,
"picture": "",
"amount": 222,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-11-01T18:15:27.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "301ffa5a-69c2-42c2-8d96-46f8dea9fe21",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "",
"shortName": "301ffa5a-69c2-42c2-8d96-46f",
"description": "",
"buttonText": null,
"picture": "",
"amount": 111,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-11-01T18:10:29.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "34116caf-6926-402e-868c-c25a6fe2e90e",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "4567t",
"shortName": "34116caf-6926-402e-868c-c25",
"description": "",
"buttonText": null,
"picture": "",
"amount": 4567,
"currency": null,
"isCustomPrice": 1,
"status": "A",
"createdAt": "2023-10-27T20:48:12.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "f7d7347d-cd8b-4070-b58d-a8ccef7aa91d",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "lol",
"shortName": "f7d7347d-cd8b-4070-b58d-a8c",
"description": "",
"buttonText": null,
"picture": "",
"amount": 1243,
"currency": null,
"isCustomPrice": 1,
"status": "A",
"createdAt": "2023-10-27T16:40:32.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "2040a827-368a-4aba-aae7-c631fcaf19af",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Test PL Smart Tips",
"shortName": "2040a827-368a-4aba-aae7-c63",
"description": "",
"buttonText": null,
"picture": "",
"amount": 2000,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-10-17T19:56:10.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":true}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "395bb4b5-56c3-429b-98ec-c09153a4ba75",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Test Paylink Tips",
"shortName": "tips",
"description": "Test Paylink Tips",
"buttonText": null,
"picture": "",
"amount": 1000,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-08-02T15:23:27.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "7863562e-2fa5-46af-8b48-c21f0cdd6fbd",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Some Link",
"shortName": "7863562e-2fa5-46af-8b48-c21",
"description": "some l",
"buttonText": null,
"picture": "",
"amount": 23333,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-05-23T21:43:29.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"isAddressRequired\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "528252de-178c-4204-b74b-833c6c6ff682",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Stuff",
"shortName": "528252de-178c-4204-b74b-833",
"description": "Mo mo ",
"buttonText": null,
"picture": "",
"amount": 9999,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-05-18T22:43:50.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"isAddressRequired\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "1f5b5d3d-75e9-497c-9a58-4e3b23d1603a",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Test",
"shortName": "1f5b5d3d-75e9-497c-9a58-4e3",
"description": "",
"buttonText": null,
"picture": "",
"amount": 400,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-05-12T19:09:50.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"isAddressRequired\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "d4f78a9f-d7f1-474e-82cd-febf0b8eab7f",
"urlType": "L",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Hel o",
"shortName": "d4f78a9f-d7f1-474e-82cd-feb",
"description": "",
"buttonText": null,
"picture": "",
"amount": null,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-04-26T22:03:24.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"isAddressRequired\":false,\"options\":[{\"index\":0,\"amount\":1000},{\"index\":1,\"amount\":250},{\"index\":2,\"amount\":100},{\"amount\":0,\"index\":3,\"disabled\":true,\"allowCustomPrice\":true}]}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 2000,
"totalSalesCount": 2
},
{
"checkoutUrlId": "f614f412-b8de-47c3-81b2-07c8e1abb8ff",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "New Link",
"shortName": "f614f412-b8de-47c3-81b2-07c",
"description": "",
"buttonText": null,
"picture": "",
"amount": 500,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-04-10T18:18:59.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"isAddressRequired\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 1000,
"totalSalesCount": 2
},
{
"checkoutUrlId": "bc465fd1-0630-4e00-a5ea-3a7cf3703219",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Test Address",
"shortName": "address",
"description": "Address",
"buttonText": null,
"picture": "",
"amount": 1212,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-04-06T17:19:14.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":true,\"isAddressRequired\":true}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "cbab28d7-c617-4dbd-86bf-673e542cd09e",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Testing Locale",
"shortName": "cbab28d7-c617-4dbd-86bf-673",
"description": "",
"buttonText": null,
"picture": "",
"amount": 123,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-04-05T21:59:13.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "0253964f-a77e-48a5-bb5d-9a2da3343241",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Test Text 2",
"shortName": "test-text-2",
"description": "",
"buttonText": null,
"picture": "",
"amount": 1000,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-02-01T20:21:22.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "336f85bf-7d3d-4809-8bbe-cb9c807a7890",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Testing Text ",
"shortName": "test-text",
"description": "",
"buttonText": null,
"picture": "",
"amount": 4000,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-02-01T20:20:51.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "765f801e-e9b0-4fa2-a3a9-db14a51ee00c",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Small Amount Validation - Required",
"shortName": "765f801e-e9b0-4fa2-a3a9-db1",
"description": "",
"buttonText": null,
"picture": "",
"amount": 0,
"currency": null,
"isCustomPrice": 1,
"status": "A",
"createdAt": "2023-01-19T17:01:41.000Z",
"updatedAt": "2023-11-27T22:39:05.988Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":true,\"collectBillingAddress\":true,\"isAddressRequired\":true,\"allowTips\":false}",
"productId": null,
"domain": "null",
"isDefault": 1,
"totalSalesAmount": 1632818,
"totalSalesCount": 30
},
{
"checkoutUrlId": "d91b4b3e-5824-4ebe-bf20-0fa6b0750710",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Frenche Ticket Dos",
"shortName": "d91b4b3e-5824-4ebe-bf20-0fa",
"description": "",
"buttonText": null,
"picture": "",
"amount": 5000,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-01-13T21:05:51.000Z",
"updatedAt": "2023-01-19T19:29:47.611Z",
"metadata": null,
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 5000,
"totalSalesCount": 1
}
]
}
Get Store Paylinks
This endpoint is designed to obtain all the paylinks created for a specific store.
Headers
- ‘Authorization: Bearer {access_token}’
- ‘Content-Type: application/json’
Query Parameters
- storeId - String: This is the ID of the store for which you wish to obtain the paylinks.
Definition
POST https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks
Sample Request
curl --location --request POST 'https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks' \
'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json'
--header
{
"urlType": "P",
"title": "string",
"shortName": "string",
"amount": 1000,
"currency": "USD",
"order": {},
"isCustomPrice": 0
}
Sample Response
{
"title": "testing",
"description": "",
"shortName": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed/f410238a-94e0-4a7b-b6a0-9d488dd7fce0",
"urlType": "P",
"picture": "",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"domain": null,
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"amount": 1000,
"isCustomPrice": 0,
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"createdAt": "2023-12-20T20:19:01.716Z",
"updatedAt": "2023-12-20T20:19:01.716Z",
"checkoutUrlId": "f410238a-94e0-4a7b-b6a0-9d488dd7fce0",
"status": "A"
}
Create a Paylink
This endpoint can be used to create a new paylink within a specific store.
Headers
- ‘Authorization: Bearer {access_token}’
- ‘Content-Type: application/json’
Query Parameters
- storeId - String: This is the ID of the store for which you wish to create a Paylink.
Request Body
- urlType - String: This is the type of Paylink you wish to create.
- title - String: This will be the displayed title for the Paylink.
- shortName - String: This will be the short version of the Paylink name.
- amount - Number: This corresponds to the associated amount for the Paylink
- currency - String: This is a 3 letter code representing the currency.
- order - JSON Object: This will be the associated order data for the Paylink.
- isCustomPrice - Number: This designates if this url is custom price.
Definition
GET https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks/all
Sample Request
curl --location --request GET https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks/all \
'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json' --header
Sample Response
{
"checkoutUrls": [
{
"checkoutUrlId": "f3348947-1775-4f2d-9877-5c5e4796eddb",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "35bd683c-884e-4bb8-b5dc-aaaa6ffa7803",
"orderText": null,
"title": "store id 35",
"shortName": "f3348947-1775-4f2d-9877-5c5",
"description": "",
"buttonText": null,
"picture": "",
"amount": 1000,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-12-19T17:05:35.000Z",
"updatedAt": "2023-12-19T17:05:35.000Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": null,
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "6da6d6e2-40ba-4c86-84e1-fa6aecf625f6",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Testing store id df",
"shortName": "6da6d6e2-40ba-4c86-84e1-fa6",
"description": "",
"buttonText": null,
"picture": "",
"amount": 500,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-12-19T17:04:30.000Z",
"updatedAt": "2023-12-19T17:04:30.000Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": null,
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "3a9ee0b9-f04d-44df-99d4-22afe4060e8c",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "35bd683c-884e-4bb8-b5dc-aaaa6ffa7803",
"orderText": null,
"title": "here we go again",
"shortName": "cjlink",
"description": "ssdvnsovn",
"buttonText": null,
"picture": "",
"amount": 222,
"currency": "USD",
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-12-18T14:13:23.000Z",
"updatedAt": "2023-12-18T14:13:23.000Z",
"metadata": "",
"productId": null,
"domain": null,
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "71282752-312f-4c94-9062-6944cce45d31",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "35bd683c-884e-4bb8-b5dc-aaaa6ffa7803",
"orderText": null,
"title": "cvbvcb",
"shortName": "vsdvsdv",
"description": "bcvbcvb",
"buttonText": null,
"picture": "",
"amount": 33333,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-12-18T14:02:36.000Z",
"updatedAt": "2023-12-18T14:02:36.000Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": null,
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "aa41b55b-5aff-44d6-8105-677399281c1e",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "test",
"shortName": "edy",
"description": "dfvdsvdfcvdsv",
"buttonText": null,
"picture": "",
"amount": 11111,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-12-18T13:57:32.000Z",
"updatedAt": "2023-12-18T13:57:32.000Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": null,
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "c2c7e8ca-ec1b-465a-acee-de28dc0a6712",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "New Link",
"shortName": "c2c7e8ca-ec1b-465a-acee-de2",
"description": "",
"buttonText": null,
"picture": "",
"amount": 0,
"currency": null,
"isCustomPrice": 1,
"status": "A",
"createdAt": "2023-11-29T16:18:20.000Z",
"updatedAt": "2023-11-29T16:18:20.000Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":true,\"collectBillingAddress\":true,\"isAddressRequired\":false,\"allowTips\":true}",
"productId": null,
"domain": null,
"isDefault": null,
"totalSalesAmount": 7250,
"totalSalesCount": 4
},
{
"checkoutUrlId": "f8c9fb08-b3ce-431e-87a1-3f4a20486575",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "opt shipping test",
"shortName": "f8c9fb08-b3ce-431e-87a1-3f4",
"description": "",
"buttonText": null,
"picture": "",
"amount": 1111,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-11-27T22:39:25.000Z",
"updatedAt": "2023-11-28T15:38:50.745Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":true,\"collectBillingAddress\":true,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": "null",
"isDefault": null,
"totalSalesAmount": 6789,
"totalSalesCount": 7
},
{
"checkoutUrlId": "e7604b60-0345-4003-8408-c6782c6b0e46",
"urlType": "L",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Google Pay Demo",
"shortName": "e7604b60-0345-4003-8408-c67",
"description": "",
"buttonText": null,
"picture": "",
"amount": 0,
"currency": null,
"isCustomPrice": 1,
"status": "A",
"createdAt": "2023-11-22T00:16:45.000Z",
"updatedAt": "2023-12-04T15:49:42.267Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false,\"options\":[{\"amount\":1625,\"index\":0,\"title\":\"op1\"},{\"index\":1,\"amount\":349},{\"amount\":0,\"index\":2,\"disabled\":true,\"allowCustomPrice\":true,\"title\":\"custom1\"}]}",
"productId": null,
"domain": "null",
"isDefault": null,
"totalSalesAmount": 8125,
"totalSalesCount": 5
},
{
"checkoutUrlId": "2c106020-026d-49df-9d2e-0f9ac19cb55b",
"urlType": "L",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "test amount options",
"shortName": "testing-katy22",
"description": "",
"buttonText": null,
"picture": "",
"amount": null,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-11-14T00:01:24.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false,\"options\":[{\"index\":0,\"amount\":100},{\"index\":1,\"amount\":200},{\"index\":2,\"amount\":300}]}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 200,
"totalSalesCount": 1
},
{
"checkoutUrlId": "4613c1ce-b684-4e0d-99a5-bb56e39961b5",
"urlType": "L",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "The Options Are Endless",
"shortName": "optionz",
"description": "",
"buttonText": null,
"picture": "",
"amount": null,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-11-09T23:44:56.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false,\"options\":[{\"index\":0,\"amount\":1000,\"title\":\"First Option Yo\"},{\"index\":1,\"amount\":2000,\"title\":\"Second Option Yo\"},{\"index\":2,\"amount\":3000,\"title\":\"Third Option Man\"}]}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 2000,
"totalSalesCount": 1
},
{
"checkoutUrlId": "bd80845c-f46a-4ffd-b07f-50848ef06e4b",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Apple Pay with Shipping",
"shortName": "testing-katy12",
"description": "",
"buttonText": null,
"picture": "",
"amount": 1000,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-11-08T18:38:20.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":true,\"collectBillingAddress\":true,\"isAddressRequired\":true,\"allowTips\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 26000,
"totalSalesCount": 26
},
{
"checkoutUrlId": "c7822712-b75f-4ff5-bfd1-8dd6b6d1cc5a",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "35bd683c-884e-4bb8-b5dc-aaaa6ffa7803",
"orderText": null,
"title": "",
"shortName": "katy-123",
"description": "",
"buttonText": null,
"picture": "",
"amount": 1000,
"currency": null,
"isCustomPrice": 0,
"status": "D",
"createdAt": "2023-11-02T19:04:16.000Z",
"updatedAt": "2023-11-02T19:04:50.030Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": null,
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "83ca2909-372e-4ddb-8391-309fc836a948",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "deactivate2",
"shortName": "83ca2909-372e-4ddb-8391-309",
"description": "test",
"buttonText": null,
"picture": "",
"amount": 222,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-11-01T18:15:27.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "301ffa5a-69c2-42c2-8d96-46f8dea9fe21",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "",
"shortName": "301ffa5a-69c2-42c2-8d96-46f",
"description": "",
"buttonText": null,
"picture": "",
"amount": 111,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-11-01T18:10:29.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "34116caf-6926-402e-868c-c25a6fe2e90e",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "4567t",
"shortName": "34116caf-6926-402e-868c-c25",
"description": "",
"buttonText": null,
"picture": "",
"amount": 4567,
"currency": null,
"isCustomPrice": 1,
"status": "A",
"createdAt": "2023-10-27T20:48:12.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "f7d7347d-cd8b-4070-b58d-a8ccef7aa91d",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "lol",
"shortName": "f7d7347d-cd8b-4070-b58d-a8c",
"description": "",
"buttonText": null,
"picture": "",
"amount": 1243,
"currency": null,
"isCustomPrice": 1,
"status": "A",
"createdAt": "2023-10-27T16:40:32.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "2040a827-368a-4aba-aae7-c631fcaf19af",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Test PL Smart Tips",
"shortName": "2040a827-368a-4aba-aae7-c63",
"description": "",
"buttonText": null,
"picture": "",
"amount": 2000,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-10-17T19:56:10.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":true}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "395bb4b5-56c3-429b-98ec-c09153a4ba75",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Test Paylink Tips",
"shortName": "tips",
"description": "Test Paylink Tips",
"buttonText": null,
"picture": "",
"amount": 1000,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-08-02T15:23:27.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "7863562e-2fa5-46af-8b48-c21f0cdd6fbd",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Some Link",
"shortName": "7863562e-2fa5-46af-8b48-c21",
"description": "some l",
"buttonText": null,
"picture": "",
"amount": 23333,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-05-23T21:43:29.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"isAddressRequired\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "528252de-178c-4204-b74b-833c6c6ff682",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Stuff",
"shortName": "528252de-178c-4204-b74b-833",
"description": "Mo mo ",
"buttonText": null,
"picture": "",
"amount": 9999,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-05-18T22:43:50.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"isAddressRequired\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "1f5b5d3d-75e9-497c-9a58-4e3b23d1603a",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Test",
"shortName": "1f5b5d3d-75e9-497c-9a58-4e3",
"description": "",
"buttonText": null,
"picture": "",
"amount": 400,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-05-12T19:09:50.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"isAddressRequired\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "d4f78a9f-d7f1-474e-82cd-febf0b8eab7f",
"urlType": "L",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Hel o",
"shortName": "d4f78a9f-d7f1-474e-82cd-feb",
"description": "",
"buttonText": null,
"picture": "",
"amount": null,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-04-26T22:03:24.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"isAddressRequired\":false,\"options\":[{\"index\":0,\"amount\":1000},{\"index\":1,\"amount\":250},{\"index\":2,\"amount\":100},{\"amount\":0,\"index\":3,\"disabled\":true,\"allowCustomPrice\":true}]}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 2000,
"totalSalesCount": 2
},
{
"checkoutUrlId": "f614f412-b8de-47c3-81b2-07c8e1abb8ff",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "New Link",
"shortName": "f614f412-b8de-47c3-81b2-07c",
"description": "",
"buttonText": null,
"picture": "",
"amount": 500,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-04-10T18:18:59.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"isAddressRequired\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 1000,
"totalSalesCount": 2
},
{
"checkoutUrlId": "bc465fd1-0630-4e00-a5ea-3a7cf3703219",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Test Address",
"shortName": "address",
"description": "Address",
"buttonText": null,
"picture": "",
"amount": 1212,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-04-06T17:19:14.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":true,\"isAddressRequired\":true}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
},
{
"checkoutUrlId": "cbab28d7-c617-4dbd-86bf-673e542cd09e",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "Testing Locale",
"shortName": "cbab28d7-c617-4dbd-86bf-673",
"description": "",
"buttonText": null,
"picture": "",
"amount": 123,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-04-05T21:59:13.000Z",
"updatedAt": "2023-11-17T20:11:43.826Z",
"metadata": "{\"isNotesRequired\":false}",
"productId": null,
"domain": "",
"isDefault": null,
"totalSalesAmount": 0,
"totalSalesCount": 0
}
]
}
Get All Paylinks
This endpoint is designed to obtain all the paylinks related to a specific business.
Headers
- ‘Authorization: Bearer {access_token}’
- ‘Content-Type: application/json’
Query Parameters
- storeId - String: This is the ID of the store for which you wish to obtain all paylinks.
Request Body
- page - Number: This value defaults to 1.
- pageSize - Number: This value defaults to 25.
Definition
GET https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks/{id}
Sample Request
curl --location --request GET 'https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks/{id}' \
'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json' --header
Sample Response
{
"checkoutUrlId": "f3348947-1775-4f2d-9877-5c5e4796eddb",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "35bd683c-884e-4bb8-b5dc-aaaa6ffa7803",
"orderText": null,
"title": "store id 35",
"shortName": "f3348947-1775-4f2d-9877-5c5",
"description": "",
"buttonText": null,
"picture": "",
"amount": 1000,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-12-19T17:05:35.000Z",
"updatedAt": "2023-12-19T17:05:35.000Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": null,
"isDefault": null
}
Get a Paylink by ID
You can use this endpoint to obtain a specific paylink using the paylink ID.
Headers
- ‘Authorization: Bearer {access_token}’
- ‘Content-Type: application/json’
Query Parameters
- storeId - String: This is the ID of the store for which you wish to obtain the paylink.
- id - String: This value corresponds to the paylink ID.
Definition
PUT https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks/{id}
Sample Request
curl --location --request PUT 'https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks/{id}' \
'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json'
--header
{
"urlType": "P",
"title": "string",
"shortName": "string",
"amount": 1000,
"currency": "USD",
"order": {},
"isCustomPrice": 0
}
Sample Response
{
"checkoutUrlId": "f410238a-94e0-4a7b-b6a0-9d488dd7fce0",
"urlType": "P",
"businessId": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed",
"storeId": "df555b6c-97f8-42de-8f4e-3f1a370f7989",
"orderText": null,
"title": "testing",
"shortName": "39a4fbf1-1c0d-4061-ae9a-26cced6d08ed/f410238a-94e0-4a7b-b6a0-9d4",
"description": "",
"buttonText": null,
"picture": "",
"amount": 1100,
"currency": null,
"isCustomPrice": 0,
"status": "A",
"createdAt": "2023-12-20T20:19:01.000Z",
"updatedAt": "2023-12-20T20:22:01.147Z",
"metadata": "{\"isNotesRequired\":false,\"collectShippingAddress\":false,\"collectBillingAddress\":false,\"isAddressRequired\":false,\"allowTips\":false}",
"productId": null,
"domain": null,
"isDefault": null
}
Update Paylink by ID
This endpoint can be used to edit and update the information related to a specific paylink.
Headers
- ‘Authorization: Bearer {access_token}’
- ‘Content-Type: application/json’
Query Parameters
- storeId - String: This is the ID of the store for which you wish to create a Paylink.
- id - String: This value is the ID of the paylink to be updated.
Request Body
- urlType - String: This is the type of Paylink you wish to create.
- title - String: This will be the displayed title for the Paylink.
- shortName - String: This will be the short version of the Paylink name.
- amount - Number: This corresponds to the associated amount for the Paylink
- currency - String: This is a 3 letter code representing the currency.
- order - JSON Object: This will be the associated order data for the Paylink.
- isCustomPrice - Number: This designates if this url is custom price.
Definition
DELETE https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks/{id}
Sample Request
curl --location --request DELETE 'https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks/{id}' \
'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json' --header
Sample Response
200 OK
Delete a Paylink by ID
You can use this endpoint to delete a specific paylink from the reecords using the paylink ID.
Headers
- ‘Authorization: Bearer {access_token}’
- ‘Content-Type: application/json’
Query Parameters
- storeId - String: This is the ID of the store for which you wish to delete the paylink.
- id - String: This value corresponds to ID of the paylink you wish to delete.
Definition
POST https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks/oneTime
Sample Request
curl --location --request POST 'https://poynt.godaddy.com/api/v2/stores/{storeId}/payLinks/oneTime' \
'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json'
--header
{
"title": "string",
"description": "string",
"amount": 1000,
"currency": "USD",
"txnId": "string",
"order": {},
"expiry": 3700
}
Sample Response
{
"expires": "2021-06-11T21:53:15.349Z",
"businessId": "aab99d15-d2da-41f9-a2b3-19c8a8eb1463",
"orderId": "6f811805-2f5b-40cd-bd14-bbeb830f5a62",
"txnId": "f7e24528-af7b-4f24-b831-db3cb328bd16",
"url": "https://poynt.godaddy.com/checkout/76e8fb61-d1e6-488c-9784-adc5ea23bb47/onetime-6f811805-2f5b-40cd-bd14-bbeb830f5a62",
"qrUrl": "https://poynt.godaddy.com/qr/png?data=https%3A%2F%2Fpoynt.godaddy.com%2Fcheckout%2F76e8fb61-d1e6-488c-9784-adc5ea23bb47%2Fonetime-6f811805-2f5b-40cd-bd14-bbeb830f5a62&size=10"
}
Create a One-time Paylink
You can use this endpoint to create a paylink that will only be used once.
Headers
- ‘Authorization: Bearer {access_token}’
- ‘Content-Type: application/json’
Query Parameters
- storeId - String: This is the ID of the store for which you wish to create a Paylink.
Request Body
- title - String: This will be the displayed title for the Paylink.
- description - String: This is the Paylink description summary.
- amount - Number: This corresponds to the associated amount for the Paylink
- currency - String: This is a 3 letter code representing the currency.
- txnId - String: This is the optional UUID to use for the transaction.
- order - JSON Object: This will be the associated order data for the Paylink.
- expiry - Number: This designates the expiration time in seconds. It will default to 3600 (1 hour)
Definition
GET https://poynt.godaddy.com/api/v2/paylink/{domain}
Sample Request
curl --location --request GET 'https://poynt.godaddy.com/api/v2/paylink/{domain}' \
'Authorization: Bearer {access_token}' \
--header 'Content-Type: application/json' --header
Sample Response
{
"checkoutUrlId": "663f6ca8-4917-47a9-b140-e7fa519d31e4",
"urlType": "P",
"businessId": "2fb8d28c-0b2a-451d-abd7-8da57d3ebf67",
"storeId": "604a0499-c6b8-4144-ae32-2d0352745234",
"orderText": null,
"title": "Online payment",
"shortName": "2ebcd5ff-a2fb-445c-8950-636",
"description": "Please enter any details in the notes section",
"buttonText": null,
"picture": "",
"amount": 0,
"currency": null,
"isCustomPrice": 1,
"status": "A",
"createdAt": "2023-07-14T21:41:03.000Z",
"updatedAt": "2023-10-26T15:16:53.694Z",
"metadata": null,
"productId": null,
"domain": "pay.test-shop.co",
"isDefault": 1
}
Get Paylink by Domain
You can use this endpoint to fetch a paylink by using the business domain.
Headers
- ‘Authorization: Bearer {access_token}’
- ‘Content-Type: application/json’
Query Parameters
- domain - String: This value corresponds to the business domain.
AVSResult
Attributes
actualResult
- string
addressResult
- string, enum['MATCH', 'NO_MATCH', 'PARTIAL_MATCH', 'NOT_PROVIDED', 'ISSUER_NOT_CERTIFIED', 'NO_RESPONSE_FROM_CARD_ASSOCIATION', 'UNKNOWN_RESPONSE_FROM_CARD_ASSOCIATION', 'NOT_VERIFIED', 'BAD_FORMAT', 'ERROR', 'UNSUPPORTED_BY_ISSUER', 'UNAVAILABLE', 'ADDRESS_AND_ZIP_MATCH']
cityResult
- string, enum['MATCH', 'NO_MATCH', 'PARTIAL_MATCH', 'NOT_PROVIDED', 'ISSUER_NOT_CERTIFIED', 'NO_RESPONSE_FROM_CARD_ASSOCIATION', 'UNKNOWN_RESPONSE_FROM_CARD_ASSOCIATION', 'NOT_VERIFIED', 'BAD_FORMAT', 'ERROR', 'UNSUPPORTED_BY_ISSUER', 'UNAVAILABLE', 'ADDRESS_AND_ZIP_MATCH']
stateResult
- string, enum['MATCH', 'NO_MATCH', 'PARTIAL_MATCH', 'NOT_PROVIDED', 'ISSUER_NOT_CERTIFIED', 'NO_RESPONSE_FROM_CARD_ASSOCIATION', 'UNKNOWN_RESPONSE_FROM_CARD_ASSOCIATION', 'NOT_VERIFIED', 'BAD_FORMAT', 'ERROR', 'UNSUPPORTED_BY_ISSUER', 'UNAVAILABLE', 'ADDRESS_AND_ZIP_MATCH']
countryResult
- string, enum['MATCH', 'NO_MATCH', 'PARTIAL_MATCH', 'NOT_PROVIDED', 'ISSUER_NOT_CERTIFIED', 'NO_RESPONSE_FROM_CARD_ASSOCIATION', 'UNKNOWN_RESPONSE_FROM_CARD_ASSOCIATION', 'NOT_VERIFIED', 'BAD_FORMAT', 'ERROR', 'UNSUPPORTED_BY_ISSUER', 'UNAVAILABLE', 'ADDRESS_AND_ZIP_MATCH']
phoneResult
- string, enum['MATCH', 'NO_MATCH', 'PARTIAL_MATCH', 'NOT_PROVIDED', 'ISSUER_NOT_CERTIFIED', 'NO_RESPONSE_FROM_CARD_ASSOCIATION', 'UNKNOWN_RESPONSE_FROM_CARD_ASSOCIATION', 'NOT_VERIFIED', 'BAD_FORMAT', 'ERROR', 'UNSUPPORTED_BY_ISSUER', 'UNAVAILABLE', 'ADDRESS_AND_ZIP_MATCH']
postalCodeResult
- string, enum['MATCH', 'NO_MATCH', 'PARTIAL_MATCH', 'NOT_PROVIDED', 'ISSUER_NOT_CERTIFIED', 'NO_RESPONSE_FROM_CARD_ASSOCIATION', 'UNKNOWN_RESPONSE_FROM_CARD_ASSOCIATION', 'NOT_VERIFIED', 'BAD_FORMAT', 'ERROR', 'UNSUPPORTED_BY_ISSUER', 'UNAVAILABLE', 'ADDRESS_AND_ZIP_MATCH']
cardHolderNameResult
- string, enum['MATCH', 'NO_MATCH', 'PARTIAL_MATCH', 'NOT_PROVIDED', 'ISSUER_NOT_CERTIFIED', 'NO_RESPONSE_FROM_CARD_ASSOCIATION', 'UNKNOWN_RESPONSE_FROM_CARD_ASSOCIATION', 'NOT_VERIFIED', 'BAD_FORMAT', 'ERROR', 'UNSUPPORTED_BY_ISSUER', 'UNAVAILABLE', 'ADDRESS_AND_ZIP_MATCH']
ActiveTime
Attributes
startHour
- integer
endHour
- integer
repeatType
- string, enum['DAILY', 'WEEKLY', 'MONTHLY']
every
- array [long]
startAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ endAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ
Address
Attributes
postalCodeExtension
- string
city
- string
countryCode
- string
line1
- string
line2
- string
postalCode
- string
territory
- string
territoryType
- string, enum['STATE', 'PROVINCE', 'OTHER']
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ status
- string, enum['ADDED']
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ primary
- boolean
id
- integer
type
- string, enum['HOME', 'WORK', 'BUSINESS', 'TRANSACTION', 'OTHER']
AdjustedDiscount
Attributes
adjustmentType
- string, enum['ADDED', 'REMOVED', 'MODIFIED']
customName
- string
amount
- integer
id
- string
AdjustedFee
Attributes
adjustmentType
- string, enum['ADDED', 'REMOVED', 'MODIFIED']
idStr
- string
amount
- integer
name
- string
id
- integer
AdjustedOrder
Attributes
adjustedFees
- array [AdjustedFee]
adjustedDiscounts
- array [AdjustedDiscount]
adjustedOrderItems
- array [AdjustedOrderItem]
AdjustedOrderItem
Attributes
adjustedFees
- array [AdjustedFee]
adjustedDiscounts
- array [AdjustedDiscount]
adjustmentType
- string, enum['ADDED', 'REMOVED', 'MODIFIED']
adjustedOrderItemTaxes
- array [AdjustedOrderItemTax]
adjustedVariants
- array [AdjustedVariant]
unitPrice
- integer
quantity
- float
name
- string
id
- integer
AdjustedOrderItemTax
Attributes
adjustmentType
- string, enum['ADDED', 'REMOVED', 'MODIFIED']
amountPrecision
- integer
amount
- integer
id
- string
AdjustedVariant
Attributes
adjustmentType
- string, enum['ADDED', 'REMOVED', 'MODIFIED']
amount
- integer
value
- string
attribute
- string
AdjustmentRecord
Attributes
sequence
- integer
amountChanges
- TransactionAmounts
systemTraceAuditNumber
- string
signatureCaptured
- boolean
reason
- TransactionReason
amounts
- TransactionAmounts
processorResponse
- ProcessorResponse
signature
- array [byte]
exchangeRate
- ExchangeRate
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ transactionNumber
- string
id
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4.
AdjustTransactionRequest
Attributes
notes
- string
receiptPhone
- Phone
receiptEmailAddress
- string
reason
- TransactionReason
amounts
- TransactionAmounts
context
- ClientContext
signature
- array [byte]
references
- array [TransactionReference]
emvData
- EMVData
adjustmentUpdates
- array [AdjustmentRecord]
customerLanguage
- string
ApplePayRegistrationRequest
Attributes
merchantName
- string
merchantUrl
- string
reason
- string
registerDomains
- array [string]
unregisterDomains
- array [string]
ApplePayRegistrationResponse
Attributes
merchantName
- string
merchantUrl
- string
domains
- array [string]
AvailableDiscount
Attributes
when
- ActiveTime
fixed
- integer
percentage
- float
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ businessId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. code
- string
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ id
- string
type
- string, enum['FIXED', 'PERCENTAGE']
scope
- string, enum['ITEM', 'ORDER']
BankAccount
Attributes
routingNumber
- string
bankName
- string
country
- string
accountNumber
- string
ownerName
- string
accountType
- string, enum['CHECKING', 'SAVINGS']
accountHolderType
- string, enum['PERSONAL', 'BUSINESS']
accountNumberLastFour
- string
id
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. currency
- string
Business
Attributes
businessUrl
- string
logoUrl
- string
stores
- array [Store]
domicileCountry
- string
externalMerchantId
- string
closingReason
- string, enum['TERMINATED', 'CHURNED', 'DUPLICATE']
closingDetails
- string
closedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ legalName
- string
doingBusinessAs
- string
emailAddress
- string
phone
- Phone
mcc
- string
acquirer
- string, enum['CHASE_PAYMENTECH', 'REDE', 'EVO', 'FIRST_DATA', 'GLOBAL_PAYMENTS', 'HEARTLAND_PAYMENT_SYSTEM', 'ELAVON', 'MERCURY', 'MONERIS', 'PAYPAL', 'ELAVON_MX', 'STRIPE', 'TSYS', 'VANTIV', 'WORLDPAY', 'EPX', 'WEPAY', 'MASHREQ', 'AXIS', 'KARTUKU', 'NEXI', 'DANA', 'MYNT', 'POYNT', 'NUVEI', 'NPAY', 'BRIDGEPAY', 'CONVERGE', 'MOCK', 'NA_BANCARD', 'CREDITCALL', 'ELAVON_EU', 'FUSEBOX', 'EVERTEC', 'GHL', 'RS2', 'JCN', 'PRISMA', 'VANTIV_EXPRESS', 'EZETAP', 'ADYEN', 'LETGO']
industryType
- string
timezone
- string
organizationId
- string
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ echeckProcessor
- string, enum['MOCK', 'CHECK_COMMERCE']
activeSince
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ sic
- string
underwritingLevel
- string
processorData
- object
status
- string, enum['ADDED', 'ACTIVATED', 'LOCKED', 'CLOSED']
processor
- string, enum['CHASE_PAYMENTECH', 'REDE', 'EVO', 'FIRST_DATA', 'GLOBAL_PAYMENTS', 'HEARTLAND_PAYMENT_SYSTEM', 'ELAVON', 'MERCURY', 'MONERIS', 'PAYPAL', 'ELAVON_MX', 'STRIPE', 'TSYS', 'VANTIV', 'WORLDPAY', 'EPX', 'WEPAY', 'MASHREQ', 'AXIS', 'KARTUKU', 'NEXI', 'DANA', 'MYNT', 'POYNT', 'NUVEI', 'NPAY', 'BRIDGEPAY', 'CONVERGE', 'MOCK', 'NA_BANCARD', 'CREDITCALL', 'ELAVON_EU', 'FUSEBOX', 'EVERTEC', 'GHL', 'RS2', 'JCN', 'PRISMA', 'VANTIV_EXPRESS', 'EZETAP', 'ADYEN', 'LETGO']
subscribedBundles
- array [BundledFeatures]
supportedCardProducts
- array [string]
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ id
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. type
- string, enum['MERCHANT', 'TEST_MERCHANT', 'DEVELOPER', 'DISTRIBUTOR', 'ORGANIZATION']
address
- Address
attributes
- object
description
- string
BusinessUser
Attributes
credentials
- array [UserCredential]
nickName
- string
firstName
- string
lastName
- string
startDate
- integer
endDate
- integer
cards
- array [Card]
emailSignupCode
- string
middleInitial
- string
middleName
- string
userId
- integer
email
- string
businessId
- string
status
- string, enum['EMPLOYED', 'TERMINATED']
employmentDetails
- EmploymentDetails
CancelRequest
Attributes
cancelReason
- string, enum['TIMEOUT', 'CARD_REMOVED', 'CHIP_DECLINE_AFTER_HOST_APPROVAL', 'PIN_PAD_NOT_AVAILABLE', 'MERCHANT_CANCELLED']
context
- ClientContext
emvData
- EMVData
Card
Attributes
cardAgreement
- CardAgreement
cardId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. encrypted
- boolean
cardHolderFullName
- string
cardHolderLastName
- string
cardHolderFirstName
- string
numberHashed
- string
numberFirst6
- string
numberLast4
- string
numberMasked
- string
number
- string
track1data
- string
track2data
- string
track3data
- string
keySerialNumber
- string
expirationYear
- integer
expirationMonth
- integer
expirationDate
- integer
serviceCode
- string
cardBrand
- CardBrand
status
- string, enum['ACTIVE', 'REMOVED']
encryptedExpirationDate
- string
sequenceNumber
- string
issuer
- string
id
- integer
key
- array [CardKeyData]
source
- string, enum['DIRECT', 'APPLE_PAY', 'PAZE', 'GOOGLE_PAY']
currency
- string
type
- string, enum['AMERICAN_EXPRESS', 'EBT', 'BANCOMAT', 'DISCOVER', 'MAESTRO', 'GOPAY', 'DINERS_CLUB', 'JCB', 'ALIPAY', 'MASTERCARD', 'DANKORT', 'OTHER', 'PAYPAL', 'INTERAC', 'UNIONPAY', 'VISA']
CardAgreement
Attributes
agreedOn
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ declinedOn
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ cardId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. txnId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. email
- string
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ businessId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. status
- string
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ metadata
- CardAgreementMetadata
version
- integer
id
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4.
CardBrand
Attributes
brand
- string
issuerBank
- string
logoUrl
- string
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ scheme
- string
displayName
- string
id
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4.
CardKeyData
Attributes
version
- string
id
- string, enum['WCK', 'WAEK', 'WMACK']
Catalog
Attributes
categories
- array [Category]
products
- array [CatalogItem]
taxes
- array [Tax]
createdAt
- string
businessId
- string
storeId
- string
updatedAt
- string
displayMetadata
- array [CatalogDisplayMetadata]
restrictions
- array [Restriction]
priceBookId
- string
availableDiscounts
- array [AvailableDiscount]
appliedRestrictions
- array [string]
id
- string
name
- string
CatalogItem
Attributes
taxes
- array [Tax]
externalId
- string
displayOrder
- integer
availableDiscounts
- array [AvailableDiscount]
id
- string
color
- string
CatalogItemWithProduct
Attributes
availableDiscounts
- array [AvailableDiscount]
taxes
- array [Tax]
product
- Product
displayOrder
- integer
color
- string
CatalogList
Attributes
CatalogWithProduct
Attributes
availableDiscounts
- array [AvailableDiscount]
products
- array [CatalogItemWithProduct]
taxes
- array [Tax]
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ categories
- array [CategoryWithProduct]
businessId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. storeId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. displayMetadata
- array [CatalogDisplayMetadata]
restrictions
- array [Restriction]
appliedRestrictions
- array [string]
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ name
- string
id
- string
Category
Attributes
products
- array [CatalogItem]
taxes
- array [Tax]
createdAt
- string
storeId
- string
updatedAt
- string
parentCategoryId
- string
externalParentCategoryId
- string
externalCategoryId
- string
displayOrder
- integer
availableDiscounts
- array [AvailableDiscount]
shortCode
- string
id
- string
source
- string
name
- string
color
- string
CategoryList
Attributes
CategoryWithProduct
Attributes
availableDiscounts
- array [AvailableDiscount]
parentCategoryId
- string
products
- array [CatalogItemWithProduct]
taxes
- array [Tax]
shortCode
- string
displayOrder
- integer
color
- string
name
- string
id
- string
ClientContext
Attributes
acquirerId
- string
channelId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. storeDeviceId
- string
mid
- string
tid
- string
transmissionAtLocal
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ transactionInstruction
- string, enum['NONE', 'EXTERNALLY_PROCESSED', 'ONLINE_AUTH_REQUIRED']
employeeUserId
- integer
mcc
- string
businessType
- string, enum['MERCHANT', 'TEST_MERCHANT', 'DEVELOPER', 'DISTRIBUTOR', 'ORGANIZATION']
sourceApp
- string
storeAddressCity
- string
storeAddressTerritory
- string
storeTimezone
- string
businessId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. storeId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. source
- string, enum['INSTORE', 'WEB', 'MOBILE', 'CALLIN', 'CATALOG']
CloudMessage
Attributes
serialNum
- string
collapseKey
- string
sender
- string
recipient
- ComponentName
ttl
- integer
businessId
- string
storeId
- string
deviceId
- string
channelIds
- array [UUID]
id
- string
data
- string
ComponentName
Attributes
packageName
- string
className
- string
CurrencyAmount
Attributes
amount
- integer
currency
- string
Customer
Attributes
businessPreferences
- CustomerBusinessPreferences
nickName
- string
userIdentities
- array [UserIdentity]
middleName
- string
middleInitial
- string
firstName
- string
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ loyaltyCustomers
- array [LoyaltyCustomer]
emails
- array [Entry]
phones
- array [Entry]
addresses
- array [Entry]
insights
- CustomerInsights
cards
- array [Card]
businessId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. devices
- array [Device]
lastName
- string
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ id
- integer
attributes
- object
CustomerBusinessPreferences
Attributes
preferredCardId
- integer
CustomerInsights
Attributes
lifetimeSpend
- array [CurrencyAmount]
scores
- array [CustomerScore]
topItems
- array [CustomerTopItem]
totalOrders
- integer
since
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ poyntLoyalty
- PoyntLoyalty
CustomerList
Attributes
CustomerScore
Attributes
score
- double
type
- string, enum['LOYALTY', 'VALUE', 'OVERALL']
CustomerTopItem
Attributes
firstPurchasedAt
- integer
lastPurchasedAt
- integer
variationId
- integer
countUnit
- string, enum['EACH', 'HOURS', 'DAYS', 'SECONDS', 'CRATE_OF_12', 'SIX_PACH', 'GALLON', 'LITRE', 'INCH', 'FOOT', 'MILLIMETER', 'CENTIMETER', 'METER', 'SQUARE_METER', 'CUBIC_METER', 'GRAM', 'KILOGRAM', 'POUND', 'ANNUAL', 'DEGREE_CELCIUS', 'DEGREE_FARENHEIT']
productId
- integer
name
- string
count
- double
CustomFundingSource
Attributes
accountId
- string
processor
- string
description
- string
provider
- string
name
- string
type
- string, enum['GIFT_CARD', 'BITCOIN', 'CHEQUE', 'VOUCHER', 'REWARD', 'COUPON', 'GIFT_CERTIFICATE', 'QR_CODE', 'OTHER', 'ALIPAY', 'DANA', 'WALLET']
DebitEBTReEntry
Attributes
origRetrievalRefNumber
- string
origApprovalCode
- string
origAuthSourceCode
- string
origResponseCode
- string
origTransactionId
- string
origTransactionNumber
- string
origCreatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ origNetworkId
- string
origTraceNumber
- string
Delivery
Attributes
secret
- string
deliveryUrl
- string
links
- array [Link]
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ resourceId
- string
deviceId
- string
businessId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. storeId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. status
- string, enum['SCHEDULED', 'RESCHEDULED', 'ERRORED_RETRYING', 'DELIVERED', 'ERRORED']
attempt
- integer
hookId
- string
merchantType
- string
applicationId
- string
eventType
- string
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ resource
- string
properties
- object
id
- string
DeliveryList
Attributes
Device
Attributes
deviceType
- string, enum['MOBILE', 'UNKNOWN']
macAddresses
- array [DeviceMacAddress]
id
- integer
DeviceMacAddress
Attributes
networkInterface
- string, enum['BLUETOOTH', 'WIFI']
macAddress
- string
id
- integer
Discount
Attributes
percentage
- float
customName
- string
externalId
- string
amount
- integer
processorResponse
- ProcessorResponse
processor
- string
id
- string
provider
- string
EBTDetails
Attributes
electronicVoucherApprovalCode
- string
electronicVoucherSerialNumber
- string
type
- string, enum['CASH_BENEFIT', 'CASH_BENEFIT_CASH_WITHDRAWAL', 'FOOD_STAMP', 'FOOD_STAMP_ELECTRONIC_VOUCHER']
EMVData
Attributes
emvTags
- object
EmploymentDetails
Attributes
customRole
- CustomRole
endAt
- integer
startAt
- integer
role
- string, enum['OWNER', 'MANAGER', 'EMPLOYEE', 'CUSTOM']
ExchangeRate
Attributes
markupInfo1
- string
markupInfo2
- string
disclaimer
- string
cardTipAmount
- integer
markupPercentage
- string
requestedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ txnAmount
- integer
txnCurrency
- string
rate
- integer
ratePrecision
- integer
cardCurrency
- string
cardAmount
- integer
signature
- string
tipAmount
- integer
businessId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. provider
- string
Fee
Attributes
percentage
- float
idStr
- string
externalId
- string
amount
- integer
name
- string
id
- integer
FundingSource
Attributes
tokenize
- boolean
interacMacKeySerialNumber
- string
paymentTokenSource
- string
bankAccount
- BankAccount
paymentToken
- string
nonce
- string
interacMac
- string
cardToken
- string
verificationData
- VerificationData
customFundingSource
- CustomFundingSource
debitEBTReEntryDetails
- DebitEBTReEntry
card
- Card
debit
- boolean
accountType
- string, enum['EBT', 'CHECKING', 'SAVINGS']
ebtDetails
- EBTDetails
emvData
- EMVData
entryDetails
- FundingSourceEntryDetails
exchangeRate
- ExchangeRate
type
- string, enum['CHEQUE', 'CUSTOM_FUNDING_SOURCE', 'CREDIT_DEBIT', 'CASH']
FundingSourceEntryDetails
Attributes
iccFallback
- boolean
customerPresenceStatus
- string, enum['PRESENT', 'MOTO', 'ECOMMERCE', 'ARU', 'INVOICING', 'VIRTUAL_TERMINAL_PRESENT', 'VIRTUAL_TERMINAL_NOT_PRESENT']
entryMode
- string, enum['KEYED', 'TRACK_DATA_FROM_MAGSTRIPE', 'CONTACTLESS_MAGSTRIPE', 'INTEGRATED_CIRCUIT_CARD', 'CONTACTLESS_INTEGRATED_CIRCUIT_CARD']
Hook
Attributes
secret
- string
deliveryUrl
- string
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ businessId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. applicationId
- string
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ id
- string
eventTypes
- array [string]
HookList
Attributes
Inventory
Attributes
availableCount
- float
reOrderLevel
- float
reOrderPoint
- float
stockCount
- float
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ storeId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ
InventorySummary
Attributes
productName
- string
availableCount
- float
reOrderLevel
- float
reOrderPoint
- float
stockCount
- float
productId
- string
sku
- string
storeId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. productShortCode
- string
InventorySummaryList
Attributes
items
- array [InventorySummary]
links
- array [Link]
JsonPatch
Attributes
Link
Attributes
rel
- string
href
- string
method
- string
LoyaltyCustomer
Attributes
providerVerification
- ProviderVerification
firstName
- string
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ businessId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. lastName
- string
identifiers
- array [Entry]
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ id
- string
provider
- string
Order
Attributes
discounts
- array [Discount]
notes
- string
orderHistories
- array [OrderHistory]
orderShipments
- array [OrderShipment]
items
- array [OrderItem]
customerUserId
- integer
externalId
- string
links
- array [Link]
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ fees
- array [Fee]
amounts
- OrderAmounts
stayType
- string, enum['GENERAL_CONTAINER', 'REGULAR_STAY', 'QUICK_STAY', 'NON_LODGING_SALE', 'NON_LODGING_NRR']
transactions
- array [Transaction]
orderNumber
- string
customer
- Customer
statuses
- OrderStatuses
parentId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ context
- ClientContext
id
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. attributes
- object
owner
- string
OrderAmounts
Attributes
taxTotal
- integer
subTotal
- integer
discountTotal
- integer
feeTotal
- integer
shippingTotal
- integer
netTotal
- integer
authorizedTotals
- TransactionAmounts
voidedTotals
- TransactionAmounts
capturedTotals
- TransactionAmounts
refundedTotals
- TransactionAmounts
savedTotals
- TransactionAmounts
currency
- string
OrderHistory
Attributes
adjustedOrder
- AdjustedOrder
event
- string, enum['ACCEPTED', 'EDITED', 'AWAITING_PICKUP', 'SHIPPED', 'DELIVERED', 'COMPLETED', 'CANCELLED']
id
- integer
timestamp
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ
OrderItem
Attributes
externalProductId
- string
fulfillmentInstruction
- string, enum['NONE', 'PICKUP_INSTORE', 'SHIP_TO']
categoryId
- string
clientNotes
- string
taxes
- array [OrderItemTax]
unitPrice
- integer
discount
- integer
fee
- integer
productId
- string
selectedVariants
- array [Variant]
unitOfMeasure
- string, enum['EACH', 'HOURS', 'DAYS', 'SECONDS', 'CRATE_OF_12', 'SIX_PACH', 'GALLON', 'LITRE', 'INCH', 'FOOT', 'MILLIMETER', 'CENTIMETER', 'METER', 'SQUARE_METER', 'CUBIC_METER', 'GRAM', 'KILOGRAM', 'POUND', 'ANNUAL', 'DEGREE_CELCIUS', 'DEGREE_FARENHEIT']
details
- string
tax
- integer
discounts
- array [Discount]
externalId
- string
quantity
- float
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ sku
- string
fees
- array [Fee]
serviceStartAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ serviceEndAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ status
- string, enum['ORDERED', 'FULFILLED', 'RETURNED']
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ name
- string
id
- integer
OrderItemTax
Attributes
taxRatePercentage
- double
taxRateFixedAmount
- integer
amountPrecision
- integer
externalId
- string
amount
- integer
id
- string
type
- string
OrderList
Attributes
OrderShipment
Attributes
estimatedDelivery
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ trackingUrl
- string
deliveredAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ fulfillAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ shippedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ pickupChannelId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. carrier
- string
trackingNumber
- string
deliveryMode
- string, enum['CURBSIDE', 'DELIVERY', 'DRIVE_THRU', 'FOR_HERE', 'PICKUP', 'SHIP', 'TO_GO']
shipmentType
- string, enum['FULFILLMENT', 'RETURN']
notes
- string
items
- array [OrderShipmentItem]
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ amount
- integer
status
- string, enum['NONE', 'AWAITING_PICKUP', 'IN_TRANSIT', 'DELIVERED', 'CANCELLED']
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ id
- integer
address
- Address
provider
- string
OrderShipmentItem
Attributes
quantity
- float
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ name
- string
id
- integer
OrderStatuses
Attributes
transactionStatusSummary
- string, enum['NONE', 'EXTERNALLY_PROCESSED', 'PENDING', 'COMPLETED', 'REFUNDED', 'CANCELED']
fulfillmentStatus
- string, enum['NONE', 'PARTIAL', 'FULFILLED']
status
- string, enum['OPENED', 'CANCELLED', 'COMPLETED']
Phone
Attributes
localPhoneNumber
- string
areaCode
- string
extensionNumber
- string
ituCountryCode
- string
primaryDayTime
- boolean
primaryEvening
- boolean
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ status
- string, enum['ADDED', 'CONFIRMED']
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ id
- integer
type
- string, enum['HOME', 'WORK', 'BUSINESS', 'MOBILE', 'FAX', 'PAGER', 'RECEIPT', 'OTHER']
PoyntLoyalty
Attributes
loyaltyId
- integer
loyalty
- array [PoyntLoyaltyCampaign]
reward
- array [PoyntLoyaltyReward]
externalId
- string
PoyntLoyaltyCampaign
Attributes
pointsRequired
- integer
totalPoints
- integer
points
- integer
campaignDescription
- string
totalVisits
- integer
totalSpend
- integer
lastIncrement
- integer
campaignName
- string
nextTier
- string
loyaltyUnit
- string
loyaltyType
- string
businessLoyaltyId
- integer
tier
- string
rewardDescription
- string
PoyntLoyaltyReward
Attributes
rewardId
- integer
preText
- string
postText
- string
businessLoyaltyId
- integer
newReward
- boolean
expireAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ status
- string
rewardDescription
- string
value
- integer
type
- string
ProcessorResponse
Attributes
processedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ scaResult
- string, enum['ENTER_PIN', 'INSERT_CARD', 'CDCVM']
adapterVariant
- string
batchAutoClosedByHost
- string
issuerResponseCode
- string
providerVerification
- ProviderVerification
interacMac
- string
echeckProcessor
- string, enum['MOCK', 'CHECK_COMMERCE']
remainingBalance
- integer
cvActualResult
- string
batchId
- string
retrievalRefNum
- string
ps2000Data
- string
debitResponseCode
- string
pinSessionKey
- string
adapterId
- string
processor
- string, enum['CHASE_PAYMENTECH', 'REDE', 'EVO', 'FIRST_DATA', 'GLOBAL_PAYMENTS', 'HEARTLAND_PAYMENT_SYSTEM', 'ELAVON', 'MERCURY', 'MONERIS', 'PAYPAL', 'ELAVON_MX', 'STRIPE', 'TSYS', 'VANTIV', 'WORLDPAY', 'EPX', 'WEPAY', 'MASHREQ', 'AXIS', 'KARTUKU', 'NEXI', 'DANA', 'MYNT', 'POYNT', 'NUVEI', 'NPAY', 'BRIDGEPAY', 'CONVERGE', 'MOCK', 'NA_BANCARD', 'CREDITCALL', 'ELAVON_EU', 'FUSEBOX', 'EVERTEC', 'GHL', 'RS2', 'JCN', 'PRISMA', 'VANTIV_EXPRESS', 'EZETAP', 'ADYEN', 'LETGO']
acquirer
- string, enum['CHASE_PAYMENTECH', 'REDE', 'EVO', 'FIRST_DATA', 'GLOBAL_PAYMENTS', 'HEARTLAND_PAYMENT_SYSTEM', 'ELAVON', 'MERCURY', 'MONERIS', 'PAYPAL', 'ELAVON_MX', 'STRIPE', 'TSYS', 'VANTIV', 'WORLDPAY', 'EPX', 'WEPAY', 'MASHREQ', 'AXIS', 'KARTUKU', 'NEXI', 'DANA', 'MYNT', 'POYNT', 'NUVEI', 'NPAY', 'BRIDGEPAY', 'CONVERGE', 'MOCK', 'NA_BANCARD', 'CREDITCALL', 'ELAVON_EU', 'FUSEBOX', 'EVERTEC', 'GHL', 'RS2', 'JCN', 'PRISMA', 'VANTIV_EXPRESS', 'EZETAP', 'ADYEN', 'LETGO']
approvedAmount
- integer
approvalCode
- string
avsResult
- AVSResult
cvResult
- string, enum['MATCH', 'NO_MATCH', 'NOT_PROCESSED', 'NO_CODE_PRESENT', 'SHOULD_HAVE_BEEN_PRESENT', 'ISSUER_NOT_CERTIFIED', 'INVALID', 'NO_RESPONSE', 'NOT_APPLICABLE']
statusMessage
- string
cardToken
- string
emvTags
- object
transactionId
- string
status
- string, enum['Successful', 'Failure']
statusCode
- string
Product
Attributes
author
- string
styleNumber
- string
relatedProducts
- array [ProductRelation]
possibleVariations
- array [Variation]
addonProducts
- array [ProductRelation]
availableDiscounts
- array [AvailableDiscount]
taxes
- array [Tax]
bundledProducts
- array [ProductRelation]
unitOfMeasure
- string, enum['EACH', 'HOURS', 'DAYS', 'SECONDS', 'CRATE_OF_12', 'SIX_PACH', 'GALLON', 'LITRE', 'INCH', 'FOOT', 'MILLIMETER', 'CENTIMETER', 'METER', 'SQUARE_METER', 'CUBIC_METER', 'GRAM', 'KILOGRAM', 'POUND', 'ANNUAL', 'DEGREE_CELCIUS', 'DEGREE_FARENHEIT']
externalId
- string
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ imageUrl
- array [string]
selectableVariants
- array [Variant]
shortCode
- string
price
- CurrencyAmount
sku
- string
brand
- string
releaseDate
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ inventory
- array [Inventory]
businessId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. storeId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. status
- string, enum['ACTIVE', 'RETIRED']
restrictionAttributes
- array [RestrictionAttribute]
templateOverrides
- array [string]
appliedRestrictions
- array [string]
productTemplateId
- string
msrp
- CurrencyAmount
avgUnitCost
- CurrencyAmount
ean
- string
upc
- string
isbn
- string
plu
- string
asin
- string
specification
- string
manufacturer
- string
publisher
- string
studio
- string
designer
- string
artist
- string
mpn
- string
modelNumber
- string
tags
- string
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ name
- string
id
- string
type
- string, enum['SIMPLE', 'BUNDLE']
variants
- array [Variant]
source
- string
description
- string
ProductList
Attributes
ProductRelation
Attributes
relatedProductId
- string
relatedProductSku
- array [string]
price
- CurrencyAmount
type
- string, enum['BUNDLE', 'RELATED', 'ADDON']
count
- integer
ProductSummary
Attributes
shortCode
- string
price
- CurrencyAmount
businessId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. name
- string
id
- string
ProductSummaryList
Attributes
products
- array [ProductSummary]
links
- array [Link]
ProductVariation
Attributes
value
- string
attribute
- string
ProviderVerification
Attributes
publicKeyHash
- string
signature
- string
QueryStatus
Attributes
stateChangeReason
- string
startedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ completedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ resourceUrl
- string
state
- string
ReceiptTemplate
Attributes
template
- string
id
- string
SelectableVariation
Attributes
valueMaxLength
- integer
cardinality
- string
values
- array [SelectableValue]
id
- string
attribute
- string
Store
Attributes
gatewayStoreId
- string
storeTerminalIds
- array [StoreTerminalId]
latitude
- float
longitude
- float
channelId
- string
defaultRetailChannelId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. defaultChannelId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. catalogId
- string
emailAddress
- string
phone
- Phone
acquirer
- string, enum['CHASE_PAYMENTECH', 'REDE', 'EVO', 'FIRST_DATA', 'GLOBAL_PAYMENTS', 'HEARTLAND_PAYMENT_SYSTEM', 'ELAVON', 'MERCURY', 'MONERIS', 'PAYPAL', 'ELAVON_MX', 'STRIPE', 'TSYS', 'VANTIV', 'WORLDPAY', 'EPX', 'WEPAY', 'MASHREQ', 'AXIS', 'KARTUKU', 'NEXI', 'DANA', 'MYNT', 'POYNT', 'NUVEI', 'NPAY', 'BRIDGEPAY', 'CONVERGE', 'MOCK', 'NA_BANCARD', 'CREDITCALL', 'ELAVON_EU', 'FUSEBOX', 'EVERTEC', 'GHL', 'RS2', 'JCN', 'PRISMA', 'VANTIV_EXPRESS', 'EZETAP', 'ADYEN', 'LETGO']
processorAdapterId
- string
timezone
- string
ventureId
- string
channelType
- string
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ echeckProcessor
- string, enum['MOCK', 'CHECK_COMMERCE']
governmentIds
- array [GovernmentId]
processorData
- object
storeDevices
- array [StoreDevice]
externalStoreId
- string
status
- string, enum['ACTIVE', 'DISABLED', 'REMOVED']
processor
- string, enum['CHASE_PAYMENTECH', 'REDE', 'EVO', 'FIRST_DATA', 'GLOBAL_PAYMENTS', 'HEARTLAND_PAYMENT_SYSTEM', 'ELAVON', 'MERCURY', 'MONERIS', 'PAYPAL', 'ELAVON_MX', 'STRIPE', 'TSYS', 'VANTIV', 'WORLDPAY', 'EPX', 'WEPAY', 'MASHREQ', 'AXIS', 'KARTUKU', 'NEXI', 'DANA', 'MYNT', 'POYNT', 'NUVEI', 'NPAY', 'BRIDGEPAY', 'CONVERGE', 'MOCK', 'NA_BANCARD', 'CREDITCALL', 'ELAVON_EU', 'FUSEBOX', 'EVERTEC', 'GHL', 'RS2', 'JCN', 'PRISMA', 'VANTIV_EXPRESS', 'EZETAP', 'ADYEN', 'LETGO']
subscribedBundles
- array [BundledFeatures]
supportedCardProducts
- array [CardProduct]
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ id
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. address
- Address
attributes
- object
displayName
- string
currency
- string
StoreDevice
Attributes
lastSeenAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ businessAgreements
- array [Entry]
channelId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. catalogId
- string
activatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ externalTerminalId
- string
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ processorData
- object
deviceId
- string
storeId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. status
- string, enum['CREATED', 'ACTIVATED', 'DEACTIVATED', 'REMOVED']
publicKeyVerification
- PublicKeyVerificationData
kekDetails
- array [DeviceKekData]
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ name
- string
type
- string, enum['TERMINAL', 'WIFI_SENSOR']
publicKey
- string
serialNumber
- string
Tax
Attributes
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ amount
- integer
rate
- double
businessId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ name
- string
id
- string
type
- string
description
- string
TaxList
Attributes
ThreeDSecureData
Attributes
cryptogram
- string
eci
- string
TokenResponse
Attributes
tokenType
- string, enum['BEARER']
accessToken
- string
refreshToken
- string
expiresIn
- integer
scope
- string
Transaction
Attributes
statementDescriptorSuffix
- string
partiallyApproved
- boolean
captureBy
- string
systemTraceAuditNumber
- string
notes
- string
stayType
- string, enum['GENERAL_CONTAINER', 'REGULAR_STAY', 'QUICK_STAY', 'NON_LODGING_SALE', 'NON_LODGING_NRR']
amountsAdjusted
- boolean
adjusted
- boolean
adjustmentHistory
- array [AdjustmentRecord]
intent
- string
emailReceipt
- boolean
receiptPhone
- Phone
receiptEmailAddress
- string
signatureCaptured
- boolean
pinCaptured
- boolean
reason
- TransactionReason
shippingAddress
- Address
poyntLoyalty
- PoyntLoyalty
links
- array [Link]
amounts
- TransactionAmounts
saveCardOnFile
- boolean
parentId
- string
voided
- boolean
authOnly
- boolean
settled
- boolean
processorResponse
- ProcessorResponse
action
- string, enum['AUTHORIZE', 'CAPTURE', 'VOID', 'OFFLINE_AUTHORIZE', 'REFUND', 'SALE', 'VERIFY']
approvalCode
- string
context
- ClientContext
processorOptions
- object
signature
- array [byte]
references
- array [TransactionReference]
fundingSource
- FundingSource
customerUserId
- integer
createdAt
- string
status
- string, enum['CREATED', 'SAVED', 'AUTHORIZED', 'PARTIALLY_CAPTURED', 'CAPTURED', 'DECLINED', 'PARTIALLY_CAPTURED_AND_PARTIALLY_REFUNDED', 'PARTIALLY_REFUNDED', 'REFUNDED', 'VOIDED', 'STEP_UP']
updatedAt
- string
signatureRequired
- boolean
partialAuthEnabled
- boolean
paymentTokenUsed
- boolean
chargebackStatus
- string, enum['CREATED', 'DISPUTED', 'MERCHANT_WON', 'MERCHANT_LOST']
transactionNumber
- string
customerLanguage
- string
processorTransactionId
- string
settlementStatus
- string
reversalVoid
- boolean
actionVoid
- boolean
mit
- boolean
id
- string
TransactionAmounts
Attributes
customerOptedNoTip
- boolean
transactionAmount
- integer
orderAmount
- integer
cashbackAmount
- integer
tipAmount
- integer
transactionFees
- array [FeeProgramFee]
currency
- string
TransactionList
Attributes
links
- array [Link]
transactions
- array [Transaction]
count
- integer
TransactionReason
Attributes
program
- string, enum['NO_SHOW', 'PURCHASE', 'CARD_DEPOSIT', 'DELAYED_CHARGE', 'EXPRESS_SERVICE', 'ASSURED_RESERVATION']
programFor
- array [string]
TransactionReceipt
Attributes
txnId
-
string
UUID version 1, 2, 3, or 4. All server generated values are version 4. data
- string
TransactionReference
Attributes
customType
- string
id
- string
type
- string, enum['POYNT_ORDER', 'POYNT_STAY', 'CUSTOM', 'CHANNEL']
UUID
Attributes
- leastSignificantBits
- integer
- mostSignificantBits
- integer
UserIdentity
Attributes
imageBase64
- string
externalId
- string
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ id
- string
provider
- string
UserCredential
Attributes
publicCredentialType
- string, enum['USERNAME', 'EMAIL', 'JWT', 'PAYPAL', 'PIN_ONLY', 'GODADDY', 'GODADDY_USERNAME', 'WORLDPAY_IQ_USERID']
privateCredentialValue
- string
privateCredentialSalt
- string
publicCredentialValue
- string
id
- integer
Variant
Attributes
selectableVariations
- array [SelectableVariation]
variations
- array [ProductVariation]
updatedAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ price
- CurrencyAmount
sku
- string
inventory
- array [Inventory]
upc
- string
createdAt
-
string
ISO 8601 format YYYY-MM-DDThh:mm:ssZ
Variation
Attributes
values
- array [string]
id
- string
attribute
- string
VerificationData
Attributes
additionalIdType
- string, enum['DRIVERS_LICENCE', 'EMAIL', 'PASSPORT', 'PHONE', 'NATIONAL_ID_CARD']
cvSkipReason
- string, enum['NOT_PRESENT', 'NOT_AVAILABLE', 'BYPASSED', 'ILLEGIBLE']
cvData
- string
pin
- string
additionalIdRefNumber
- string
threeDSecureData
- ThreeDSecureData
keySerialNumber
- string
cardHolderBillingAddress
- Address
VoidRequest
Attributes
context
- ClientContext
emvTags
- object
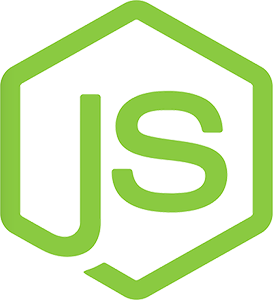
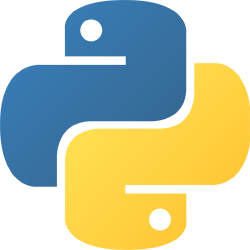
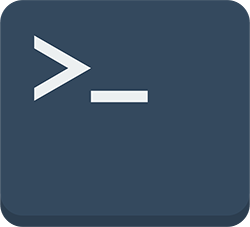