
# reCAPTCHA Integration
# General Overview
Poynt Collect currently uses reCAPTCHA; a well-known method to screen potential bots using your application when attempting to perform payments.
reCAPTCHA is enabled in Poynt Collect by default and cannot be disabled. Initially, we do not display the reCAPTCHA badge to ensure backward compatibility with existing integrations. However, Google mandates that the reCAPTCHA branding must always be visible in the user flow, making it a mandatory step in the integration process.
# Getting Started
# Enabling reCAPTCHA
To display the default reCAPTCHA badge, simply set the enableReCaptcha flag to true in the mount options when calling collect.mount
.
Code Sample:
const poyntCollect = document.createElement("script");
poyntCollect.src = "https://cdn.poynt.net/collect.js";
poyntCollect.async = true;
poyntCollect.onload = () => {
const collect = new TokenizeJs(
"business-uuid",
"urn:aid:application-uuid"
);
collect.mount("collect-container", document, {
...
enableReCaptcha: true,
});
};
document.head && document.head.appendChild(poyntCollect);
# Payment Form UI Verification
const poyntCollect = document.createElement("script");
poyntCollect.src = "https://cdn.poynt.net/collect.js";
poyntCollect.async = true;
poyntCollect.onload = () => {
const collect = new TokenizeJs(
"business-uuid",
"urn:aid:application-uuid"
);
collect.mount("collect-container", document, {
...
iFrame: {
width: "100%",
height: "400px", // <-- increase the height of the iFrame per your needs
border: "0px",
},
enableReCaptcha: true,
});
};
document.head && document.head.appendChild(poyntCollect);
# Example 1 - Small Payment Form
- Possible UI Issue:


- Posible Solution:
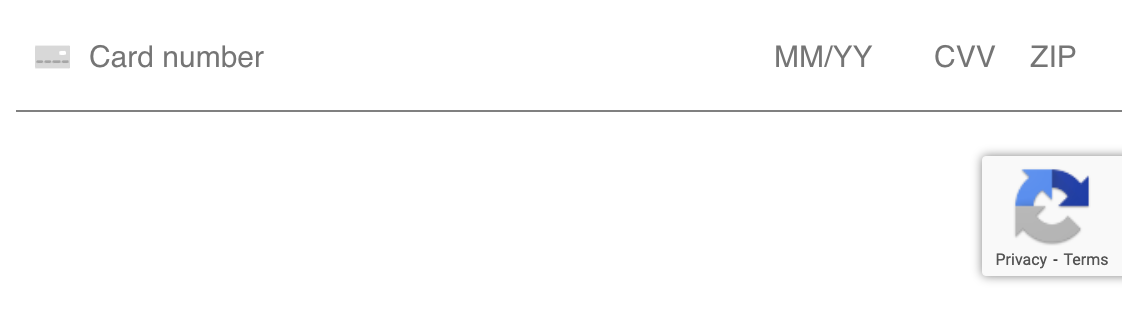
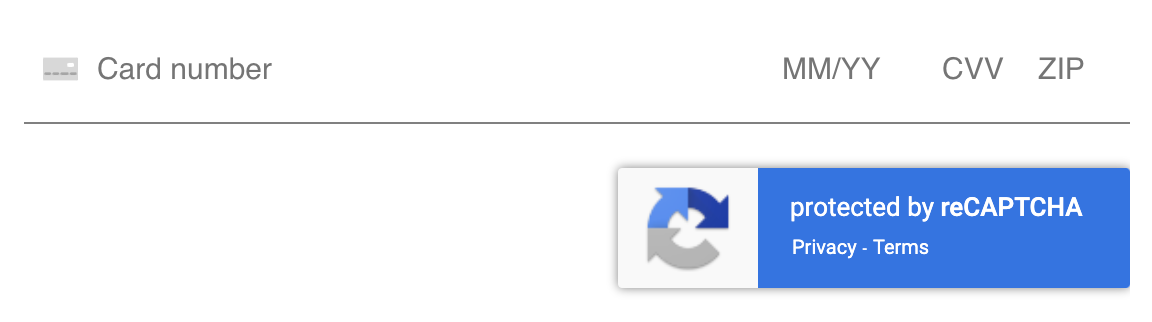
# Example 2 - Large Payment Form
- Possible UI Issue:
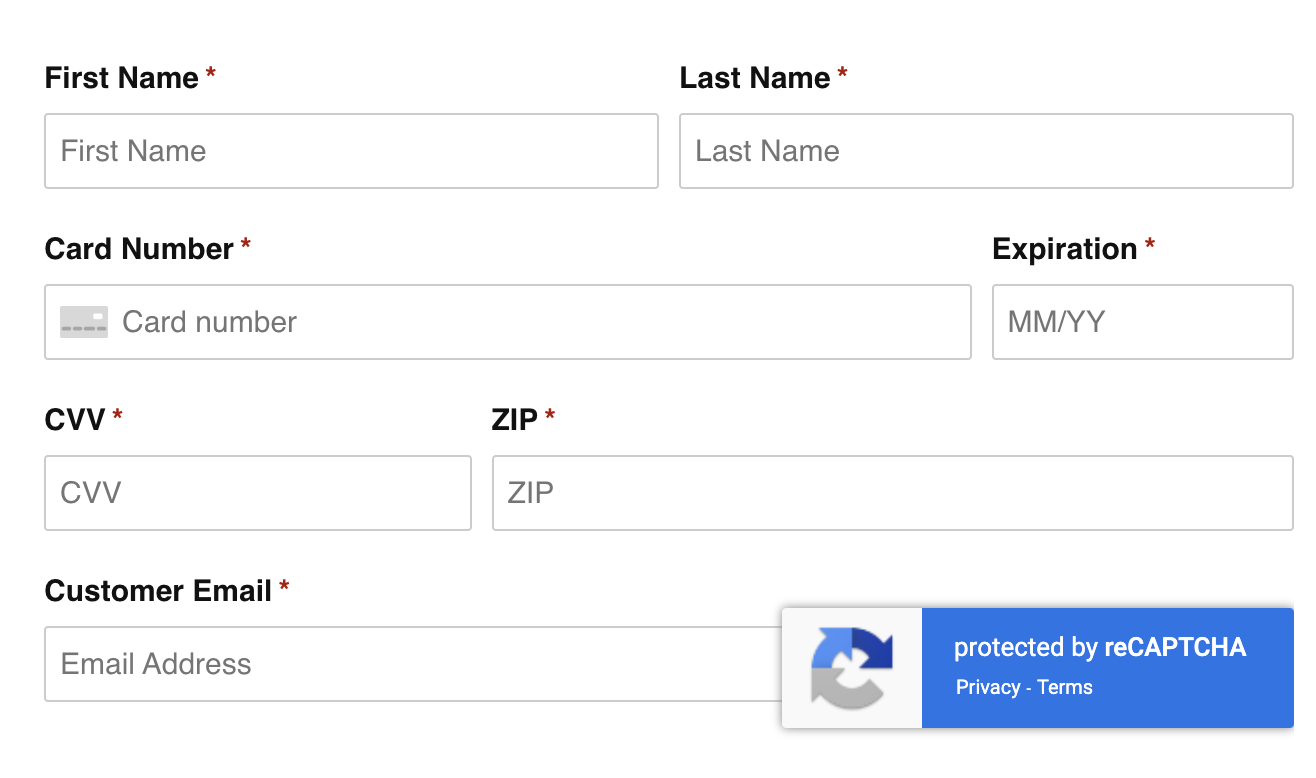
- Posible Solution:
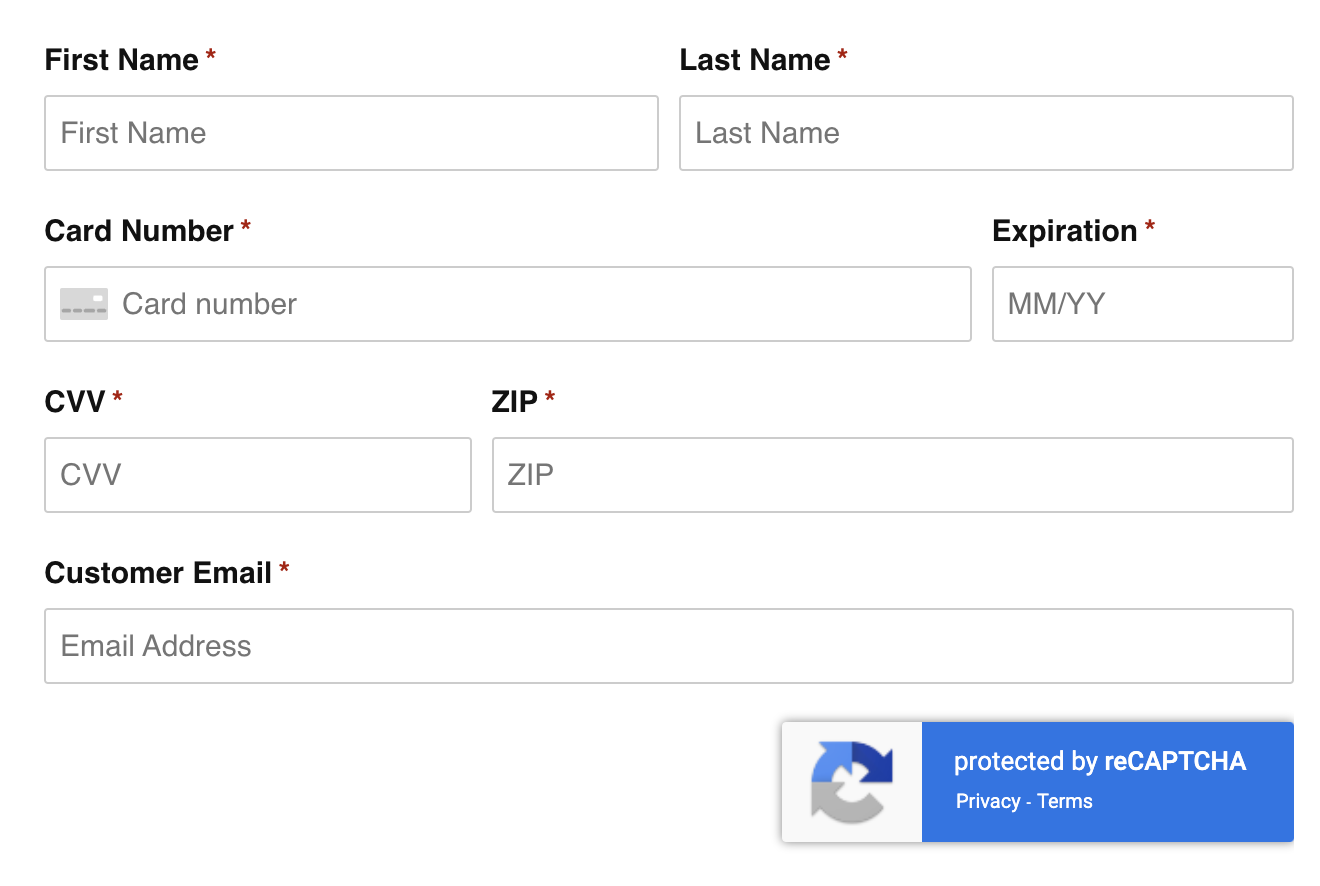
# reCAPTCHA Styling
If you would like to change the type of reCAPTCHA badge style, you also can pass a type parameter in reCaptchaOptions object in the mount options when calling collect.mount
.
Currently, Poynt Collect supports two types:
DEFAULT
(This type is used by default if no options are passed)
TEXT
TIP
If you use TEXT
type, you can also specify your own CSS properties to style the reCAPTCHA text, if needed. To do this, you can use the customCss
object in the mount options when calling collect.mount
.
Code Sample
const poyntCollect = document.createElement("script");
poyntCollect.src = "https://cdn.poynt.net/collect.js";
poyntCollect.async = true;
poyntCollect.onload = () => {
const collect = new TokenizeJs(
"business-uuid",
"urn:aid:application-uuid"
);
collect.mount("collect-container", document, {
...
enableReCaptcha: true,
reCaptchaOptions: {
type: "TEXT",
},
customCss: {
...
reCaptcha: {
text: {
"font-size": "20px",
"color": "black",
},
link: {
"text-decoration": "none",
"color": "red",
},
},
},
});
};
document.head && document.head.appendChild(poyntCollect);
Example 1 - DEFAULT
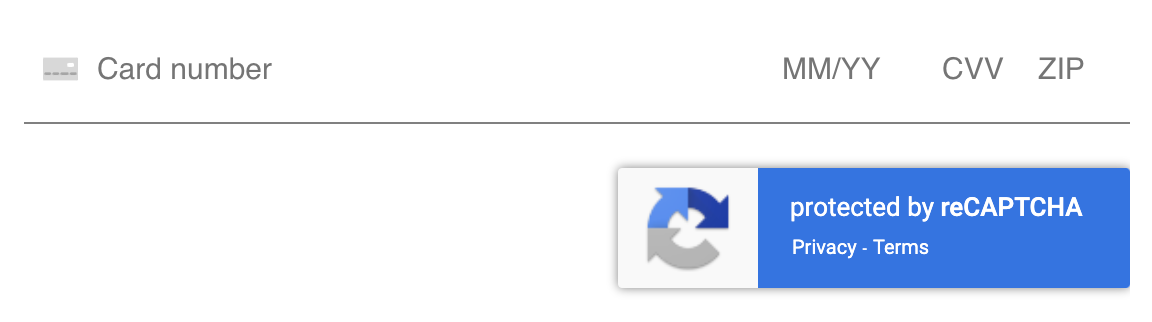
Example 2 - DEFAULT
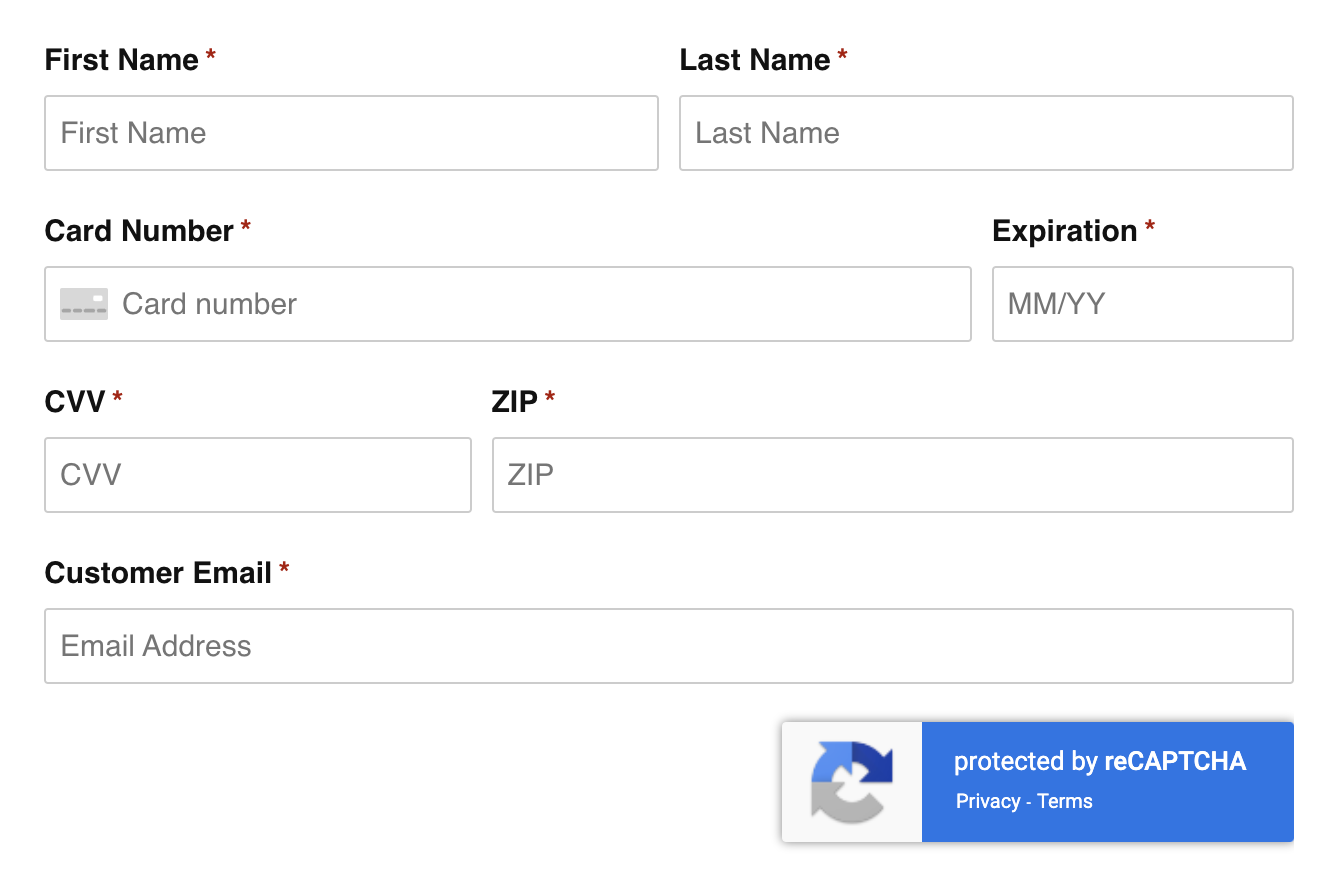
Example 1 - TEXT

Example 1 - TEXT
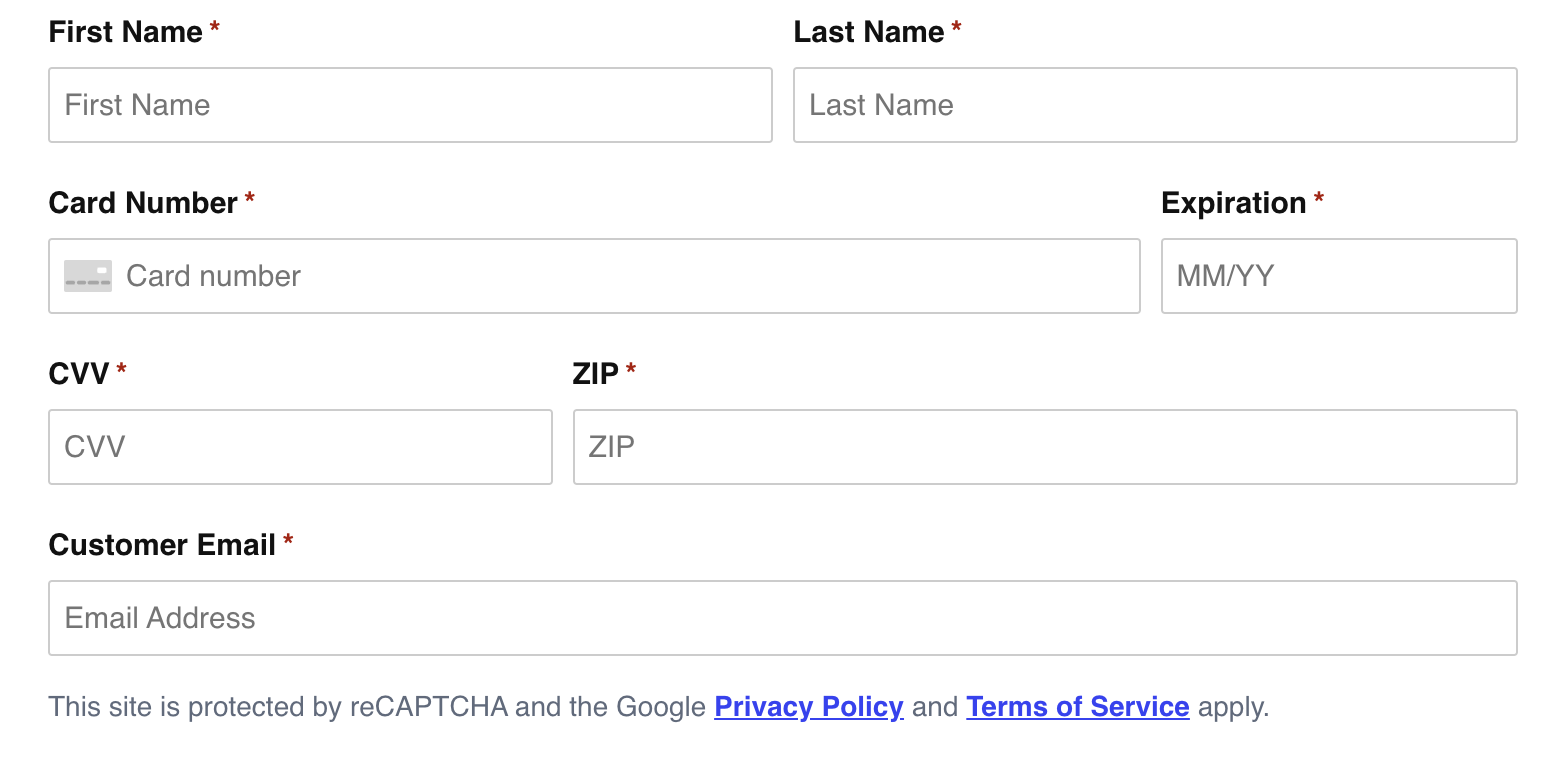
# Assesment Interpretation (Score)
reCAPTCHA assessments occur on our backend, behind the scenes. Currently, we automatically secure requests made to generate a nonce, so there are no additional actions required from the integration side.
# Types Definition
# Mount Option
interface IFrame {
width?: string;
height?: string;
border?: string;
borderRadius?: string;
boxShadow?: string;
};
interface ReCaptchaOptions {
type?: "DEFAULT" | "TEXT";
};
interface CustomCss {
...
reCaptcha?: {
text?: Record<string, string>;
link?: Record<string, string>;
};
};
interface MountOptions {
...
iFrame?: IFrame;
customCss?: CustomCss;
enableReCaptcha?: boolean;
reCaptchaOptions?: ReCaptchaOptions;
};