
# Subscriptions API Integration
In some cases, developer organizations and their apps also have a web application to increase visibility online as well as bringing in more subscribers to the App Center.
In order to integrate these new subscribers into the GoDaddy Poynt ecosystem, we have designed an API that allows developers to include a subscription route on their web application.
Please follow the steps outlined below to integrate the Subscriptions API on your web application.
# Prerequisites
# Fetching Plans
In order to provide GoDaddy Poynt merchants with the ability to subscribe to your app directly from your web application, you must connect with the billing system first to fetch your active billing plans.
To do this, you must create a JWT using your public/private key. After this, you will be able to use your JWT to create a new access token.
Once you have an access token, you can do a GET call to the endpoint to fetch the existing billing plans for your app.
Headers
- Content-Type: application/json'
- Authorization: Bearer {appAccessToken}' \
Query Parameters
- currency=USD - Optional
- businessId={businessId} - Optional
- status={status} - Optional
Sample Request
curl --location --request GET 'https://billing.poynt.net/organizations/{orgId}/apps/{appId}/plans' \
--header 'Authorization: Bearer {app_access_token}' \
--header 'Content-Type: application/json'
Sample Response
{
"list": [
{
"description": "plan test auth",
"scope": "BUSINESS",
"status": "ACTIVE",
"createdAt": "2022-11-27T23:33:51Z",
"updatedAt": "2022-11-27T23:33:51Z",
"appId": "urn:aid:39b5deb9-3bac-49de-8456-22c59415c470",
"startPolicy": "IMMEDIATE",
"amount": 1000,
"payer": "MERCHANT",
"planId": "5ebac9e0-a81d-413c-a61e-4337c993e309",
"trialPeriodDays": 10,
"amounts": [
{
"country": "US",
"currency": "USD",
"value": 1000
}
],
"cancelPolicy": "BILLING",
"interval": "MONTH",
"distributors": [],
"distributorPlan": false,
"merchantActionable": true,
"name": "Plan Teste Auth",
"type": "APP"
},
{
"description": "123123123123",
"scope": "BUSINESS",
"status": "ACTIVE",
"createdAt": "2022-12-08T17:44:43Z",
"updatedAt": "2022-12-08T17:44:43Z",
"appId": "urn:aid:39b5deb9-3bac-49de-8456-22c59415c470",
"startPolicy": "IMMEDIATE",
"amount": 2222,
"payer": "MERCHANT",
"planId": "ffaee414-6ab8-466c-a668-048c42406f49",
"trialPeriodDays": 22,
"amounts": [
{
"country": "US",
"currency": "USD",
"value": 2222
}
],
"cancelPolicy": "BILLING",
"interval": "MONTH",
"distributors": [],
"distributorPlan": false,
"merchantActionable": true,
"name": "123123123",
"type": "APP"
}
],
"start": 0,
"total": 2,
"count": 2
}
# Merchant Subscription
For merchants to subscribe to your application, you must configure the OAuth flow (opens new window) and add a URL Callback to redirect the merchant to the sign-in page for GoDaddy Poynt.
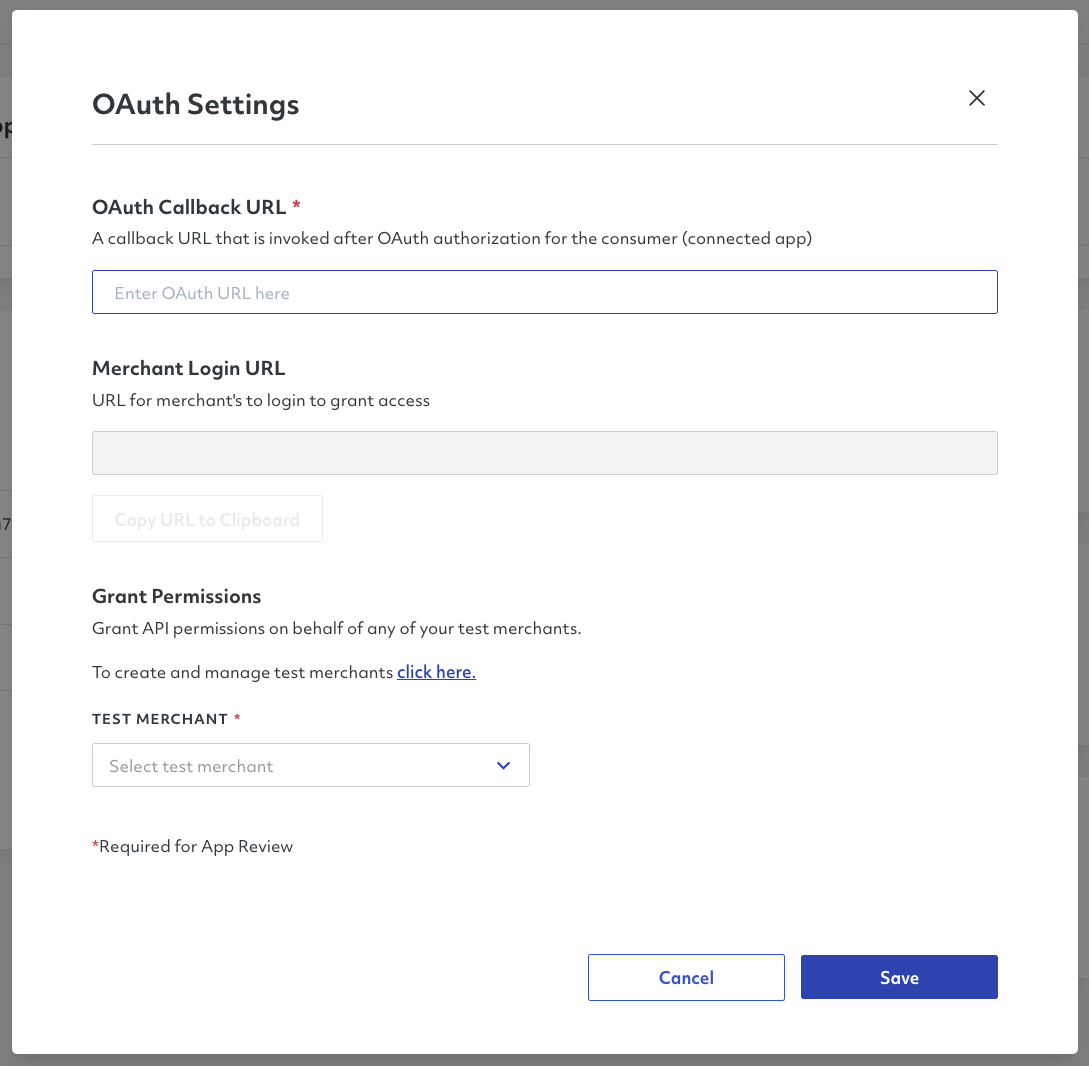
After the merchants grants the access and you receive the Authorization Code, you must create a Merchant Access Token that will allow you to subscribe on behalf of the merchant.
Once you have obtained the merchant authorization, you can use the POST endpoint to create, downgrade, and upgrade subscriptions on behalf of the merchant.
Headers
- Authorization: Bearer {merchantAccessToken} \
- Content-Type: application/json \
Sample Request
curl --location --request POST 'https://billing.poynt.net/organizations/{orgId}/apps/{appId}/subscriptions' \
--header 'Authorization: Bearer {merchant_access_token}' \
--header 'Content-Type: application/json' \
--data '{
"businessId": "5c51b42c-bdcf-42de-b986-06da00955e96",
"orgId": "25a81a4e-f76a-4e42-88fd-6d4890089600",
"planId": "ffaee414-6ab8-466c-a668-048c42406f49",
"replaceV2": false
"startAt": "YYY-MM-DDT19:41:24.649Z",
"addOns": [
{
"planId": "string",
"count": 0,
"action": "ADD",
"addOnId": "string"
}
],
"channelAttribution": "string"
}
TIP
If you would like to upgrade or downgrade the subscription, you must set the replaceV2 field to true
Sample Response
{
"scope": "BUSINESS",
"status": "ACTIVE",
"phase": "FULL",
"createdAt": "2023-03-30T04:26:39.716Z",
"updatedAt": "2023-03-30T04:26:39.716Z",
"bundleId": "f1bed559-376e-433e-97b8-91c7f76da127",
"subscriptionId": "9528a26d-410d-4ea4-9e49-f9c71ae610f5",
"appId": "urn:aid:39b5deb9-3bac-49de-8456-22c59415c470",
"payer": "MERCHANT",
"businessId": "5c51b42c-bdcf-42de-b986-06da00955e96",
"planId": "ffaee414-6ab8-466c-a668-048c42406f49",
"startAt": "2023-03-30T00:00:00Z",
"paymentStatus": "OVERDUE"
}
# Fetching Subscriptions
Once you are able to subscribe on behalf of the merchants, you can also list all the subscriptions for any of your applications using the GET endpoint.
Headers
- Authorization: Bearer {merchantAccessToken} \
- Content-Type: application/json
Query Parameters
- businessId={businessId}
- storeId={storeId} - Optional
- deviceId={deviceId} - Optional
- status={status} - Optional
Sample Request
curl --location --request GET 'https://billing.poynt.net/organizations/{orgId}/apps/{appId}/subscriptions?businessId={businessId}' \
--header 'Authorization: Bearer {merchant_access_token}' \
--header 'Content-Type: application/json'
Sample Response
{
"list": [
{
"scope": "BUSINESS",
"status": "ACTIVE",
"phase": "TRIAL",
"createdAt": "2023-03-29T16:02:47Z",
"updatedAt": "2023-03-29T16:02:47Z",
"bundleId": "90542ebf-076b-4cf4-961f-5a4c24bb2afc",
"subscriptionId": "6b1e433c-eaff-4b3f-a303-db893cebb0bb",
"appId": "urn:aid:39b5deb9-3bac-49de-8456-22c59415c470",
"payer": "MERCHANT",
"businessId": "5c51b42c-bdcf-42de-b986-06da00955e96",
"planId": "5ebac9e0-a81d-413c-a61e-4337c993e309",
"startAt": "2023-03-29T00:00:00Z",
"planName": "Plan Teste Auth"
},
{
"scope": "BUSINESS",
"status": "ACTIVE",
"phase": "FULL",
"createdAt": "2023-03-30T04:26:40Z",
"updatedAt": "2023-03-30T04:26:40Z",
"bundleId": "f1bed559-376e-433e-97b8-91c7f76da127",
"subscriptionId": "9528a26d-410d-4ea4-9e49-f9c71ae610f5",
"appId": "urn:aid:39b5deb9-3bac-49de-8456-22c59415c470",
"payer": "MERCHANT",
"businessId": "5c51b42c-bdcf-42de-b986-06da00955e96",
"planId": "ffaee414-6ab8-466c-a668-048c42406f49",
"startAt": "2023-03-30T00:00:00Z",
"planName": "123123123",
"paymentStatus": "PAID"
}
],
"start": 0,
"total": 2,
"count": 2
}
# Deleting Subscriptions
Finally, if you decide to delete one of the active subscriptions for a business, you can do so by using the DELETE endpoint as outlined below.
Headers
- Authorization: Bearer {merchant_access_token} \
- Content-Type: application/json
TIP
Please note that you must pass the Merchant Access Token as the Authorization header in your request to be able to delete a subscription.
Sample Request
curl --location --request DELETE 'https://billing.poynt.net/organizations/{orgId}/apps/{appId}/subscriptions/{subscriptionId}' \
--header 'Authorization: Bearer {merchant_access_token}' \
--header 'Content-Type: application/json'
Sample Response
If the request is processed successfully by the server, you will receive a 204 http code in the response but there will be no content in the payload.
NOTE
Please keep in mind we are no longer processing immediate cancellation refunds.
If a merchant decides to cancel their subscription, the cancellation will be effective at the end of the billing cycle, allowing them to use the application until the cancellation is effective.
To verify if a subscription has been deleted successfully, you can do a GET call for the subscriptions available for a specific business. You should see the endAt parameter show up for the subscription that was deleted.
An example of the response from the GET call would be as shown below.
{
"scope": "BUSINESS",
"status": "ACTIVE",
"phase": "FULL",
"bundleId": "5c06f5cb-b001-4264-a84c-5de8342948ed",
"planId": "ffaee414-6ab8-466c-a668-048c42406f49",
"businessId": "5c51b42c-bdcf-42de-b986-06da00955e96",
"appId": "urn:aid:39b5deb9-3bac-49de-8456-22c59415c470",
"subscriptionId": "de20ffaf-aff7-4486-93fa-8b11af55c758",
"planName": "123123123",
"createdAt": "2023-03-29T16:04:07Z",
"updatedAt": "2023-04-26T19:24:40Z",
"endAt": "2023-05-01T00:00:00Z",
"paymentStatus": "PAID",
"payer": "MERCHANT",
"startAt": "2023-03-29T00:00:00Z"
}