
# Billing Service Integration
Integrating GoDaddy Poynt's Billing Services into your application is relatively straightforward. However, the process of development and testing will require a special setup to make sure your application can handle all possible scenarios related to billing.
We highly recommend checking the development apps section first before writing your code to integrate with GoDaddy Poynt Billing. Additionally, make sure to read the app billing & monetization section to get more information on the subject.
Please follow the steps below to complete the integration with GoDaddy Poynt Billing:
# In-App Changes
In-App Billing services provide developers with an easy way to check the status of their subscriptions and request merchants to subscribe for other plans as necessary (See example bellow)
interface IPoyntInAppBillingService{
/**
* This method returns the current subscriptions for the calling app owned by the merchant.
* Result will be returned in {@link IPoyntInAppBillingServiceListener#onResponse}.
* @param packageName Package name of the call originator -
this is verified against the calling Uid provided by the android system.
* @param requestId Request id of the call originator.
* @param callback {@link IPoyntActivationServiceListener}
*/
void getSubscriptions( String packageName,
String requestId,
IPoyntInAppBillingServiceListener callback);
/**
* This method returns an intent that can be used to launch Poynt billing fragment to allow
a merchant to subscribe to your application.
* @param packageName Package name of the call originator - this is verified against the
calling Uid provided by the android system.
* @param extras bundle containing extra fields used to identify things like planId, etc.
* extras supported: plan_id
*
* @return Bundle containing a pending intent
*/
Bundle getBillingIntent( String packageName, in Bundle extras);
/**
* This method returns the current plans associated with the given packageName
* Result will be returned in {@link IPoyntInAppBillingServiceListener#onResponse}.
*
* @param packageName Package name of the call originator - this is verified against the
calling Uid provided by the android system.
* @param requestId Request id of the call originator.
* @param callback {@link IPoyntActivationServiceListener}
*/
void getPlans( String packageName,
String requestId,
IPoyntInAppBillingServiceListener callback);
}
# IPoyntInAppBillingService Integration
Integration with In-App Billing service in your application involves:
Creating your subscription plans.
When you upload your APK to the developer portal, you will be required to create a subscription plan.
Once the plans are approved, they will be accessible via the API. Below is a simple plan that charges $5.00 per month per business.
{
"name": "Basic Plan",
"amounts": [
{
"country": "US",
"currency": "USD",
"value": 500
}
],
"interval": "MONTH",
"scope": "BUSINESS"
}
TIP
You can create multiple subscription plans based on the structure of your application.
- Add the following permissions to your Android Manifest file so you can invoke the PoyntInAppBillingService.
...
<uses-permission android:name="com.poynt.store.BILLING" />
...
- Bind to PoyntInAppBillingService from your activity or service.
NOTE
You will need to have the corresponding "unbindService" call in the same activity or service.
Intent serviceIntent = new Intent("com.poynt.store.PoyntInAppBillingService.BIND");
serviceIntent.setPackage("com.poynt.store");
bindService(serviceIntent, mServiceConn, Context.BIND_AUTO_CREATE);
- Check the current subscriptions for the merchant. Please note that the merchant is inferred from the Smart Terminal Settings.
mBillingService.getSubscriptions("<your-package-name>", requestId,
new IPoyntInAppBillingServiceListener.Stub() {
@Override
public void onResponse(final String resultJson, final PoyntError poyntError, String requestId)
throws RemoteException {
if (poyntError != null) {
// handle errors
} else {
// resultJson is a json list of subscriptions
}
}
});
As an optional step, you can launch the In-App Billing fragment for additional subscriptions (Eg. upsell, additional services), which involves two steps:
5.1. Request a launch intent from
PoyntInAppBillingService
5.2. Launch the billing fragment using
'startIntentSenderForResult()'
.
private void launchBillingFragment() throws RemoteException {
Bundle bundle = new Bundle();
// add plan Id
bundle.putString("plan_id", "<your-plan-id>");
Bundle launchBundle = mBillingService.getBillingIntent(getPackageName(), bundle);
if (launchBundle != null && launchBundle.containsKey("BUY_INTENT")) {
PendingIntent intent = launchBundle.getParcelable("BUY_INTENT");
if (intent != null) {
try {
startIntentSenderForResult(
intent.getIntentSender(),
BUY_INTENT_REQUEST_CODE,
null,
Integer.valueOf(0),
Integer.valueOf(0),
Integer.valueOf(0));
} catch (IntentSender.SendIntentException e) {
e.printStackTrace();
// handle error - Failed to launch billing fragment!
}
} else {
// handle error - Did not receive buy intent!
}
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
// Check which request we're responding to
if (requestCode == BUY_INTENT_REQUEST_CODE) {
// Make sure the request was successful
if (resultCode == RESULT_OK) {
// Subscription request was successful - run Check Subscription to confirm!"
} else if (resultCode == RESULT_CANCELED) {
// Subscription request canceled
}
}
}
# Sample App
The PoyntSamples (opens new window) repository on GitHub contains a working sample of PoyntInAppBillingService.
TIP
Please refer to InAppBillingActivity.java (opens new window) for the billing service specific code.
Below are some screenshots of what you would see when you run the PoyntSamples application with your own packageName and Plans.
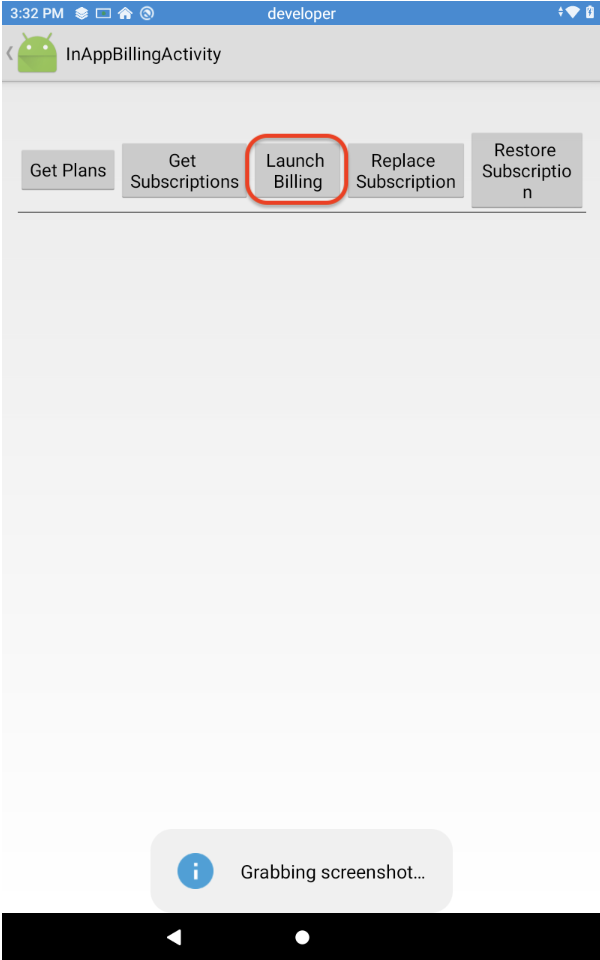
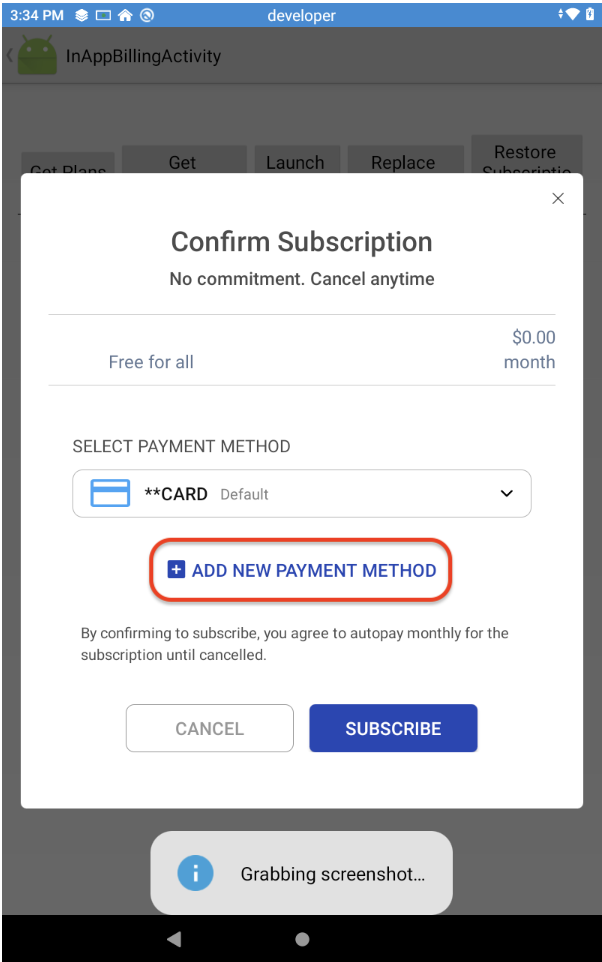
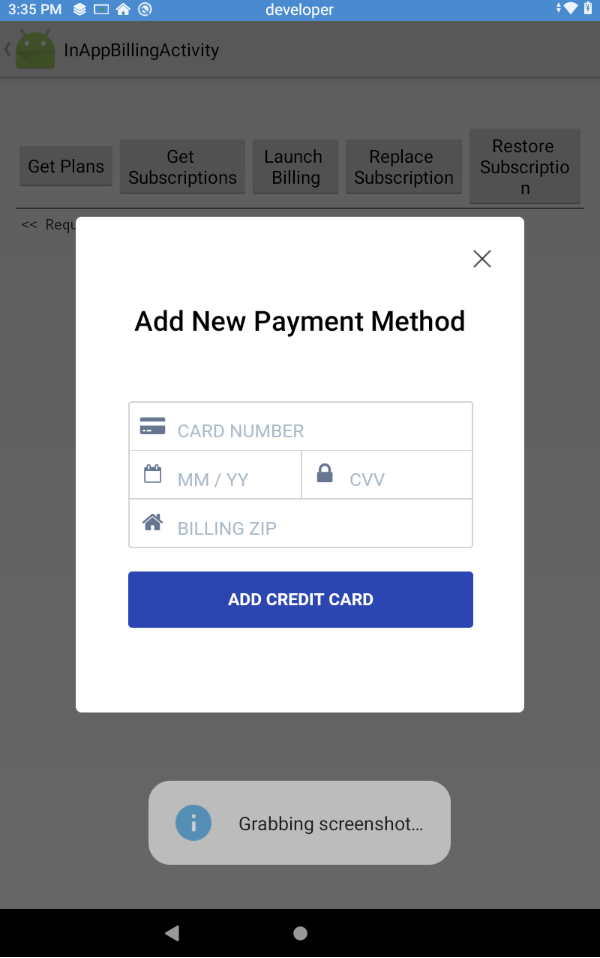
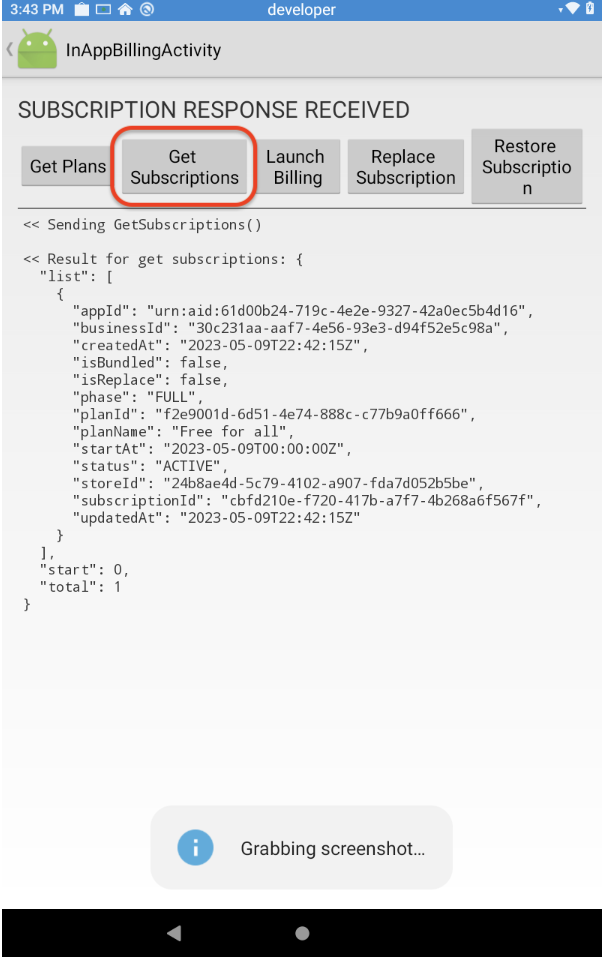
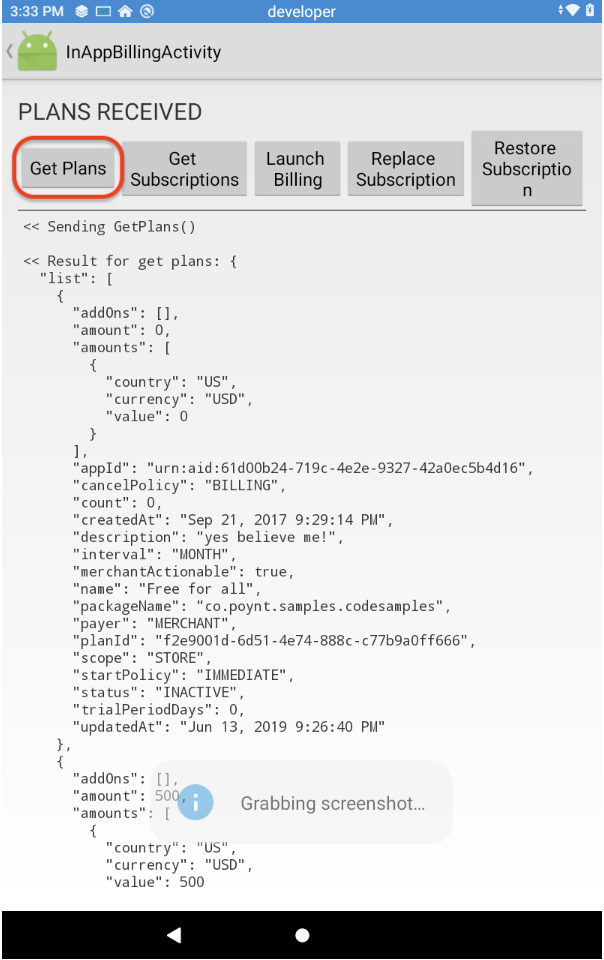
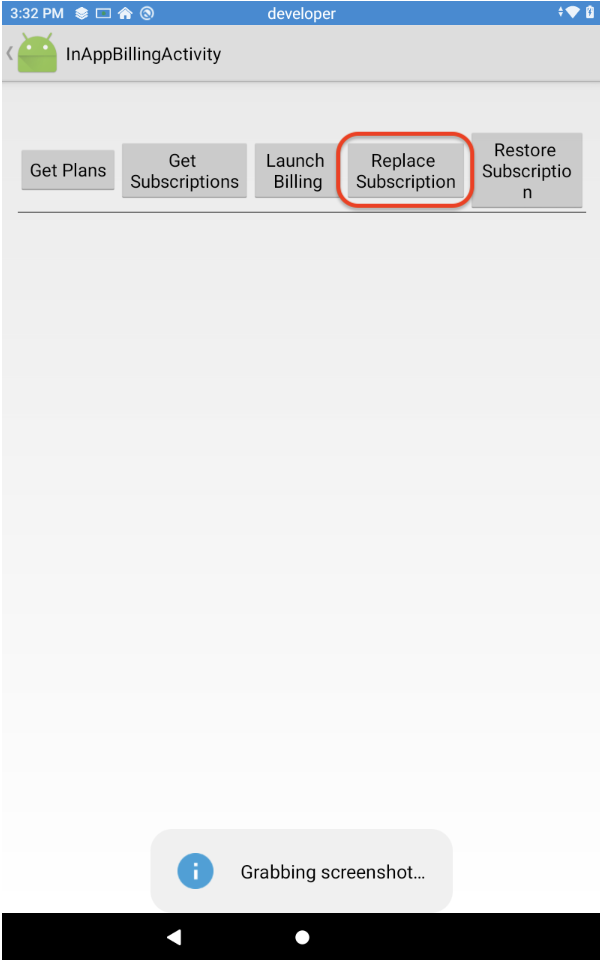
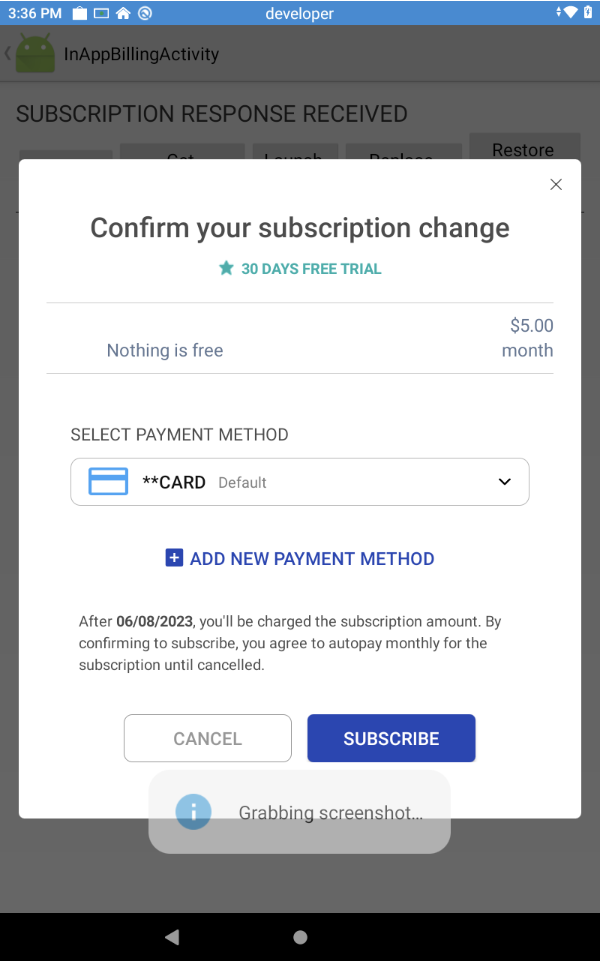