
# App Development Basics
Smart Terminal Apps are solutions that have direct interaction with merchants. They can collect real-time data or integrate with other accessories connected to the terminal.
GoDaddy Poynt Developers can build highly dynamic UIs for their applications as well as background services that run on GoDaddy Poynt Terminals using Poynt SDK for Android.
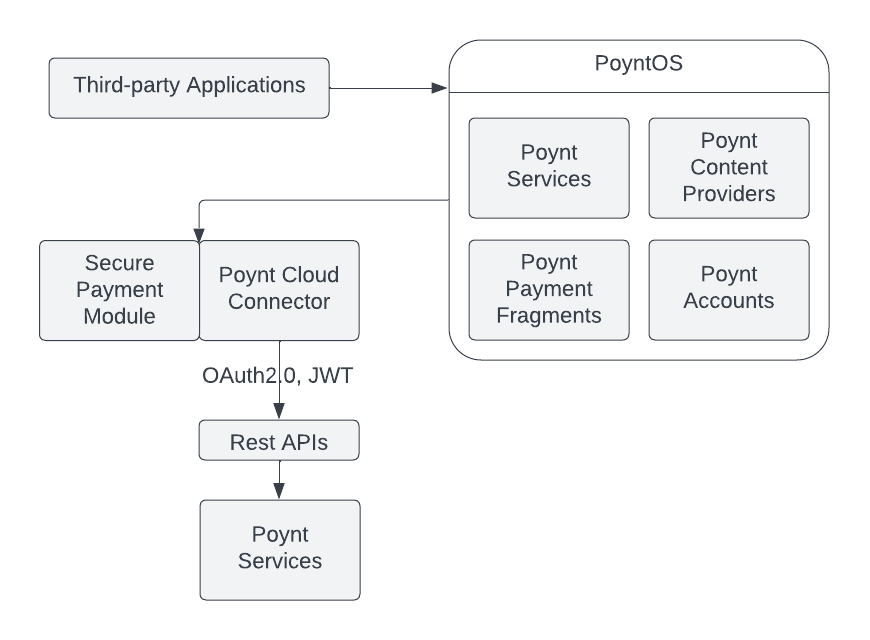
# App Requirements
One of the main functions of the Smart Terminal is payment processing. For this reason, there are a few security and compliance requirements that developers must abide by.
NOTE
Please keep in mind you should not include the card reader permissions poynt.permission.CARD_READER_SERVICE
or poynt.permission.CARD_READER
in your app manifest file.
Do not request access to sensitive information - All payments are securely processed by Poynt Services. The only information made available to third-party apps is the post-payment transaction information.
There are limited elements you can display on the client-facing screen - This is limited to what Poynt exposes as part of the SDK. However, there are multiple methods in the
IPoyntSecondScreenService
to scan QR codes, request customer contact information (email, phone), provide tips, etc.Application and service sideloading are not allowed - Sideloading is not authorized regardless even through the
adb
or downloaded via the internet.The Android Debug Bridge (adb) is disabled on live merchant Smart Terminals.
Access to custom USB devices must be approved directly - Any access to custom USB devices from your applications must be approved by GoDaddy Poynt during the review process and by the Merchant at the time of installation.
Applications must be compatible with PST3 Devices - For apps to be displayed and featured in the App Center, they must be compatible with the device.
TIP
Please refer to the section for app compatibility for PST3 to learn more about this topic.
Application review & approval is mandatory - All applications must be reviewed and distributed by GoDaddy Poynt through a secure channel.
NOTE
Please remember to integrate crash reporting on your terminal app to help you identify, track, and fix issues. You can use Firebase Crashlytics or any other crash reporting tool of your choice.
This integration must be completed before submitting your application for review.
# General Concepts
Developing applications for GoDaddy Poynt is very similar to the application development process with Android. This means you will have access to the standard development tools available at developer.android.com (opens new window).
However, our Smart Terminals run on a customized version of Android 4.4.4 (KitKat); therefore, it is ideal to set the minSdkVersion to Android API Level 21.
We recommend using Android Studio with the Gradle Build Toolkit with a screen resolution of 800x1280.
TIP
We highly recommend the developer edition of the Smart Terminal to perform comprehensive testing and successful implementations to avoid any incompatibility issues when releasing your application to the App Center.
Developer editions also have the adb
enabled so you can quickly test applications in your development environment.
# Poynt SDK
Poynt SDK for Android provides developers with all the necessary interfaces and help classes to access Poynt Services and data stored on the device and in the cloud. It can also help initiate the secure payment process from your applications if necessary.
If you wish to learn more about Poynt SDK, please refer to the GoDaddy Poynt SDK section to go into detail about usage and characteristics.
# Poynt Services
Poynt Services are a series of actions that provide the core functionalities exposed as AIDL services for third-party applications. These services include the following:
Poynt Transaction Service (opens new window) - Provides the payment's transactional information (status, amounts, etc.)
Poynt Order Service (opens new window) - Provides the order management APIs.
Poynt Business Service (opens new window) - Provides the information about the merchant’s business, stores, employees, etc.
Poynt Customer Service (opens new window) - Provides the necessary APIs to search and manage the merchant's customers.
Poynt Product Service (opens new window) - Provides the necessary APIs to get the product catalog for the merchant.
Poynt Receipt Printing Service (opens new window) - Includes the APIs to print receipts for payment transaction and/or orders.
Poynt Second Screen Service (opens new window) - Includes the APIs to request content to be displayed in the consumer screen.
Poynt Session Service (opens new window) - Provides the APIs to obtain current logged in business user (merchant) information.
Poynt Authenticator (opens new window) - Provides business user (merchant) login/authentication using Android’s Account Manager API.
All the AIDL files required to generate the service stubs through Android SDK are bundled with the Poynt SDK. Please refer to calling an IPC method (opens new window) in the Android developer documentation to learn how to use AIDL services.
# Poynt Content Providers
Poynt Content Providers offer data storage for different resources related to merchant information. These content providers include business, products, transactions, orders, and customers, among others, through the standard Android Content Providers (opens new window) interface.
All the Poynt Content Provider interfaces are delivered through the SDK. They can also be used with the standard android widgets that support content resolver integration (e.g., Lists).
# Poynt Payment Fragments
GoDaddy Poynt provides Payment Fragments that third-party applications can launch to collect payment card information securely and successfully process transactions.
The Payment Fragments can be launched by delivering a ‘Payment’ object containing transactional information like amounts, tips, and reference identifiers.
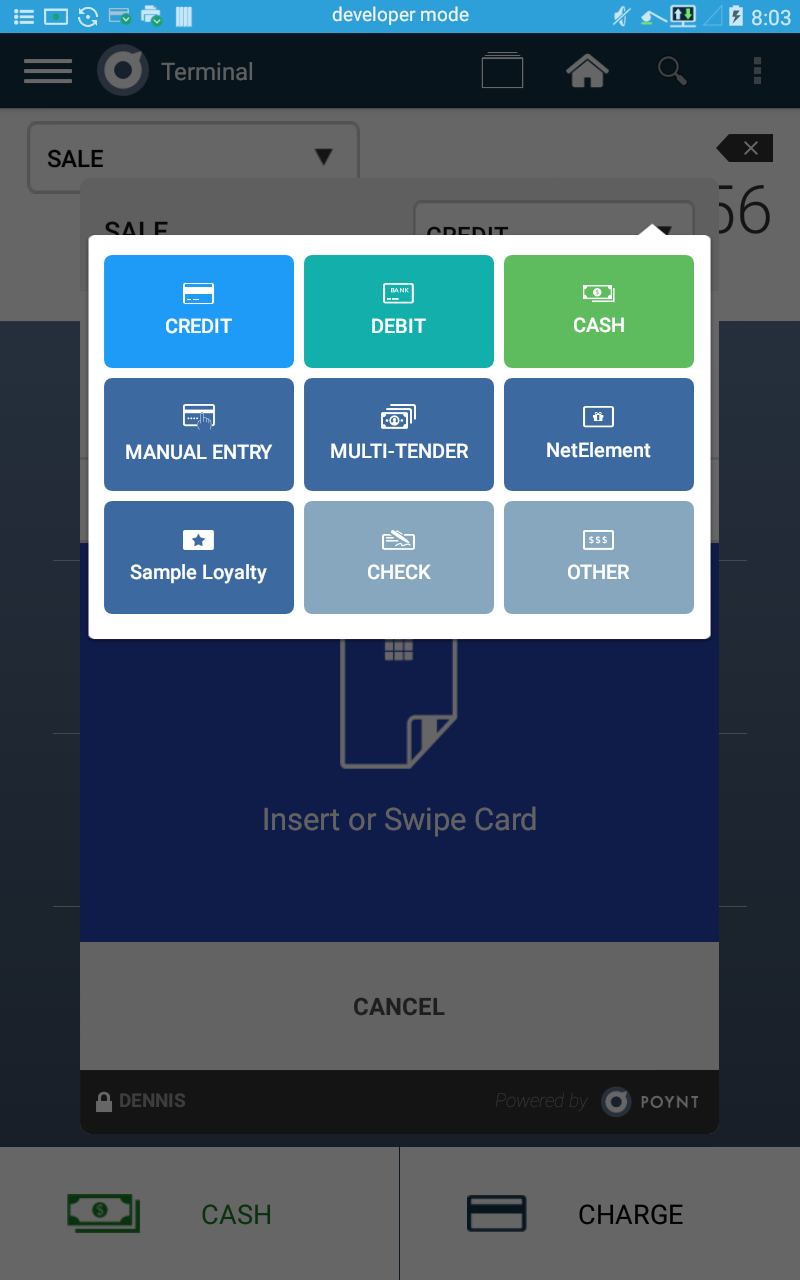
Currently, there are three types of Payment Fragments shown through GoDaddy Poynt SDKs:
Payment - This fragment provides the necessary interface and callbacks to process a single transaction. Once a transaction is processed, the transactional information is passed back through the callback interface.
MultiTender Payment - This fragment provides the necessary interface and callbacks to process several payment transactions using different methods like cash, credit, debit card, etc., and split the amounts among them (auto-split or custom).
Display Transactions - This fragment provides the necessary interface to display the transactional information for a given transaction ID or a reference ID. This ID is usually linked to one or more transactions.
Merchants can also capture a pending transaction, void an authorization, or refund a processed transaction from the Display Transactions fragment.
When an application launches the Payment Fragments, the consumer-facing screen is taken over by the Payment Fragments to collect and process the payment securely.
# GoDaddy Poynt Intents
Intents refer to a standard form of cross-application notifications in AndroidOS. Poynt Services broadcast various events (opens new window) as implicit intents related to payments, orders, and other activities on the smart terminal in real-time. Ultimately, these events can be received by applications and services.
Inside the Javadocs (opens new window) you will find a complete list of Intent Actions defined in the PoyntOS platform.
Poynt Intents are broadcasted in addition to the common intents shown by the Android system. You can refer to the Intents and Intent Filters for more information on how to handle intents programmatically or through the Android Manifest file.
TIP
We also promote the broadcasting of useful events to other apps running on the same terminal. In the long run, this will help us build an integrated experience for the merchant.
# Poynt Register Intents
During their daily operations, merchants can add or remove items from the Poynt Register application. These actions broadcast several intents. Below are some of the most common intents so that you can respond to these actions.
ADD_PRODUCT_TO_CART
Whenever a single item is added to an order, the following intent is broadcasted.
poynt.intent.action.ADD_PRODUCT_TO_CART
The following values are available as Intent extras.
- order_id
- the order id (STRING)
- product_name
- OPTIONAL
- the name of the order item, if available (STRING)
- product_id:
- OPTOINAL
- the product id, if available (STRING)
- product_sku:
- OPTIONAL
- the sku of the product, if available (STRING)
- product_quantity
- OPTIONAL
- the quantity of the product that was added (FLOAT)
REMOVE_PRODUCT_FROM_CART
Whenever a single item is removed from an order, the following intent is broadcasted.
poynt.intent.action.REMOVE_PRODUCT_FROM_CART
- order_id
- the order id (STRING)
- product_name
- OPTIONAL
- the name of the order item, if available (STRING)
- product_id:
- OPTOINAL
- the product id, if available (STRING)
- product_sku:
- OPTIONAL
- the sku of the product, if available (STRING)
TIP
If you want to implement other intents, check out our complete list of PoyntOS SDK Broadcast Intents (opens new window)