
# Poynt Payment Fragments
Poynt Payment Fragments provide a secure and consistent payment experience for merchants and consumers across all applications running on GoDaddy Poynt Smart Terminals.
Processing payment transactions within the PoyntOS level means that your applications do not need to worry about handling multiple payment methods, payment processing, and compliance regulations (e.g. PCI Compliance).
Currently, Payment Fragments support the following features:
- Payments using different payment card interfaces (MSR, EMV, NFC, etc.)
- Cash payments
- Tips, receipt options, and signature collection
- Collection of secure pins for chips and pin cards (EMV)
- Multi-tenders (aka split payments)
- Non-referenced credits and auth-only transactions
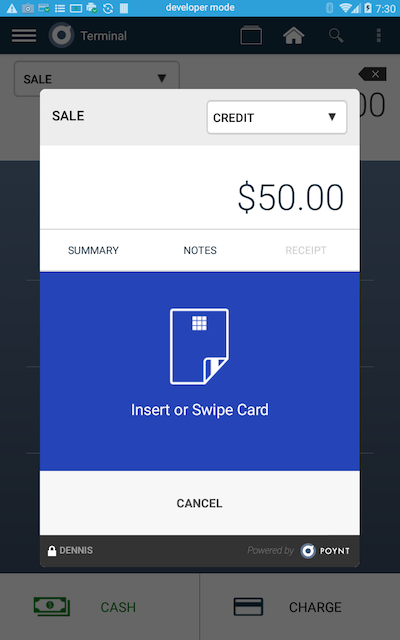
# Integration
When an application needs to collect a payment, it can launch the Payment Fragments using the Poynt Payment Activity.
To launch the payment activity, you will have to create a Payment object and specify the total amount to be collected, the currency, and an optional reference ID. The Payment activity has to be launched with the Payment object using the standard android 'startActivityForResult()' method.
The Payment Activity will walk both the merchant and consumer through the payment flows (payment method entry and consumer authorization).
Once a payment is processed successfully, the fragment will return an updated payment object with the payment status and processed transaction, including the redacted payment card information that you can use to display in your application.
Payment payment = new Payment();
String referenceId = UUID.randomUUID().toString();
payment.setReferenceId(referenceId);
payment.setAmount(amount);
payment.setCurrency(currencyCode);
// start Payment activity for result
try {
Intent collectPaymentIntent = new Intent(Intents.ACTION_COLLECT_PAYMENT);
collectPaymentIntent.putExtra(Intents.INTENT_EXTRAS_PAYMENT, payment);
startActivityForResult(collectPaymentIntent, COLLECT_PAYMENT_REQUEST);
} catch (ActivityNotFoundException ex) {
Log.e(TAG, "Poynt Payment Activity not found - did you install PoyntServices?", ex);
}
To handle the response from the Payment Fragments, you will need to override the 'onActivityResult()' method and process the response.
@Override
public void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
// Check which request we're responding to
if (requestCode == COLLECT_PAYMENT_REQUEST) {
// Make sure the request was successful
if (resultCode == Activity.RESULT_OK) {
if (data != null) {
Payment payment = data.getParcelableExtra(Intents.INTENT_EXTRAS_PAYMENT);
Log.d(TAG, "Received onPaymentAction from PaymentFragment w/ Status("
+ payment.getStatus() + ")");
if (payment.getStatus().equals(PaymentStatus.COMPLETED)) {
Toast.makeText(this, "Payment Completed", Toast.LENGTH_LONG).show();
} else if (payment.getStatus().equals(PaymentStatus.AUTHORIZED)) {
Toast.makeText(this, "Payment Authorized", Toast.LENGTH_LONG).show();
} else if (payment.getStatus().equals(PaymentStatus.CANCELED)) {
Toast.makeText(this, "Payment Canceled", Toast.LENGTH_LONG).show();
} else if (payment.getStatus().equals(PaymentStatus.FAILED)) {
Toast.makeText(this, "Payment Failed", Toast.LENGTH_LONG).show();
} else if (payment.getStatus().equals(PaymentStatus.REFUNDED)) {
Toast.makeText(this, "Payment Refunded", Toast.LENGTH_LONG).show();
} else if (payment.getStatus().equals(PaymentStatus.VOIDED)) {
Toast.makeText(this, "Payment Voided", Toast.LENGTH_LONG).show();
} else {
Toast.makeText(this, "Payment Completed", Toast.LENGTH_LONG).show();
}
}
} else if (resultCode == Activity.RESULT_CANCELED) {
Toast.makeText(this, "Payment Canceled", Toast.LENGTH_LONG).show();
}
}
}
TIP
If you would like to see these steps in action, please refer to the step by step guide on Building a POS Application
# Mock Amounts
Mock amounts are designed to register the calls they receive. You can use them to verify that all expected actions were performed without invoking production code and to verify that the intended code was successfully executed.
Below is a list of mock accounts for Payment Fragments.
Amount | Response |
---|---|
47.41 | Partial approval for 42.41 |
47.71 | Authorization or Sale declined |
47.72 | Void declined |
47.73 | Refund declined |
47.74 | Capture declined |
47.75 | Cancellation declined |
# Post-Payment Actions
Oftentimes, it is necessary to provide the merchants with the ability to execute different actions once a payment has been processed.
These include payment actions like voiding, capturing, refunding, and getting details. These actions are provided by Payment Fragments through the "DISPLAY_PAYMENT" intent.
// start Payment activity to display transaction details
try {
Intent displayPaymentIntent = new Intent(Intents.ACTION_DISPLAY_PAYMENT);
displayPaymentIntent.putExtra(Intents.INTENT_EXTRAS_TRANSACTION_ID, transactionId);
startActivityForResult(displayPaymentIntent, DISPLAY_PAYMENT_REQUEST);
} catch (ActivityNotFoundException ex) {
Log.e(TAG, "Poynt Payment Activity not found - did you install PoyntServices?", ex);
}
This will launch Poynt Payment Activity to display the details of the payment along with the supported actions (void, capture, refund) based on its current status.
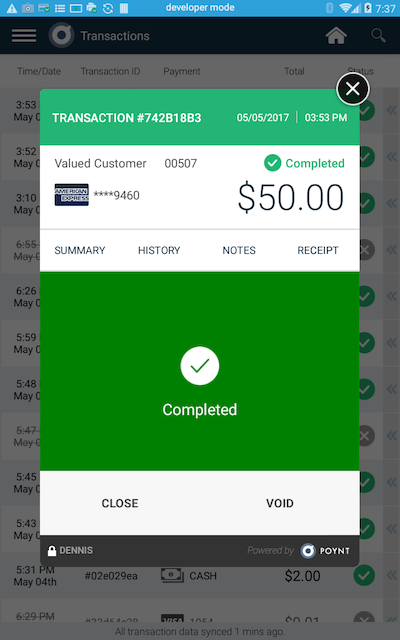